멀티 스레딩 및 멀티 프로세싱을위한 우수한 파이썬 기술 : 앱 성능 향상
내 Amazon 작가 페이지에서 다양한 책을 찾아보세요. 더 많은 통찰력과 업데이트를 보려면 Medium에서 저를 팔로우하세요! 여러분의 많은 지원 부탁드립니다.
Python의 멀티스레딩 및 멀티프로세싱 기능을 활용하여 애플리케이션의 속도와 효율성을 획기적으로 향상시키세요. 이 가이드에서는 이러한 기능을 효과적으로 활용하기 위한 8가지 필수 기술을 소개합니다.
스레딩은 I/O 중심 작업에 탁월합니다. Python의 threading
모듈은 스레드 관리를 위한 사용자 친화적인 인터페이스를 제공합니다. 여러 파일을 동시에 다운로드하는 방법은 다음과 같습니다.
import threading import requests def download_file(url): response = requests.get(url) filename = url.split('/')[-1] with open(filename, 'wb') as f: f.write(response.content) print(f"Downloaded {filename}") urls = ['http://example.com/file1.txt', 'http://example.com/file2.txt', 'http://example.com/file3.txt'] threads = [] for url in urls: thread = threading.Thread(target=download_file, args=(url,)) threads.append(thread) thread.start() for thread in threads: thread.join() print("All downloads complete")
이 코드는 각 다운로드를 별도의 스레드에 할당하여 동시 실행을 가능하게 합니다.
CPU 바인딩 작업의 경우 Python의 GIL(Global Interpreter Lock) 덕분에 multiprocessing
모듈이 더 우수합니다. 멀티프로세싱은 각각 고유한 메모리 공간과 GIL을 갖춘 독립적인 프로세스를 생성하여 GIL의 제한을 피합니다. 다음은 병렬 계산의 예입니다.
import multiprocessing def calculate_square(number): return number * number if __name__ == '__main__': numbers = range(10) with multiprocessing.Pool() as pool: results = pool.map(calculate_square, numbers) print(results)
프로세스 풀을 활용하여 계산을 효율적으로 분배합니다.
concurrent.futures
모듈은 스레드와 프로세스 모두에서 원활하게 작동하는 비동기 작업 실행을 위한 더 높은 수준의 추상화를 제공합니다. 다음은 ThreadPoolExecutor
:
from concurrent.futures import ThreadPoolExecutor import time def worker(n): print(f"Worker {n} starting") time.sleep(2) print(f"Worker {n} finished") with ThreadPoolExecutor(max_workers=3) as executor: executor.map(worker, range(5)) print("All workers complete")
5개의 작업자 작업을 관리하는 스레드 풀이 생성됩니다.
비동기 I/O의 경우 asyncio
모듈이 빛을 발하여 코루틴을 사용한 효율적인 비동기 프로그래밍을 가능하게 합니다. 예는 다음과 같습니다.
import asyncio import aiohttp async def fetch_url(url): async with aiohttp.ClientSession() as session: async with session.get(url) as response: return await response.text() async def main(): urls = ['http://example.com', 'http://example.org', 'http://example.net'] tasks = [fetch_url(url) for url in urls] results = await asyncio.gather(*tasks) for url, result in zip(urls, results): print(f"Content length of {url}: {len(result)}") asyncio.run(main())
이렇게 하면 여러 URL에서 동시에 콘텐츠를 효율적으로 가져올 수 있습니다.
프로세스 간 데이터 공유에는 특정 도구가 필요합니다. multiprocessing
모듈은 공유 메모리를 위한 Value
과 같은 메커니즘을 제공합니다.
from multiprocessing import Process, Value import time def increment(counter): for _ in range(100): with counter.get_lock(): counter.value += 1 time.sleep(0.01) if __name__ == '__main__': counter = Value('i', 0) processes = [Process(target=increment, args=(counter,)) for _ in range(4)] for p in processes: p.start() for p in processes: p.join() print(f"Final counter value: {counter.value}")
이는 여러 프로세스에 걸친 안전한 카운터 증가를 보여줍니다.
스레드 동기화는 여러 스레드가 공유 리소스에 액세스할 때 경쟁 조건을 방지합니다. Python은 Lock
:
import threading class Counter: def __init__(self): self.count = 0 self.lock = threading.Lock() def increment(self): with self.lock: self.count += 1 def worker(counter, num_increments): for _ in range(num_increments): counter.increment() counter = Counter() threads = [] for _ in range(5): thread = threading.Thread(target=worker, args=(counter, 100000)) threads.append(thread) thread.start() for thread in threads: thread.join() print(f"Final count: {counter.count}")
이 예에서는 원자 카운터 증가를 보장하기 위해 잠금을 사용합니다.
ProcessPoolExecutor
은 CPU 바인딩 작업에 이상적입니다. 다음은 소수를 찾는 예입니다.
from concurrent.futures import ProcessPoolExecutor import math def is_prime(n): if n <= 1: return False if n <= 3: return True if n % 2 == 0 or n % 3 == 0: return False i = 5 while i * i <= n: if n % i == 0 or n % (i + 2) == 0: return False i += 6 return True if __name__ == '__main__': numbers = range(100000) with ProcessPoolExecutor() as executor: results = list(executor.map(is_prime, numbers)) print(sum(results))
이렇게 하면 여러 프로세스에 걸쳐 소수 검사가 분산됩니다.
멀티스레딩과 멀티프로세싱 중에서 선택하는 것은 작업에 따라 다릅니다. I/O 바인딩 작업은 멀티스레딩의 이점을 누리는 반면, CPU 바인딩 작업에는 진정한 병렬 처리를 위해 다중 처리가 필요한 경우가 많습니다. 로드 밸런싱과 작업 종속성은 병렬 처리에서 중요한 고려 사항입니다. 공유 리소스를 처리할 때는 적절한 동기화 메커니즘이 필수적입니다. 성능 비교는 작업 및 시스템에 따라 다릅니다. 데이터 처리 및 과학 컴퓨팅에서는 다중 처리가 매우 효과적일 수 있습니다. 웹 애플리케이션의 경우 asyncio
은 동시 연결을 효율적으로 처리합니다. Python의 다양한 병렬 처리 도구를 사용하면 개발자가 고성능 애플리케이션을 만들 수 있습니다.
101권
작가Aarav Joshi가 공동 설립한 AI 기반 출판사인 101 Books는 저렴한 고품질 도서를 제공하며 일부 도서는 $4.
아마존에서Golang Clean Code 책을 찾아보세요. Aarav Joshi를 검색해 더 많은 책과 특별 할인을 만나보세요!
기타 프로젝트
다른 프로젝트 살펴보기:
Investor Central(영어, 스페인어, 독일어), Smart Living, Epochs & Echoes, Puzzling Mysteries, 힌두트바, 엘리트 Dev, JS Schools
Medium에서 팔로우하세요
Medium에서 우리와 소통하세요:
Tech Koala Insights, Epochs & Echoes World, Investor Central Medium, Puzzling Mysteries Medium, 과학 및 신기원 매체 및 현대 힌두트바.
위 내용은 멀티 스레딩 및 멀티 프로세싱을위한 우수한 파이썬 기술 : 앱 성능 향상의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
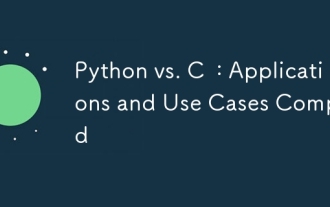
Python은 데이터 과학, 웹 개발 및 자동화 작업에 적합한 반면 C는 시스템 프로그래밍, 게임 개발 및 임베디드 시스템에 적합합니다. Python은 단순성과 강력한 생태계로 유명하며 C는 고성능 및 기본 제어 기능으로 유명합니다.
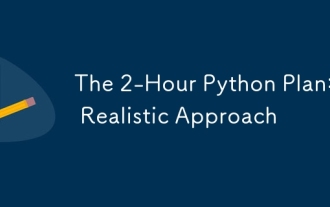
2 시간 이내에 Python의 기본 프로그래밍 개념과 기술을 배울 수 있습니다. 1. 변수 및 데이터 유형을 배우기, 2. 마스터 제어 흐름 (조건부 명세서 및 루프), 3. 기능의 정의 및 사용을 이해하십시오. 4. 간단한 예제 및 코드 스 니펫을 통해 Python 프로그래밍을 신속하게 시작하십시오.
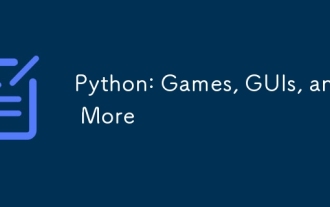
Python은 게임 및 GUI 개발에서 탁월합니다. 1) 게임 개발은 Pygame을 사용하여 드로잉, 오디오 및 기타 기능을 제공하며 2D 게임을 만드는 데 적합합니다. 2) GUI 개발은 Tkinter 또는 PYQT를 선택할 수 있습니다. Tkinter는 간단하고 사용하기 쉽고 PYQT는 풍부한 기능을 가지고 있으며 전문 개발에 적합합니다.
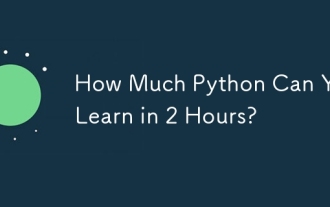
2 시간 이내에 파이썬의 기본 사항을 배울 수 있습니다. 1. 변수 및 데이터 유형을 배우십시오. 이를 통해 간단한 파이썬 프로그램 작성을 시작하는 데 도움이됩니다.
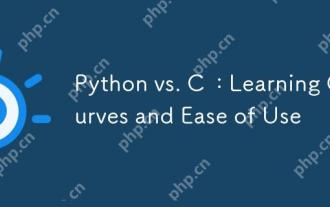
Python은 배우고 사용하기 쉽고 C는 더 강력하지만 복잡합니다. 1. Python Syntax는 간결하며 초보자에게 적합합니다. 동적 타이핑 및 자동 메모리 관리를 사용하면 사용하기 쉽지만 런타임 오류가 발생할 수 있습니다. 2.C는 고성능 응용 프로그램에 적합한 저수준 제어 및 고급 기능을 제공하지만 학습 임계 값이 높고 수동 메모리 및 유형 안전 관리가 필요합니다.
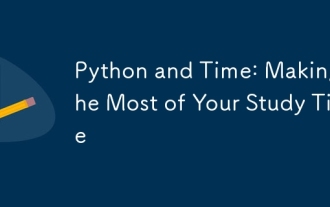
제한된 시간에 Python 학습 효율을 극대화하려면 Python의 DateTime, Time 및 Schedule 모듈을 사용할 수 있습니다. 1. DateTime 모듈은 학습 시간을 기록하고 계획하는 데 사용됩니다. 2. 시간 모듈은 학습과 휴식 시간을 설정하는 데 도움이됩니다. 3. 일정 모듈은 주간 학습 작업을 자동으로 배열합니다.
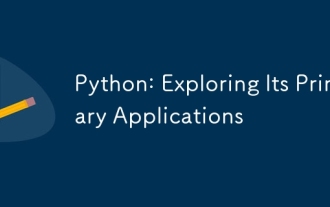
Python은 웹 개발, 데이터 과학, 기계 학습, 자동화 및 스크립팅 분야에서 널리 사용됩니다. 1) 웹 개발에서 Django 및 Flask 프레임 워크는 개발 프로세스를 단순화합니다. 2) 데이터 과학 및 기계 학습 분야에서 Numpy, Pandas, Scikit-Learn 및 Tensorflow 라이브러리는 강력한 지원을 제공합니다. 3) 자동화 및 스크립팅 측면에서 Python은 자동화 된 테스트 및 시스템 관리와 같은 작업에 적합합니다.
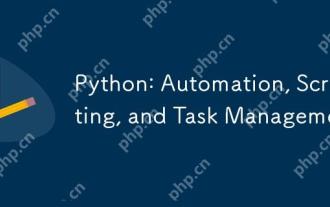
파이썬은 자동화, 스크립팅 및 작업 관리가 탁월합니다. 1) 자동화 : 파일 백업은 OS 및 Shutil과 같은 표준 라이브러리를 통해 실현됩니다. 2) 스크립트 쓰기 : PSUTIL 라이브러리를 사용하여 시스템 리소스를 모니터링합니다. 3) 작업 관리 : 일정 라이브러리를 사용하여 작업을 예약하십시오. Python의 사용 편의성과 풍부한 라이브러리 지원으로 인해 이러한 영역에서 선호하는 도구가됩니다.
