Go에서 MongoDB 문서를 언마샬링할 때 Null 값을 무시하는 방법은 무엇입니까?
MongoDB 문서 언마샬링 중 Null 값 무시
MongoDB 문서를 Null을 허용하지 않는 문자열 필드가 포함된 Go 구조체로 언마샬링할 때 null이 발생함 문서의 값에 오류가 발생할 수 있습니다. 이 문제를 해결하려면 언마샬링 중에 이러한 null 값을 무시하는 방법을 찾아야 합니다.
사용자 정의 디코더 사용
null 값을 처리하는 한 가지 접근 방식은 다음과 같습니다. 문자열 유형에 대한 사용자 정의 디코더를 생성합니다. 이 사용자 정의 디코더는 null 값을 인식하고 해당 필드를 빈 문자열로 설정하여 이를 처리하며 null 값을 효과적으로 무시합니다.
import ( "go.mongodb.org/mongo-driver/bson/bsoncodec" "go.mongodb.org/mongo-driver/bson/bsonrw" "go.mongodb.org/mongo-driver/bson/bsontype" ) type nullawareStrDecoder struct{} func (nullawareStrDecoder) DecodeValue(dctx bsoncodec.DecodeContext, vr bsonrw.ValueReader, val reflect.Value) error { if !val.CanSet() || val.Kind() != reflect.String { return errors.New("bad type or not settable") } var str string var err error switch vr.Type() { case bsontype.String: if str, err = vr.ReadString(); err != nil { return err } case bsontype.Null: if err = vr.ReadNull(); err != nil { return err } default: return fmt.Errorf("cannot decode %v into a string type", vr.Type()) } val.SetString(str) return nil }
그런 다음 이 사용자 정의 디코더를 bsoncodec.Registry에 등록하고 다음에 적용할 수 있습니다. mongo.Client 객체:
clientOpts := options.Client(). ApplyURI("mongodb://localhost:27017/"). SetRegistry( bson.NewRegistryBuilder(). RegisterDecoder(reflect.TypeOf(""), nullawareStrDecoder{}). Build(), ) client, err := mongo.Connect(ctx, clientOpts)
유형 중립 Null 인식 생성 디코더
여러 유형의 null 값을 처리하기 위해 null 값을 확인하고, 발견되면 해당 필드를 해당 필드의 0 값으로 설정하는 단일 유형 중립 디코더를 생성할 수 있습니다. 유형:
type nullawareDecoder struct { defDecoder bsoncodec.ValueDecoder zeroValue reflect.Value } func (d *nullawareDecoder) DecodeValue(dctx bsoncodec.DecodeContext, vr bsonrw.ValueReader, val reflect.Value) error { if vr.Type() != bsontype.Null { return d.defDecoder.DecodeValue(dctx, vr, val) } if !val.CanSet() { return errors.New("value not settable") } if err := vr.ReadNull(); err != nil { return err } val.Set(d.zeroValue) return nil }
이 디코더는 특정 유형 또는 모든 유형에 대해 bsoncodec.Registry에 등록할 수 있습니다. 유형:
customValues := []interface{}{ "", // string int(0), // int int32(0), // int32 } rb := bson.NewRegistryBuilder() for _, v := range customValues { t := reflect.TypeOf(v) defDecoder, err := bson.DefaultRegistry.LookupDecoder(t) if err != nil { panic(err) } rb.RegisterDecoder(t, &nullawareDecoder{defDecoder, reflect.Zero(t)}) }
위 내용은 Go에서 MongoDB 문서를 언마샬링할 때 Null 값을 무시하는 방법은 무엇입니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










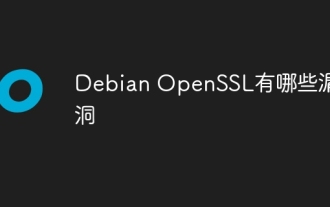
보안 통신에 널리 사용되는 오픈 소스 라이브러리로서 OpenSSL은 암호화 알고리즘, 키 및 인증서 관리 기능을 제공합니다. 그러나 역사적 버전에는 알려진 보안 취약점이 있으며 그 중 일부는 매우 유해합니다. 이 기사는 데비안 시스템의 OpenSSL에 대한 일반적인 취약점 및 응답 측정에 중점을 둘 것입니다. DebianopensSL 알려진 취약점 : OpenSSL은 다음과 같은 몇 가지 심각한 취약점을 경험했습니다. 심장 출혈 취약성 (CVE-2014-0160) :이 취약점은 OpenSSL 1.0.1 ~ 1.0.1F 및 1.0.2 ~ 1.0.2 베타 버전에 영향을 미칩니다. 공격자는이 취약점을 사용하여 암호화 키 등을 포함하여 서버에서 무단 읽기 민감한 정보를 사용할 수 있습니다.
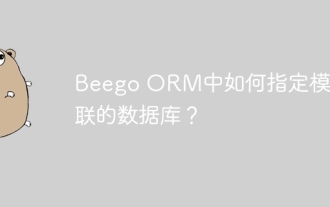
Beegoorm 프레임 워크에서 모델과 관련된 데이터베이스를 지정하는 방법은 무엇입니까? 많은 Beego 프로젝트에서는 여러 데이터베이스를 동시에 작동해야합니다. Beego를 사용할 때 ...
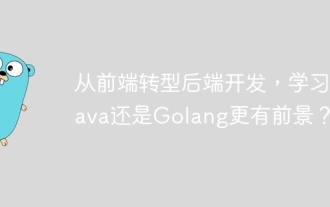
백엔드 학습 경로 : 프론트 엔드에서 백엔드 초보자로서 프론트 엔드에서 백엔드까지의 탐사 여행은 프론트 엔드 개발에서 변화하는 백엔드 초보자로서 이미 Nodejs의 기초를 가지고 있습니다.
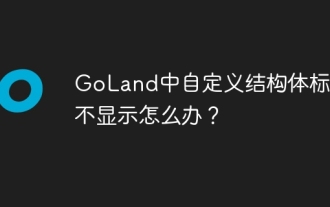
골란드의 사용자 정의 구조 레이블이 표시되지 않으면 어떻게해야합니까? Go Language 개발을 위해 Goland를 사용할 때 많은 개발자가 사용자 정의 구조 태그를 만날 것입니다 ...
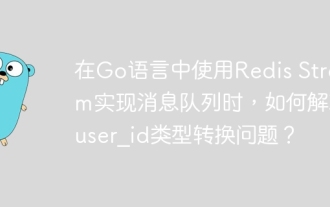
Go Language에서 메시지 대기열을 구현하기 위해 Redisstream을 사용하는 문제는 Go Language와 Redis를 사용하는 것입니다 ...
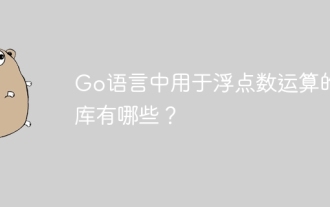
Go Language의 부동 소수점 번호 작동에 사용되는 라이브러리는 정확도를 보장하는 방법을 소개합니다.
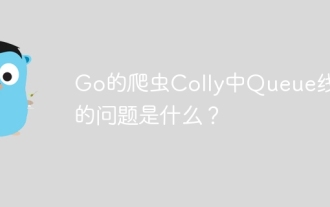
Go Crawler Colly의 대기열 스레딩 문제는 Colly Crawler 라이브러리를 GO 언어로 사용하는 문제를 탐구합니다. � ...
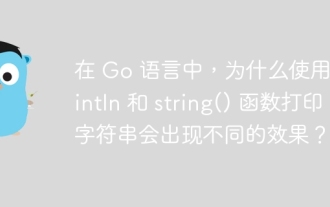
Go Language의 문자열 인쇄의 차이 : println 및 String () 함수 사용 효과의 차이가 진행 중입니다 ...
