더 나은 성능을 위해 C#에서 비트맵 조작을 어떻게 최적화할 수 있습니까?
C# 비트맵 처리 성능 향상을 위한 팁
비트맵 픽셀에 직접 액세스하고 수정하는 것은 특히 표준 Bitmap.GetPixel()
및 Bitmap.SetPixel()
방법을 사용할 때 계산 집약적인 작업입니다. 성능을 향상하려면 다음 기술을 고려하십시오.
비트맵을 바이트 배열로 변환한 후 다시 되돌리기
비트맵 픽셀에 대한 빠른 일괄 작업을 위해 비트맵을 바이트 배열로 변환하고 원시 픽셀 데이터를 직접 처리할 수 있습니다. 이는 개별 픽셀 액세스의 오버헤드를 방지하여 런타임을 크게 줄일 수 있습니다.
다음 코드는 비트맵을 바이트 배열로 변환하는 방법을 보여줍니다.
public static byte[] BitmapToByteArray(Bitmap bitmap) { int size = bitmap.Width * bitmap.Height * 4; // 假设为32位RGBA格式 byte[] data = new byte[size]; BitmapData bData = bitmap.LockBits( new Rectangle(0, 0, bitmap.Width, bitmap.Height), ImageLockMode.ReadOnly, PixelFormat.Format32bppArgb); Marshal.Copy(bData.Scan0, data, 0, size); return data; }
다음 코드는 바이트 배열을 다시 비트맵으로 변환하는 방법을 보여줍니다.
public static Bitmap ByteArrayToBitmap(byte[] data, int width, int height) { int size = width * height * 4; // 假设为32位RGBA格式 Bitmap bitmap = new Bitmap(width, height, PixelFormat.Format32bppArgb); BitmapData bData = bitmap.LockBits( new Rectangle(0, 0, width, height), ImageLockMode.WriteOnly, PixelFormat.Format32bppArgb); Marshal.Copy(data, 0, bData.Scan0, size); return bitmap; }
안전하지 않은 코드를 사용하여 픽셀 데이터에 직접 액세스
최고의 성능을 위해서는 안전하지 않은 코드를 사용하여 비트맵 픽셀 데이터에 직접 액세스할 수 있습니다. 이는 마샬링의 오버헤드를 방지하고 가능한 가장 빠른 액세스 속도를 제공합니다.
다음 코드는 안전하지 않은 코드를 사용하여 비트맵 픽셀을 수정하는 예를 보여줍니다.
public unsafe void ModifyPixelsUnsafe(Bitmap bitmap) { BitmapData bData = bitmap.LockBits( new Rectangle(0, 0, bitmap.Width, bitmap.Height), ImageLockMode.ReadWrite, PixelFormat.Format32bppArgb); byte* scan0 = (byte*)bData.Scan0.ToPointer(); // ... 直接操作 scan0 指针访问像素数据 ... }
안전하지 않은 코드는 잘못 사용할 경우 메모리 손상을 일으킬 수 있으므로 주의해서 사용해야 합니다.
위 내용은 더 나은 성능을 위해 C#에서 비트맵 조작을 어떻게 최적화할 수 있습니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










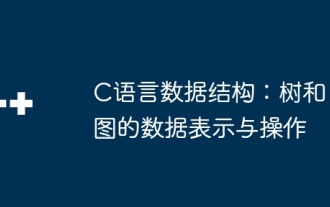
C 언어 데이터 구조 : 트리 및 그래프의 데이터 표현은 노드로 구성된 계층 적 데이터 구조입니다. 각 노드에는 데이터 요소와 하위 노드에 대한 포인터가 포함되어 있습니다. 이진 트리는 특별한 유형의 트리입니다. 각 노드에는 최대 두 개의 자식 노드가 있습니다. 데이터는 structtreenode {intdata; structtreenode*왼쪽; structReenode*오른쪽;}을 나타냅니다. 작업은 트리 트래버스 트리 (사전 조정, 인 순서 및 나중에 순서) 검색 트리 삽입 노드 삭제 노드 그래프는 요소가 정점 인 데이터 구조 모음이며 이웃을 나타내는 오른쪽 또는 무의미한 데이터로 모서리를 통해 연결할 수 있습니다.
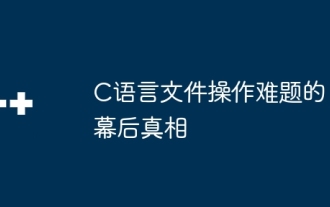
파일 작동 문제에 대한 진실 : 파일 개방이 실패 : 불충분 한 권한, 잘못된 경로 및 파일이 점유 된 파일. 데이터 쓰기 실패 : 버퍼가 가득 차고 파일을 쓸 수 없으며 디스크 공간이 불충분합니다. 기타 FAQ : 파일이 느리게 이동, 잘못된 텍스트 파일 인코딩 및 이진 파일 읽기 오류.
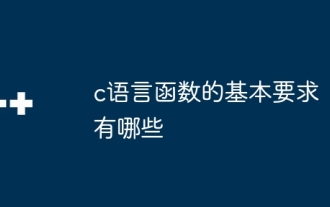
C 언어 기능은 코드 모듈화 및 프로그램 구축의 기초입니다. 그들은 선언 (함수 헤더)과 정의 (기능 본문)로 구성됩니다. C 언어는 값을 사용하여 기본적으로 매개 변수를 전달하지만 주소 패스를 사용하여 외부 변수를 수정할 수도 있습니다. 함수는 반환 값을 가질 수 있거나 가질 수 있으며 반환 값 유형은 선언과 일치해야합니다. 기능 명명은 낙타 또는 밑줄을 사용하여 명확하고 이해하기 쉬워야합니다. 단일 책임 원칙을 따르고 기능 단순성을 유지하여 유지 관리 및 가독성을 향상시킵니다.
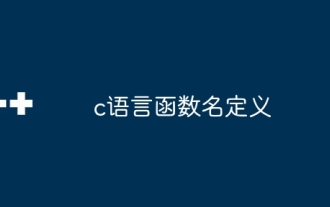
C 언어 함수 이름 정의에는 다음이 포함됩니다. 반환 값 유형, 기능 이름, 매개 변수 목록 및 기능 본문. 키워드와의 충돌을 피하기 위해 기능 이름은 명확하고 간결하며 스타일이 통일되어야합니다. 기능 이름에는 범위가 있으며 선언 후 사용할 수 있습니다. 함수 포인터를 사용하면 기능을 인수로 전달하거나 할당 할 수 있습니다. 일반적인 오류에는 명명 충돌, 매개 변수 유형의 불일치 및 선언되지 않은 함수가 포함됩니다. 성능 최적화는 기능 설계 및 구현에 중점을두고 명확하고 읽기 쉬운 코드는 중요합니다.
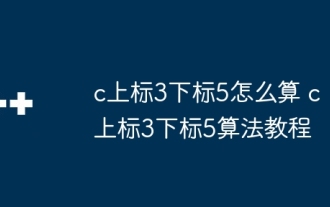
C35의 계산은 본질적으로 조합 수학이며, 5 개의 요소 중 3 개 중에서 선택된 조합 수를 나타냅니다. 계산 공식은 C53 = 5입니다! / (3! * 2!)는 효율을 향상시키고 오버플로를 피하기 위해 루프에 의해 직접 계산할 수 있습니다. 또한 확률 통계, 암호화, 알고리즘 설계 등의 필드에서 많은 문제를 해결하는 데 조합의 특성을 이해하고 효율적인 계산 방법을 마스터하는 데 중요합니다.
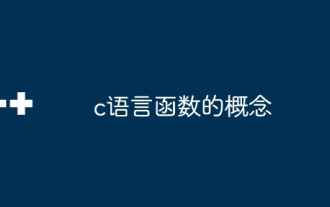
C 언어 기능은 재사용 가능한 코드 블록입니다. 입력, 작업을 수행하며 결과를 반환하여 모듈 식 재사성을 향상시키고 복잡성을 줄입니다. 기능의 내부 메커니즘에는 매개 변수 전달, 함수 실행 및 리턴 값이 포함됩니다. 전체 프로세스에는 기능이 인라인과 같은 최적화가 포함됩니다. 좋은 기능은 단일 책임, 소수의 매개 변수, 이름 지정 사양 및 오류 처리 원칙에 따라 작성됩니다. 함수와 결합 된 포인터는 외부 변수 값 수정과 같은보다 강력한 기능을 달성 할 수 있습니다. 함수 포인터는 함수를 매개 변수 또는 저장 주소로 전달하며 함수에 대한 동적 호출을 구현하는 데 사용됩니다. 기능 기능과 기술을 이해하는 것은 효율적이고 유지 가능하며 이해하기 쉬운 C 프로그램을 작성하는 데 핵심입니다.
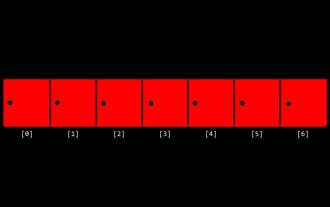
알고리즘은 문제를 해결하기위한 일련의 지침이며 실행 속도 및 메모리 사용량은 다양합니다. 프로그래밍에서 많은 알고리즘은 데이터 검색 및 정렬을 기반으로합니다. 이 기사에서는 여러 데이터 검색 및 정렬 알고리즘을 소개합니다. 선형 검색은 배열 [20,500,10,5,100,1,50]이 있으며 숫자 50을 찾아야한다고 가정합니다. 선형 검색 알고리즘은 대상 값이 발견되거나 전체 배열이 통과 될 때까지 배열의 각 요소를 하나씩 점검합니다. 알고리즘 플로우 차트는 다음과 같습니다. 선형 검색의 의사 코드는 다음과 같습니다. 각 요소를 확인하십시오. 대상 값이 발견되는 경우 : true return false clanue 구현 : #includeintmain (void) {i 포함
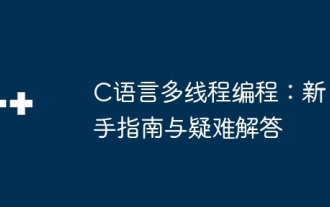
C 언어 멀티 스레딩 프로그래밍 안내서 : 스레드 생성 : pthread_create () 함수를 사용하여 스레드 ID, 속성 및 스레드 함수를 지정합니다. 스레드 동기화 : 뮤텍스, 세마포어 및 조건부 변수를 통한 데이터 경쟁 방지. 실제 사례 : 멀티 스레딩을 사용하여 Fibonacci 번호를 계산하고 여러 스레드에 작업을 할당하고 결과를 동기화하십시오. 문제 해결 : 프로그램 충돌, 스레드 정지 응답 및 성능 병목 현상과 같은 문제를 해결합니다.
