수신기 유형에 관계없이 Go에서 인터페이스의 메서드를 동적으로 호출하는 방법은 무엇인가요?{}
수신기 유형에 관계없이 인터페이스에서 동적으로 메서드 호출{}
Go의 템플릿 시스템 영역에서는 리플렉션이 중요한 역할을 합니다. 다양한 수신기 유형이 있는 인터페이스에서 메서드를 동적으로 호출할 때 문제가 발생합니다.{} 이는 알려진 유형과 원활하게 작동하지만 데이터가 인터페이스 내에 래핑되면 실패합니다{}.
문제 설명
다음을 고려하세요. 코드:
type Test struct { Start string } func (t *Test) Finish() string { return t.Start + "finish" } func Pass(i interface{}) { _, ok := reflect.TypeOf(&i).MethodByName("Finish") if ok { fmt.Println(reflect.ValueOf(&i).MethodByName("Finish").Call([]reflect.Value{})[0]) } else { fmt.Println("Pass() fail") } } func main() { i := Test{Start: "start"} Pass(i) _, ok := reflect.TypeOf(&i).MethodByName("Finish") if ok { fmt.Println(reflect.ValueOf(&i).MethodByName("Finish").Call([]reflect.Value{})[0]) } else { fmt.Println("main() fail") } }
관찰
- 데이터가 알려진 유형(main())인 경우 동적 메서드 호출이 성공합니다.
- 데이터가 인터페이스(Pass())에 래핑되면 실패하고 첫 번째에서 "Pass() 실패"를 반환합니다. call.
문제
문제는 인터페이스에 래핑된 데이터의 주소에 액세스하는 데 있습니다{}. 일반적으로 포인터를 가리키는 &i 사용은 이 시나리오에서는 작동하지 않습니다.
해결책
이 문제를 해결하려면 가능한 모든 시나리오를 처리해야 합니다.
-
인터페이스{} 데이터를 값으로, 메서드 수신자로 value:
- 데이터가 값인 경우 해당 값에 대한 포인터를 만듭니다.
-
인터페이스{} 데이터를 포인터로서의 값 및 메소드 수신자:
- 위와 같이 데이터에 대한 포인터.
-
인터페이스{} 데이터는 포인터로, 메서드 수신자는 값으로:
- 데이터가 포인터인 경우 해당 데이터가 가리키는 값을 검색합니다. to.
-
interface{} 데이터를 포인터로, 메서드 수신자를 포인터로 사용:
- 포인터 사용
구현
이 논리를 기반으로 메소드를 동적으로 호출하는 일반화된 함수를 생성할 수 있습니다.
func CallMethod(i interface{}, methodName string) interface{} { // Handle all scenarios var ptr, value, finalMethod reflect.Value value = reflect.ValueOf(i) if value.Type().Kind() == reflect.Ptr { ptr = value value = ptr.Elem() } else { ptr = reflect.New(reflect.TypeOf(i)) temp := ptr.Elem() temp.Set(value) } // Check for method on value and pointer method := value.MethodByName(methodName) if method.IsValid() { finalMethod = method } method = ptr.MethodByName(methodName) if method.IsValid() { finalMethod = method } // Call the method if (finalMethod.IsValid()) { return finalMethod.Call([]reflect.Value{})[0].Interface() } // Method not found return "" }
이제 이 함수를 사용하면 수신기 유형 및 인터페이스에 관계없이 동적으로 메서드를 호출할 수 있습니다.{} 래퍼:
i := Test{Start: "start"} j := Test{Start: "start2"} fmt.Println(CallMethod(i, "Finish")) // Output: startfinish fmt.Println(CallMethod(&i, "Finish")) // Output: startfinish fmt.Println(CallMethod(i, "Another")) // Output: fmt.Println(CallMethod(&i, "Another")) // Output: start2another fmt.Println(CallMethod(j, "Finish")) // Output: startfinish fmt.Println(CallMethod(&j, "Finish")) // Output: start2finish fmt.Println(CallMethod(j, "Another")) // Output: fmt.Println(CallMethod(&j, "Another")) // Output: start2another
위 내용은 수신기 유형에 관계없이 Go에서 인터페이스의 메서드를 동적으로 호출하는 방법은 무엇인가요?{}의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
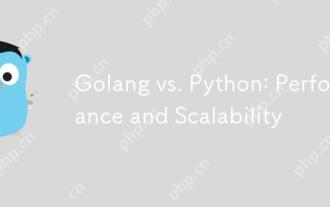
Golang은 성능과 확장 성 측면에서 Python보다 낫습니다. 1) Golang의 컴파일 유형 특성과 효율적인 동시성 모델은 높은 동시성 시나리오에서 잘 수행합니다. 2) 해석 된 언어로서 파이썬은 천천히 실행되지만 Cython과 같은 도구를 통해 성능을 최적화 할 수 있습니다.
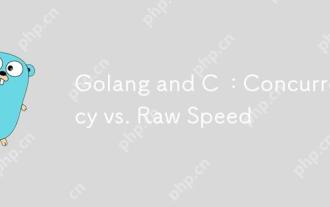
Golang은 동시성에서 C보다 낫고 C는 원시 속도에서 Golang보다 낫습니다. 1) Golang은 Goroutine 및 Channel을 통해 효율적인 동시성을 달성하며, 이는 많은 동시 작업을 처리하는 데 적합합니다. 2) C 컴파일러 최적화 및 표준 라이브러리를 통해 하드웨어에 가까운 고성능을 제공하며 극도의 최적화가 필요한 애플리케이션에 적합합니다.
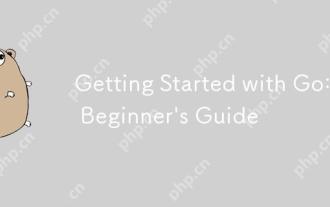
goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity, 효율성, 및 콘크리 론 피처
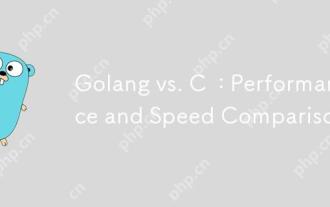
Golang은 빠른 개발 및 동시 시나리오에 적합하며 C는 극도의 성능 및 저수준 제어가 필요한 시나리오에 적합합니다. 1) Golang은 쓰레기 수집 및 동시성 메커니즘을 통해 성능을 향상시키고, 고전성 웹 서비스 개발에 적합합니다. 2) C는 수동 메모리 관리 및 컴파일러 최적화를 통해 궁극적 인 성능을 달성하며 임베디드 시스템 개발에 적합합니다.
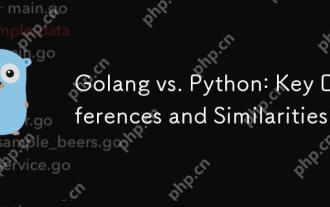
Golang과 Python은 각각 고유 한 장점이 있습니다. Golang은 고성능 및 동시 프로그래밍에 적합하지만 Python은 데이터 과학 및 웹 개발에 적합합니다. Golang은 동시성 모델과 효율적인 성능으로 유명하며 Python은 간결한 구문 및 풍부한 라이브러리 생태계로 유명합니다.
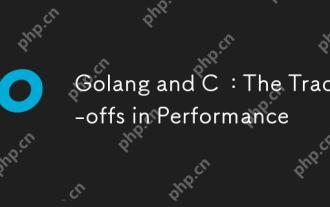
Golang과 C의 성능 차이는 주로 메모리 관리, 컴파일 최적화 및 런타임 효율에 반영됩니다. 1) Golang의 쓰레기 수집 메커니즘은 편리하지만 성능에 영향을 줄 수 있습니다. 2) C의 수동 메모리 관리 및 컴파일러 최적화는 재귀 컴퓨팅에서 더 효율적입니다.
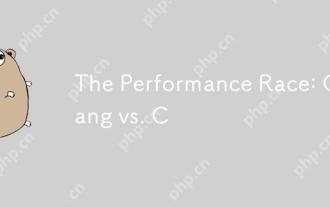
Golang과 C는 각각 공연 경쟁에서 고유 한 장점을 가지고 있습니다. 1) Golang은 높은 동시성과 빠른 발전에 적합하며 2) C는 더 높은 성능과 세밀한 제어를 제공합니다. 선택은 프로젝트 요구 사항 및 팀 기술 스택을 기반으로해야합니다.
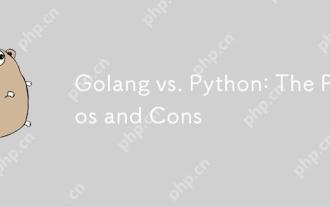
golangisidealforbuildingscalablesystemsdueToitsefficiencyandconcurrency
