Go에서 LLM으로 전송된 토큰 수 계산(1부)
소개
몇 주 전 저는 비즈니스 파트너 회사의 CFO와 자사 솔루션 내에서 watsonx.ai 기능 구현에 관해 논의했습니다. 비용에 대한 논의 중에 "토큰"이라는 단어를 발음했는데 갑자기 패닉이 일어났습니다 ?
토큰이 무엇인지 설명한 후 질문이 생겼습니다. “우리가 보내고 받는 토큰을 어떻게 계산하나요? 비용이 얼마나 드나요?”
답은 아주 쉬웠습니다. 우리는 watsonx.ai 스튜디오 프롬프트 랩에 가서 몇 가지 간단한 프롬프트를 가지고 왔다 갔다 했고 거기에서 토큰의 수를 확인했습니다. 또한 간단한 입력을 통해 LLM에 보내는 토큰 수를 확인할 수 있는 매우 멋진 웹사이트도 보여주었습니다.
나중에 저는 스스로 토큰 카운터 애플리케이션을 만들어 보는 것이 어떨까요(그리고 오랫동안 Golang을 사용하지 않았기 때문에 Go 언어로 작성하려는 의도였습니다!)라고 생각했습니다. 글쎄요, 그보다 좀 더 복잡할 것 같은데요?
첫 번째 시도 — Regex 사용
처음에는 Regex를 사용하면 어느 정도 만족스러운 결과를 얻을 수 있겠다는 생각이 들었습니다.
다음 Go 앱을 설정했습니다.
package main import ( "bufio" "fmt" "log" "os" "regexp" "strings" "github.com/sqweek/dialog" ) // countTokens approximates the number of tokens in a text based on whitespace and punctuation. func countTokens(text string) int { // A simple regex to split text into words and punctuation tokenizer := regexp.MustCompile(`\w+|[^\w\s]`) tokens := tokenizer.FindAllString(text, -1) return len(tokens) } func main() { // Open a file dialog box and let the user select a text file filePath, err := dialog.File().Filter("Text Files", "txt").Load() if err != nil { if err.Error() == "Cancelled" { fmt.Println("File selection was cancelled.") return } log.Fatalf("Error selecting file: %v", err) } // Output the selected file name fmt.Printf("Selected file: %s\n", filePath) // Specify the file to read //filePath := "input.txt" // Open the file file, err := os.Open(filePath) if err != nil { fmt.Printf("Error opening file: %v\n", err) return } defer file.Close() // Read the file line by line var content strings.Builder scanner := bufio.NewScanner(file) for scanner.Scan() { content.WriteString(scanner.Text()) content.WriteString("\n") } if err := scanner.Err(); err != nil { fmt.Printf("Error reading file: %v\n", err) return } // Get the text content text := content.String() // Count the tokens tokenCount := countTokens(text) // Output the result fmt.Printf("The file contains approximately %d tokens.\n", tokenCount) }
아시겠지만 제가 GUI와 대화 상자를 좋아해서 입력 텍스트 파일을 선택할 수 있는 대화 상자를 구현했습니다.
여기 텍스트 파일이 있습니다(내가 찾은 임의의 텍스트?).
The popularity of the Rust language continues to explode; yet, many critical codebases remain authored in C, and cannot be realistically rewritten by hand. Automatically translating C to Rust is thus an appealing course of action. Several works have gone down this path, handling an ever-increasing subset of C through a variety of Rust features, such as unsafe. While the prospect of automation is appealing, producing code that relies on unsafe negates the memory safety guarantees offered by Rust, and therefore the main advantages of porting existing codebases to memory-safe languages. We instead explore a different path, and explore what it would take to translate C to safe Rust; that is, to produce code that is trivially memory safe, because it abides by Rust's type system without caveats. Our work sports several original contributions: a type-directed translation from (a subset of) C to safe Rust; a novel static analysis based on "split trees" that allows expressing C's pointer arithmetic using Rust's slices and splitting operations; an analysis that infers exactly which borrows need to be mutable; and a compilation strategy for C's struct types that is compatible with Rust's distinction between non-owned and owned allocations. We apply our methodology to existing formally verified C codebases: the HACL* cryptographic library, and binary parsers and serializers from EverParse, and show that the subset of C we support is sufficient to translate both applications to safe Rust. Our evaluation shows that for the few places that do violate Rust's aliasing discipline, automated, surgical rewrites suffice; and that the few strategic copies we insert have a negligible performance impact. Of particular note, the application of our approach to HACL* results in a 80,000 line verified cryptographic library, written in pure Rust, that implements all modern algorithms - the first of its kind.
코드를 실행하면 다음과 같은 결과가 나타납니다.
The file contains approximately 359 tokens.
괜찮을 것 같긴 한데, 음… 그렇긴 한데… 어떤 모델과 비교하면?? 그리고 Regex를 구현하는 방법도 다양하므로 이 방법은 전혀 포함되지 않습니다 ?!
두 번째 시도 - 특정 모델에 대해 실행
내가 알아낸 것은 특정 LLM에 특정 "토크나이저"를 사용하지 않는 한 이전 방법은 정확하지 않다는 것입니다. 그래서 나는 현재 시중에 나와 있는 gpt 3.5와 같은 모델에 대해 어떻게 하면 정확한 결과를 얻을 수 있는지 알아보기 시작했습니다. 인터넷에서 좀 조사한 끝에 제가 만든 앱이 나왔습니다.
package main import ( "bufio" "bytes" "fmt" "log" "os" "os/exec" "github.com/joho/godotenv" "github.com/sqweek/dialog" ) func main() { // Open a file dialog box and let the user select a text file filePath, err := dialog.File().Filter("Text Files", "txt").Load() if err != nil { if err.Error() == "Cancelled" { fmt.Println("File selection was cancelled.") return } log.Fatalf("Error selecting file: %v", err) } // Output the selected file name fmt.Printf("Selected file: %s\n", filePath) // Open the file file, err := os.Open(filePath) if err != nil { fmt.Printf("Error opening file: %v\n", err) return } defer file.Close() // Read the file content var content bytes.Buffer scanner := bufio.NewScanner(file) for scanner.Scan() { content.WriteString(scanner.Text()) content.WriteString("\n") } if err := scanner.Err(); err != nil { fmt.Printf("Error reading file: %v\n", err) return } // Specify the model model := "gpt-3.5-turbo" // Execute the Python script cmd := exec.Command("python3", "tokenizer.py", model) cmd.Stdin = bytes.NewReader(content.Bytes()) output, err := cmd.Output() if err != nil { fmt.Printf("Error running tokenizer script: %v\n", err) return } // Print the token count fmt.Printf("Token count: %s", output) }
위 코드에서 볼 수 있듯이 Microsoft 사이트에서 찾은 Python 앱에 대한 호출이 있습니다. 이 앱은 (구현되었기 때문에) "tiktoken” 라이브러리를 사용하여 gpt에 대한 토큰 수를 결정합니다! 모델명도 하드코딩 되어있습니다.
import sys from tiktoken import encoding_for_model def count_tokens(model, text): enc = encoding_for_model(model) tokens = enc.encode(text) return len(tokens) if __name__ == "__main__": # Read model name and text from stdin model = sys.argv[1] # E.g., "gpt-3.5-turbo" text = sys.stdin.read() print(count_tokens(model, text))
잘 작동합니다. 이전에 제공된 동일한 텍스트에 대해 이제 내가 찾은 모든 웹사이트에 대해 정확한 366개의 토큰 수를 얻었고 모델을 GPT 3.5
로 설정했습니다.내가 작성하고 싶은 것은 "Golang"에 완전히 포함된 코드입니다... 그리고 Huggingface에서 찾을 수 있는 모든 모델(또는 거의 모든 모델)에서 실행할 수 있기를 원합니다. ibm-granite/granite-3.1–8b-instruct) ?
이 글의 2부(WIP)입니다.
지금까지 다음을 탐색 중입니다(좋죠?) Github repos;
- 토크나이저: https://github.com/sugarme/tokenizer
- 토크나이저: https://github.com/daulet/tokenizers
- 그리고 마지막으로 중요한 것은 -> 고허깅페이스: https://github.com/gomlx/go-huggingface?tab=readme-ov-file
결론
읽어주셔서 감사하고 의견을 남겨주세요.
그리고 두 번째 앱이 나올 때까지 지켜봐주세요... ?
위 내용은 Go에서 LLM으로 전송된 토큰 수 계산(1부)의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










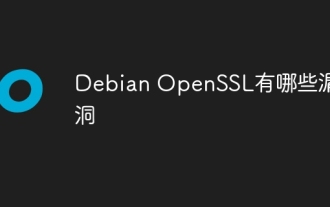
보안 통신에 널리 사용되는 오픈 소스 라이브러리로서 OpenSSL은 암호화 알고리즘, 키 및 인증서 관리 기능을 제공합니다. 그러나 역사적 버전에는 알려진 보안 취약점이 있으며 그 중 일부는 매우 유해합니다. 이 기사는 데비안 시스템의 OpenSSL에 대한 일반적인 취약점 및 응답 측정에 중점을 둘 것입니다. DebianopensSL 알려진 취약점 : OpenSSL은 다음과 같은 몇 가지 심각한 취약점을 경험했습니다. 심장 출혈 취약성 (CVE-2014-0160) :이 취약점은 OpenSSL 1.0.1 ~ 1.0.1F 및 1.0.2 ~ 1.0.2 베타 버전에 영향을 미칩니다. 공격자는이 취약점을 사용하여 암호화 키 등을 포함하여 서버에서 무단 읽기 민감한 정보를 사용할 수 있습니다.
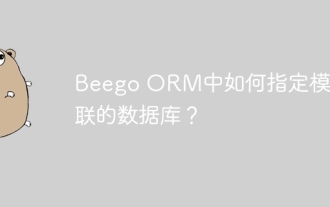
Beegoorm 프레임 워크에서 모델과 관련된 데이터베이스를 지정하는 방법은 무엇입니까? 많은 Beego 프로젝트에서는 여러 데이터베이스를 동시에 작동해야합니다. Beego를 사용할 때 ...
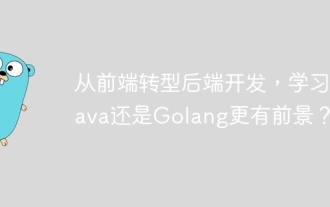
백엔드 학습 경로 : 프론트 엔드에서 백엔드 초보자로서 프론트 엔드에서 백엔드까지의 탐사 여행은 프론트 엔드 개발에서 변화하는 백엔드 초보자로서 이미 Nodejs의 기초를 가지고 있습니다.
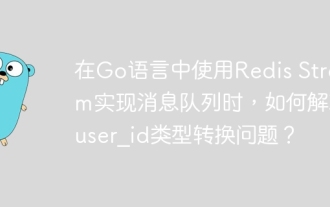
Go Language에서 메시지 대기열을 구현하기 위해 Redisstream을 사용하는 문제는 Go Language와 Redis를 사용하는 것입니다 ...
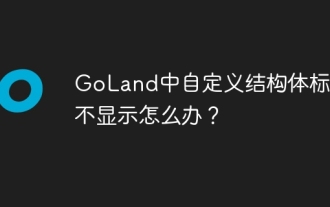
골란드의 사용자 정의 구조 레이블이 표시되지 않으면 어떻게해야합니까? Go Language 개발을 위해 Goland를 사용할 때 많은 개발자가 사용자 정의 구조 태그를 만날 것입니다 ...
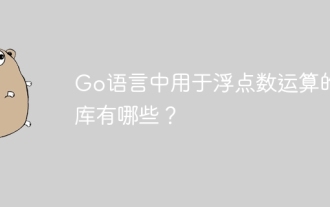
Go Language의 부동 소수점 번호 작동에 사용되는 라이브러리는 정확도를 보장하는 방법을 소개합니다.
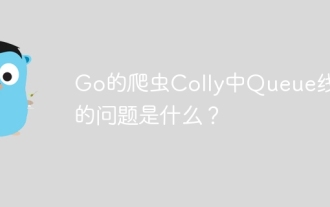
Go Crawler Colly의 대기열 스레딩 문제는 Colly Crawler 라이브러리를 GO 언어로 사용하는 문제를 탐구합니다. � ...

이 기사는 데비안 시스템에서 MongoDB를 구성하여 자동 확장을 달성하는 방법을 소개합니다. 주요 단계에는 MongoDB 복제 세트 및 디스크 공간 모니터링 설정이 포함됩니다. 1. MongoDB 설치 먼저 MongoDB가 데비안 시스템에 설치되어 있는지 확인하십시오. 다음 명령을 사용하여 설치하십시오. sudoaptupdatesudoaptinstall-imongb-org 2. MongoDB Replica 세트 MongoDB Replica 세트 구성은 자동 용량 확장을 달성하기위한 기초 인 고 가용성 및 데이터 중복성을 보장합니다. MongoDB 서비스 시작 : sudosystemctlstartMongodsudosys
