JavaScript의 this가 다른 OOP 언어와 다른 이유
JavaScript의 this
키워드는 특히 self
가 현재 개체 인스턴스를 일관되게 참조하는 C#, Java 또는 Python과 같은 언어를 사용하는 개발자에게 혼란을 야기하는 경우가 많습니다. 이러한 언어와 달리 JavaScript의 this
은 동적이며 해당 값은 함수의 호출 컨텍스트에 따라 결정됩니다. 이 가이드에는 this
의 행동에 영향을 미치는 다양한 시나리오가 요약되어 있습니다.
1. 글로벌 범위:
-
비엄격 모드:
this
는 전역 개체(브라우저에서는window
, Node.js에서는global
)를 가리킵니다.
console.log(this); // window or global
- 엄격 모드:
this
는undefined
입니다.
"use strict"; console.log(this); // undefined
2. 내부 기능:
- 정규 함수: 비엄격 모드에서
this
는 전역 객체를 참조합니다. 엄격 모드에서는undefined
입니다.
function myFunc() { console.log(this); } myFunc(); // window (non-strict), undefined (strict)
3. 객체 메소드:
- 객체 메소드로 함수를 호출할 때
this
는 해당 객체를 참조합니다.
const myObj = { name: "JavaScript", greet() { console.log(this.name); // this refers to myObj } }; myObj.greet(); // Output: JavaScript
4. 화살표 기능:
- 화살표 함수에는 자체
this
가 부족합니다. 어휘 범위(주변 컨텍스트)에서this
을 상속합니다.
const myObj = { name: "JavaScript", arrowFunc: () => { console.log(this.name); // Inherits this from the global scope } }; myObj.arrowFunc(); // undefined (in browsers, this is window)
5. 생성자:
- 생성자 함수 또는 클래스 내에서
this
는 새로 생성된 인스턴스를 나타냅니다.
class Person { constructor(name) { this.name = name; } greet() { console.log(`Hello, ${this.name}`); } } const person = new Person("Alice"); person.greet(); // Output: Hello, Alice
6. 명시적 바인딩(call
, apply
, bind
):
JavaScript 함수는 call
을 명시적으로 설정하기 위한 메서드(apply
, bind
, this
)가 있는 개체입니다.
call
및apply
는 지정된this
값을 사용하여 함수를 호출합니다.call
쉼표로 구분된 인수를 사용합니다.apply
은 배열을 사용합니다.
function greet(greeting) { console.log(`${greeting}, ${this.name}`); } const user = { name: "Alice" }; greet.call(user, "Hello"); // Output: Hello, Alice greet.apply(user, ["Hi"]); // Output: Hi, Alice
bind
은this
이 영구적으로 바인딩된 새 함수를 반환합니다.
const boundGreet = greet.bind(user); boundGreet("Hello"); // Output: Hello, Alice
7. 이벤트 리스너:
- 일반 함수:
this
는 이벤트를 트리거하는 요소를 나타냅니다.
const btn = document.querySelector("button"); btn.addEventListener("click", function() { console.log(this); // The button element });
- 화살표 함수:
this
는 요소가 아닌 주변 범위에서 상속됩니다.
btn.addEventListener("click", () => { console.log(this); // this depends on the arrow function's definition context });
8. setTimeout
/ setInterval
:
- 일반 함수:
this
기본값은 전역 개체입니다.
setTimeout(function() { console.log(this); // window in browsers }, 1000);
- 화살표 기능:
this
은 어휘적으로 상속됩니다.
setTimeout(() => { console.log(this); // Inherits this from surrounding context }, 1000);
9. 수업:
- 클래스 메소드 내에서
this
는 클래스 인스턴스를 의미합니다.
console.log(this); // window or global
10. 컨텍스트 손실(메소드 추출):
메서드를 변수에 할당하거나 이를 콜백으로 전달하면 this
바인딩 손실이 발생할 수 있습니다.
"use strict"; console.log(this); // undefined
해결책: .bind(obj)
또는 화살표 기능을 사용하여 상황을 유지하세요.
11. new
키워드:
함수와 함께 new
을 사용하면 새로운 객체가 생성되고 this
는 해당 객체를 참조합니다.
function myFunc() { console.log(this); } myFunc(); // window (non-strict), undefined (strict)
요약 표:
Context |
this Refers To |
---|---|
Global (non-strict) | Global object (window/global) |
Global (strict) | undefined |
Object Method | The object owning the method |
Arrow Function | Lexical scope (surrounding context) |
Constructor/Class | The instance being created |
call , apply , bind
|
Explicitly defined value |
Event Listener | The element triggering the event |
setTimeout /setInterval
|
Global object (regular function), lexical scope (arrow function) |
new Keyword |
The newly created object |
정의되지 않음
호출
, 적용
, 바인딩
setTimeout
/setInterval
키워드
this
올바르고 예측 가능한 JavaScript 코드를 작성하려면 이러한 시나리오를 이해하는 것이 중요합니다. 예상치 못한 동작을 방지하려면 필요한 경우 명시적 바인딩과 같은 기술을 활용하는 것을 잊지 마세요.위 내용은 JavaScript의 this가 다른 OOP 언어와 다른 이유의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
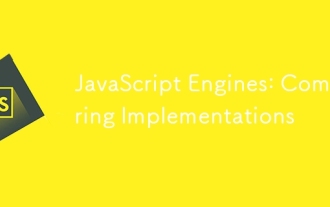
각각의 엔진의 구현 원리 및 최적화 전략이 다르기 때문에 JavaScript 엔진은 JavaScript 코드를 구문 분석하고 실행할 때 다른 영향을 미칩니다. 1. 어휘 분석 : 소스 코드를 어휘 단위로 변환합니다. 2. 문법 분석 : 추상 구문 트리를 생성합니다. 3. 최적화 및 컴파일 : JIT 컴파일러를 통해 기계 코드를 생성합니다. 4. 실행 : 기계 코드를 실행하십시오. V8 엔진은 즉각적인 컴파일 및 숨겨진 클래스를 통해 최적화하여 Spidermonkey는 유형 추론 시스템을 사용하여 동일한 코드에서 성능이 다른 성능을 제공합니다.
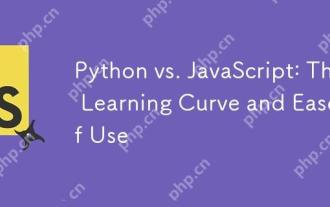
Python은 부드러운 학습 곡선과 간결한 구문으로 초보자에게 더 적합합니다. JavaScript는 가파른 학습 곡선과 유연한 구문으로 프론트 엔드 개발에 적합합니다. 1. Python Syntax는 직관적이며 데이터 과학 및 백엔드 개발에 적합합니다. 2. JavaScript는 유연하며 프론트 엔드 및 서버 측 프로그래밍에서 널리 사용됩니다.
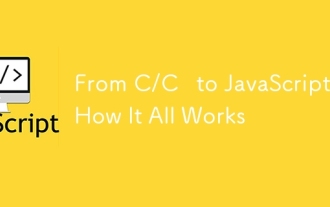
C/C에서 JavaScript로 전환하려면 동적 타이핑, 쓰레기 수집 및 비동기 프로그래밍으로 적응해야합니다. 1) C/C는 수동 메모리 관리가 필요한 정적으로 입력 한 언어이며 JavaScript는 동적으로 입력하고 쓰레기 수집이 자동으로 처리됩니다. 2) C/C를 기계 코드로 컴파일 해야하는 반면 JavaScript는 해석 된 언어입니다. 3) JavaScript는 폐쇄, 프로토 타입 체인 및 약속과 같은 개념을 소개하여 유연성과 비동기 프로그래밍 기능을 향상시킵니다.
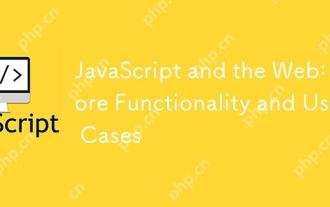
웹 개발에서 JavaScript의 주요 용도에는 클라이언트 상호 작용, 양식 검증 및 비동기 통신이 포함됩니다. 1) DOM 운영을 통한 동적 컨텐츠 업데이트 및 사용자 상호 작용; 2) 사용자가 사용자 경험을 향상시키기 위해 데이터를 제출하기 전에 클라이언트 확인이 수행됩니다. 3) 서버와의 진실한 통신은 Ajax 기술을 통해 달성됩니다.
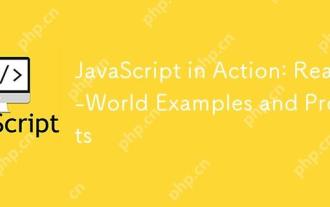
실제 세계에서 JavaScript의 응용 프로그램에는 프론트 엔드 및 백엔드 개발이 포함됩니다. 1) DOM 운영 및 이벤트 처리와 관련된 TODO 목록 응용 프로그램을 구축하여 프론트 엔드 애플리케이션을 표시합니다. 2) Node.js를 통해 RESTFULAPI를 구축하고 Express를 통해 백엔드 응용 프로그램을 시연하십시오.
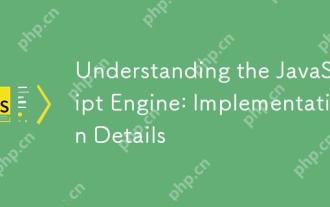
보다 효율적인 코드를 작성하고 성능 병목 현상 및 최적화 전략을 이해하는 데 도움이되기 때문에 JavaScript 엔진이 내부적으로 작동하는 방식을 이해하는 것은 개발자에게 중요합니다. 1) 엔진의 워크 플로에는 구문 분석, 컴파일 및 실행; 2) 실행 프로세스 중에 엔진은 인라인 캐시 및 숨겨진 클래스와 같은 동적 최적화를 수행합니다. 3) 모범 사례에는 글로벌 변수를 피하고 루프 최적화, Const 및 Lets 사용 및 과도한 폐쇄 사용을 피하는 것이 포함됩니다.
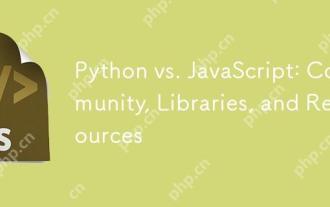
Python과 JavaScript는 커뮤니티, 라이브러리 및 리소스 측면에서 고유 한 장점과 단점이 있습니다. 1) Python 커뮤니티는 친절하고 초보자에게 적합하지만 프론트 엔드 개발 리소스는 JavaScript만큼 풍부하지 않습니다. 2) Python은 데이터 과학 및 기계 학습 라이브러리에서 강력하며 JavaScript는 프론트 엔드 개발 라이브러리 및 프레임 워크에서 더 좋습니다. 3) 둘 다 풍부한 학습 리소스를 가지고 있지만 Python은 공식 문서로 시작하는 데 적합하지만 JavaScript는 MDNWebDocs에서 더 좋습니다. 선택은 프로젝트 요구와 개인적인 이익을 기반으로해야합니다.
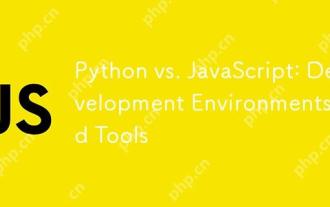
개발 환경에서 Python과 JavaScript의 선택이 모두 중요합니다. 1) Python의 개발 환경에는 Pycharm, Jupyternotebook 및 Anaconda가 포함되어 있으며 데이터 과학 및 빠른 프로토 타이핑에 적합합니다. 2) JavaScript의 개발 환경에는 Node.js, VScode 및 Webpack이 포함되어 있으며 프론트 엔드 및 백엔드 개발에 적합합니다. 프로젝트 요구에 따라 올바른 도구를 선택하면 개발 효율성과 프로젝트 성공률이 향상 될 수 있습니다.
