Go를 사용하여 OTP 기반 인증 서버 구축: 1부
시작하기
프로젝트에 대한 새 폴더를 생성하고 다음 명령을 사용하여 Go 모듈을 초기화하는 것으로 시작하세요.
go mod init github.com/vishaaxl/cheershare
프로젝트 구조 설정
다음 폴더 구조로 새 Go 프로젝트를 설정하는 것으로 시작하세요.
my-otp-auth-server/ ├── cmd/ │ └── api/ │ └── main.go │ └── user.go │ └── token.go ├── internal/ │ └── data/ │ ├── models.go │ └── user.go │ └── token.go ├── docker-compose.yml ├── go.mod └── Makefile
다음으로 docker-compose.yml 파일을 설정합니다. 이 구성은 이 튜토리얼 전체에서 작업하게 될 서비스(PostgreSQL 및 Redis)를 정의합니다.
Docker Compose를 사용하여 서비스 설정
프로젝트에 필요한 서비스를 구성하는 것부터 시작하겠습니다. 백엔드에는 다음이 필요합니다.
Redis: redis:6 이미지를 사용하겠습니다. 이 서비스는 보안 액세스를 위해 비밀번호를 구성하고, 포트 6379를 노출하며 --requirepass 플래그를 사용하여 비밀번호 인증을 시행하여 Redis 액세스를 보호합니다.
PostgreSQL: postgres:13 이미지를 사용하겠습니다. 이 서비스는 기본 사용자, 비밀번호 및 데이터베이스를 정의하고 통신을 위해 포트 5432를 노출하며 명명된 볼륨(postgres_data)으로 데이터를 유지하여 내구성을 보장합니다.
선택사항:
- 기본 백엔드 서비스: 여기서도 PostgreSQL 및 Redis와 상호 작용하는 기본 백엔드 서비스를 정의할 수 있습니다.
// docker-compose.yml services: postgres: image: postgres:13 container_name: postgres environment: POSTGRES_USER: postgres POSTGRES_PASSWORD: mysecretpassword POSTGRES_DB: cheershare ports: - "5432:5432" volumes: - postgres_data:/var/lib/postgresql/data redis: image: redis:6 container_name: redis environment: REDIS_PASSWORD: mysecretpassword ports: - "6379:6379" command: ["redis-server", "--requirepass", "mysecretpassword"] volumes: postgres_data:
주요 백엔드 서비스
HTTP 요청을 라우팅하고 처리하기 위해 github.com/julienschmidt/httprouter 패키지를 사용합니다. 종속성을 설치하려면 다음 명령을 실행하십시오.
go get github.com/julienschmidt/httprouter
다음으로 cmd/api/main.go에 파일을 생성하고 다음 코드를 붙여넣습니다. 각 줄에 대한 설명은 댓글에 나와 있습니다:
// main.go package main import ( "fmt" "log" "net/http" "os" "time" "github.com/julienschmidt/httprouter" ) /* config struct: - Holds application-wide configuration settings such as: - `port`: The port number on which the server will listen. - `env`: The current environment (e.g., "development", "production"). */ type config struct { port int env string } /* applications struct: - Encapsulates the application's dependencies, including: - `config`: The application's configuration settings. - `logger`: A logger instance to handle log messages. */ type applications struct { config config logger *log.Logger } func main() { cfg := &config{ port: 4000, env: "development", } logger := log.New(os.Stdout, "INFO\t", log.Ldate|log.Ltime) app := &applications{ config: *cfg, logger: logger, } router := httprouter.New() router.GET("/", func(w http.ResponseWriter, r *http.Request, _ httprouter.Params) { w.WriteHeader(http.StatusOK) fmt.Fprintln(w, "Welcome to the Go application!") }) /* Initialize the HTTP server - Set the server's address to listen on the specified port. - Assign the router as the handler. - Configure timeouts for idle, read, and write operations. - Set up an error logger to capture server errors. */ srv := &http.Server{ Addr: fmt.Sprintf(":%d", app.config.port), Handler: router, IdleTimeout: time.Minute, ReadTimeout: 10 * time.Second, WriteTimeout: 30 * time.Second, } app.logger.Printf("Starting server on port %d in %s mode", app.config.port, app.config.env) err := srv.ListenAndServe() if err != nil { app.logger.Fatalf("Could not start server: %s", err) } }
지금은 go run ./cmd/api를 사용하여 서버를 시작하고 http://localhost:4000에 요청을 보내 환영 메시지를 반환함으로써 설정을 테스트할 수 있습니다. 다음으로 핵심 기능을 구현하기 위한 세 가지 추가 경로를 정의하겠습니다.
/send-otp: 이 경로는 사용자에게 OTP 전송을 처리합니다. 고유한 OTP를 생성하여 Redis에 저장한 후 사용자에게 전달합니다.
/verify-otp: 이 경로는 사용자가 제공한 OTP를 확인합니다. 사용자의 신원을 확인하기 위해 Redis에 저장된 값을 확인합니다.
/login: 이 경로는 OTP가 확인되고 사용자가 성공적으로 생성되면 사용자 로그인 기능을 처리합니다.
하지만 계속하기 전에 앞서 docker-compose.yml 파일에서 정의한 서비스에 연결하는 데 필요한 전화번호 및 일회용 비밀번호와 같은 사용자 정보를 저장할 방법이 필요합니다.
도우미 기능 정의
경로를 구현하기 전에 두 가지 필수 도우미 기능을 정의해 보겠습니다. 이러한 기능은 Redis 및 PostgreSQL 서버에 대한 연결을 처리하여 백엔드가 이러한 서비스와 상호 작용할 수 있도록 보장합니다.
서비스에 대한 정보를 저장하려면 'config' 구조체를 수정하세요. 이러한 기능은 설명이 필요하지 않습니다.
my-otp-auth-server/ ├── cmd/ │ └── api/ │ └── main.go │ └── user.go │ └── token.go ├── internal/ │ └── data/ │ ├── models.go │ └── user.go │ └── token.go ├── docker-compose.yml ├── go.mod └── Makefile
docker-compose up -d 명령으로 서비스를 시작한 후 이 기능을 사용하여 PostgreSQL 데이터베이스 및 Redis 서버에 대한 연결을 설정할 수 있습니다.
다음 부분에서는 앞서 이야기한 경로에 대한 작업을 시작하겠습니다. 이것이 이제 main.go 파일의 모습입니다.
// docker-compose.yml services: postgres: image: postgres:13 container_name: postgres environment: POSTGRES_USER: postgres POSTGRES_PASSWORD: mysecretpassword POSTGRES_DB: cheershare ports: - "5432:5432" volumes: - postgres_data:/var/lib/postgresql/data redis: image: redis:6 container_name: redis environment: REDIS_PASSWORD: mysecretpassword ports: - "6379:6379" command: ["redis-server", "--requirepass", "mysecretpassword"] volumes: postgres_data:
위 내용은 Go를 사용하여 OTP 기반 인증 서버 구축: 1부의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
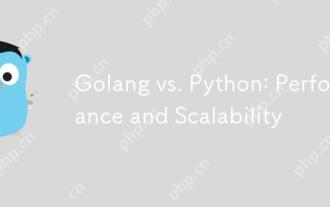
Golang은 성능과 확장 성 측면에서 Python보다 낫습니다. 1) Golang의 컴파일 유형 특성과 효율적인 동시성 모델은 높은 동시성 시나리오에서 잘 수행합니다. 2) 해석 된 언어로서 파이썬은 천천히 실행되지만 Cython과 같은 도구를 통해 성능을 최적화 할 수 있습니다.
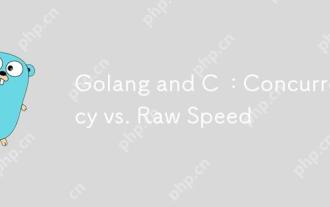
Golang은 동시성에서 C보다 낫고 C는 원시 속도에서 Golang보다 낫습니다. 1) Golang은 Goroutine 및 Channel을 통해 효율적인 동시성을 달성하며, 이는 많은 동시 작업을 처리하는 데 적합합니다. 2) C 컴파일러 최적화 및 표준 라이브러리를 통해 하드웨어에 가까운 고성능을 제공하며 극도의 최적화가 필요한 애플리케이션에 적합합니다.
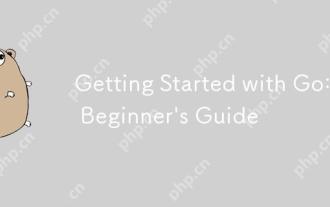
goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity, 효율성, 및 콘크리 론 피처
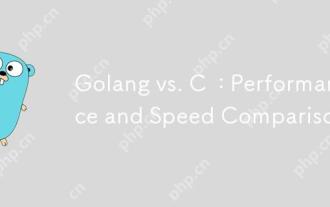
Golang은 빠른 개발 및 동시 시나리오에 적합하며 C는 극도의 성능 및 저수준 제어가 필요한 시나리오에 적합합니다. 1) Golang은 쓰레기 수집 및 동시성 메커니즘을 통해 성능을 향상시키고, 고전성 웹 서비스 개발에 적합합니다. 2) C는 수동 메모리 관리 및 컴파일러 최적화를 통해 궁극적 인 성능을 달성하며 임베디드 시스템 개발에 적합합니다.
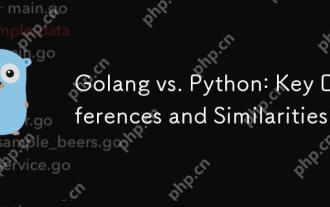
Golang과 Python은 각각 고유 한 장점이 있습니다. Golang은 고성능 및 동시 프로그래밍에 적합하지만 Python은 데이터 과학 및 웹 개발에 적합합니다. Golang은 동시성 모델과 효율적인 성능으로 유명하며 Python은 간결한 구문 및 풍부한 라이브러리 생태계로 유명합니다.
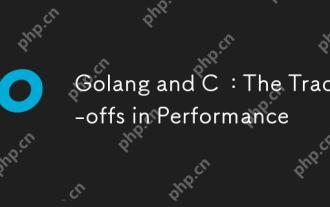
Golang과 C의 성능 차이는 주로 메모리 관리, 컴파일 최적화 및 런타임 효율에 반영됩니다. 1) Golang의 쓰레기 수집 메커니즘은 편리하지만 성능에 영향을 줄 수 있습니다. 2) C의 수동 메모리 관리 및 컴파일러 최적화는 재귀 컴퓨팅에서 더 효율적입니다.
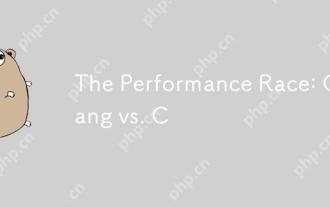
Golang과 C는 각각 공연 경쟁에서 고유 한 장점을 가지고 있습니다. 1) Golang은 높은 동시성과 빠른 발전에 적합하며 2) C는 더 높은 성능과 세밀한 제어를 제공합니다. 선택은 프로젝트 요구 사항 및 팀 기술 스택을 기반으로해야합니다.
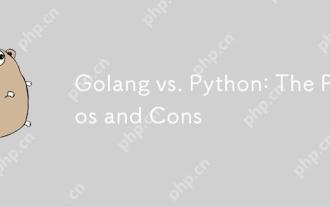
golangisidealforbuildingscalablesystemsdueToitsefficiencyandconcurrency
