SQL 마인드를 위한 ChromaDB
안녕하세요. Chroma DB는 GenAI 애플리케이션 작업에 유용한 벡터 데이터베이스입니다. 이 기사에서는 MySQL의 유사한 관계를 살펴봄으로써 Chroma DB에서 쿼리를 실행할 수 있는 방법을 살펴보겠습니다.
개요
SQL과 달리 자체 스키마를 정의할 수 없습니다. Chroma에서는 각각 고유한 목적을 가진 고정된 열이 제공됩니다.
import chromadb #setiing up the client client = chromadb.Client() collection = client.create_collection(name="name") collection.add( documents = ["str1","str2","str3",...] ids = [1,2,3,....] metadatas=[{"chapter": "3", "verse": "16"},{"chapter":"3", "verse":"5"}, ..] embeddings = [[1,2,3], [3,4,5], [5,6,7]] )
ID: 고유한 ID입니다. SQL과 달리 자동 증가가 없으므로 직접 제공해야 합니다.
문서: 임베딩을 생성하는 데 사용되는 텍스트 데이터를 삽입하는 데 사용됩니다. 텍스트를 제공하면 자동으로 임베딩이 생성됩니다. 또는 임베딩을 직접 제공하고 다른 곳에 텍스트를 저장할 수도 있습니다.
임베딩: 내 생각에는 유사성 검색을 수행하는 데 사용되는 임베딩이 데이터베이스에서 가장 중요한 부분입니다.
메타데이터: 추가 컨텍스트를 위해 데이터베이스에 추가할 수 있는 추가 데이터를 연결하는 데 사용됩니다.
이제 컬렉션의 기본 사항이 명확해졌으므로 CRUD 작업으로 넘어가서 데이터베이스를 쿼리하는 방법을 살펴보겠습니다.
CRUD 작업
참고: 컬렉션은 Chroma의 테이블과 같습니다
컬렉션을 생성하려면 create_collection()을 사용하고 필요에 따라 작업을 수행할 수 있지만 컬렉션이 이미 만들어져서 이를 다시 참조해야 하는 경우 get_collection()을 사용해야 합니다. 그렇지 않으면 오류가 발생합니다.
Create Table tablename
#Create a collection collection = client.create_collection(name="name") #If a collection is already made and you need to use it again the use collection = client.get_collection(name="name")
Insert into tablename Values(... , ..., ...)
collection.add( ids = [1] documents = ["some text"] metadatas = [{"key":"value"}] embeddings = [[1,2,3]] )
삽입된 데이터를 업데이트하거나 데이터를 삭제하려면 다음 명령을 사용할 수 있습니다
collection.update( ids = [2] documents = ["some text"] metadatas = [{"key":"value"}] embeddings = [[1,2,3]] ) # If the id does not exist update will do nothing. to add data if id does not exist use collection.upsert( ids = [2] documents = ["some text"] metadatas = [{"key":"value"}] embeddings = [[1,2,3]] ) # To delete data use delete and refrence the document or id or the feild collection.delete( documents = ["some text"] ) # Or you can delete from a bunch of ids using where that will apply filter on metadata collection.delete( ids=["id1", "id2", "id3",...], where={"chapter": "20"} )
쿼리
이제 특정 검색어가 어떻게 나타나는지 살펴보겠습니다
Select * from tablename Select * from tablename limit value Select Documents, Metadata from tablename
collection.get() collection.get(limit = val) collection.get(include = ["documents","metadata"])
더 고급 쿼리를 위해 대규모 테이블 세트를 가져오는 데 get()이 있지만 쿼리 방법을 사용해야 합니다
Select A,B from table limit val
collection.query( n_results = val #limit includes = [A,B] )
이제 데이터를 필터링하는 세 가지 가능한 방법이 있습니다: 유사성 검색(주로 사용되는 벡터 데이터베이스), 메타데이터 필터 및 문서 필터
유사성 검색
텍스트나 임베딩을 기반으로 검색하여 가장 유사한 결과를 얻을 수 있습니다
collection.query(query_texts=["string"]) collection.query(query_embeddings=[[1,2,3]])
ChromaDB에서는 쿼리 중 결과를 필터링하는 데 where 및 where_document 매개변수가 사용됩니다. 이 필터를 사용하면 메타데이터 또는 특정 문서 콘텐츠를 기반으로 유사성 검색을 세분화할 수 있습니다.
메타데이터로 필터링
where 매개변수를 사용하면 관련 메타데이터를 기준으로 문서를 필터링할 수 있습니다. 메타데이터는 일반적으로 문서 삽입 중에 제공하는 키-값 쌍의 사전입니다.
카테고리, 작성자, 날짜 등의 메타데이터를 기준으로 문서를 필터링하세요.
import chromadb #setiing up the client client = chromadb.Client() collection = client.create_collection(name="name") collection.add( documents = ["str1","str2","str3",...] ids = [1,2,3,....] metadatas=[{"chapter": "3", "verse": "16"},{"chapter":"3", "verse":"5"}, ..] embeddings = [[1,2,3], [3,4,5], [5,6,7]] )
Create Table tablename
문서 내용으로 필터링
where_document 매개변수를 사용하면 문서 내용을 기준으로 직접 필터링할 수 있습니다.
특정 키워드가 포함된 문서만 검색합니다.
#Create a collection collection = client.create_collection(name="name") #If a collection is already made and you need to use it again the use collection = client.get_collection(name="name")
주요 사항:
- $contains, $startsWith 또는 $endsWith와 같은 연산자를 사용하세요.
- $contains: 하위 문자열이 포함된 문서를 일치시킵니다.
- $startsWith: 하위 문자열로 시작하는 문서를 일치시킵니다.
- $endsWith: 하위 문자열로 끝나는 문서를 일치시킵니다.
-
예:
Insert into tablename Values(... , ..., ...)
로그인 후 복사로그인 후 복사
일반적인 사용 사례:
다음과 같이 세 가지 필터를 모두 결합할 수 있습니다.
-
특정 카테고리 내에서 검색:
collection.add( ids = [1] documents = ["some text"] metadatas = [{"key":"value"}] embeddings = [[1,2,3]] )
로그인 후 복사로그인 후 복사 -
특정 용어가 포함된 문서 검색:
collection.update( ids = [2] documents = ["some text"] metadatas = [{"key":"value"}] embeddings = [[1,2,3]] ) # If the id does not exist update will do nothing. to add data if id does not exist use collection.upsert( ids = [2] documents = ["some text"] metadatas = [{"key":"value"}] embeddings = [[1,2,3]] ) # To delete data use delete and refrence the document or id or the feild collection.delete( documents = ["some text"] ) # Or you can delete from a bunch of ids using where that will apply filter on metadata collection.delete( ids=["id1", "id2", "id3",...], where={"chapter": "20"} )
로그인 후 복사로그인 후 복사 -
메타데이터와 문서 콘텐츠 필터 결합:
Select * from tablename Select * from tablename limit value Select Documents, Metadata from tablename
로그인 후 복사로그인 후 복사
이러한 필터는 유사성 검색의 정확성을 향상시켜 ChromaDB를 대상 문서 검색을 위한 강력한 도구로 만듭니다.
결론
나만의 프로그램을 만들려고 할 때 문서가 욕심이 많이 남는다고 느껴서 이 글을 썼습니다. 도움이 되었으면 좋겠습니다!
글을 읽어주셔서 감사합니다. 좋아요와 공유 부탁드립니다. 또한 귀하가 소프트웨어 아키텍처를 처음 접하고 더 많은 것을 알고 싶다면 저는 개인적으로 귀하와 함께 일할 그룹 기반 코호트를 시작하고 소그룹을 통해 소프트웨어 아키텍처 및 디자인 원칙에 대한 모든 것을 가르칠 것입니다. 관심이 있으시면 아래 양식을 작성해 주세요. https://forms.gle/SUAxrzRyvbnV8uCGA
위 내용은 SQL 마인드를 위한 ChromaDB의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
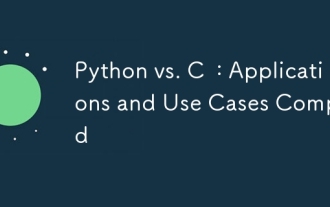
Python은 데이터 과학, 웹 개발 및 자동화 작업에 적합한 반면 C는 시스템 프로그래밍, 게임 개발 및 임베디드 시스템에 적합합니다. Python은 단순성과 강력한 생태계로 유명하며 C는 고성능 및 기본 제어 기능으로 유명합니다.
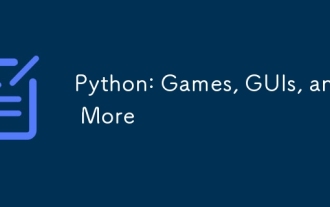
Python은 게임 및 GUI 개발에서 탁월합니다. 1) 게임 개발은 Pygame을 사용하여 드로잉, 오디오 및 기타 기능을 제공하며 2D 게임을 만드는 데 적합합니다. 2) GUI 개발은 Tkinter 또는 PYQT를 선택할 수 있습니다. Tkinter는 간단하고 사용하기 쉽고 PYQT는 풍부한 기능을 가지고 있으며 전문 개발에 적합합니다.
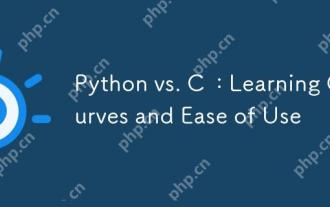
Python은 배우고 사용하기 쉽고 C는 더 강력하지만 복잡합니다. 1. Python Syntax는 간결하며 초보자에게 적합합니다. 동적 타이핑 및 자동 메모리 관리를 사용하면 사용하기 쉽지만 런타임 오류가 발생할 수 있습니다. 2.C는 고성능 응용 프로그램에 적합한 저수준 제어 및 고급 기능을 제공하지만 학습 임계 값이 높고 수동 메모리 및 유형 안전 관리가 필요합니다.
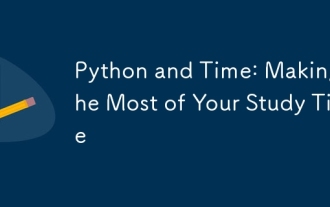
제한된 시간에 Python 학습 효율을 극대화하려면 Python의 DateTime, Time 및 Schedule 모듈을 사용할 수 있습니다. 1. DateTime 모듈은 학습 시간을 기록하고 계획하는 데 사용됩니다. 2. 시간 모듈은 학습과 휴식 시간을 설정하는 데 도움이됩니다. 3. 일정 모듈은 주간 학습 작업을 자동으로 배열합니다.
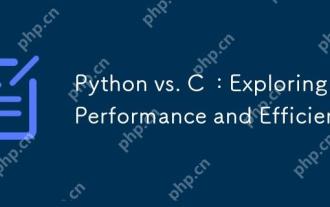
Python은 개발 효율에서 C보다 낫지 만 C는 실행 성능이 높습니다. 1. Python의 간결한 구문 및 풍부한 라이브러리는 개발 효율성을 향상시킵니다. 2.C의 컴파일 유형 특성 및 하드웨어 제어는 실행 성능을 향상시킵니다. 선택할 때는 프로젝트 요구에 따라 개발 속도 및 실행 효율성을 평가해야합니다.
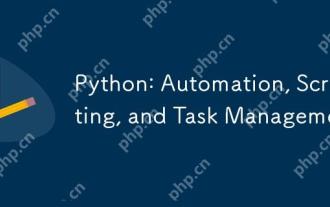
파이썬은 자동화, 스크립팅 및 작업 관리가 탁월합니다. 1) 자동화 : 파일 백업은 OS 및 Shutil과 같은 표준 라이브러리를 통해 실현됩니다. 2) 스크립트 쓰기 : PSUTIL 라이브러리를 사용하여 시스템 리소스를 모니터링합니다. 3) 작업 관리 : 일정 라이브러리를 사용하여 작업을 예약하십시오. Python의 사용 편의성과 풍부한 라이브러리 지원으로 인해 이러한 영역에서 선호하는 도구가됩니다.
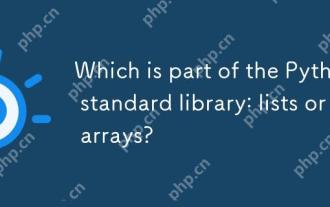
Pythonlistsarepartoftsandardlardlibrary, whileraysarenot.listsarebuilt-in, 다재다능하고, 수집 할 수있는 반면, arraysarreprovidedByTearRaymoduledlesscommonlyusedDuetolimitedFunctionality.
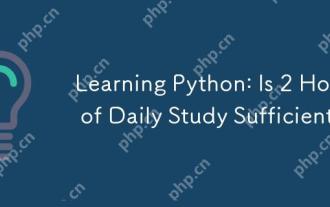
하루에 2 시간 동안 파이썬을 배우는 것으로 충분합니까? 목표와 학습 방법에 따라 다릅니다. 1) 명확한 학습 계획을 개발, 2) 적절한 학습 자원 및 방법을 선택하고 3) 실습 연습 및 검토 및 통합 연습 및 검토 및 통합,이 기간 동안 Python의 기본 지식과 고급 기능을 점차적으로 마스터 할 수 있습니다.
