바닐라 JavaScript 및 CSS로 다중 단계 양식을 만드는 방법
.
.
html
코드를 살펴보면 세 섹션과 내비게이션 그룹을 볼 수 있습니다. 섹션에는 양식 입력이 포함되어 있으며 기본 양식 검증이 없습니다. 기본 양식 유효성 검사가 제출 버튼을 클릭 할 때만 트리거되기 때문에 오류 메시지 표시를 더 잘 제어 할 수 있습니다.
열린 기본 스타일
<form > <section > <div > <div > <label for="name">Name <span style="color: red;">*</span></label> <input type="text" name="name" placeholder="Enter your name"> </div> <div > <label for="idNum">ID number <span style="color: red;">*</span></label> <input type="number" name="idNum" placeholder="Enter your ID number"> </div> </div> <div > <div > <label for="email">Email <span style="color: red;">*</span></label> <input type="email" name="email" placeholder="Enter your email"> </div> <div > <label for="birthdate">Date of Birth <span style="color: red;">*</span></label> <input type="date" name="birthdate" max="2006-10-01" min="1924-01-01"> </div> </div> </section> <section > <div > <label for="document">Upload CV <span style="color: red;">*</span></label> <input type="file" name="document" > </div> <div > <label for="department">Department <span style="color: red;">*</span></label> <select name="department"> <option value="">Select a department</option> <option value="hr">Human Resources</option> <option value="it">Information Technology</option> <option value="finance">Finance</option> </select> </div> </section> <section > <div > <label for="skills">Skills (Optional)</label> <textarea name="skills" rows="4" placeholder="Enter your skills"></textarea> </div> <div > <input type="checkbox" name="terms" > <label for="terms">I agree to the terms and conditions <span style="color: red;">*</span></label> </div> <button type="submit">Confirm and Submit</button> </section> <div > <button type="button" >Previous</button> <span ></span> <button type="button" >Next</button> </div> </form> <script src="script.js"></script>
열린 기본 스크립트
이 스크립트는 양식 섹션의 ID에 해당하는 FormStep 값에 따라 섹션을 표시하고 숨기는 메소드를 정의합니다. 양식의 현재 활성 섹션으로 StepInfo를 업데이트합니다. 이 동적 텍스트는 사용자에게 진행 지표 역할을합니다.
그런 다음 페이지가로드하고 내비게이션 버튼에 이벤트를로드하고 클릭 할 수있는 논리를 추가하여 다른 양식 섹션을 통해 순환 할 수 있습니다. 페이지를 새로 고치면 멀티 단계 양식이 예상대로 작동한다는 것을 알 수 있습니다.
마지막으로, 우리는 첫 번째 단계에 있다면 이전 버튼을 숨기고 마지막 섹션에 있으면 다음 버튼을 숨 깁니다.
:root { --primary-color: #8c852a; --secondary-color: #858034; } body { font-family: sans-serif; line-height: 1.4; margin: 0 auto; padding: 20px; background-color: #f4f4f4; max-width: 600px; } h1 { text-align: center; } form { background: #fff; padding: 40px; border-radius: 5px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); display: flex; flex-direction: column; } .form-group { display: flex; gap: 7%; } .form-group > div { width: 100%; } input:not([type="checkbox"]), select, textarea { width: 100%; padding: 8px; border: 1px solid #ddd; border-radius: 4px; } .form-control { margin-bottom: 15px; } button { display: block; width: 100%; padding: 10px; color: white; background-color: var(--primary-color); border: none; border-radius: 4px; cursor: pointer; font-size: 16px; } button:hover { background-color: var(--secondary-color); } .group-two, .group-three { display: none; } .arrows { display: flex; justify-content: space-between align-items: center; margin-top: 10px; } #navLeft, #navRight { width: fit-content; } @media screen and (max-width: 600px) { .form-group { flex-direction: column; } }
유효성 검사 스크립트
<form > <section > <div > <div > <label for="name">Name <span style="color: red;">*</span></label> <input type="text" name="name" placeholder="Enter your name"> </div> <div > <label for="idNum">ID number <span style="color: red;">*</span></label> <input type="number" name="idNum" placeholder="Enter your ID number"> </div> </div> <div > <div > <label for="email">Email <span style="color: red;">*</span></label> <input type="email" name="email" placeholder="Enter your email"> </div> <div > <label for="birthdate">Date of Birth <span style="color: red;">*</span></label> <input type="date" name="birthdate" max="2006-10-01" min="1924-01-01"> </div> </div> </section> <section > <div > <label for="document">Upload CV <span style="color: red;">*</span></label> <input type="file" name="document" > </div> <div > <label for="department">Department <span style="color: red;">*</span></label> <select name="department"> <option value="">Select a department</option> <option value="hr">Human Resources</option> <option value="it">Information Technology</option> <option value="finance">Finance</option> </select> </div> </section> <section > <div > <label for="skills">Skills (Optional)</label> <textarea name="skills" rows="4" placeholder="Enter your skills"></textarea> </div> <div > <input type="checkbox" name="terms" > <label for="terms">I agree to the terms and conditions <span style="color: red;">*</span></label> </div> <button type="submit">Confirm and Submit</button> </section> <div > <button type="button" >Previous</button> <span ></span> <button type="button" >Next</button> </div> </form> <script src="script.js"></script>
열린 유효성 검사 스타일
:root { --primary-color: #8c852a; --secondary-color: #858034; } body { font-family: sans-serif; line-height: 1.4; margin: 0 auto; padding: 20px; background-color: #f4f4f4; max-width: 600px; } h1 { text-align: center; } form { background: #fff; padding: 40px; border-radius: 5px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); display: flex; flex-direction: column; } .form-group { display: flex; gap: 7%; } .form-group > div { width: 100%; } input:not([type="checkbox"]), select, textarea { width: 100%; padding: 8px; border: 1px solid #ddd; border-radius: 4px; } .form-control { margin-bottom: 15px; } button { display: block; width: 100%; padding: 10px; color: white; background-color: var(--primary-color); border: none; border-radius: 4px; cursor: pointer; font-size: 16px; } button:hover { background-color: var(--secondary-color); } .group-two, .group-three { display: none; } .arrows { display: flex; justify-content: space-between align-items: center; margin-top: 10px; } #navLeft, #navRight { width: fit-content; } @media screen and (max-width: 600px) { .form-group { flex-direction: column; } }
실시간 유효성 검사 스크립트를 열어
const stepInfo = document.getElementById("stepInfo"); const navLeft = document.getElementById("navLeft"); const navRight = document.getElementById("navRight"); const form = document.getElementById("myForm"); const formSteps = ["one", "two", "three"]; let currentStep = 0; function updateStepVisibility() { formSteps.forEach((step) => { document.getElementById(step).style.display = "none"; }); document.getElementById(formSteps[currentStep]).style.display = "block"; stepInfo.textContent = `Step ${currentStep + 1} of ${formSteps.length}`; navLeft.style.display = currentStep === 0 ? "none" : "block"; navRight.style.display = currentStep === formSteps.length - 1 ? "none" : "block"; } document.addEventListener("DOMContentLoaded", () => { navLeft.style.display = "none"; updateStepVisibility(); navRight.addEventListener("click", () => { if (currentStep < formSteps.length - 1) { currentStep++; updateStepVisibility(); } }); navLeft.addEventListener("click", () => { if (currentStep > 0) { currentStep--; updateStepVisibility(); } }); });
다단계 형식으로, 제출하기 전에 마지막에 모든 답변에 대한 요약을 사용자에게 보여주고 필요한 경우 답변을 편집 할 수있는 옵션을 제공하는 것이 가치가 있습니다. 그 사람은 뒤로 탐색하지 않고는 이전 단계를 볼 수 없으므로 마지막 단계에서 요약을 보여 주면 확신과 실수를 수정할 수 있습니다. 마크 업에 네 번째 섹션을 추가 하여이 요약보기를 보유하고 제출 버튼을 움직입니다. index.html의 세 번째 섹션 바로 아래에 붙여 넣습니다
html
<form > <section > <div > <div > <label for="name">Name <span style="color: red;">*</span></label> <input type="text" name="name" placeholder="Enter your name"> </div> <div > <label for="idNum">ID number <span style="color: red;">*</span></label> <input type="number" name="idNum" placeholder="Enter your ID number"> </div> </div> <div > <div > <label for="email">Email <span style="color: red;">*</span></label> <input type="email" name="email" placeholder="Enter your email"> </div> <div > <label for="birthdate">Date of Birth <span style="color: red;">*</span></label> <input type="date" name="birthdate" max="2006-10-01" min="1924-01-01"> </div> </div> </section> <section > <div > <label for="document">Upload CV <span style="color: red;">*</span></label> <input type="file" name="document" > </div> <div > <label for="department">Department <span style="color: red;">*</span></label> <select name="department"> <option value="">Select a department</option> <option value="hr">Human Resources</option> <option value="it">Information Technology</option> <option value="finance">Finance</option> </select> </div> </section> <section > <div > <label for="skills">Skills (Optional)</label> <textarea name="skills" rows="4" placeholder="Enter your skills"></textarea> </div> <div > <input type="checkbox" name="terms" > <label for="terms">I agree to the terms and conditions <span style="color: red;">*</span></label> </div> <button type="submit">Confirm and Submit</button> </section> <div > <button type="button" >Previous</button> <span ></span> <button type="button" >Next</button> </div> </form> <script src="script.js"></script>
우리의 다중 단계 양식은 이제 사용자가 제출하기 전에 제공하는 모든 정보를 편집하고 볼 수 있습니다. 접근성 팁
다단계 양식에 접근 할 수있는 것은 기본 사항으로 시작됩니다 :시맨틱 html 사용.
이것은 전투의 절반입니다. 적절한 양식 레이블을 사용하여 밀접하게 뒤따른다.:root { --primary-color: #8c852a; --secondary-color: #858034; } body { font-family: sans-serif; line-height: 1.4; margin: 0 auto; padding: 20px; background-color: #f4f4f4; max-width: 600px; } h1 { text-align: center; } form { background: #fff; padding: 40px; border-radius: 5px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); display: flex; flex-direction: column; } .form-group { display: flex; gap: 7%; } .form-group > div { width: 100%; } input:not([type="checkbox"]), select, textarea { width: 100%; padding: 8px; border: 1px solid #ddd; border-radius: 4px; } .form-control { margin-bottom: 15px; } button { display: block; width: 100%; padding: 10px; color: white; background-color: var(--primary-color); border: none; border-radius: 4px; cursor: pointer; font-size: 16px; } button:hover { background-color: var(--secondary-color); } .group-two, .group-three { display: none; } .arrows { display: flex; justify-content: space-between align-items: center; margin-top: 10px; } #navLeft, #navRight { width: fit-content; } @media screen and (max-width: 600px) { .form-group { flex-direction: column; } }
이것은 스크린 리더에게 기본 검증에 의존하지 않고 필드가 필요하다는 것을 알 수 있습니다. acd add acd acd add add add add add add add add add add add add add add add add add add add ad add add add ad add ad add ad ad add ad add ad add ad ad add ad add ad ad ad add prans.
이것은 스크린 리더가 입력이 오류 상태에있을 때 중요성을 줄 수 있도록 도와줍니다. . add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add add ad add ad add add add ad add ad add ad add ad add add ad add ad add ad add ad add ad add ad add ad add add ad add aia-live = "polite".
결론 우리는 구직 응용 프로그램을위한 4 부분으로 된 다중 단계 양식입니다! 이 기사의 맨 위에서 말했듯이, 저글링 할 것이 많습니다. 너무 많은 상자 솔루션을 찾는 것에 대해 잘못을 겪지 않을 것입니다.
..
웹 양식 (MDN)을 구조화하는 방법
위 내용은 바닐라 JavaScript 및 CSS로 다중 단계 양식을 만드는 방법의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










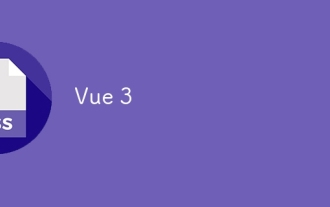
그것은#039; VUE 팀에게 그것을 끝내는 것을 축하합니다. 나는 그것이 막대한 노력과 오랜 시간이라는 것을 알고 있습니다. 모든 새로운 문서도 있습니다.
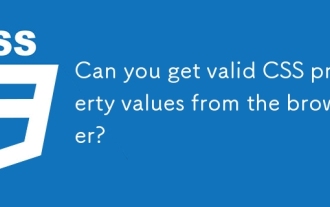
나는 누군가이 매우 합법적 인 질문으로 글을 썼습니다. Lea는 브라우저에서 유효한 CSS 속성 자체를 얻는 방법에 대해 블로그를 작성했습니다. 이는 이와 같습니다.
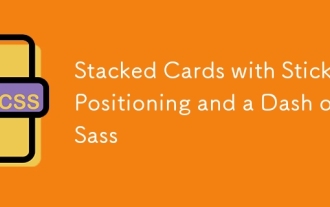
다른 날, 나는 Corey Ginnivan의 웹 사이트에서 스크롤 할 때 카드 모음이 서로 쌓이는 것을 발견했습니다.
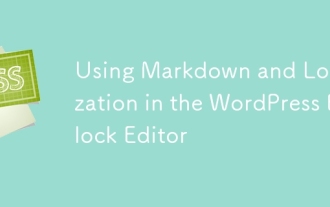
WordPress 편집기에서 사용자에게 직접 문서를 표시 해야하는 경우 가장 좋은 방법은 무엇입니까?
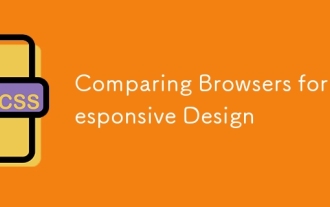
목표가 귀하의 사이트를 동시에 다른 크기로 표시하는 이러한 데스크탑 앱이 많이 있습니다. 예를 들어, 글을 쓸 수 있습니다
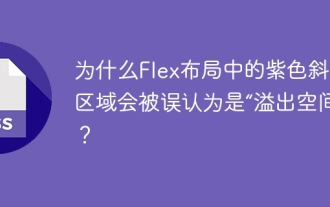
플렉스 레이아웃의 보라색 슬래시 영역에 대한 질문 플렉스 레이아웃을 사용할 때 개발자 도구 (d ...)와 같은 혼란스러운 현상이 발생할 수 있습니다.
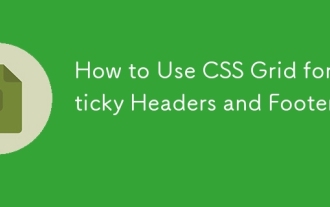
CSS 그리드는 레이아웃이 그 어느 때보 다 쉽게 레이아웃을 만들 수 있도록 설계된 속성 모음입니다. 어쨌든, 약간의 학습 곡선이 있지만 그리드는
