ExecutorService에서 중단 가능한 작업에 대한 시간 초과를 어떻게 구현할 수 있습니까?
중단 가능한 작업에 대한 시간 제한이 있는 ExecutorService
작업을 동시에 실행할 때 지정된 시간을 초과하는 작업을 정상적으로 중단하는 메커니즘을 갖는 것이 종종 바람직합니다. 시간 초과. 이는 장기 실행 작업으로 인해 애플리케이션이 잠기거나 성능 문제가 발생할 수 있는 상황에서 특히 유용합니다.
TimeoutExecutorService 구현
다음은 TimeoutThreadPoolExecutor를 확장하는 구현입니다. 표준 ThreadPoolExecutor 및 시간 제한 통합 기능:
class TimeoutThreadPoolExecutor extends ThreadPoolExecutor { private final long timeout; private final TimeUnit timeoutUnit; private final ScheduledExecutorService timeoutExecutor = Executors.newSingleThreadScheduledExecutor(); private final ConcurrentMap<Runnable, ScheduledFuture> runningTasks = new ConcurrentHashMap<>(); public TimeoutThreadPoolExecutor(int corePoolSize, int maximumPoolSize, long keepAliveTime, TimeUnit unit, BlockingQueue<Runnable> workQueue, long timeout, TimeUnit timeoutUnit) { super(corePoolSize, maximumPoolSize, keepAliveTime, unit, workQueue); this.timeout = timeout; this.timeoutUnit = timeoutUnit; } // Override methods to implement timeout behavior @Override protected void beforeExecute(Thread t, Runnable r) { if (timeout > 0) { ScheduledFuture scheduled = timeoutExecutor.schedule(new TimeoutTask(t), timeout, timeoutUnit); runningTasks.put(r, scheduled); } } @Override protected void afterExecute(Runnable r, Throwable t) { ScheduledFuture timeoutTask = runningTasks.remove(r); if (timeoutTask != null) { timeoutTask.cancel(false); } } // Timeout task to interrupt threads class TimeoutTask implements Runnable { private final Thread thread; public TimeoutTask(Thread thread) { this.thread = thread; } @Override public void run() { thread.interrupt(); } } }
사용법:
TimeoutThreadPoolExecutor를 사용하려면 간단히 시간 초과 값으로 인스턴스화하면 됩니다.
TimeoutThreadPoolExecutor executor = new TimeoutThreadPoolExecutor( 4, // corePoolSize 8, // maximumPoolSize 1, // keepAliveTime TimeUnit.SECONDS, // timeUnit new LinkedBlockingQueue<>(), // workQueue 5, // timeout TimeUnit.SECONDS // timeoutUnit );
그런 다음 , 다음과 같이 실행자에게 작업을 제출합니다. 평소:
executor.submit(() -> { // long-running task });
작업이 지정된 시간 초과보다 오래 걸리면 작업을 실행하는 스레드가 중단되어 작업이 정상적으로 종료됩니다.
대체 해결 방법
작업에 대한 시간 초과를 구현하는 또 다른 접근 방식은 응답에서 제안된 대로 ScheduledExecutorService를 사용하는 것입니다. 여기에는 작업을 Callable로 제출하고 생성된 미래를 유지하는 것이 포함됩니다. 그런 다음 특정 기간이 지나면 미래를 취소하도록 두 번째 작업을 예약하여 작업을 효과적으로 중단할 수 있습니다.
위 내용은 ExecutorService에서 중단 가능한 작업에 대한 시간 초과를 어떻게 구현할 수 있습니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
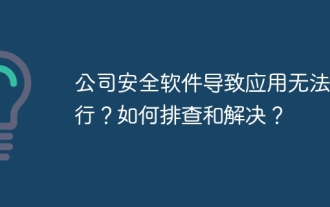
일부 애플리케이션이 제대로 작동하지 않는 회사의 보안 소프트웨어에 대한 문제 해결 및 솔루션. 많은 회사들이 내부 네트워크 보안을 보장하기 위해 보안 소프트웨어를 배포 할 것입니다. ...
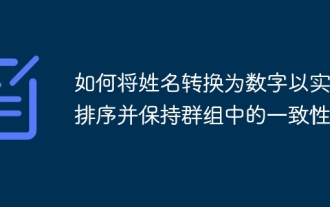
많은 응용 프로그램 시나리오에서 정렬을 구현하기 위해 이름으로 이름을 변환하는 솔루션, 사용자는 그룹으로, 특히 하나로 분류해야 할 수도 있습니다.
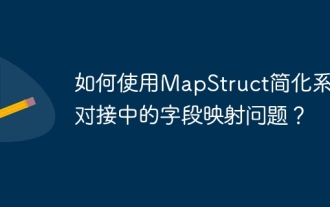
시스템 도킹의 필드 매핑 처리 시스템 도킹을 수행 할 때 어려운 문제가 발생합니다. 시스템의 인터페이스 필드를 효과적으로 매핑하는 방법 ...
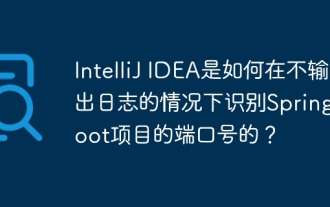
IntellijideAultimate 버전을 사용하여 봄을 시작하십시오 ...
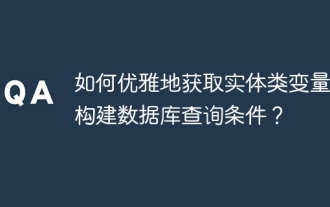
데이터베이스 작업에 MyBatis-Plus 또는 기타 ORM 프레임 워크를 사용하는 경우 엔티티 클래스의 속성 이름을 기반으로 쿼리 조건을 구성해야합니다. 매번 수동으로 ...
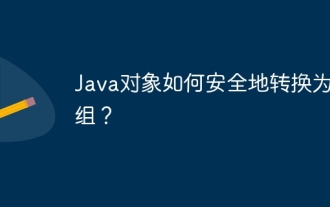
Java 객체 및 배열의 변환 : 캐스트 유형 변환의 위험과 올바른 방법에 대한 심층적 인 논의 많은 Java 초보자가 객체를 배열로 변환 할 것입니다 ...
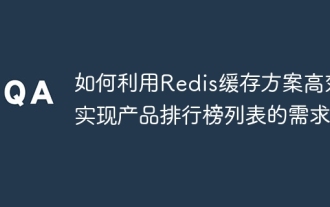
Redis 캐싱 솔루션은 제품 순위 목록의 요구 사항을 어떻게 인식합니까? 개발 과정에서 우리는 종종 a ... 표시와 같은 순위의 요구 사항을 처리해야합니다.
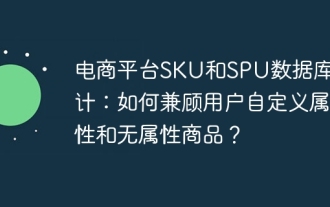
전자 상거래 플랫폼에서 SKU 및 SPU 테이블의 디자인에 대한 자세한 설명이 기사는 전자 상거래 플랫폼에서 SKU 및 SPU의 데이터베이스 설계 문제, 특히 사용자 정의 판매를 처리하는 방법에 대해 논의 할 것입니다 ...
