PHP, MySQL, JSON을 사용하여 Google 차트를 어떻게 만들 수 있나요?
PHP MySQL Google Chart JSON - 전체 예
기술 환경이 발전함에 따라 데이터 시각화 기능이 점점 더 중요해지고 있습니다. 데이터 시각화를 위한 강력한 도구 중 하나는 Google 차트입니다. 이를 통해 개발자는 원형 차트, 막대 차트, 꺾은선형 차트를 포함한 다양한 차트를 만들 수 있습니다. 그러나 Google 차트를 MySQL 데이터 소스와 통합하면 특히 PHP를 프로그래밍 언어로 사용할 때 문제가 발생할 수 있습니다.
이 문서에서는 PHP 및 MySQL을 사용하여 Google 차트를 생성하기 위한 포괄적인 솔루션을 제공합니다. 다양한 PHP 데이터 액세스 방법의 사용을 설명하는 여러 가지 예를 다룰 것입니다:
예 1: PHP-MySQL-JSON-Google 차트(비 Ajax)
사용법:
- 라는 이름의 MySQL 데이터베이스를 생성합니다. "chart."
- "weekly_task"와 "percentage"라는 두 개의 열이 있는 "googlechart"라는 테이블을 만듭니다.
- 예제 데이터를 테이블에 삽입하고 "백분율" 열에 다음이 포함되는지 확인합니다. 숫자만 값.
코드:
$con = mysql_connect("localhost", "Username", "Password") or die("Failed to connect with database!!!!"); mysql_select_db("Database Name", $con); // The Chart table contains two fields: weekly_task and percentage // This example will display a pie chart. If you need other charts such as a Bar chart, you will need to modify the code a little to make it work with bar chart and other charts $sth = mysql_query("SELECT * FROM chart"); /* --------------------------- example data: Table (Chart) -------------------------- weekly_task percentage Sleep 30 Watching Movie 40 work 44 */ //flag is not needed $flag = true; $table = array(); $table['cols'] = array( // Labels for your chart, these represent the column titles // Note that one column is in "string" format and another one is in "number" format as pie chart only required "numbers" for calculating percentage and string will be used for column title array('label' => 'Weekly Task', 'type' => 'string'), array('label' => 'Percentage', 'type' => 'number') ); $rows = array(); while($r = mysql_fetch_assoc($sth)) { $temp = array(); // The following line will be used to slice the Pie chart $temp[] = array('v' => (string) $r['Weekly_task']); // Values of each slice $temp[] = array('v' => (int) $r['percentage']); $rows[] = array('c' => $temp); } $table['rows'] = $rows; $jsonTable = json_encode($table); //echo $jsonTable; ?> <html> <head> <!--Load the Ajax API--> <script type="text/javascript" src="https://www.google.com/jsapi"></script> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.8.2/jquery.min.js"></script> <script type="text/javascript"> // Load the Visualization API and the piechart package. google.load('visualization', '1', {'packages':['corechart']}); // Set a callback to run when the Google Visualization API is loaded. google.setOnLoadCallback(drawChart); function drawChart() { // Create our data table out of JSON data loaded from server. var data = new google.visualization.DataTable(<?=$jsonTable?>); var options = { title: 'My Weekly Plan', is3D: 'true', width: 800, height: 600 }; // Instantiate and draw our chart, passing in some options. // Do not forget to check your div ID var chart = new google.visualization.PieChart(document.getElementById('chart_div')); chart.draw(data, options); } </script> </head> <body> <!--this is the div that will hold the pie chart--> <div>
예 2: PHP-PDO-JSON-MySQL-Google 차트
이 예에서는 PDO(PHP Data Objects)를 사용하여 데이터베이스에 연결함으로써 유연성을 높이고 security.
코드:
/* ... (code) ... */ try { /* Establish the database connection */ $conn = new PDO("mysql:host=localhost;dbname=$dbname", $username, $password); $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); /* Select all the weekly tasks from the table googlechart */ $result = $conn->query('SELECT * FROM googlechart'); /* --------------------------- example data: Table (googlechart) -------------------------- weekly_task percentage Sleep 30 Watching Movie 10 job 40 Exercise 20 */ $rows = array(); $table = array(); $table['cols'] = array( // Labels for your chart, these represent the column titles. /* Note that one column is in "string" format and another one is in "number" format as pie chart only required "numbers" for calculating percentage and string will be used for Slice title */ array('label' => 'Weekly Task', 'type' => 'string'), array('label' => 'Percentage', 'type' => 'number') ); /* Extract the information from $result */ foreach($result as $r) { $temp = array(); // The following line will be used to slice the Pie chart $temp[] = array('v' => (string) $r['weekly_task']); // Values of each slice $temp[] = array('v' => (int) $r['percentage']); $rows[] = array('c' => $temp); } $table['rows'] = $rows; // convert data into JSON format $jsonTable = json_encode($table); //echo $jsonTable; } catch(PDOException $e) { echo 'ERROR: ' . $e->getMessage(); } ?>
예제 3: PHP-MySQLi-JSON-Google 차트
이 예 데이터베이스 상호 작용을 위해 MySQL 확장의 향상된 버전인 MySQLi를 활용합니다.
/* ... (code) ... */ /* Establish the database connection */ $mysqli = new mysqli($DB_HOST, $DB_USER, $DB_PASS, $DB_NAME); if (mysqli_connect_errno()) { printf("Connect failed: %s\n", mysqli_connect_error()); exit(); } /* Select all the weekly tasks from the table googlechart */ $result = $mysqli->query('SELECT * FROM googlechart'); /* --------------------------- example data: Table (googlechart) -------------------------- Weekly_Task percentage Sleep 30 Watching Movie 10 job 40 Exercise 20 */ $rows = array(); $table = array(); $table['cols'] = array( // Labels for your chart, these represent the column titles. /* Note that one column is in "string" format and another one is in "number" format as pie chart only required "numbers" for calculating percentage and string will be used for Slice title */ array('label' => 'Weekly Task', 'type' => 'string'), array('label' => 'Percentage', 'type' => 'number') ); /* Extract the information from $result */ foreach($result as $r) { $temp = array(); // The following line will be used to slice the Pie chart $temp[] = array('v' => (string) $r['weekly_task']); // Values of the each slice $temp[] = array('v' => (int) $r['percentage']); $rows[] = array('c' => $temp); } $table['rows'] = $rows; // convert data into JSON format $jsonTable = json_encode($table); //echo $jsonTable;
위 내용은 PHP, MySQL, JSON을 사용하여 Google 차트를 어떻게 만들 수 있나요?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
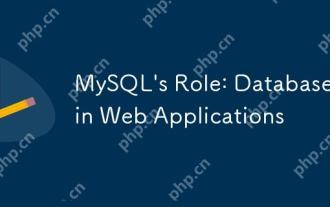
웹 응용 프로그램에서 MySQL의 주요 역할은 데이터를 저장하고 관리하는 것입니다. 1. MySQL은 사용자 정보, 제품 카탈로그, 트랜잭션 레코드 및 기타 데이터를 효율적으로 처리합니다. 2. SQL 쿼리를 통해 개발자는 데이터베이스에서 정보를 추출하여 동적 컨텐츠를 생성 할 수 있습니다. 3.mysql은 클라이언트-서버 모델을 기반으로 작동하여 허용 가능한 쿼리 속도를 보장합니다.
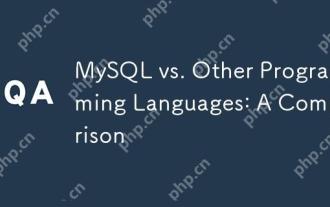
다른 프로그래밍 언어와 비교할 때 MySQL은 주로 데이터를 저장하고 관리하는 데 사용되는 반면 Python, Java 및 C와 같은 다른 언어는 논리적 처리 및 응용 프로그램 개발에 사용됩니다. MySQL은 데이터 관리 요구에 적합한 고성능, 확장 성 및 크로스 플랫폼 지원으로 유명하며 다른 언어는 데이터 분석, 엔터프라이즈 애플리케이션 및 시스템 프로그래밍과 같은 해당 분야에서 이점이 있습니다.
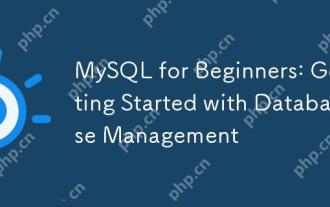
MySQL의 기본 작업에는 데이터베이스, 테이블 작성 및 SQL을 사용하여 데이터에서 CRUD 작업을 수행하는 것이 포함됩니다. 1. 데이터베이스 생성 : createAbasemy_first_db; 2. 테이블 만들기 : CreateTableBooks (idintauto_incrementprimarykey, titlevarchar (100) notnull, authorvarchar (100) notnull, published_yearint); 3. 데이터 삽입 : InsertIntobooks (Title, Author, Published_year) VA

innodbbufferpool은 데이터와 인덱싱 페이지를 캐싱하여 디스크 I/O를 줄여 데이터베이스 성능을 향상시킵니다. 작업 원칙에는 다음이 포함됩니다. 1. 데이터 읽기 : BufferPool의 데이터 읽기; 2. 데이터 작성 : 데이터 수정 후 BufferPool에 쓰고 정기적으로 디스크로 새로 고치십시오. 3. 캐시 관리 : LRU 알고리즘을 사용하여 캐시 페이지를 관리합니다. 4. 읽기 메커니즘 : 인접한 데이터 페이지를 미리로드합니다. Bufferpool을 크기를 조정하고 여러 인스턴스를 사용하여 데이터베이스 성능을 최적화 할 수 있습니다.
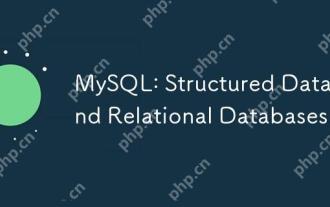
MySQL은 테이블 구조 및 SQL 쿼리를 통해 구조화 된 데이터를 효율적으로 관리하고 외래 키를 통해 테이블 간 관계를 구현합니다. 1. 테이블을 만들 때 데이터 형식을 정의하고 입력하십시오. 2. 외래 키를 사용하여 테이블 간의 관계를 설정하십시오. 3. 인덱싱 및 쿼리 최적화를 통해 성능을 향상시킵니다. 4. 데이터 보안 및 성능 최적화를 보장하기 위해 데이터베이스를 정기적으로 백업 및 모니터링합니다.
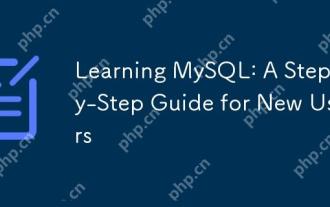
MySQL은 데이터 저장, 관리 및 분석에 적합한 강력한 오픈 소스 데이터베이스 관리 시스템이기 때문에 학습 할 가치가 있습니다. 1) MySQL은 SQL을 사용하여 데이터를 작동하고 구조화 된 데이터 관리에 적합한 관계형 데이터베이스입니다. 2) SQL 언어는 MySQL과 상호 작용하는 열쇠이며 CRUD 작업을 지원합니다. 3) MySQL의 작동 원리에는 클라이언트/서버 아키텍처, 스토리지 엔진 및 쿼리 최적화가 포함됩니다. 4) 기본 사용에는 데이터베이스 및 테이블 작성이 포함되며 고급 사용량은 Join을 사용하여 테이블을 결합하는 것과 관련이 있습니다. 5) 일반적인 오류에는 구문 오류 및 권한 문제가 포함되며 디버깅 기술에는 구문 확인 및 설명 명령 사용이 포함됩니다. 6) 성능 최적화에는 인덱스 사용, SQL 문의 최적화 및 데이터베이스의 정기 유지 보수가 포함됩니다.

MySQL은 초보자가 데이터베이스 기술을 배우는 데 적합합니다. 1. MySQL 서버 및 클라이언트 도구를 설치하십시오. 2. SELECT와 같은 기본 SQL 쿼리를 이해하십시오. 3. 마스터 데이터 작업 : 데이터를 만들고, 삽입, 업데이트 및 삭제합니다. 4. 고급 기술 배우기 : 하위 쿼리 및 창 함수. 5. 디버깅 및 최적화 : 구문 확인, 인덱스 사용, 선택*을 피하고 제한을 사용하십시오.
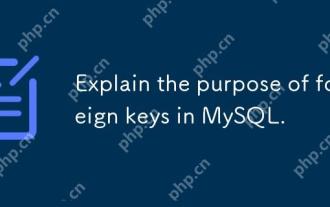
MySQL에서 외국 키의 기능은 테이블 간의 관계를 설정하고 데이터의 일관성과 무결성을 보장하는 것입니다. 외국 키는 참조 무결성 검사 및 계단식 작업을 통해 데이터의 효과를 유지합니다. 성능 최적화에주의를 기울이고 사용할 때 일반적인 오류를 피하십시오.
