응용 프로그램에 초점이 없는 경우에도 응용 프로그램 이벤트를 트리거하도록 C#에서 전역 단축키를 설정하려면 어떻게 해야 합니까?
이 C# 코드는 애플리케이션이 최소화되거나 초점이 맞지 않는 경우에도 이벤트를 트리거하는 전역 단축키를 생성합니다. 이해를 돕기 위해 설명과 코드 명확성을 개선해 보겠습니다.
C#에서 전역 단축키 만들기
이 문서에서는 애플리케이션 초점에 관계없이 키보드 단축키에 응답하기 위해 C#에서 전역 단축키를 구현하는 방법을 보여줍니다. Ctrl Alt J와 같은 조합을 등록하고 처리하는 데 중점을 둘 것입니다.
해결책: user32.dll
이를 달성하는 열쇠는 user32.dll
라이브러리, 특히 RegisterHotKey
및 UnregisterHotKey
함수에 있습니다. 이러한 함수에는 창 핸들이 필요합니다. 따라서 이 솔루션은 Windows Forms 애플리케이션(WinForms)에 가장 적합합니다. 콘솔 애플리케이션에는 필요한 창 컨텍스트가 부족합니다.
코드 구현:
아래의 개선된 코드는 가독성을 높이고 포괄적인 설명을 포함합니다.
using System; using System.Runtime.InteropServices; using System.Windows.Forms; public sealed class GlobalHotkey : IDisposable { // Import necessary functions from user32.dll [DllImport("user32.dll")] private static extern bool RegisterHotKey(IntPtr hWnd, int id, uint fsModifiers, uint vk); [DllImport("user32.dll")] private static extern bool UnregisterHotKey(IntPtr hWnd, int id); private const int WM_HOTKEY = 0x0312; // Windows message for hotkey events private readonly NativeWindow _window; // Internal window for message handling private int _hotKeyId; // Unique ID for each registered hotkey public GlobalHotkey() { _window = new NativeWindow(); _window.AssignHandle(new CreateParams().CreateHandle()); // Create the window handle _window.WndProc += WndProc; // Assign the window procedure } // Registers a hotkey public void Register(ModifierKeys modifiers, Keys key) { _hotKeyId++; // Generate a unique ID if (!RegisterHotKey(_window.Handle, _hotKeyId, (uint)modifiers, (uint)key)) { throw new Exception("Failed to register hotkey."); } } // Unregisters all hotkeys public void Unregister() { for (int i = 1; i <= _hotKeyId; i++) { UnregisterHotKey(_window.Handle, i); } _window.ReleaseHandle(); // Release the window handle } // Window procedure to handle hotkey messages private void WndProc(ref Message m) { if (m.Msg == WM_HOTKEY) { // Extract key and modifiers from message parameters Keys key = (Keys)(((int)m.LParam >> 16) & 0xFFFF); ModifierKeys modifiers = (ModifierKeys)((int)m.LParam & 0xFFFF); // Raise the HotkeyPressed event HotkeyPressed?.Invoke(this, new HotkeyPressedEventArgs(modifiers, key)); } } // Event fired when a registered hotkey is pressed public event EventHandler<HotkeyPressedEventArgs> HotkeyPressed; // IDisposable implementation public void Dispose() { Unregister(); _window.Dispose(); } } // Event arguments for HotkeyPressed event public class HotkeyPressedEventArgs : EventArgs { public ModifierKeys Modifiers { get; } public Keys Key { get; } public HotkeyPressedEventArgs(ModifierKeys modifiers, Keys key) { Modifiers = modifiers; Key = key; } } // Enum for hotkey modifiers [Flags] public enum ModifierKeys : uint { None = 0, Alt = 1, Control = 2, Shift = 4, Win = 8 }
사용 예(WinForms):
public partial class Form1 : Form { private GlobalHotkey _globalHotkey; public Form1() { InitializeComponent(); _globalHotkey = new GlobalHotkey(); _globalHotkey.HotkeyPressed += GlobalHotkey_HotkeyPressed; _globalHotkey.Register(ModifierKeys.Control | ModifierKeys.Alt, Keys.J); } private void GlobalHotkey_HotkeyPressed(object sender, HotkeyPressedEventArgs e) { // Handle the hotkey press here MessageBox.Show($"Hotkey pressed: Ctrl+Alt+J"); } protected override void OnFormClosing(FormClosingEventArgs e) { _globalHotkey.Dispose(); // Important: Dispose of the hotkey when the form closes base.OnFormClosing(e); } }
리소스 누출을 방지하려면 GlobalHotkey
에 표시된 대로 오류 처리를 추가하고 OnFormClosing
인스턴스를 적절하게 폐기해야 합니다. 이 수정된 코드는 C# WinForms 애플리케이션에서 전역 단축키를 관리하기 위한 더욱 강력하고 이해하기 쉬운 솔루션을 제공합니다.
위 내용은 응용 프로그램에 초점이 없는 경우에도 응용 프로그램 이벤트를 트리거하도록 C#에서 전역 단축키를 설정하려면 어떻게 해야 합니까?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
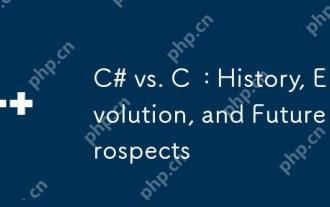
C#과 C의 역사와 진화는 독특하며 미래의 전망도 다릅니다. 1.C는 1983 년 Bjarnestroustrup에 의해 발명되어 객체 지향 프로그래밍을 C 언어에 소개했습니다. Evolution 프로세스에는 자동 키워드 소개 및 Lambda Expressions 소개 C 11, C 20 도입 개념 및 코 루틴과 같은 여러 표준화가 포함되며 향후 성능 및 시스템 수준 프로그래밍에 중점을 둘 것입니다. 2.C#은 2000 년 Microsoft에 의해 출시되었으며 C와 Java의 장점을 결합하여 진화는 단순성과 생산성에 중점을 둡니다. 예를 들어, C#2.0은 제네릭과 C#5.0 도입 된 비동기 프로그래밍을 소개했으며, 이는 향후 개발자의 생산성 및 클라우드 컴퓨팅에 중점을 둘 것입니다.
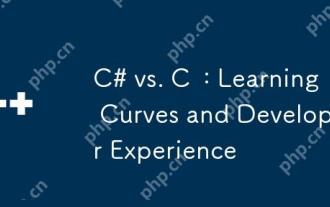
C# 및 C 및 개발자 경험의 학습 곡선에는 상당한 차이가 있습니다. 1) C#의 학습 곡선은 비교적 평평하며 빠른 개발 및 기업 수준의 응용 프로그램에 적합합니다. 2) C의 학습 곡선은 가파르고 고성능 및 저수준 제어 시나리오에 적합합니다.
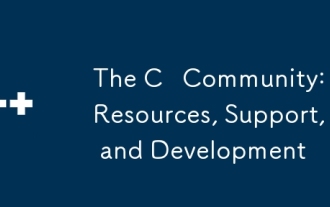
C 학습자와 개발자는 StackoverFlow, Reddit의 R/CPP 커뮤니티, Coursera 및 EDX 코스, GitHub의 오픈 소스 프로젝트, 전문 컨설팅 서비스 및 CPPCon에서 리소스와 지원을받을 수 있습니다. 1. StackoverFlow는 기술적 인 질문에 대한 답변을 제공합니다. 2. Reddit의 R/CPP 커뮤니티는 최신 뉴스를 공유합니다. 3. Coursera와 Edx는 공식적인 C 과정을 제공합니다. 4. LLVM 및 부스트 기술 향상과 같은 GitHub의 오픈 소스 프로젝트; 5. JetBrains 및 Perforce와 같은 전문 컨설팅 서비스는 기술 지원을 제공합니다. 6. CPPCON 및 기타 회의는 경력을 돕습니다
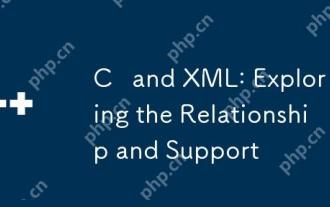
C는 XML과 타사 라이브러리 (예 : TinyXML, Pugixml, Xerces-C)와 상호 작용합니다. 1) 라이브러리를 사용하여 XML 파일을 구문 분석하고 C- 처리 가능한 데이터 구조로 변환하십시오. 2) XML을 생성 할 때 C 데이터 구조를 XML 형식으로 변환하십시오. 3) 실제 애플리케이션에서 XML은 종종 구성 파일 및 데이터 교환에 사용되어 개발 효율성을 향상시킵니다.
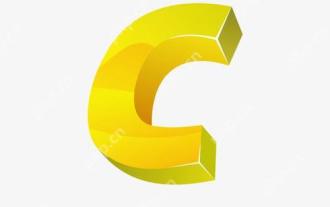
C에서 정적 분석의 적용에는 주로 메모리 관리 문제 발견, 코드 로직 오류 확인 및 코드 보안 개선이 포함됩니다. 1) 정적 분석은 메모리 누출, 이중 릴리스 및 초기화되지 않은 포인터와 같은 문제를 식별 할 수 있습니다. 2) 사용하지 않은 변수, 데드 코드 및 논리적 모순을 감지 할 수 있습니다. 3) Coverity와 같은 정적 분석 도구는 버퍼 오버플로, 정수 오버플로 및 안전하지 않은 API 호출을 감지하여 코드 보안을 개선 할 수 있습니다.
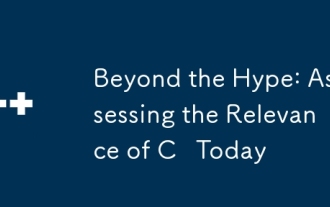
C는 여전히 현대 프로그래밍과 관련이 있습니다. 1) 고성능 및 직접 하드웨어 작동 기능은 게임 개발, 임베디드 시스템 및 고성능 컴퓨팅 분야에서 첫 번째 선택이됩니다. 2) 스마트 포인터 및 템플릿 프로그래밍과 같은 풍부한 프로그래밍 패러다임 및 현대적인 기능은 유연성과 효율성을 향상시킵니다. 학습 곡선은 가파르지만 강력한 기능은 오늘날의 프로그래밍 생태계에서 여전히 중요합니다.
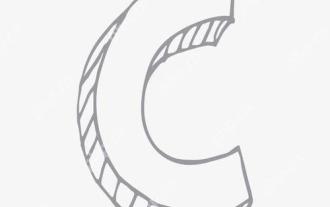
C에서 Chrono 라이브러리를 사용하면 시간과 시간 간격을보다 정확하게 제어 할 수 있습니다. 이 도서관의 매력을 탐구합시다. C의 크로노 라이브러리는 표준 라이브러리의 일부로 시간과 시간 간격을 다루는 현대적인 방법을 제공합니다. 시간과 C 시간으로 고통받는 프로그래머에게는 Chrono가 의심 할 여지없이 혜택입니다. 코드의 가독성과 유지 가능성을 향상시킬뿐만 아니라 더 높은 정확도와 유연성을 제공합니다. 기본부터 시작합시다. Chrono 라이브러리에는 주로 다음 주요 구성 요소가 포함됩니다. std :: Chrono :: System_Clock : 현재 시간을 얻는 데 사용되는 시스템 클럭을 나타냅니다. STD :: 크론
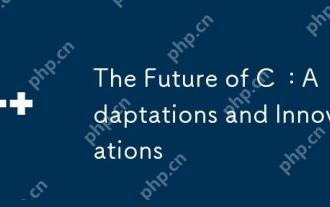
C의 미래는 병렬 컴퓨팅, 보안, 모듈화 및 AI/기계 학습에 중점을 둘 것입니다. 1) 병렬 컴퓨팅은 코 루틴과 같은 기능을 통해 향상 될 것입니다. 2)보다 엄격한 유형 검사 및 메모리 관리 메커니즘을 통해 보안이 향상 될 것입니다. 3) 변조는 코드 구성 및 편집을 단순화합니다. 4) AI 및 머신 러닝은 C가 수치 컴퓨팅 및 GPU 프로그래밍 지원과 같은 새로운 요구에 적응하도록 촉구합니다.
