useNavigate Hook을 사용하여 React에서 탐색 마스터하기
React에서 Navigate Hook 사용
useNavigate 후크는 React Router(v6 이상)의 일부이며 애플리케이션의 여러 경로를 프로그래밍 방식으로 탐색하는 데 사용됩니다. 기존 탐색(예: 링크 클릭)과 달리 useNavigate 후크를 사용하면 양식 제출, 버튼 클릭 또는 상태 변경과 같은 사용자 작업에 따라 동적으로 탐색할 수 있습니다.
이 후크는 React Router v5의 이전 useHistory 후크를 대체하고 기능 구성 요소 내에서 탐색을 더 쉽게 처리할 수 있게 해줍니다.
Navigate 작동 방식
useNavigate 후크는 프로그래밍 방식으로 특정 경로를 탐색하는 데 사용할 수 있는 함수를 반환합니다. 이 함수에 경로나 위치 개체를 전달할 수 있으며 그에 따라 탐색이 수행됩니다.
구문:
const navigate = useNavigate();
매개변수:
- path(string): 이동할 경로(예: "/home", "/profile/:id").
-
옵션(선택 사항, 개체):
- 교체: 탐색이 현재 기록 항목을 대체해야 하는지 여부를 결정하는 부울입니다(기본값은 false).
- state: 새 경로에 전달할 수 있는 선택적 상태입니다. 이는 대상 경로에 정보를 전달하는 데 유용할 수 있습니다.
일반적인 사용법:
- 다른 경로로 이동하세요.
- 현재 기록 항목을 바꿉니다(뒤로 작업 없음).
- 대상 경로에 추가 상태를 전달합니다.
예: useNavigate를 사용한 기본 탐색
다음은 사용자가 버튼을 클릭할 때 useNavigate 후크를 사용하여 프로그래밍 방식으로 탐색하는 방법에 대한 간단한 예입니다.
import React from 'react'; import { useNavigate } from 'react-router-dom'; const Home = () => { const navigate = useNavigate(); const goToProfile = () => { // Navigate to the profile page navigate('/profile'); }; return ( <div> <h2>Home Page</h2> <button onClick={goToProfile}>Go to Profile</button> </div> ); }; export default Home;
설명:
- useNavigate 후크는 탐색 기능을 가져오는 데 사용됩니다.
- 버튼을 클릭하면 navigate('/profile')를 사용하여 프로그래밍 방식으로 /profile 경로로 이동하는 goToProfile 함수가 호출됩니다.
예: 동적 매개변수를 사용한 탐색
useNavigate를 사용하여 매개변수를 전달하는 동적 경로로 이동할 수도 있습니다.
const navigate = useNavigate();
설명:
- navigate(/user/${userId})는 userId 매개변수를 기반으로 URL을 동적으로 생성합니다.
- 사용자 1 또는 사용자 2에 대한 버튼을 클릭하면 /user/1 또는 /user/2로 이동합니다.
예: 내역 항목을 교체 옵션으로 교체
탐색할 때 바꾸기 옵션을 사용하면 새 항목을 푸시하는 대신 기록 스택의 현재 항목을 바꿀 수 있습니다. 즉, 사용자가 브라우저의 "뒤로" 버튼을 클릭하면 이전 경로로 돌아가지 않습니다.
import React from 'react'; import { useNavigate } from 'react-router-dom'; const Home = () => { const navigate = useNavigate(); const goToProfile = () => { // Navigate to the profile page navigate('/profile'); }; return ( <div> <h2>Home Page</h2> <button onClick={goToProfile}>Go to Profile</button> </div> ); }; export default Home;
설명:
- navigate('/thank-you', { replacement: true })는 /thank-you 경로로 이동하여 기록 스택의 현재 항목을 대체합니다. 즉, 사용자는 다음을 사용하여 양식 제출 페이지로 돌아갈 수 없습니다. "뒤로" 버튼을 누르세요.
예: 탐색으로 상태 전달
내비게이션과 함께 추가 상태를 전달할 수 있으며, 이는 useLocation을 사용하여 대상 경로에서 액세스할 수 있습니다.
import React from 'react'; import { useNavigate } from 'react-router-dom'; const UserList = () => { const navigate = useNavigate(); const goToUserProfile = (userId) => { // Navigate to the profile of a specific user by ID navigate(`/user/${userId}`); }; return ( <div> <h2>User List</h2> <button onClick={() => goToUserProfile(1)}>Go to User 1's Profile</button> <button onClick={() => goToUserProfile(2)}>Go to User 2's Profile</button> </div> ); }; export default UserList;
/settings 경로에서 다음과 같이 전달된 상태에 액세스할 수 있습니다.
import React from 'react'; import { useNavigate } from 'react-router-dom'; const SubmitForm = () => { const navigate = useNavigate(); const handleSubmit = () => { // Perform form submission logic // Then navigate to a "Thank You" page, replacing the current entry in history navigate('/thank-you', { replace: true }); }; return ( <div> <h2>Submit Form</h2> <button onClick={handleSubmit}>Submit</button> </div> ); }; export default SubmitForm;
설명:
- navigate('/settings', { state: { userId: 123, userName: 'John Doe' } })는 객체를 상태로 전달합니다.
- /settings 구성 요소에서는 useLocation을 사용하여 userId 및 userName이 포함된 전달된 상태에 액세스합니다.
일반적인 사용 사례Navigate
양식 제출 후 리디렉션:
양식(예: 사용자 등록)을 제출한 후 사용자를 성공 또는 로그인 페이지로 리디렉션할 수 있습니다.조건부 탐색:
사용자 작업이나 조건(예: 인증)에 따라 동적으로 다른 경로로 이동할 수 있습니다.프로그래밍 방식 라우팅:
작업이 완료되거나 이벤트가 트리거되는 경우와 같은 사용자 지정 논리를 기반으로 프로그래밍 방식으로 탐색할 수 있습니다.API 호출 성공 후 탐색:
성공적인 API 호출(예: 로그인) 후 사용자를 프로필 페이지나 대시보드로 리디렉션할 수 있습니다.
결론
React Router의 useNavigate 후크는 기능 구성 요소에서 프로그래밍 방식 탐색을 처리하기 위한 강력한 도구입니다. 이를 통해 사용자 작업이나 애플리케이션 상태에 따라 동적으로 다른 경로로 이동할 수 있습니다. 교체 및 상태 전달 기능과 같은 옵션을 통해 useNavigate는 React 애플리케이션에서 탐색 동작을 제어하기 위한 유연성을 제공합니다.
위 내용은 useNavigate Hook을 사용하여 React에서 탐색 마스터하기의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










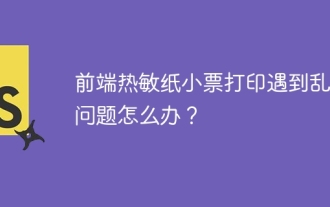
프론트 엔드 개발시 프론트 엔드 열지대 티켓 인쇄를위한 자주 묻는 질문과 솔루션, 티켓 인쇄는 일반적인 요구 사항입니다. 그러나 많은 개발자들이 구현하고 있습니다 ...
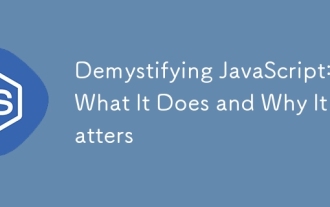
JavaScript는 현대 웹 개발의 초석이며 주요 기능에는 이벤트 중심 프로그래밍, 동적 컨텐츠 생성 및 비동기 프로그래밍이 포함됩니다. 1) 이벤트 중심 프로그래밍을 사용하면 사용자 작업에 따라 웹 페이지가 동적으로 변경 될 수 있습니다. 2) 동적 컨텐츠 생성을 사용하면 조건에 따라 페이지 컨텐츠를 조정할 수 있습니다. 3) 비동기 프로그래밍은 사용자 인터페이스가 차단되지 않도록합니다. JavaScript는 웹 상호 작용, 단일 페이지 응용 프로그램 및 서버 측 개발에 널리 사용되며 사용자 경험 및 크로스 플랫폼 개발의 유연성을 크게 향상시킵니다.
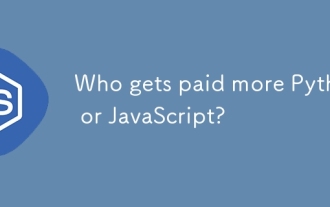
기술 및 산업 요구에 따라 Python 및 JavaScript 개발자에 대한 절대 급여는 없습니다. 1. 파이썬은 데이터 과학 및 기계 학습에서 더 많은 비용을 지불 할 수 있습니다. 2. JavaScript는 프론트 엔드 및 풀 스택 개발에 큰 수요가 있으며 급여도 상당합니다. 3. 영향 요인에는 경험, 지리적 위치, 회사 규모 및 특정 기술이 포함됩니다.
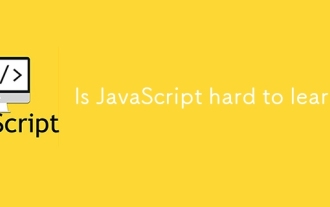
JavaScript를 배우는 것은 어렵지 않지만 어려운 일입니다. 1) 변수, 데이터 유형, 기능 등과 같은 기본 개념을 이해합니다. 2) 마스터 비동기 프로그래밍 및 이벤트 루프를 통해이를 구현하십시오. 3) DOM 운영을 사용하고 비동기 요청을 처리합니다. 4) 일반적인 실수를 피하고 디버깅 기술을 사용하십시오. 5) 성능을 최적화하고 모범 사례를 따르십시오.
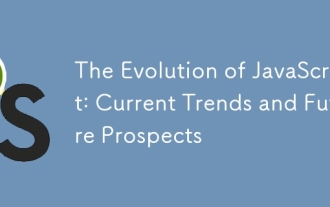
JavaScript의 최신 트렌드에는 Typescript의 Rise, 현대 프레임 워크 및 라이브러리의 인기 및 WebAssembly의 적용이 포함됩니다. 향후 전망은보다 강력한 유형 시스템, 서버 측 JavaScript 개발, 인공 지능 및 기계 학습의 확장, IoT 및 Edge 컴퓨팅의 잠재력을 포함합니다.
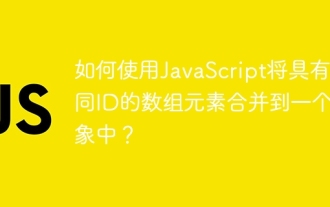
동일한 ID로 배열 요소를 JavaScript의 하나의 객체로 병합하는 방법은 무엇입니까? 데이터를 처리 할 때 종종 동일한 ID를 가질 필요가 있습니다 ...
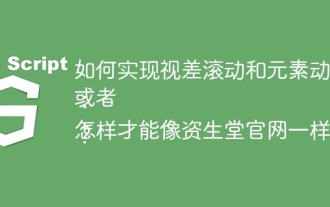
이 기사에서 시차 스크롤 및 요소 애니메이션 효과 실현에 대한 토론은 Shiseido 공식 웹 사이트 (https://www.shiseido.co.jp/sb/wonderland/)와 유사하게 달성하는 방법을 살펴볼 것입니다.
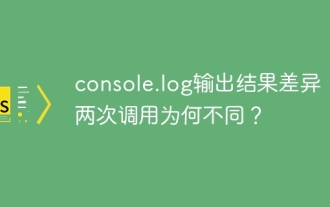
Console.log 출력의 차이의 근본 원인에 대한 심층적 인 논의. 이 기사에서는 Console.log 함수의 출력 결과의 차이점을 코드에서 분석하고 그에 따른 이유를 설명합니다. � ...
