SSL 오류가 발생할 때 Golang에서 HTTPS 인증서를 확인하는 방법은 무엇입니까?
Golang으로 HTTPS 인증서 확인
맞춤형 인증서를 사용하여 서버에 HTTPS 요청을 하는 경우 보안 통신을 보장하기 위해 진위 여부를 확인하는 것이 중요합니다.
그러한 시나리오 중 하나에서는 별도의 포트에서 실행되는 애플리케이션이 HTTPS에서 호스팅되는 REST API에 액세스하려고 할 때 SSL 오류가 발생했습니다. 이 오류의 원인은 인식할 수 없는 인증 기관입니다.
이 문제를 해결하려면 HTTPS 요청 중에 전송에 인증 기관을 추가하는 것이 중요합니다. 다음 코드 조각은 이를 달성하는 방법을 보여줍니다.
package main import ( "crypto/tls" "io/ioutil" "log" "net/http" "crypto/x509" ) func main() { // Read the root CA certificate from file caCert, err := ioutil.ReadFile("rootCA.crt") if err != nil { log.Fatal(err) } // Create an X.509 certificate pool and add the CA certificate caCertPool := x509.NewCertPool() caCertPool.AppendCertsFromPEM(caCert) // Configure the TLS client to trust the CA tlsConfig := &tls.Config{ RootCAs: caCertPool, } // Create a new HTTP client with the modified TLS configuration client := &http.Client{ Transport: &http.Transport{ TLSClientConfig: tlsConfig, }, } // Make an HTTPS request using the client _, err = client.Get("https://secure.domain.com") if err != nil { panic(err) } }
또는 기존 CA 인증서를 사용할 수 없는 경우 솔루션은 자체 CA를 생성하고 해당 CA에서 서명한 인증서를 발급할 것을 제안합니다. 다음 명령은 단계별 접근 방식을 제공합니다.
CA 생성:
openssl genrsa -out rootCA.key 4096 openssl req -x509 -new -key rootCA.key -days 3650 -out rootCA.crt
CA가 서명한 secure.domain.com에 대한 인증서 생성 :
openssl genrsa -out secure.domain.com.key 2048 openssl req -new -key secure.domain.com.key -out secure.domain.com.csr #In answer to question `Common Name (e.g. server FQDN or YOUR name) []:` you should set `secure.domain.com` (your real domain name) openssl x509 -req -in secure.domain.com.csr -CA rootCA.crt -CAkey rootCA.key -CAcreateserial -days 365 -out secure.domain.com.crt
위 내용은 SSL 오류가 발생할 때 Golang에서 HTTPS 인증서를 확인하는 방법은 무엇입니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
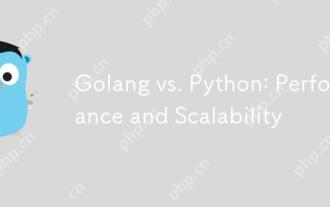
Golang은 성능과 확장 성 측면에서 Python보다 낫습니다. 1) Golang의 컴파일 유형 특성과 효율적인 동시성 모델은 높은 동시성 시나리오에서 잘 수행합니다. 2) 해석 된 언어로서 파이썬은 천천히 실행되지만 Cython과 같은 도구를 통해 성능을 최적화 할 수 있습니다.
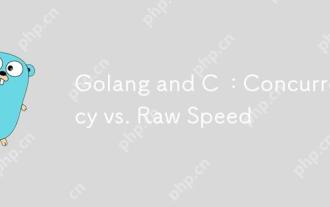
Golang은 동시성에서 C보다 낫고 C는 원시 속도에서 Golang보다 낫습니다. 1) Golang은 Goroutine 및 Channel을 통해 효율적인 동시성을 달성하며, 이는 많은 동시 작업을 처리하는 데 적합합니다. 2) C 컴파일러 최적화 및 표준 라이브러리를 통해 하드웨어에 가까운 고성능을 제공하며 극도의 최적화가 필요한 애플리케이션에 적합합니다.
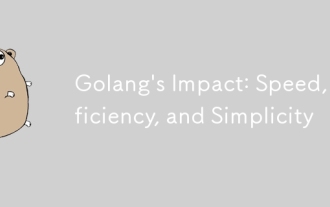
goimpactsdevelopmentpositively throughlyspeed, 효율성 및 단순성.
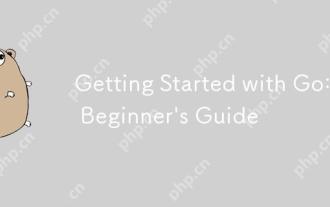
goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity, 효율성, 및 콘크리 론 피처
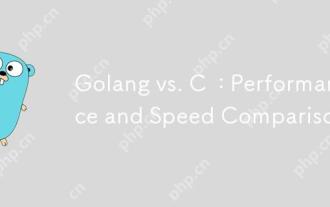
Golang은 빠른 개발 및 동시 시나리오에 적합하며 C는 극도의 성능 및 저수준 제어가 필요한 시나리오에 적합합니다. 1) Golang은 쓰레기 수집 및 동시성 메커니즘을 통해 성능을 향상시키고, 고전성 웹 서비스 개발에 적합합니다. 2) C는 수동 메모리 관리 및 컴파일러 최적화를 통해 궁극적 인 성능을 달성하며 임베디드 시스템 개발에 적합합니다.
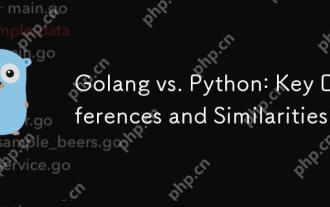
Golang과 Python은 각각 고유 한 장점이 있습니다. Golang은 고성능 및 동시 프로그래밍에 적합하지만 Python은 데이터 과학 및 웹 개발에 적합합니다. Golang은 동시성 모델과 효율적인 성능으로 유명하며 Python은 간결한 구문 및 풍부한 라이브러리 생태계로 유명합니다.
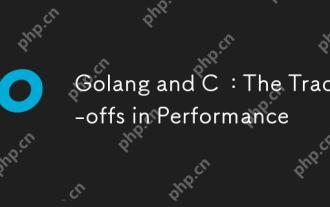
Golang과 C의 성능 차이는 주로 메모리 관리, 컴파일 최적화 및 런타임 효율에 반영됩니다. 1) Golang의 쓰레기 수집 메커니즘은 편리하지만 성능에 영향을 줄 수 있습니다. 2) C의 수동 메모리 관리 및 컴파일러 최적화는 재귀 컴퓨팅에서 더 효율적입니다.
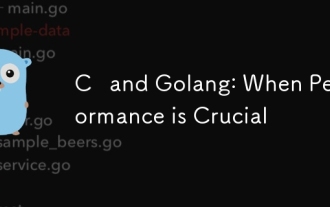
C는 하드웨어 리소스 및 고성능 최적화가 직접 제어되는 시나리오에 더 적합하지만 Golang은 빠른 개발 및 높은 동시성 처리가 필요한 시나리오에 더 적합합니다. 1.C의 장점은 게임 개발과 같은 고성능 요구에 적합한 하드웨어 특성 및 높은 최적화 기능에 가깝습니다. 2. Golang의 장점은 간결한 구문 및 자연 동시성 지원에 있으며, 이는 동시성 서비스 개발에 적합합니다.
