Node.JS에 Google 캘린더 API 통합: 이벤트 생성 및 회의 예약 가이드
Google Calendar API는 프로그래밍 방식으로 이벤트를 관리하고 회의 일정을 예약할 수 있는 강력한 도구입니다. 이 튜토리얼에서는 API를 Node.js 애플리케이션에 통합하여 OAuth 인증을 처리하고, 액세스 토큰을 검색하고, 이벤트를 생성하는 과정을 안내합니다. 또한 원활한 사용자 경험을 위해 프런트엔드 애플리케이션의 리디렉션 처리에 대해서도 다룹니다.
전제조건
- Node.js(버전 18).
- 캘린더 API가 활성화된 Google 클라우드 프로젝트
-
Google Cloud Console의 자격 증명. 설정하려면 다음 단계를 따르세요.
- Google Cloud Console으로 이동합니다.
- 새 프로젝트를 만들거나 기존 프로젝트를 선택하세요.
- 프로젝트에 Google Calendar API를 활성화하세요.
- API 및 서비스 >로 이동 자격 증명 및 OAuth 2.0 자격 증명을 생성합니다.
- 자격증명 JSON 파일을 다운로드하여 프로젝트에 cleint_secrets.json으로 저장하세요.
- 자격증명(client_id, client_secret 등)을 안전하게 저장하기 위한 .env 또는 client_secrets.json 파일.
- googleapis npm 패키지가 설치되었습니다(npm install googleapis).
Google OAuth 인증 설정
첫 번째 단계는 사용자가 Google 계정으로 인증하고 캘린더 관리에 대한 액세스 권한을 부여하도록 허용하는 것입니다. 이는 OAuth2 프로토콜
을 사용하여 달성됩니다.1단계: 인증 URL 생성
Google OAuth2 인증 URL을 생성하는 방법은 다음과 같습니다.
async googleAuthConsent() { try { // Read credentials from a file const credentials = JSON.parse( await promisify(fs.readFile)('./client_secrets.json', 'utf-8'), ); // change this with your redirect url const REDIRECT_URI = "http://localhost:3000"; const oauth2Client = new google.auth.OAuth2( credentials.web.client_id, credentials.web.client_secret, REDIRECT_URI, ); const scopes = ['https://www.googleapis.com/auth/calendar']; const authUrl = oauth2Client.generateAuthUrl({ access_type: 'offline', scope: scopes, }); return { message: 'Auth URL created successfully', url: authUrl, }; } catch (error) { throw new Error(error.message || 'Internal Server Error'); } }
핵심 포인트:
- 범위: 액세스 수준을 지정합니다. 캘린더 이벤트의 경우 범위는 https://www.googleapis.com/auth/calendar입니다.
- 액세스 유형: 오프라인으로 설정하면 새로 고침 토큰이 반환됩니다.
2단계: 액세스 토큰 처리
사용자는 생성된 URL을 통해 인증한 후 코드를 통해 애플리케이션으로 리디렉션됩니다. 이 코드는 토큰으로 교환됩니다.
async generateGoogleOAuthToken( data: { code: string; scope: string }, ) { try { const { code } = data; const credentials = JSON.parse( await promisify(fs.readFile)('./client_secrets.json', 'utf-8'), ); // change this with your redirect url const REDIRECT_URI = "http://localhost:3000"; const oauth2Client = new google.auth.OAuth2( credentials.web.client_id, credentials.web.client_secret, REDIRECT_URI, ); const { tokens } = await oauth2Client.getToken(code); // your logic for storing token i.g. database or file return { message: 'User OAuth Token Generated Successfully', token, }; } catch (error) { throw new Error(error.message || 'Internal Server Error'); } }
3단계: 이벤트 생성
토큰이 안전하게 저장되었으므로 이제 Google Calendar API를 사용하여 이벤트를 만들 수 있습니다. 다음은 시간, 참석자, 알림 및 선택적 Google Meet 링크와 같은 이벤트 세부정보를 포함하여 회의를 예약하는 일반적인 방법입니다.
일반 이벤트 생성 방법
이 방법은 다음을 달성합니다.
- 사용자 인증: OAuth2를 사용하여 Google로 인증합니다.
- 이벤트 만들기: 사용자의 기본 캘린더에 이벤트를 삽입합니다.
- 추가 기능 포함: Google Meet 링크, 참석자, 맞춤 알림과 같은 옵션을 추가하세요.
async googleAuthConsent() { try { // Read credentials from a file const credentials = JSON.parse( await promisify(fs.readFile)('./client_secrets.json', 'utf-8'), ); // change this with your redirect url const REDIRECT_URI = "http://localhost:3000"; const oauth2Client = new google.auth.OAuth2( credentials.web.client_id, credentials.web.client_secret, REDIRECT_URI, ); const scopes = ['https://www.googleapis.com/auth/calendar']; const authUrl = oauth2Client.generateAuthUrl({ access_type: 'offline', scope: scopes, }); return { message: 'Auth URL created successfully', url: authUrl, }; } catch (error) { throw new Error(error.message || 'Internal Server Error'); } }
핵심 포인트:
- OAuth2 인증: 사용자의 Google 캘린더에 대한 보안 액세스를 보장합니다.
- 회의 데이터: 지정 시 Google Meet 링크를 자동으로 추가합니다.
- 알림: 이메일 및 팝업 알림과 같은 맞춤형 옵션
- 오류 처리: 더 나은 디버깅을 위한 적절한 오류 메시지.
프런트엔드 통합
Google 인증 후 리디렉션을 처리하려면 프런트엔드에서 다음을 수행해야 합니다.
- Google 인증 URL로 리디렉션: 사용자가 인증 프로세스를 시작할 때 트리거됩니다.
- 콜백 처리: 인증 코드를 캡처하여 토큰 생성을 위해 백엔드로 보냅니다.
예시 흐름:
- 버튼 클릭 -> 인증 URL로 리디렉션합니다.
- Google이 인증하고 다시 앱으로 리디렉션합니다.
- 앱이 코드를 캡처하고 API 엔드포인트를 호출하여 토큰을 생성합니다.
최종 생각
이 통합은 NodeJS 애플리케이션에 강력한 일정 기능을 구축하기 위한 첫 번째 단계입니다. 다음 부분에서는 일반적인 이벤트 생성 방법을 다룹니다. 사용자 상호 작용을 위한 프런트엔드와 결합하면 최소한의 노력으로 강력한 일정 관리 솔루션을 만들 수 있습니다.
완전한 구현과 이벤트 관리 과정을 지켜봐 주시기 바랍니다!
이 튜토리얼 영상을 보고 싶으시다면 아래 댓글을 남겨주세요!
위 내용은 Node.JS에 Google 캘린더 API 통합: 이벤트 생성 및 회의 예약 가이드의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
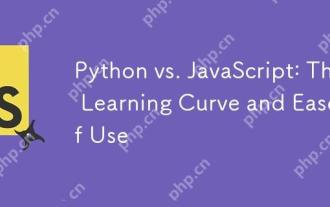
Python은 부드러운 학습 곡선과 간결한 구문으로 초보자에게 더 적합합니다. JavaScript는 가파른 학습 곡선과 유연한 구문으로 프론트 엔드 개발에 적합합니다. 1. Python Syntax는 직관적이며 데이터 과학 및 백엔드 개발에 적합합니다. 2. JavaScript는 유연하며 프론트 엔드 및 서버 측 프로그래밍에서 널리 사용됩니다.
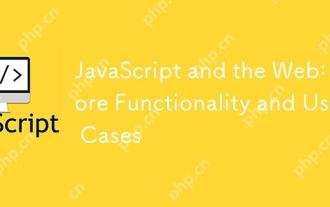
웹 개발에서 JavaScript의 주요 용도에는 클라이언트 상호 작용, 양식 검증 및 비동기 통신이 포함됩니다. 1) DOM 운영을 통한 동적 컨텐츠 업데이트 및 사용자 상호 작용; 2) 사용자가 사용자 경험을 향상시키기 위해 데이터를 제출하기 전에 클라이언트 확인이 수행됩니다. 3) 서버와의 진실한 통신은 Ajax 기술을 통해 달성됩니다.
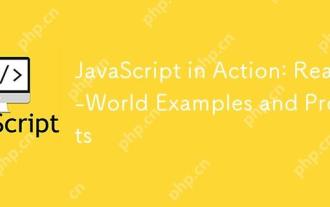
실제 세계에서 JavaScript의 응용 프로그램에는 프론트 엔드 및 백엔드 개발이 포함됩니다. 1) DOM 운영 및 이벤트 처리와 관련된 TODO 목록 응용 프로그램을 구축하여 프론트 엔드 애플리케이션을 표시합니다. 2) Node.js를 통해 RESTFULAPI를 구축하고 Express를 통해 백엔드 응용 프로그램을 시연하십시오.
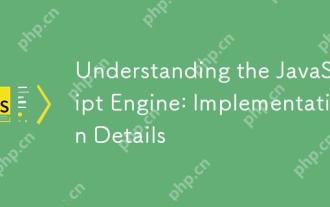
보다 효율적인 코드를 작성하고 성능 병목 현상 및 최적화 전략을 이해하는 데 도움이되기 때문에 JavaScript 엔진이 내부적으로 작동하는 방식을 이해하는 것은 개발자에게 중요합니다. 1) 엔진의 워크 플로에는 구문 분석, 컴파일 및 실행; 2) 실행 프로세스 중에 엔진은 인라인 캐시 및 숨겨진 클래스와 같은 동적 최적화를 수행합니다. 3) 모범 사례에는 글로벌 변수를 피하고 루프 최적화, Const 및 Lets 사용 및 과도한 폐쇄 사용을 피하는 것이 포함됩니다.
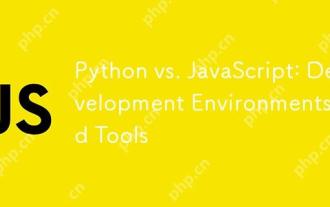
개발 환경에서 Python과 JavaScript의 선택이 모두 중요합니다. 1) Python의 개발 환경에는 Pycharm, Jupyternotebook 및 Anaconda가 포함되어 있으며 데이터 과학 및 빠른 프로토 타이핑에 적합합니다. 2) JavaScript의 개발 환경에는 Node.js, VScode 및 Webpack이 포함되어 있으며 프론트 엔드 및 백엔드 개발에 적합합니다. 프로젝트 요구에 따라 올바른 도구를 선택하면 개발 효율성과 프로젝트 성공률이 향상 될 수 있습니다.
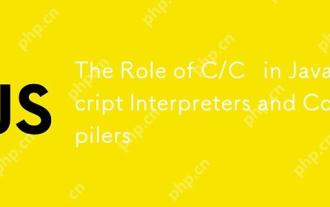
C와 C는 주로 통역사와 JIT 컴파일러를 구현하는 데 사용되는 JavaScript 엔진에서 중요한 역할을합니다. 1) C는 JavaScript 소스 코드를 구문 분석하고 추상 구문 트리를 생성하는 데 사용됩니다. 2) C는 바이트 코드 생성 및 실행을 담당합니다. 3) C는 JIT 컴파일러를 구현하고 런타임에 핫스팟 코드를 최적화하고 컴파일하며 JavaScript의 실행 효율을 크게 향상시킵니다.

Python은 데이터 과학 및 자동화에 더 적합한 반면 JavaScript는 프론트 엔드 및 풀 스택 개발에 더 적합합니다. 1. Python은 데이터 처리 및 모델링을 위해 Numpy 및 Pandas와 같은 라이브러리를 사용하여 데이터 과학 및 기계 학습에서 잘 수행됩니다. 2. 파이썬은 간결하고 자동화 및 스크립팅이 효율적입니다. 3. JavaScript는 프론트 엔드 개발에 없어서는 안될 것이며 동적 웹 페이지 및 단일 페이지 응용 프로그램을 구축하는 데 사용됩니다. 4. JavaScript는 Node.js를 통해 백엔드 개발에 역할을하며 전체 스택 개발을 지원합니다.
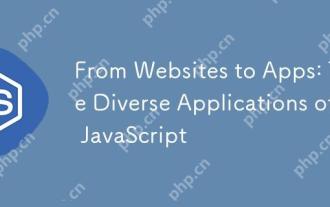
JavaScript는 웹 사이트, 모바일 응용 프로그램, 데스크탑 응용 프로그램 및 서버 측 프로그래밍에서 널리 사용됩니다. 1) 웹 사이트 개발에서 JavaScript는 HTML 및 CSS와 함께 DOM을 운영하여 동적 효과를 달성하고 jQuery 및 React와 같은 프레임 워크를 지원합니다. 2) 반응 및 이온 성을 통해 JavaScript는 크로스 플랫폼 모바일 애플리케이션을 개발하는 데 사용됩니다. 3) 전자 프레임 워크를 사용하면 JavaScript가 데스크탑 애플리케이션을 구축 할 수 있습니다. 4) node.js는 JavaScript가 서버 측에서 실행되도록하고 동시 요청이 높은 높은 요청을 지원합니다.
