스트리밍 및 동적 데이터를 사용한 고급 React SSR 기술
애플리케이션이 성장할수록 과제도 늘어납니다. 앞서 나가려면 원활한 고성능 사용자 경험을 제공하기 위해 고급 SSR 기술을 익히는 것이 필수적입니다.
이전 기사에서 React 프로젝트에서 서버 측 렌더링을 위한 기반을 구축한 후 프로젝트 확장성을 유지하고, 서버에서 클라이언트로 데이터를 효율적으로 로드하고, 하이드레이션 문제를 해결하는 데 도움이 되는 기능을 공유하게 되어 기쁩니다.
목차
- SSR 스트리밍이란
- 지연 로딩과 SSR
-
지연 로딩으로 스트리밍 구현
- React 구성 요소 업데이트
- 스트리밍을 위한 서버 업데이트
-
서버-클라이언트 데이터
- 서버에 데이터 전달
- 클라이언트에서 환경 변수 처리
-
수분 공급 문제
- 예시 시나리오
- 수분 문제 해결
- 결론
SSR 스트리밍이란?
서버 측 렌더링(SSR) 스트리밍은 전체 페이지가 준비될 때까지 기다리지 않고 서버가 생성되는 HTML 페이지의 일부를 청크로 브라우저에 보내는 기술입니다. 배달하기 전에. 이를 통해 브라우저는 콘텐츠 렌더링을 즉시 시작할 수 있어 로드 시간과 사용자 성능이 향상됩니다.
스트리밍은 다음과 같은 경우에 특히 효과적입니다.
- 대형 페이지: 전체 HTML을 생성하는 데 상당한 시간이 걸릴 수 있습니다.
- 동적 콘텐츠: 페이지의 일부가 외부 API 호출이나 동적으로 생성된 청크에 의존하는 경우.
- 트래픽이 많은 애플리케이션: 사용량이 가장 많은 동안 서버 로드와 대기 시간을 줄입니다.
스트리밍은 기존 SSR과 최신 클라이언트측 상호 작용 간의 격차를 해소하여 사용자가 성능 저하 없이 의미 있는 콘텐츠를 더 빠르게 볼 수 있도록 합니다.
지연 로딩과 SSR
지연 로딩은 구성요소나 모듈이 실제로 필요할 때까지 로딩을 연기하여 초기 로딩 시간을 줄이고 성능을 향상시키는 기술입니다. SSR과 결합하면 지연 로딩이 서버와 클라이언트 워크로드를 모두 크게 최적화할 수 있습니다.
지연 로딩은 구성 요소를 Promise로 동적으로 가져오는 React.lazy를 사용합니다. 기존 SSR에서는 렌더링이 동기식입니다. 즉, 전체 HTML을 생성하여 브라우저에 보내기 전에 서버가 모든 약속을 해결해야 합니다.
스트리밍은 구성 요소가 렌더링될 때 서버가 HTML을 청크로 전송할 수 있도록 하여 이러한 문제를 해결합니다. 이 접근 방식을 사용하면 Suspense 폴백이 즉시 브라우저로 전송되어 사용자가 의미 있는 콘텐츠를 조기에 볼 수 있습니다. 지연 로드된 구성 요소가 해결되면 렌더링된 HTML이 점진적으로 브라우저로 스트리밍되어 대체 콘텐츠가 원활하게 대체됩니다. 이렇게 하면 렌더링 프로세스가 차단되는 것을 방지하고, 지연을 줄이고, 인지된 로드 시간을 개선할 수 있습니다.
지연 로딩으로 스트리밍 구현
이 가이드는 이전 기사인 생산 가능한 SSR React 애플리케이션 구축에서 소개된 개념을 기반으로 하며, 해당 기사는 하단에 링크되어 있습니다. React에서 SSR을 활성화하고 지연 로드 구성 요소를 지원하기 위해 React 구성 요소와 서버 모두에 몇 가지 업데이트를 적용할 예정입니다.
React 구성 요소 업데이트
서버 진입점
React의 renderToString 메서드는 SSR에 일반적으로 사용되지만 전체 HTML 콘텐츠가 준비될 때까지 기다렸다가 브라우저에 보냅니다. renderToPipeableStream으로 전환하면 생성되는 HTML의 일부를 보내는 스트리밍을 활성화할 수 있습니다.
// ./src/entry-server.tsx import { renderToPipeableStream, RenderToPipeableStreamOptions } from 'react-dom/server' import App from './App' export function render(options?: RenderToPipeableStreamOptions) { return renderToPipeableStream(<App />, options) }
지연 로드 구성요소 생성
이 예에서는 개념을 보여주기 위해 간단한 카드 구성 요소를 만들어 보겠습니다. 프로덕션 애플리케이션에서 이 기술은 일반적으로 성능을 최적화하기 위해 더 큰 모듈이나 전체 페이지에 사용됩니다.
// ./src/Card.tsx import { useState } from 'react' function Card() { const [count, setCount] = useState(0) return ( <div className="card"> <button onClick={() => setCount((count) => count + 1)}> count is {count} </button> <p> Edit <code>src/App.tsx</code> and save to test HMR </p> </div> ) } export default Card
앱에서 지연 로드 구성요소 사용
지연 로드 구성 요소를 사용하려면 React.lazy를 사용하여 동적으로 가져오고 Suspense로 래핑하여 로드 중에 대체 UI를 제공하세요
// ./src/App.tsx import { lazy, Suspense } from 'react' import reactLogo from './assets/react.svg' import viteLogo from '/vite.svg' import './App.css' const Card = lazy(() => import('./Card')) function App() { return ( <> <div> <a href="https://vite.dev" target="_blank"> <img src={viteLogo} className="logo" alt="Vite logo" /> </a> <a href="https://react.dev" target="_blank"> <img src={reactLogo} className="logo react" alt="React logo" /> </a> </div> <h1>Vite + React</h1> <Suspense fallback='Loading...'> <Card /> </Suspense> <p className="read-the-docs"> Click on the Vite and React logos to learn more </p> </> ) } export default App
스트리밍을 위해 서버 업데이트
스트리밍을 활성화하려면 개발 및 프로덕션 설정 모두 일관된 HTML 렌더링 프로세스를 지원해야 합니다. 두 환경 모두 프로세스가 동일하므로 재사용 가능한 단일 기능을 생성하여 스트리밍 콘텐츠를 효과적으로 처리할 수 있습니다.
스트림 콘텐츠 함수 생성
// ./server/constants.ts export const ABORT_DELAY = 5000
streamContent 함수는 렌더링 프로세스를 시작하고, HTML의 증분 청크를 응답에 쓰고, 적절한 오류 처리를 보장합니다.
// ./server/streamContent.ts import { Transform } from 'node:stream' import { Request, Response, NextFunction } from 'express' import { ABORT_DELAY, HTML_KEY } from './constants' import type { render } from '../src/entry-server' export type StreamContentArgs = { render: typeof render html: string req: Request res: Response next: NextFunction } export function streamContent({ render, html, res }: StreamContentArgs) { let renderFailed = false // Initiates the streaming process by calling the render function const { pipe, abort } = render({ // Handles errors that occur before the shell is ready onShellError() { res.status(500).set({ 'Content-Type': 'text/html' }).send('<pre class="brush:php;toolbar:false">Something went wrong') }, // Called when the shell (initial HTML) is ready for streaming onShellReady() { res.status(renderFailed ? 500 : 200).set({ 'Content-Type': 'text/html' }) // Split the HTML into two parts using the placeholder const [htmlStart, htmlEnd] = html.split(HTML_KEY) // Write the starting part of the HTML to the response res.write(htmlStart) // Create a transform stream to handle the chunks of HTML from the renderer const transformStream = new Transform({ transform(chunk, encoding, callback) { // Write each chunk to the response res.write(chunk, encoding) callback() }, }) // When the streaming is finished, write the closing part of the HTML transformStream.on('finish', () => { res.end(htmlEnd) }) // Pipe the render output through the transform stream pipe(transformStream) }, onError(error) { // Logs errors encountered during rendering renderFailed = true console.error((error as Error).stack) }, }) // Abort the rendering process after a delay to avoid hanging requests setTimeout(abort, ABORT_DELAY) }
개발 구성 업데이트 중
// ./server/dev.ts import { Application } from 'express' import fs from 'fs' import path from 'path' import { StreamContentArgs } from './streamContent' const HTML_PATH = path.resolve(process.cwd(), 'index.html') const ENTRY_SERVER_PATH = path.resolve(process.cwd(), 'src/entry-server.tsx') // Add to args the streamContent callback export async function setupDev(app: Application, streamContent: (args: StreamContentArgs) => void) { const vite = await ( await import('vite') ).createServer({ root: process.cwd(), server: { middlewareMode: true }, appType: 'custom', }) app.use(vite.middlewares) app.get('*', async (req, res, next) => { try { let html = fs.readFileSync(HTML_PATH, 'utf-8') html = await vite.transformIndexHtml(req.originalUrl, html) const { render } = await vite.ssrLoadModule(ENTRY_SERVER_PATH) // Use the same callback for production and development process streamContent({ render, html, req, res, next }) } catch (e) { vite.ssrFixStacktrace(e as Error) console.error((e as Error).stack) next(e) } }) }
프로덕션 구성 업데이트 중
// ./server/prod.ts import { Application } from 'express' import fs from 'fs' import path from 'path' import compression from 'compression' import sirv from 'sirv' import { StreamContentArgs } from './streamContent' const CLIENT_PATH = path.resolve(process.cwd(), 'dist/client') const HTML_PATH = path.resolve(process.cwd(), 'dist/client/index.html') const ENTRY_SERVER_PATH = path.resolve(process.cwd(), 'dist/ssr/entry-server.js') // Add to Args the streamContent callback export async function setupProd(app: Application, streamContent: (args: StreamContentArgs) => void) { app.use(compression()) app.use(sirv(CLIENT_PATH, { extensions: [] })) app.get('*', async (req, res, next) => { try { const html = fs.readFileSync(HTML_PATH, 'utf-8') const { render } = await import(ENTRY_SERVER_PATH) // Use the same callback for production and development process streamContent({ render, html, req, res, next }) } catch (e) { console.error((e as Error).stack) next(e) } }) }
익스프레스 서버 업데이트
streamContent 함수를 각 구성에 전달합니다.
// ./server/app.ts import express from 'express' import { PROD, APP_PORT } from './constants' import { setupProd } from './prod' import { setupDev } from './dev' import { streamContent } from './streamContent' export async function createServer() { const app = express() if (PROD) { await setupProd(app, streamContent) } else { await setupDev(app, streamContent) } app.listen(APP_PORT, () => { console.log(`http://localhost:${APP_PORT}`) }) } createServer()
이러한 변경 사항을 구현한 후 서버는 다음을 수행합니다.
- HTML을 브라우저로 점진적으로 스트리밍하여 첫 번째 페인트 시간을 단축합니다.
- 지연 로드 구성요소를 원활하게 처리하여 성능과 사용자 경험을 모두 향상시킵니다.
서버-클라이언트 데이터
HTML을 클라이언트에 보내기 전에 서버에서 생성된 HTML을 완전히 제어할 수 있습니다. 이를 통해 필요에 따라 태그, 스타일, 링크 또는 기타 요소를 추가하여 구조를 동적으로 수정할 수 있습니다.
특히 강력한 기술 중 하나는

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
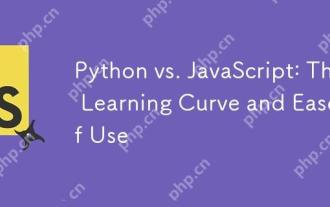
Python은 부드러운 학습 곡선과 간결한 구문으로 초보자에게 더 적합합니다. JavaScript는 가파른 학습 곡선과 유연한 구문으로 프론트 엔드 개발에 적합합니다. 1. Python Syntax는 직관적이며 데이터 과학 및 백엔드 개발에 적합합니다. 2. JavaScript는 유연하며 프론트 엔드 및 서버 측 프로그래밍에서 널리 사용됩니다.
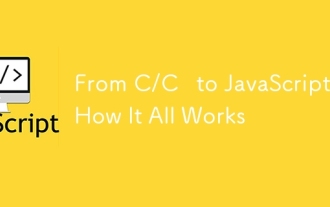
C/C에서 JavaScript로 전환하려면 동적 타이핑, 쓰레기 수집 및 비동기 프로그래밍으로 적응해야합니다. 1) C/C는 수동 메모리 관리가 필요한 정적으로 입력 한 언어이며 JavaScript는 동적으로 입력하고 쓰레기 수집이 자동으로 처리됩니다. 2) C/C를 기계 코드로 컴파일 해야하는 반면 JavaScript는 해석 된 언어입니다. 3) JavaScript는 폐쇄, 프로토 타입 체인 및 약속과 같은 개념을 소개하여 유연성과 비동기 프로그래밍 기능을 향상시킵니다.
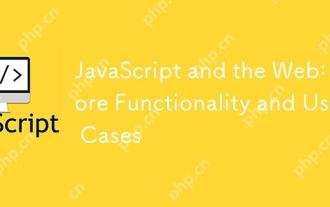
웹 개발에서 JavaScript의 주요 용도에는 클라이언트 상호 작용, 양식 검증 및 비동기 통신이 포함됩니다. 1) DOM 운영을 통한 동적 컨텐츠 업데이트 및 사용자 상호 작용; 2) 사용자가 사용자 경험을 향상시키기 위해 데이터를 제출하기 전에 클라이언트 확인이 수행됩니다. 3) 서버와의 진실한 통신은 Ajax 기술을 통해 달성됩니다.
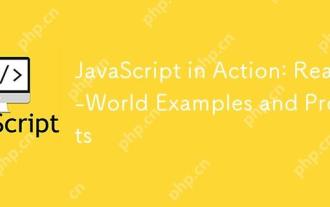
실제 세계에서 JavaScript의 응용 프로그램에는 프론트 엔드 및 백엔드 개발이 포함됩니다. 1) DOM 운영 및 이벤트 처리와 관련된 TODO 목록 응용 프로그램을 구축하여 프론트 엔드 애플리케이션을 표시합니다. 2) Node.js를 통해 RESTFULAPI를 구축하고 Express를 통해 백엔드 응용 프로그램을 시연하십시오.
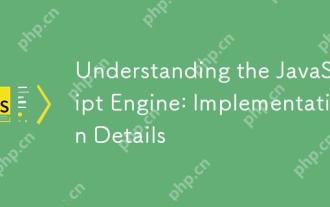
보다 효율적인 코드를 작성하고 성능 병목 현상 및 최적화 전략을 이해하는 데 도움이되기 때문에 JavaScript 엔진이 내부적으로 작동하는 방식을 이해하는 것은 개발자에게 중요합니다. 1) 엔진의 워크 플로에는 구문 분석, 컴파일 및 실행; 2) 실행 프로세스 중에 엔진은 인라인 캐시 및 숨겨진 클래스와 같은 동적 최적화를 수행합니다. 3) 모범 사례에는 글로벌 변수를 피하고 루프 최적화, Const 및 Lets 사용 및 과도한 폐쇄 사용을 피하는 것이 포함됩니다.
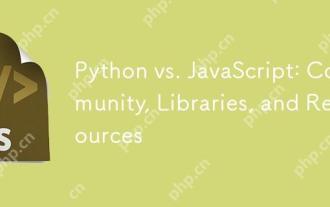
Python과 JavaScript는 커뮤니티, 라이브러리 및 리소스 측면에서 고유 한 장점과 단점이 있습니다. 1) Python 커뮤니티는 친절하고 초보자에게 적합하지만 프론트 엔드 개발 리소스는 JavaScript만큼 풍부하지 않습니다. 2) Python은 데이터 과학 및 기계 학습 라이브러리에서 강력하며 JavaScript는 프론트 엔드 개발 라이브러리 및 프레임 워크에서 더 좋습니다. 3) 둘 다 풍부한 학습 리소스를 가지고 있지만 Python은 공식 문서로 시작하는 데 적합하지만 JavaScript는 MDNWebDocs에서 더 좋습니다. 선택은 프로젝트 요구와 개인적인 이익을 기반으로해야합니다.
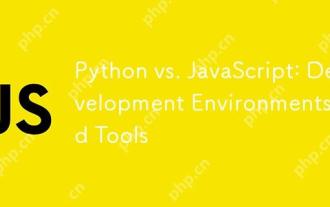
개발 환경에서 Python과 JavaScript의 선택이 모두 중요합니다. 1) Python의 개발 환경에는 Pycharm, Jupyternotebook 및 Anaconda가 포함되어 있으며 데이터 과학 및 빠른 프로토 타이핑에 적합합니다. 2) JavaScript의 개발 환경에는 Node.js, VScode 및 Webpack이 포함되어 있으며 프론트 엔드 및 백엔드 개발에 적합합니다. 프로젝트 요구에 따라 올바른 도구를 선택하면 개발 효율성과 프로젝트 성공률이 향상 될 수 있습니다.
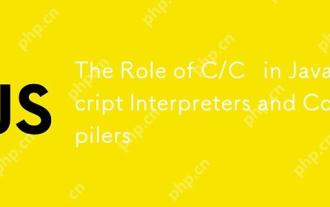
C와 C는 주로 통역사와 JIT 컴파일러를 구현하는 데 사용되는 JavaScript 엔진에서 중요한 역할을합니다. 1) C는 JavaScript 소스 코드를 구문 분석하고 추상 구문 트리를 생성하는 데 사용됩니다. 2) C는 바이트 코드 생성 및 실행을 담당합니다. 3) C는 JIT 컴파일러를 구현하고 런타임에 핫스팟 코드를 최적화하고 컴파일하며 JavaScript의 실행 효율을 크게 향상시킵니다.
