4에서 알아야 할 무료 API
API(애플리케이션 프로그래밍 인터페이스)는 개발자가 타사 서비스를 애플리케이션에 통합할 수 있도록 해주는 필수 도구입니다. 다음은 다양한 카테고리에 걸쳐 2024년에 사용할 수 있는 무료 API의 광범위한 목록과 각 API에 대한 웹사이트 링크, 설명, 샘플 코드입니다.
게임 API
Steam 커뮤니티 API
웹사이트: steamcommunity.com/dev
설명: Steamworks Web API는 사용자 인증, 인벤토리 관리, 게임 데이터 등 다양한 Steam 기능에 대한 인터페이스를 제공합니다.
샘플 코드
const fetch = require('node-fetch'); const steamApiKey = 'YOUR_STEAM_API_KEY'; const steamId = 'STEAM_USER_ID'; const url = `http://api.steampowered.com/ISteamUser/GetPlayerSummaries/v0002/?key=${steamApiKey}&steamids=${steamId}`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
라이엇 게임즈 API
웹사이트:developer.riotgames.com
설명: League of Legends, Teamfight Tactics, Valorant 등과 같은 게임의 데이터에 액세스합니다. 경기, 랭킹, 챔피언 등 게임 관련 통계자료를 제공합니다.
샘플 코드
const fetch = require('node-fetch'); const riotApiKey = 'YOUR_RIOT_API_KEY'; const summonerName = 'SUMMONER_NAME'; const url = `https://na1.api.riotgames.com/lol/summoner/v4/summoners/by-name/${summonerName}?api_key=${riotApiKey}`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
언어 API
사악한 모욕 생성기 API
웹사이트: evilinsult.com/api
설명: 재미나 테스트 목적으로 다양한 언어로 무작위 모욕을 생성합니다.
샘플 코드
const fetch = require('node-fetch'); const url = 'https://evilinsult.com/generate_insult.php?lang=en&type=json'; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
재미있는 번역 API
웹사이트: funtranslations.com/api
설명: 텍스트를 Yoda, Shakespeare, Minion talk 등과 같은 다양하고 재미있는 언어로 번역하세요.
샘플 코드
const fetch = require('node-fetch'); const text = 'Hello, world!'; const url = `https://api.funtranslations.com/translate/yoda.json?text=${encodeURIComponent(text)}`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
음악 API
스포티파이 웹 API
웹사이트: 개발자.spotify.com/documentation/web-api
설명: 앨범, 아티스트, 재생목록, 사용자 데이터 등의 음악 데이터에 액세스합니다. Spotify 재생 등을 제어하세요.
샘플 코드
const fetch = require('node-fetch'); const accessToken = 'YOUR_SPOTIFY_ACCESS_TOKEN'; const url = 'https://api.spotify.com/v1/me/player/recently-played'; fetch(url, { headers: { 'Authorization': `Bearer ${accessToken}` } }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
보안 API
나는 API를 받았습니까?
웹사이트: haveibeenpwned.com/API/v2
설명: 귀하의 이메일이나 사용자 이름이 데이터 유출의 일부인지 확인하세요. 위반, 붙여넣기, 비밀번호 노출에 대한 데이터를 제공합니다.
샘플 코드
const fetch = require('node-fetch'); const email = 'test@example.com'; const url = `https://haveibeenpwned.com/api/v2/breachedaccount/${email}`; fetch(url, { headers: { 'User-Agent': 'Node.js' } }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
쇼단 API
웹사이트:developer.shodan.io
설명: Shodan은 인터넷에 연결된 장치를 위한 검색 엔진입니다. 전 세계의 다양한 서버, 디바이스, 시스템에 대한 데이터를 제공합니다.
샘플 코드
const fetch = require('node-fetch'); const steamApiKey = 'YOUR_STEAM_API_KEY'; const steamId = 'STEAM_USER_ID'; const url = `http://api.steampowered.com/ISteamUser/GetPlayerSummaries/v0002/?key=${steamApiKey}&steamids=${steamId}`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
과학 및 수학 API
NASA API
웹사이트: api.nasa.gov
설명: 천문학 사진, 행성 데이터 등을 포함한 NASA 데이터 세트의 데이터에 액세스하세요.
샘플 코드
const fetch = require('node-fetch'); const riotApiKey = 'YOUR_RIOT_API_KEY'; const summonerName = 'SUMMONER_NAME'; const url = `https://na1.api.riotgames.com/lol/summoner/v4/summoners/by-name/${summonerName}?api_key=${riotApiKey}`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
울프램 알파 API
웹사이트: products.wolframalpha.com/api
설명: 수학 계산, 데이터 분석 등을 포함한 Wolfram Alpha의 방대한 계산 지식에 대한 액세스를 제공합니다.
샘플 코드
const fetch = require('node-fetch'); const url = 'https://evilinsult.com/generate_insult.php?lang=en&type=json'; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
개방형 과학 프레임워크 API
웹사이트:developer.osf.io
설명: Open Science Framework에서 연구 데이터, 프로젝트 관리 도구 및 기타 과학 리소스에 액세스하세요.
샘플 코드
const fetch = require('node-fetch'); const text = 'Hello, world!'; const url = `https://api.funtranslations.com/translate/yoda.json?text=${encodeURIComponent(text)}`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
스포츠 API
NBA API
웹사이트: any-api.com/nba_com/nba_com/docs/API_Description
설명: NBA 팀, 선수 및 게임에 대한 데이터에 액세스하세요.
샘플 코드
const fetch = require('node-fetch'); const accessToken = 'YOUR_SPOTIFY_ACCESS_TOKEN'; const url = 'https://api.spotify.com/v1/me/player/recently-played'; fetch(url, { headers: { 'Authorization': `Bearer ${accessToken}` } }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
웹 앱 API
디스코드 API
웹사이트: discord.com/developers/docs/intro
설명: 애플리케이션을 Discord와 통합하여 사용자 인증, 메시징 등을 허용합니다.
샘플 코드
const fetch = require('node-fetch'); const email = 'test@example.com'; const url = `https://haveibeenpwned.com/api/v2/breachedaccount/${email}`; fetch(url, { headers: { 'User-Agent': 'Node.js' } }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
슬랙 API
웹사이트: api.slack.com
설명: 메시징, 사용자 데이터, 작업 공간 관리 등 Slack 기능에 액세스합니다.
샘플 코드
const fetch = require('node-fetch'); const shodanApiKey = 'YOUR_SHODAN_API_KEY'; const query = 'apache'; const url = `https://api.shodan.io/shodan/host/search?key=${shodanApiKey}&query=${query}`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
제품 및 사물 API
자동차 쿼리 API
웹사이트: carqueryapi.com
설명:
을 포함한 자동차 데이터에 액세스합니다.
제조업체, 모델, 연식 정보
샘플 코드
const fetch = require('node-fetch'); const nasaApiKey = 'YOUR_NASA_API_KEY'; const url = `https://api.nasa.gov/planetary/apod?api_key=${nasaApiKey}`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
옐프 API
웹사이트: yelp.com/developers
설명: 리뷰, 평점, 비즈니스 세부정보 등 지역 비즈니스에 대한 데이터에 액세스하세요.
샘플 코드
const fetch = require('node-fetch'); const wolframAppId = 'YOUR_WOLFRAM_APP_ID'; const query = 'integrate x^2'; const url = `http://api.wolframalpha.com/v2/query?input=${encodeURIComponent(query)}&appid=${wolframAppId}&output=json`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
건강 API
Healthcare.gov API
웹사이트: Healthcare.gov/developers
설명: 의료 계획, 서비스 제공자 디렉토리 및 기타 건강 관련 정보에 대한 데이터에 액세스하세요.
샘플 코드
const fetch = require('node-fetch'); const steamApiKey = 'YOUR_STEAM_API_KEY'; const steamId = 'STEAM_USER_ID'; const url = `http://api.steampowered.com/ISteamUser/GetPlayerSummaries/v0002/?key=${steamApiKey}&steamids=${steamId}`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
정부 및 지리 API
Code.gov API
웹사이트: code.gov
설명: 코드 저장소 및 프로젝트 세부 정보를 포함하여 연방 정부 소프트웨어 프로젝트에 대한 데이터에 액세스합니다.
샘플 코드
const fetch = require('node-fetch'); const riotApiKey = 'YOUR_RIOT_API_KEY'; const summonerName = 'SUMMONER_NAME'; const url = `https://na1.api.riotgames.com/lol/summoner/v4/summoners/by-name/${summonerName}?api_key=${riotApiKey}`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
Data.gov API
웹사이트: data.gov/developers/apis
설명: 날씨, 교육, 건강 데이터를 포함하여 미국 정부의 광범위한 데이터 세트에 액세스하세요.
샘플 코드
const fetch = require('node-fetch'); const url = 'https://evilinsult.com/generate_insult.php?lang=en&type=json'; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
Data.europa.eu API
웹사이트: data.europa.eu/en
설명: 유럽 연합 기관 및 기관의 공개 데이터에 액세스하세요.
샘플 코드
const fetch = require('node-fetch'); const text = 'Hello, world!'; const url = `https://api.funtranslations.com/translate/yoda.json?text=${encodeURIComponent(text)}`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
트랜스락 API
웹사이트: rapidapi.com/transloc/api/openapi-1-2/details
설명: 도착 예측, 차량 위치 등을 포함한 실시간 대중교통 데이터에 액세스하세요.
샘플 코드
const fetch = require('node-fetch'); const accessToken = 'YOUR_SPOTIFY_ACCESS_TOKEN'; const url = 'https://api.spotify.com/v1/me/player/recently-played'; fetch(url, { headers: { 'Authorization': `Bearer ${accessToken}` } }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
식품 API
개방형 식품 정보 API
웹사이트: world.openfoodfacts.org/data
설명: 성분, 영양 정보, 알레르기 유발 물질 정보 등 전 세계 식품에 대한 데이터에 액세스하세요.
샘플 코드
const fetch = require('node-fetch'); const email = 'test@example.com'; const url = `https://haveibeenpwned.com/api/v2/breachedaccount/${email}`; fetch(url, { headers: { 'User-Agent': 'Node.js' } }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
타코 팬시 API
웹사이트: github.com/evz/tacofancy-api
설명: 재료, 준비 방법 등 타코 레시피에 대한 데이터에 액세스하세요.
샘플 코드
const fetch = require('node-fetch'); const shodanApiKey = 'YOUR_SHODAN_API_KEY'; const query = 'apache'; const url = `https://api.shodan.io/shodan/host/search?key=${shodanApiKey}&query=${query}`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
오픈 소스 프로젝트 API
Libraries.io API
웹사이트: library.io/api
설명: 종속성 정보, 버전 기록 등을 포함한 오픈 소스 프로젝트의 데이터에 액세스하세요.
샘플 코드
const fetch = require('node-fetch'); const nasaApiKey = 'YOUR_NASA_API_KEY'; const url = `https://api.nasa.gov/planetary/apod?api_key=${nasaApiKey}`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
영화 및 만화 API
척 노리스 농담 API
웹사이트: api.chucknorris.io
설명: Chuck Norris 농담 컬렉션에 액세스하세요.
샘플 코드
const fetch = require('node-fetch'); const wolframAppId = 'YOUR_WOLFRAM_APP_ID'; const query = 'integrate x^2'; const url = `http://api.wolframalpha.com/v2/query?input=${encodeURIComponent(query)}&appid=${wolframAppId}&output=json`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
최종 공간 API
웹사이트: finalspaceapi.com
설명: 캐릭터, 에피소드 등을 포함한 Final Space TV 쇼의 데이터에 액세스하세요.
샘플 코드
const fetch = require('node-fetch'); const steamApiKey = 'YOUR_STEAM_API_KEY'; const steamId = 'STEAM_USER_ID'; const url = `http://api.steampowered.com/ISteamUser/GetPlayerSummaries/v0002/?key=${steamApiKey}&steamids=${steamId}`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
키츠 API
웹사이트: kitsu.docs.apiary.io
설명: 시리즈 정보, 리뷰, 사용자 평점을 포함한 애니메이션 및 만화 관련 데이터에 액세스하세요.
샘플 코드
const fetch = require('node-fetch'); const riotApiKey = 'YOUR_RIOT_API_KEY'; const summonerName = 'SUMMONER_NAME'; const url = `https://na1.api.riotgames.com/lol/summoner/v4/summoners/by-name/${summonerName}?api_key=${riotApiKey}`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
마블 API
웹사이트:developer.marvel.com
설명: 마블 만화, 캐릭터, 창작자에 대한 데이터에 접근하세요.
샘플 코드
const fetch = require('node-fetch'); const url = 'https://evilinsult.com/generate_insult.php?lang=en&type=json'; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
포케API
웹사이트: pokeapi.co
설명: 포켓몬의 종, 능력, 게임 정보를 포함한 데이터에 액세스합니다.
샘플 코드
const fetch = require('node-fetch'); const text = 'Hello, world!'; const url = `https://api.funtranslations.com/translate/yoda.json?text=${encodeURIComponent(text)}`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
릭 앤 모티 API
웹사이트: rickandmortyapi.com
설명: 캐릭터, 에피소드, 장소 등 Rick and Morty TV 프로그램의 데이터에 액세스하세요.
샘플 코드
const fetch = require('node-fetch'); const accessToken = 'YOUR_SPOTIFY_ACCESS_TOKEN'; const url = 'https://api.spotify.com/v1/me/player/recently-played'; fetch(url, { headers: { 'Authorization': `Bearer ${accessToken}` } }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
심슨 인용 API
웹사이트: thesimpsonsquoteapi.glitch.me
설명: The Simpsons TV 쇼의 인용문 컬렉션에 액세스하세요.
견본
코드
const fetch = require('node-fetch'); const email = 'test@example.com'; const url = `https://haveibeenpwned.com/api/v2/breachedaccount/${email}`; fetch(url, { headers: { 'User-Agent': 'Node.js' } }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
스타워즈 API
웹사이트: swapi.tech
설명: 영화, 캐릭터, 우주선, 행성 등 스타워즈 세계관에 대한 데이터에 액세스하세요.
샘플 코드
const fetch = require('node-fetch'); const shodanApiKey = 'YOUR_SHODAN_API_KEY'; const query = 'apache'; const url = `https://api.shodan.io/shodan/host/search?key=${shodanApiKey}&query=${query}`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
슈퍼히어로 API
웹사이트: Superheroapi.com
설명: 능력, 약력, 이미지 등 다양한 슈퍼히어로에 대한 데이터에 액세스하세요.
샘플 코드
const fetch = require('node-fetch'); const nasaApiKey = 'YOUR_NASA_API_KEY'; const url = `https://api.nasa.gov/planetary/apod?api_key=${nasaApiKey}`; fetch(url) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
결론
2024년 무료 API의 이 포괄적인 목록은 광범위한 카테고리를 포괄하며 개발자에게 강력하고 다양한 기능으로 애플리케이션을 향상할 수 있는 수많은 기회를 제공합니다. 게임, 음악부터 과학, 정부 데이터에 이르기까지 이러한 API는 혁신적이고 매력적인 프로젝트를 만드는 데 유용한 리소스를 제공합니다.
이러한 API를 자유롭게 탐색하고 프로젝트에 통합하여 새로운 가능성과 기능을 활용해 보세요. 즐거운 코딩하세요!
? 저희와 계속 연락하세요!
우리는 혁신이 번창하고 기술 애호가가 함께 성장하는 커뮤니티를 구축하고 있습니다. 영감을 주고, 배우고, 창조하는 우리의 여정에 동참하세요!
? 자세히 살펴보기:
- Discord: 기술 매니아와 소통하세요
- WhatsApp: 실시간 업데이트 받기
- 텔레그램: 일일 인사이트 및 팁
? 매일 영감을 얻으려면 팔로우하세요:
- 인스타그램: @thecampuscoders
- LinkedIn: @thecampuscoders
- 페이스북: @thecampuscoders
? 언제든지 방문해주세요!
? thecampuscoders.com
? 기술 여행에 활력을 불어넣는 리소스, 튜토리얼, 업데이트를 살펴보세요!
✨ 함께 협력하고, 배우고, 미래를 만들어 갑시다!
아이디어나 제안이 있으신가요? 우리에게 연락하여 특별한 일에 참여해 보세요!
? 문의: deepak@thecampuscoders.com
위 내용은 4에서 알아야 할 무료 API의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
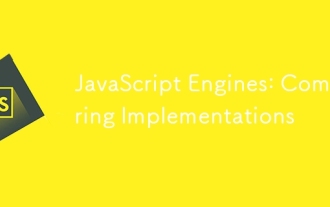
각각의 엔진의 구현 원리 및 최적화 전략이 다르기 때문에 JavaScript 엔진은 JavaScript 코드를 구문 분석하고 실행할 때 다른 영향을 미칩니다. 1. 어휘 분석 : 소스 코드를 어휘 단위로 변환합니다. 2. 문법 분석 : 추상 구문 트리를 생성합니다. 3. 최적화 및 컴파일 : JIT 컴파일러를 통해 기계 코드를 생성합니다. 4. 실행 : 기계 코드를 실행하십시오. V8 엔진은 즉각적인 컴파일 및 숨겨진 클래스를 통해 최적화하여 Spidermonkey는 유형 추론 시스템을 사용하여 동일한 코드에서 성능이 다른 성능을 제공합니다.
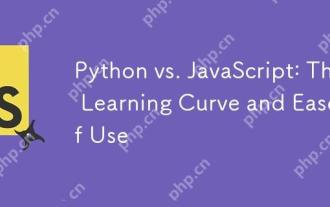
Python은 부드러운 학습 곡선과 간결한 구문으로 초보자에게 더 적합합니다. JavaScript는 가파른 학습 곡선과 유연한 구문으로 프론트 엔드 개발에 적합합니다. 1. Python Syntax는 직관적이며 데이터 과학 및 백엔드 개발에 적합합니다. 2. JavaScript는 유연하며 프론트 엔드 및 서버 측 프로그래밍에서 널리 사용됩니다.
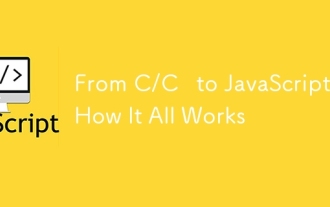
C/C에서 JavaScript로 전환하려면 동적 타이핑, 쓰레기 수집 및 비동기 프로그래밍으로 적응해야합니다. 1) C/C는 수동 메모리 관리가 필요한 정적으로 입력 한 언어이며 JavaScript는 동적으로 입력하고 쓰레기 수집이 자동으로 처리됩니다. 2) C/C를 기계 코드로 컴파일 해야하는 반면 JavaScript는 해석 된 언어입니다. 3) JavaScript는 폐쇄, 프로토 타입 체인 및 약속과 같은 개념을 소개하여 유연성과 비동기 프로그래밍 기능을 향상시킵니다.
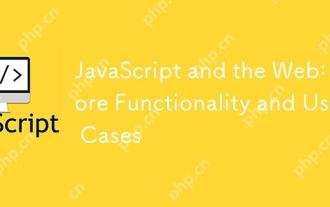
웹 개발에서 JavaScript의 주요 용도에는 클라이언트 상호 작용, 양식 검증 및 비동기 통신이 포함됩니다. 1) DOM 운영을 통한 동적 컨텐츠 업데이트 및 사용자 상호 작용; 2) 사용자가 사용자 경험을 향상시키기 위해 데이터를 제출하기 전에 클라이언트 확인이 수행됩니다. 3) 서버와의 진실한 통신은 Ajax 기술을 통해 달성됩니다.
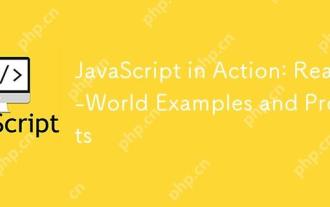
실제 세계에서 JavaScript의 응용 프로그램에는 프론트 엔드 및 백엔드 개발이 포함됩니다. 1) DOM 운영 및 이벤트 처리와 관련된 TODO 목록 응용 프로그램을 구축하여 프론트 엔드 애플리케이션을 표시합니다. 2) Node.js를 통해 RESTFULAPI를 구축하고 Express를 통해 백엔드 응용 프로그램을 시연하십시오.
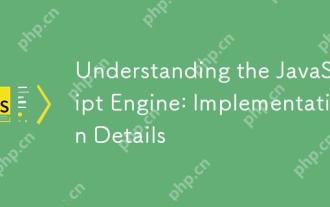
보다 효율적인 코드를 작성하고 성능 병목 현상 및 최적화 전략을 이해하는 데 도움이되기 때문에 JavaScript 엔진이 내부적으로 작동하는 방식을 이해하는 것은 개발자에게 중요합니다. 1) 엔진의 워크 플로에는 구문 분석, 컴파일 및 실행; 2) 실행 프로세스 중에 엔진은 인라인 캐시 및 숨겨진 클래스와 같은 동적 최적화를 수행합니다. 3) 모범 사례에는 글로벌 변수를 피하고 루프 최적화, Const 및 Lets 사용 및 과도한 폐쇄 사용을 피하는 것이 포함됩니다.
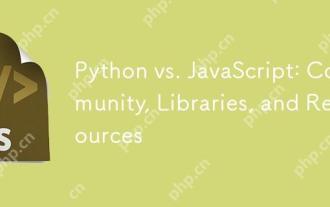
Python과 JavaScript는 커뮤니티, 라이브러리 및 리소스 측면에서 고유 한 장점과 단점이 있습니다. 1) Python 커뮤니티는 친절하고 초보자에게 적합하지만 프론트 엔드 개발 리소스는 JavaScript만큼 풍부하지 않습니다. 2) Python은 데이터 과학 및 기계 학습 라이브러리에서 강력하며 JavaScript는 프론트 엔드 개발 라이브러리 및 프레임 워크에서 더 좋습니다. 3) 둘 다 풍부한 학습 리소스를 가지고 있지만 Python은 공식 문서로 시작하는 데 적합하지만 JavaScript는 MDNWebDocs에서 더 좋습니다. 선택은 프로젝트 요구와 개인적인 이익을 기반으로해야합니다.
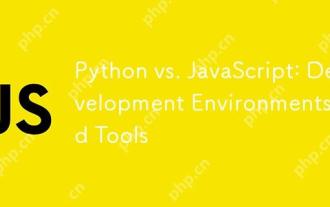
개발 환경에서 Python과 JavaScript의 선택이 모두 중요합니다. 1) Python의 개발 환경에는 Pycharm, Jupyternotebook 및 Anaconda가 포함되어 있으며 데이터 과학 및 빠른 프로토 타이핑에 적합합니다. 2) JavaScript의 개발 환경에는 Node.js, VScode 및 Webpack이 포함되어 있으며 프론트 엔드 및 백엔드 개발에 적합합니다. 프로젝트 요구에 따라 올바른 도구를 선택하면 개발 효율성과 프로젝트 성공률이 향상 될 수 있습니다.
