JavaScript_javascript 기술의 브라우저 호환성 문제에 대한 간략한 분석
브라우저 호환성 문제는 간과되기 쉽지만 실제 개발에 있어서 가장 중요한 부분입니다. 이전 버전의 브라우저와의 호환성 문제에 대해 이야기하기 전에 먼저 브라우저에 이 기능이 있는지 감지하는 데 사용됩니다. 즉, 현재 브라우저가 해당 속성이나 메서드를 지원하는지 확인하는 데 사용됩니다. 라고 불리는. 다음은 몇 가지 간략한 소개입니다.
1.innerText 및 innerContent
1) innerText와 innerContent는 동일한 기능을 갖습니다
2) innerText는 IE8 이전의 브라우저에서 지원됩니다
3) innerContent는 이전 버전의 Firefox에서 지원됩니다
4) 새 버전의 브라우저는 두 가지 방법을 모두 지원합니다
1 // 老版本浏览器兼容 innerText 和 innerContent 2 if (element.textContent) { 3 return element.textContent ; 4 } else { 5 return element.innerText; 6 }
2. 형제 노드/요소 획득 시 호환성 문제
1) 브라더 노드, 모든 브라우저 지원
①nextSibling 다음 형제 노드는 요소가 아닌 노드일 수 있습니다.
가 획득됩니다.
②previousSibling 이전 형제 노드는 요소가 아닌 노드일 수 있습니다. 텍스트 노드
를 얻습니다.
2) Brother 요소, IE8은 이전에 지원하지 않았습니다
①previousElementSibling 이전 형제 요소를 가져오고 공백을 무시합니다
②NEXTELEMENTSIBLING은 다음 이웃 형제 요소를 얻으려면 공백을 무시합니다
//兼容浏览器 // 获取下一个紧邻的兄弟元素 function getNextElement(element) { // 能力检测 if(element.nextElementSibling) { return element.nextElementSibling; } else { var node = element.nextSibling; while(node && node.nodeType !== 1) { node = node.nextibling; } return node; } }
/** * 返回上一个元素 * @param element * @returns {*} */ function getPreviousElement(element) { if(element.previousElementSibling) { return element.previousElementSibling; }else { var el = element.previousSibling; while(el && el.nodeType !== 1) { el = el.previousSibling; } return el; } }
/** * 返回第一个元素firstElementChild的浏览器兼容 * @param parent * @returns {*} */ function getFirstElement(parent) { if(parent.firstElementChild) { return parent.firstElementChild; }else { var el = parent.firstChild; while(el && el.nodeType !== 1) { el = el.nextSibling; } return el; } }
/** * 返回最后一个元素 * @param parent * @returns {*} */ function getLastElement(parent) { if(parent.lastElementChild) { return parent.lastElementChild; }else { var el = parent.lastChild; while(el && el.nodeType !== 1) { el = el.previousSibling; } return el; } }
/** *获取当前元素的所有兄弟元素 * @param element * @returns {Array} */ function sibling(element) { if(!element) return ; var elements = [ ]; var el = element.previousSibling; while(el) { if(el.nodeType === 1) { elements.push(el); } el = el.previousSibling; } el = element.previousSibling; while(el ) { if(el.nodeType === 1) { elements.push(el); } el = el.nextSibling; } return elements; }
3. 배열.필터()
// 지정된 함수를 사용하여 모든 요소를 테스트하고 테스트를 통과한 모든 요소를 포함하는 새 배열을 만듭니다.
// 兼容旧环境 if (!Array.prototype.filter) { Array.prototype.filter = function(fun /*, thisArg */) { "use strict"; if (this === void 0 || this === null) throw new TypeError(); var t = Object(this); var len = t.length >>> 0; if (typeof fun !== "function") throw new TypeError(); var res = []; var thisArg = arguments.length >= 2 ? arguments[1] : void 0; for (var i = 0; i < len; i++) { if (i in t) { var val = t[i]; // NOTE: Technically this should Object.defineProperty at // the next index, as push can be affected by // properties on Object.prototype and Array.prototype. // But that method's new, and collisions should be // rare, so use the more-compatible alternative. if (fun.call(thisArg, val, i, t)) res.push(val); } } return res; }; }
4. array.forEach();
// 배열 탐색
//兼容旧环境 // Production steps of ECMA-262, Edition 5, 15.4.4.18 // Reference: http://es5.github.io/#x15.4.4.18 if (!Array.prototype.forEach) { Array.prototype.forEach = function(callback, thisArg) { var T, k; if (this == null) { throw new TypeError(' this is null or not defined'); } // 1. Let O be the result of calling toObject() passing the // |this| value as the argument. var O = Object(this); // 2. Let lenValue be the result of calling the Get() internal // method of O with the argument "length". // 3. Let len be toUint32(lenValue). var len = O.length >>> 0; // 4. If isCallable(callback) is false, throw a TypeError exception. // See: http://es5.github.com/#x9.11 if (typeof callback !== "function") { throw new TypeError(callback + ' is not a function'); } // 5. If thisArg was supplied, let T be thisArg; else let // T be undefined. if (arguments.length > 1) { T = thisArg; } // 6. Let k be 0 k = 0; // 7. Repeat, while k < len while (k < len) { var kValue; // a. Let Pk be ToString(k). // This is implicit for LHS operands of the in operator // b. Let kPresent be the result of calling the HasProperty // internal method of O with argument Pk. // This step can be combined with c // c. If kPresent is true, then if (k in O) { // i. Let kValue be the result of calling the Get internal // method of O with argument Pk. kValue = O[k]; // ii. Call the Call internal method of callback with T as // the this value and argument list containing kValue, k, and O. callback.call(T, kValue, k, O); } // d. Increase k by 1. k++; } // 8. return undefined }; }
5. 등록 이벤트
.addEventListener = 함수(유형,리스너,useCapture) { };
//첫 번째 매개변수 이벤트 이름
//두 번째 매개변수 이벤트 처리 함수(리스너)
//세 번째 매개변수 true는 거짓 버블링을 캡처합니다.
//IE9 이후에만 지원
// 기존 환경과 호환
var EventTools = { addEventListener: function (element, eventName, listener) { //能力检测 if(element.addEventListener) { element.addEventListener(eventName, listener,false); }else if(element.attachEvent) { element.attachEvent("on" + eventName, listener); }else{ element["on" + eventName] = listener; } }, // 想要移除事件,不能使用匿名函数 removeEventListener: function (element, eventName, listener) { if(element.removeEventListener) { element.removeEventListener(eventName,listener,false); }else if(element.detachEvent) { //IE8以前注册.attachEvent和移除事件.detachEvent element.detachEvent("on"+eventName,listener); }else{ element["on" + eventName] = null; } } };
1) 이벤트 매개변수 e는 이벤트 객체, 표준 획득 방식
btn.onclick = 함수(e) { }
2) IE8 이전에는 지원되지 않았던 e.eventPhase 이벤트 단계
3)e.target은 항상 이벤트(클릭된 버튼)를 트리거하는 개체입니다.
i) IE8 srcElement 이전
ii) 브라우저 호환
var target = e.target || window.event.srcElement;
// 获取事件对象 兼容浏览器 getEvent: function(e) { return e || window.event; // e事件对象 标准的获取方式; window.event IE8以前获取事件对象的方式 } // 兼容target getTarget: function(e) { return e.target || e.srcElement; }
①보이는 영역의 위치 : e.clientX e.clientY
②문서 내 위치 :
i) e.pageX e.pageY
ii) 브라우저 호환
var scrollTop = document.documentElement.scrollTop || document.body.scrollTop; var pageY = e.clientY + scrollTop;
// 兼容浏览器 var scrollTop = document.documentElement.scrollTop || document.body.scrolltop;
// 兼容浏览器 window.getSelection ? window.getSelection().removeAllRanges() : document.selection.empty();
위의 JavaScript 브라우저 호환성 문제에 대한 간략한 분석은 모두 편집자가 공유한 내용이므로 참고가 되기를 바라며, Script Home을 지원해 주시길 바랍니다.

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










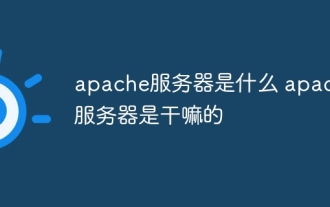
Apache Server는 브라우저와 웹 사이트 서버 간의 브리지 역할을하는 강력한 웹 서버 소프트웨어입니다. 1. HTTP 요청을 처리하고 요청에 따라 웹 페이지 컨텐츠를 반환합니다. 2. 모듈 식 디자인은 SSL 암호화 지원 및 동적 웹 페이지와 같은 확장 된 기능을 허용합니다. 3. 보안 취약점을 피하고 고성능 웹 애플리케이션을 구축하기 위해 보안 취약점을 피하고 스레드 카운트 및 타임 아웃 시간과 같은 성능 매개 변수를 최적화하기 위해 구성 파일 (예 : 가상 호스트 구성)을 신중하게 설정해야합니다.
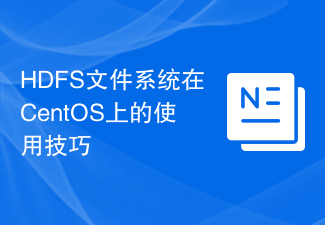
CentOS 시스템 하의 HDFS 파일 시스템에 대한 설치, 구성 및 최적화 안내서이 기사에서는 CentOS 시스템에 HDF (Hadoop Distributed File System)를 설치, 구성 및 최적화하는 방법을 안내합니다. HDFS 설치 및 구성 Java 환경 설치 : 먼저 적절한 Java 환경이 설치되어 있는지 확인하십시오. /etc/profile 파일 편집, 다음을 추가하고 /usr/lib/java-1.8.0/jdk1.8.0_144를 실제 Java 설치 경로로 바꾸십시오 : Exportjava_home =/usr/lib/java-1.8.0/jdk1.8.0_144 exportpath = $ j
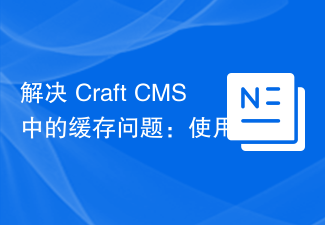
CRAFTCMS를 사용하여 웹 사이트를 개발할 때 특히 CSS 및 JavaScript 파일을 자주 업데이트 할 때 자주 리소스 파일 캐싱 문제가 발생하면 이전 버전의 파일이 여전히 브라우저에서 캐싱 될 수 있으므로 사용자는 최신 변경 사항을 볼 수 없습니다. 이 문제는 사용자 경험에 영향을 줄뿐만 아니라 개발 및 디버깅의 어려움을 증가시킵니다. 최근에 나는 프로젝트에서 비슷한 문제를 겪었고, 약간의 탐색 후 플러그인 Wiejeben/Craft-Laravel-Mix를 발견하여 캐싱 문제를 완벽하게 해결했습니다.
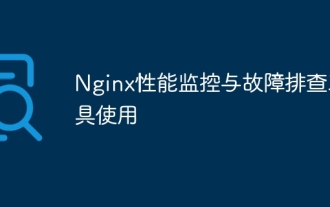
NGINX 성능 모니터링 및 문제 해결은 주로 다음 단계를 통해 수행됩니다. 1. NGINX-V를 사용하여 버전 정보를보고 STUB_STATUS 모듈을 활성화하여 활성 연결 수, 요청 및 캐시 적중률을 모니터링합니다. 2. 상위 명령을 사용하여 시스템 리소스 점유, Iostat 및 VMSTAT 모니터 디스크 I/O 및 메모리 사용을 모니터링합니다. 3. TCPDUMP를 사용하여 패킷을 캡처하여 네트워크 트래픽을 분석하고 네트워크 연결 문제를 해결합니다. 4. 동시 처리 기능이 충분하지 않거나 과도한 프로세스 컨텍스트 오버 헤드를 피하기 위해 작업자 프로세스 수를 올바르게 구성합니다. 5. 부적절한 캐시 크기 설정을 피하기 위해 Nginx 캐시를 올바르게 구성하십시오. 6. awk 및 grep 명령 또는 elk 사용과 같은 nginx 로그를 분석하여
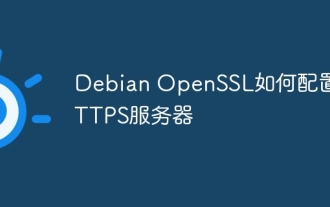
데비안 시스템에서 HTTPS 서버를 구성하려면 필요한 소프트웨어 설치, SSL 인증서 생성 및 SSL 인증서를 사용하기 위해 웹 서버 (예 : Apache 또는 Nginx)를 구성하는 등 여러 단계가 포함됩니다. 다음은 Apacheweb 서버를 사용하고 있다고 가정하는 기본 안내서입니다. 1. 필요한 소프트웨어를 먼저 설치하고 시스템이 최신 상태인지 확인하고 Apache 및 OpenSSL을 설치하십시오 : Sudoaptupdatesudoaptupgradesudoaptinsta
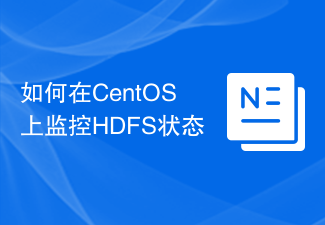
CentOS 시스템에서 HDFS (Hadoop 분산 파일 시스템)의 상태를 모니터링하는 방법에는 여러 가지가 있습니다. 이 기사는 가장 적합한 솔루션을 선택하는 데 도움이되는 몇 가지 일반적으로 사용되는 방법을 소개합니다. 1. Hadoop의 자체 웹 인터페이스 인 Hadoop의 자체 Webui를 사용하여 클러스터 상태 모니터링 기능을 제공하십시오. 단계 : Hadoop 클러스터가 가동되고 있는지 확인하십시오. webui에 액세스하십시오 : 브라우저에 http : // : 50070 (hadoop2.x) 또는 http : // : 9870 (hadoop3.x)을 입력하십시오. 기본 사용자 이름과 비밀번호는 일반적으로 HDFS/HDF입니다. 2. 명령 줄 도구 모니터링 Hadoop은 모니터링을 용이하게하기위한 일련의 명령 줄 도구를 제공합니다.
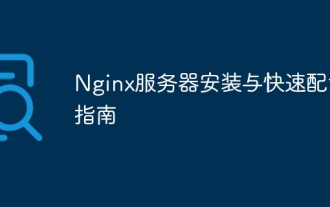
이 기사에서는 Nginx의 구성 및 구성 방법을 소개합니다. 1. nginx 설치 : Centos에서 sudoyumininstallnginx를 사용하고 Ubuntu에서 sudoapt-getinstallnginx를 사용하고 설치 후 sudosystemctlstartnginx로 시작하십시오. 2. 기본 구성 : /etc/nginx/nginx.conf 파일을 수정하고 주로 서버 블록에서 청취 (포트) 및 루트 (사이트 루 디렉토리) 지침을 수정하고 수정 후 sudosystemctlrestartnginx를 사용하여 다시 시작하고 적용하십시오. 3. 가상 호스트 구성 : nginx.co에서
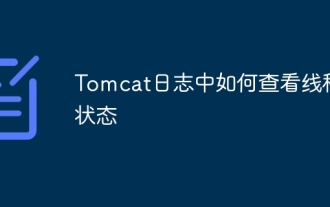
Tomcat 로그에서 스레드 상태를 보려면 다음 방법을 사용할 수 있습니다. TomcatmanagerWeb 인터페이스 : 브라우저에 Tomcat (일반적으로 http : // localhost : 8080/manager)의 관리 주소를 입력하고 로그인 한 후 JMX 모니터링 도구를 사용하여 Tomcat의 MBEAN Server를 사용하여 MBEAN의 MBEAN의 MBEN 모니터를 사용합니다. 스레드 풀. jconsole에서 선택하십시오
