스택 하단에 요소를 삽입하는 Java 프로그램
스택이 비어 있으면 새 요소를 스택으로 밀어야합니다. 예
큐 초기화 : 배열, LinkedList, ArrayList 등과 같은 다른 데이터 구조를 큐 대신 사용할 수 있습니다.
메인 스택이 비어 있으면 새 요소를 메인 스택으로 밀어야하거나 원한다면 보조 스택 위에 요소를 밀 수 있습니다.
원래 순서를 복원하십시오 :
보조 스택에서 모든 요소를 팝하고 메인 스택으로 다시 밀어 넣으십시오. 이것은 원래의 요소 순서를 복원합니다
단계
기본 케이스 :
를 사용하여 스택 하단에 요소를 삽입하는 단계입니다.
임시 변수 초기화 :
스택을 통해 반복 할 때 요소를 일시적으로 유지하기 위해 변수를 만듭니다.
전송 요소 : import java.util.Stack;
public class InsertAtBottomUsingTwoStacks {
public static void insertElementAtBottom(Stack<Integer> mainStack, int x) {
// Create an extra auxiliary stack
Stack<Integer> St2 = new Stack<>();
/* Step 1: Pop all elements from the main stack
and push them into the auxiliary stack */
while (!mainStack.isEmpty()) {
St2.push(mainStack.pop());
}
// Step 2: Push the new element into the main stack
mainStack.push(x);
/* Step 3: Restore the original order by popping each
element from the auxiliary stack and push back to main stack */
while (!St2.isEmpty()) {
mainStack.push(St2.pop());
}
}
public static void main(String[] args) {
Stack<Integer> stack1 = new Stack<>();
stack1.push(1);
stack1.push(2);
stack1.push(3);
stack1.push(4);
System.out.println("Original Stack: " + stack1);
insertElementAtBottom(stack1, 0);
System.out.println("Stack after inserting 0 at the bottom: " + stack1);
}
}
요소를 삽입 한 후 임시 변수의 요소를 다시 스택으로 밀어 넣습니다.
단계
새 요소를 스택에 밀어 넣으십시오.
요소를 복원하십시오 :
import java.util.Stack;
public class InsertAtBottomUsingTwoStacks {
public static void insertElementAtBottom(Stack<Integer> mainStack, int x) {
// Create an extra auxiliary stack
Stack<Integer> St2 = new Stack<>();
/* Step 1: Pop all elements from the main stack
and push them into the auxiliary stack */
while (!mainStack.isEmpty()) {
St2.push(mainStack.pop());
}
// Step 2: Push the new element into the main stack
mainStack.push(x);
/* Step 3: Restore the original order by popping each
element from the auxiliary stack and push back to main stack */
while (!St2.isEmpty()) {
mainStack.push(St2.pop());
}
}
public static void main(String[] args) {
Stack<Integer> stack1 = new Stack<>();
stack1.push(1);
stack1.push(2);
stack1.push(3);
stack1.push(4);
System.out.println("Original Stack: " + stack1);
insertElementAtBottom(stack1, 0);
System.out.println("Stack after inserting 0 at the bottom: " + stack1);
}
}
위 내용은 스택 하단에 요소를 삽입하는 Java 프로그램의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
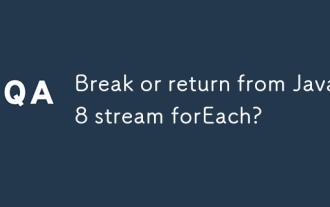
Java 8은 스트림 API를 소개하여 데이터 컬렉션을 처리하는 강력하고 표현적인 방법을 제공합니다. 그러나 스트림을 사용할 때 일반적인 질문은 다음과 같은 것입니다. 기존 루프는 조기 중단 또는 반환을 허용하지만 스트림의 Foreach 메소드는이 방법을 직접 지원하지 않습니다. 이 기사는 이유를 설명하고 스트림 처리 시스템에서 조기 종료를 구현하기위한 대체 방법을 탐색합니다. 추가 읽기 : Java Stream API 개선 스트림 foreach를 이해하십시오 Foreach 메소드는 스트림의 각 요소에서 하나의 작업을 수행하는 터미널 작동입니다. 디자인 의도입니다
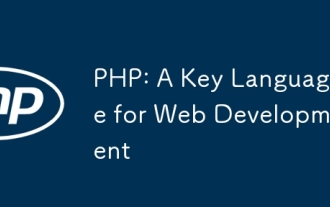
PHP는 서버 측에서 널리 사용되는 스크립팅 언어이며 특히 웹 개발에 적합합니다. 1.PHP는 HTML을 포함하고 HTTP 요청 및 응답을 처리 할 수 있으며 다양한 데이터베이스를 지원할 수 있습니다. 2.PHP는 강력한 커뮤니티 지원 및 오픈 소스 리소스를 통해 동적 웹 컨텐츠, 프로세스 양식 데이터, 액세스 데이터베이스 등을 생성하는 데 사용됩니다. 3. PHP는 해석 된 언어이며, 실행 프로세스에는 어휘 분석, 문법 분석, 편집 및 실행이 포함됩니다. 4. PHP는 사용자 등록 시스템과 같은 고급 응용 프로그램을 위해 MySQL과 결합 할 수 있습니다. 5. PHP를 디버깅 할 때 error_reporting () 및 var_dump ()와 같은 함수를 사용할 수 있습니다. 6. 캐싱 메커니즘을 사용하여 PHP 코드를 최적화하고 데이터베이스 쿼리를 최적화하며 내장 기능을 사용하십시오. 7
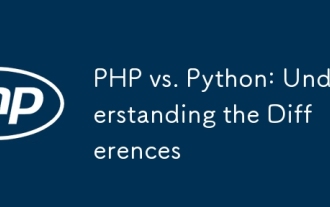
PHP와 Python은 각각 고유 한 장점이 있으며 선택은 프로젝트 요구 사항을 기반으로해야합니다. 1.PHP는 간단한 구문과 높은 실행 효율로 웹 개발에 적합합니다. 2. Python은 간결한 구문 및 풍부한 라이브러리를 갖춘 데이터 과학 및 기계 학습에 적합합니다.
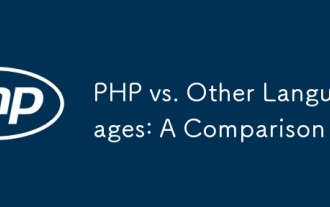
PHP는 특히 빠른 개발 및 동적 컨텐츠를 처리하는 데 웹 개발에 적합하지만 데이터 과학 및 엔터프라이즈 수준의 애플리케이션에는 적합하지 않습니다. Python과 비교할 때 PHP는 웹 개발에 더 많은 장점이 있지만 데이터 과학 분야에서는 Python만큼 좋지 않습니다. Java와 비교할 때 PHP는 엔터프라이즈 레벨 애플리케이션에서 더 나빠지지만 웹 개발에서는 더 유연합니다. JavaScript와 비교할 때 PHP는 백엔드 개발에서 더 간결하지만 프론트 엔드 개발에서는 JavaScript만큼 좋지 않습니다.
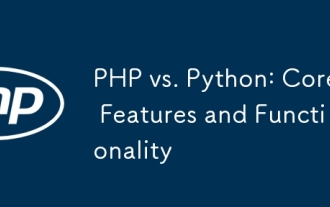
PHP와 Python은 각각 고유 한 장점이 있으며 다양한 시나리오에 적합합니다. 1.PHP는 웹 개발에 적합하며 내장 웹 서버 및 풍부한 기능 라이브러리를 제공합니다. 2. Python은 간결한 구문과 강력한 표준 라이브러리가있는 데이터 과학 및 기계 학습에 적합합니다. 선택할 때 프로젝트 요구 사항에 따라 결정해야합니다.
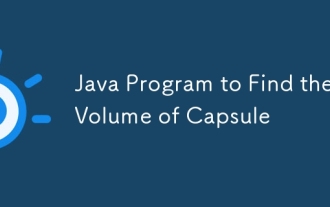
캡슐은 3 차원 기하학적 그림이며, 양쪽 끝에 실린더와 반구로 구성됩니다. 캡슐의 부피는 실린더의 부피와 양쪽 끝에 반구의 부피를 첨가하여 계산할 수 있습니다. 이 튜토리얼은 다른 방법을 사용하여 Java에서 주어진 캡슐의 부피를 계산하는 방법에 대해 논의합니다. 캡슐 볼륨 공식 캡슐 볼륨에 대한 공식은 다음과 같습니다. 캡슐 부피 = 원통형 볼륨 2 반구 볼륨 안에, R : 반구의 반경. H : 실린더의 높이 (반구 제외). 예 1 입력하다 반경 = 5 단위 높이 = 10 단위 산출 볼륨 = 1570.8 입방 단위 설명하다 공식을 사용하여 볼륨 계산 : 부피 = π × r2 × h (4
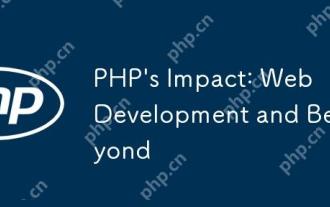
phphassignificallyimpactedwebdevelopmentandextendsbeyondit
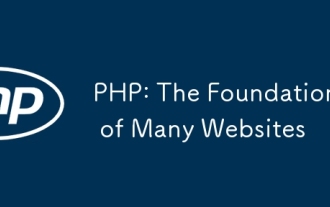
PHP가 많은 웹 사이트에서 선호되는 기술 스택 인 이유에는 사용 편의성, 강력한 커뮤니티 지원 및 광범위한 사용이 포함됩니다. 1) 배우고 사용하기 쉽고 초보자에게 적합합니다. 2) 거대한 개발자 커뮤니티와 풍부한 자원이 있습니다. 3) WordPress, Drupal 및 기타 플랫폼에서 널리 사용됩니다. 4) 웹 서버와 밀접하게 통합하여 개발 배포를 단순화합니다.
