C의 버블정렬
정렬은 모든 프로그래밍 언어에서 배워야 하는 필수 개념입니다. 대부분 정렬은 숫자와 관련된 배열에서 수행되며 배열의 데이터를 탐색하고 액세스하는 기술을 익히는 디딤돌입니다.
오늘 글에서 다룰 정렬기법의 종류는 버블정렬(Bubble Sort)입니다.
버블정렬
버블 정렬은 인접한 요소의 순서가 잘못된 경우 반복적으로 교체하여 작동하는 간단한 정렬 알고리즘입니다. 이 배열 정렬 방법은 평균 및 최악의 시나리오에 대한 시간 복잡도가 매우 높기 때문에 대규모 데이터 세트에는 적합하지 않습니다.
버블정렬 알고리즘 :
- 버블 정렬은 여러 단계를 거쳐 정렬하여 배열을 구성합니다.
- 첫 번째 패스: 가장 큰 요소가 마지막 위치, 즉 올바른 위치로 이동합니다.
- 두 번째 패스: 두 번째로 큰 요소가 마지막에서 두 번째 위치로 이동하며, 이는 후속 패스에서도 계속됩니다.
- 각 패스마다 배열의 정렬되지 않은 부분만 처리됩니다.
- k개를 통과한 후 가장 큰 k개 요소가 마지막 k개 슬롯의 올바른 위치에 있습니다.
- 각 패스 동안:
- 정렬되지 않은 섹션에서 인접한 요소를 비교하세요.
- 큰 요소가 작은 요소보다 먼저 나타나면 요소를 교체하세요.
- 패스가 끝나면 정렬되지 않은 가장 큰 요소가 올바른 위치로 이동합니다. 이 프로세스는 전체 배열이 정렬될 때까지 반복됩니다.
버블 정렬은 어떻게 작동하나요?
아래는 버블 정렬의 구현입니다. 내부 루프로 인해 스왑이 발생하지 않은 경우 알고리즘을 중지하여 최적화할 수 있습니다.
// Easy implementation of Bubble sort #include <stdio.h> int main(){ int i, j, size, temp, count=0, a[100]; //Asking the user for size of array printf("Enter the size of array you want to enter = \t"); scanf("%d", &size); //taking the input array through loop for (i=0;i<size;i++){ printf("Enter the %dth element",i); scanf("%d",&a[i]); } //printing the unsorted list printf("The list you entered is : \n"); for (i=0;i<size;i++){ printf("%d,\t",a[i]); } //sorting the list for (i = 0; i < size - 1; i++) { count = 1; for (j = 0; j < size - i - 1; j++) { if (a[j] > a[j + 1]) { //swapping elements temp=a[j]; a[j]=a[j+1]; a[j+1]=temp; count = 1; } } // If no two elements were swapped by inner loop, // then break if (count == 1) break; } // printing the sorted list printf("\nThe sorted list is : \n"); for (i=0;i<size;i++){ printf("%d,\t",a[i]); } return 0; }
출력 :
**
버블 정렬의 복잡성 분석:
시간 복잡도: O(n2)
보조공간 : O(1)
버블 정렬의 장점:
- 버블 정렬은 이해하고 구현하기 쉽습니다.
- 추가 메모리 공간이 필요하지 않습니다.
- 안정적인 정렬 알고리즘입니다. 즉, 동일한 키 값을 가진 요소는 정렬된 출력에서 상대적 순서를 유지합니다.
버블 정렬의 단점:
- 버블 정렬의 시간 복잡도는 O(n2)이므로 대규모 데이터 세트의 경우 속도가 매우 느립니다.
- 버블 정렬은 비교 기반 정렬 알고리즘입니다. 즉, 입력 데이터 세트에서 요소의 상대적 순서를 결정하려면 비교 연산자가 필요합니다. 특정 경우에는 알고리즘의 효율성이 제한될 수 있습니다.
문의사항은 댓글 달아주세요!!
그리고 모든 토론에 감사하겠습니다 :)
위 내용은 C의 버블정렬의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
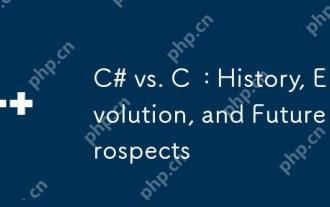
C#과 C의 역사와 진화는 독특하며 미래의 전망도 다릅니다. 1.C는 1983 년 Bjarnestroustrup에 의해 발명되어 객체 지향 프로그래밍을 C 언어에 소개했습니다. Evolution 프로세스에는 자동 키워드 소개 및 Lambda Expressions 소개 C 11, C 20 도입 개념 및 코 루틴과 같은 여러 표준화가 포함되며 향후 성능 및 시스템 수준 프로그래밍에 중점을 둘 것입니다. 2.C#은 2000 년 Microsoft에 의해 출시되었으며 C와 Java의 장점을 결합하여 진화는 단순성과 생산성에 중점을 둡니다. 예를 들어, C#2.0은 제네릭과 C#5.0 도입 된 비동기 프로그래밍을 소개했으며, 이는 향후 개발자의 생산성 및 클라우드 컴퓨팅에 중점을 둘 것입니다.
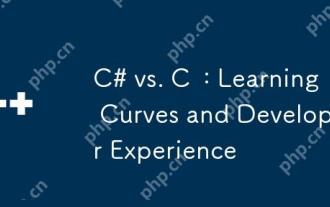
C# 및 C 및 개발자 경험의 학습 곡선에는 상당한 차이가 있습니다. 1) C#의 학습 곡선은 비교적 평평하며 빠른 개발 및 기업 수준의 응용 프로그램에 적합합니다. 2) C의 학습 곡선은 가파르고 고성능 및 저수준 제어 시나리오에 적합합니다.
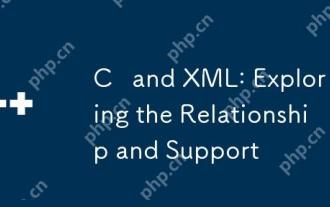
C는 XML과 타사 라이브러리 (예 : TinyXML, Pugixml, Xerces-C)와 상호 작용합니다. 1) 라이브러리를 사용하여 XML 파일을 구문 분석하고 C- 처리 가능한 데이터 구조로 변환하십시오. 2) XML을 생성 할 때 C 데이터 구조를 XML 형식으로 변환하십시오. 3) 실제 애플리케이션에서 XML은 종종 구성 파일 및 데이터 교환에 사용되어 개발 효율성을 향상시킵니다.
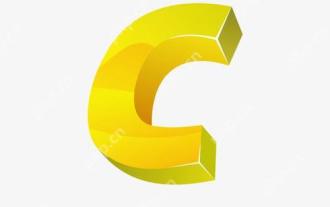
C에서 정적 분석의 적용에는 주로 메모리 관리 문제 발견, 코드 로직 오류 확인 및 코드 보안 개선이 포함됩니다. 1) 정적 분석은 메모리 누출, 이중 릴리스 및 초기화되지 않은 포인터와 같은 문제를 식별 할 수 있습니다. 2) 사용하지 않은 변수, 데드 코드 및 논리적 모순을 감지 할 수 있습니다. 3) Coverity와 같은 정적 분석 도구는 버퍼 오버플로, 정수 오버플로 및 안전하지 않은 API 호출을 감지하여 코드 보안을 개선 할 수 있습니다.
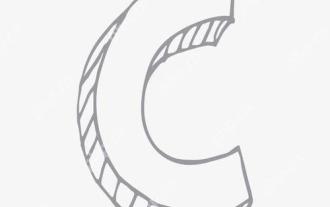
C에서 Chrono 라이브러리를 사용하면 시간과 시간 간격을보다 정확하게 제어 할 수 있습니다. 이 도서관의 매력을 탐구합시다. C의 크로노 라이브러리는 표준 라이브러리의 일부로 시간과 시간 간격을 다루는 현대적인 방법을 제공합니다. 시간과 C 시간으로 고통받는 프로그래머에게는 Chrono가 의심 할 여지없이 혜택입니다. 코드의 가독성과 유지 가능성을 향상시킬뿐만 아니라 더 높은 정확도와 유연성을 제공합니다. 기본부터 시작합시다. Chrono 라이브러리에는 주로 다음 주요 구성 요소가 포함됩니다. std :: Chrono :: System_Clock : 현재 시간을 얻는 데 사용되는 시스템 클럭을 나타냅니다. STD :: 크론
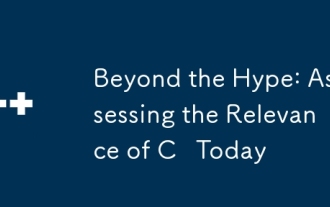
C는 여전히 현대 프로그래밍과 관련이 있습니다. 1) 고성능 및 직접 하드웨어 작동 기능은 게임 개발, 임베디드 시스템 및 고성능 컴퓨팅 분야에서 첫 번째 선택이됩니다. 2) 스마트 포인터 및 템플릿 프로그래밍과 같은 풍부한 프로그래밍 패러다임 및 현대적인 기능은 유연성과 효율성을 향상시킵니다. 학습 곡선은 가파르지만 강력한 기능은 오늘날의 프로그래밍 생태계에서 여전히 중요합니다.
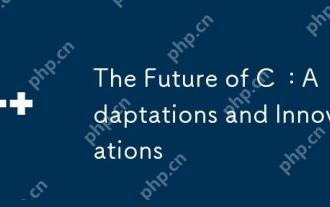
C의 미래는 병렬 컴퓨팅, 보안, 모듈화 및 AI/기계 학습에 중점을 둘 것입니다. 1) 병렬 컴퓨팅은 코 루틴과 같은 기능을 통해 향상 될 것입니다. 2)보다 엄격한 유형 검사 및 메모리 관리 메커니즘을 통해 보안이 향상 될 것입니다. 3) 변조는 코드 구성 및 편집을 단순화합니다. 4) AI 및 머신 러닝은 C가 수치 컴퓨팅 및 GPU 프로그래밍 지원과 같은 새로운 요구에 적응하도록 촉구합니다.
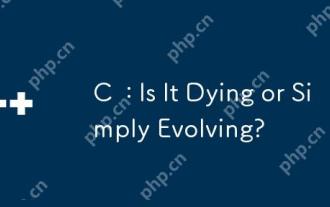
c is nontdying; it'sevolving.1) c COMINGDUETOITSTIONTIVENICICICICINICE INPERFORMICALEPPLICATION.2) thelugageIscontinuousUllyUpdated, witcentfeatureslikemodulesandCoroutinestoimproveusActionalance.3) despitechallen
