Python에서 간단한 SQLite 라이브러리 관리자 구축
Python에서 간단한 SQLite 라이브러리 관리자 구축
데이터를 효율적으로 관리하는 것은 모든 프로젝트의 핵심 부분이며 SQLite는 이 작업을 간단하고 가볍게 만듭니다. 이 튜토리얼에서는 최소한의 노력으로 책을 추가하고 검색할 수 있도록 도서관 데이터베이스를 관리하는 작은 Python 애플리케이션을 구축합니다.
이 기사가 끝나면 다음 방법을 알게 될 것입니다.
- SQLite 데이터베이스와 테이블을 생성하세요.
- 중복을 방지하면서 기록을 삽입하세요.
- 특정 기준에 따라 데이터를 검색합니다.
1. 데이터베이스 및 테이블 생성
SQLite 데이터베이스 파일을 생성하고 books 테이블을 정의하는 것부터 시작해 보겠습니다. 각 책에는 제목, 저자, ISBN, 출판 날짜 및 장르에 대한 필드가 있습니다.
import sqlite3 import os def create_library_database(): """Creates the library database if it doesn't already exist.""" db_name = "library.db" if not os.path.exists(db_name): print(f"Creating database: {db_name}") conn = sqlite3.connect(db_name) cursor = conn.cursor() cursor.execute(''' CREATE TABLE IF NOT EXISTS books ( id INTEGER PRIMARY KEY AUTOINCREMENT, title TEXT, author TEXT, isbn TEXT UNIQUE, published_date DATE, genre TEXT ) ''') conn.commit() conn.close() else: print(f"Database already exists: {db_name}")
데이터베이스를 초기화하려면 다음 함수를 실행하세요.
create_library_database()
이렇게 하면 지정된 필드가 있는 책 테이블이 포함된 library.db 파일이 프로젝트 디렉토리에 생성됩니다.
- 데이터베이스에 도서 삽입
도서를 삽입할 때 isbn 필드를 기준으로 중복 항목이 발생하지 않도록 하려고 합니다. 중복을 수동으로 확인하는 대신 SQLite의 INSERT OR IGNORE 문을 사용하겠습니다.
도서 추가 기능은 다음과 같습니다.
def insert_book(book): """ Inserts a book into the database. If a book with the same ISBN already exists, the insertion is ignored. """ conn = sqlite3.connect("library.db") cursor = conn.cursor() try: # Insert the book. Ignore the insertion if the ISBN already exists. cursor.execute(''' INSERT OR IGNORE INTO books (title, author, isbn, published_date, genre) VALUES (?, ?, ?, ?, ?) ''', (book["title"], book["author"], book["isbn"], book["published_date"], book["genre"])) conn.commit() if cursor.rowcount == 0: print(f"The book with ISBN '{book['isbn']}' already exists in the database.") else: print(f"Book inserted: {book['title']} by {book['author']}") except sqlite3.Error as e: print(f"Database error: {e}") finally: conn.close()
이 함수는 INSERT OR IGNORE SQL 문을 사용하여 중복 항목을 효율적으로 건너뛰도록 합니다.
3. 일부 도서 추가
라이브러리에 책을 추가하여 insert_book 기능을 테스트해 보겠습니다.
books = [ { "title": "To Kill a Mockingbird", "author": "Harper Lee", "isbn": "9780061120084", "published_date": "1960-07-11", "genre": "Fiction" }, { "title": "1984", "author": "George Orwell", "isbn": "9780451524935", "published_date": "1949-06-08", "genre": "Dystopian" }, { "title": "Pride and Prejudice", "author": "Jane Austen", "isbn": "9781503290563", "published_date": "1813-01-28", "genre": "Romance" } ] for book in books: insert_book(book)
위 코드를 실행하면 해당 도서가 데이터베이스에 추가됩니다. 다시 실행하면 다음과 같은 메시지가 표시됩니다.
The book with ISBN '9780061120084' already exists in the database. The book with ISBN '9780451524935' already exists in the database. The book with ISBN '9781503290563' already exists in the database.
4. 도서 검색
데이터베이스를 쿼리하여 쉽게 데이터를 검색할 수 있습니다. 예를 들어, 도서관에 있는 모든 책을 가져오려면:
def fetch_all_books(): conn = sqlite3.connect("library.db") cursor = conn.cursor() cursor.execute("SELECT * FROM books") rows = cursor.fetchall() conn.close() return rows books = fetch_all_books() for book in books: print(book)
결론
이제 Python 몇 줄만 있으면 중복을 방지하면서 책을 삽입하고 손쉽게 레코드를 검색할 수 있는 기능적인 라이브러리 관리자가 있습니다. SQLite의 INSERT OR IGNORE는 제약 조건 처리를 단순화하여 코드를 더욱 간결하고 효율적으로 만드는 강력한 기능입니다.
다음과 같은 기능으로 이 프로젝트를 자유롭게 확장하세요.
- 제목이나 저자별로 책을 검색합니다.
- 도서 정보를 업데이트 중입니다.
- 도서 삭제.
다음에는 무엇을 만들 예정인가요? ?
위 내용은 Python에서 간단한 SQLite 라이브러리 관리자 구축의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










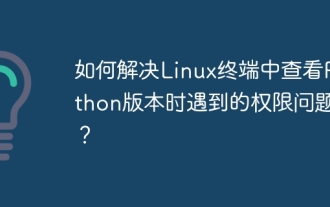
Linux 터미널에서 Python 버전을 보려고 할 때 Linux 터미널에서 Python 버전을 볼 때 권한 문제에 대한 솔루션 ... Python을 입력하십시오 ...
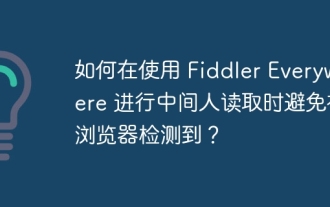
Fiddlerevery Where를 사용할 때 Man-in-the-Middle Reading에 Fiddlereverywhere를 사용할 때 감지되는 방법 ...
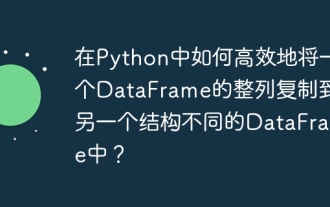
Python의 Pandas 라이브러리를 사용할 때는 구조가 다른 두 데이터 프레임 사이에서 전체 열을 복사하는 방법이 일반적인 문제입니다. 두 개의 dats가 있다고 가정 해
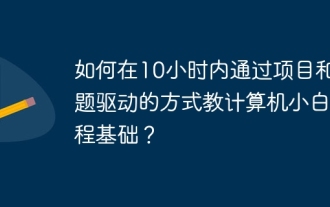
10 시간 이내에 컴퓨터 초보자 프로그래밍 기본 사항을 가르치는 방법은 무엇입니까? 컴퓨터 초보자에게 프로그래밍 지식을 가르치는 데 10 시간 밖에 걸리지 않는다면 무엇을 가르치기로 선택 하시겠습니까?
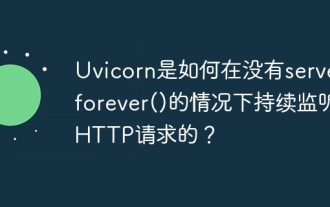
Uvicorn은 HTTP 요청을 어떻게 지속적으로 듣습니까? Uvicorn은 ASGI를 기반으로 한 가벼운 웹 서버입니다. 핵심 기능 중 하나는 HTTP 요청을 듣고 진행하는 것입니다 ...
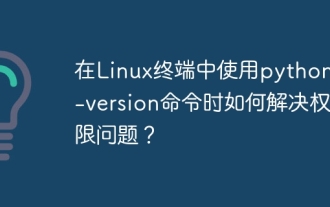
Linux 터미널에서 Python 사용 ...
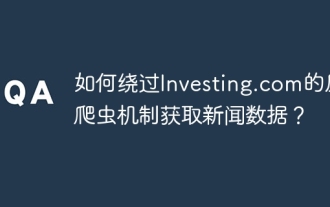
Investing.com의 크롤링 전략 이해 많은 사람들이 종종 Investing.com (https://cn.investing.com/news/latest-news)에서 뉴스 데이터를 크롤링하려고합니다.
