AngularJS는 ngOption을 사용하여 드롭다운 목록 예제 code_AngularJS를 구현합니다.
드롭다운 목록을 이용한 간단한 사용
ng-option 지시문은 사용이 매우 간단합니다.
두 가지 속성만 바인딩하면 됩니다.
하나는 선택한 값을 가져오는 데 사용되는 ng-model입니다.
다른 하나는 드롭다운 목록을 결정하기 위해 ng-options에서 사용하는 요소 배열입니다.
<select ng-model="engineer.currentActivity" class="form-control" ng-options="act for act in activities"></select>
위 문은 선택한 값과 Engineer.currentActivity 사이에 양방향 데이터 바인딩을 수행한 후 목록에 있는 옵션이 활동의 각 값입니다. 데이터는 다음과 같습니다.
$scope.engineer = { name: "Dani", currentActivity: "Fixing bugs" }; $scope.activities = [ "Writing code", "Testing code", "Fixing bugs", "Dancing" ];
작업 결과는 다음과 같습니다.
여기서는 아름다움을 위해 부트스트랩을 인용합니다.
<html ng-app="myApp"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <script src="http://apps.bdimg.com/libs/angular.js/1.2.16/angular.min.js"></script> <link rel="stylesheet" href="http://apps.bdimg.com/libs/bootstrap/3.3.0/css/bootstrap.min.css"> <script src="http://apps.bdimg.com/libs/jquery/2.1.1/jquery.min.js"></script> <script src="http://apps.bdimg.com/libs/bootstrap/3.3.0/js/bootstrap.min.js"></script> </head> <body> <div ng-controller="EngineeringController" class="container"> <div class="col-md-12"> {{engineer.name}} is currently: {{ engineer.currentActivity}} </div> <div class="col-md-4"> <label for="name">Choose a new activity:</label> <select ng-model="engineer.currentActivity" class="form-control" ng-options="act for act in activities"> </select> </div> </div> <script type="text/javascript"> var myAppModule = angular.module("myApp",[]); myAppModule.controller("EngineeringController",["$scope",function($scope){ $scope.engineer = { name: "Dani", currentActivity: "Fixing bugs" }; $scope.activities = [ "Writing code", "Testing code", "Fixing bugs", "Dancing" ]; }]); </script> </body> </html>
복잡한 개체, 사용자 정의 목록 이름
때때로 드롭다운 목록은 단순한 문자열 배열이 아니지만 json 객체일 수 있습니다. 예:
$scope.activities = [ { id: 1, type: "Work" , name: "Writing code" }, { id: 2, type: "Work" , name: "Testing code" }, { id: 3, type: "Work" , name: "Fixing bugs" }, { id: 4, type: "Play" , name: "Dancing" } ];
이때 바인딩된 데이터는 여기에 있는 데이터와 동일한 형식이어야 합니다. 예를 들어 다음 중 하나를 직접 복사하세요.
$scope.engineer = { name: "Dani" , currentActivity: { id: 3, type: "Work" , name: "Fixing bugs" } };
물론 다음과 같이 직접 지정할 수도 있습니다.
$scope.engineer = {currentActivity:activities[3]}
그런 다음 명령에서 목록이 이어진 드롭다운 상자의 이름을 반복할 수 있습니다.
<select ng-model = "engineer.currentActivity" class="form-control" ng-options = "a.name +' (' + a.type + ')' for a in activities" > </select >
작동 효과는 다음과 같습니다.
모든 코드는 다음과 같습니다.
{{engineer.name}} is currently: {{ engineer.currentActivity}}<select ng-model = "engineer.currentActivity" class="form-control" ng-options = "a.name +' (' + a.type + ')' for a in activities" > </select >
드롭다운 목록 그룹화 구현
실제로 그룹화는 이전 예와 매우 유사합니다. 공간의 ng-options 값을 다음과 같이 변경하면 됩니다.
<select ng-model = "engineer.currentActivity" class="form-control" ng-options = "a.name group by a.type for a in activities" > </select >
그룹 기준을 추가하면 다음 값에 따라 그룹화됩니다
모든 코드:
{{engineer.name}} is currently: {{ engineer.currentActivity}}<select ng-model = "engineer.currentActivity" class="form-control" ng-options = "a.name group by a.type for a in activities" > </select >
ID로 식별
이전 ng-model은 초기에 값을 설정하는 것과 동일하기 때문입니다. 드롭다운 목록 옵션을 선택하면 이 초기 값을 덮어씁니다.
식별을 위해 ID를 사용하는 경우가 많아지므로 할당을 초기화할 때 ID만 설정하면 됩니다.
$scope.engineer = { currentActivityId: 3 }; $scope.activities = [ { id: 1, type: "Work" , name: "Writing code" }, { id: 2, type: "Work" , name: "Testing code" }, { id: 3, type: "Work" , name: "Fixing bugs" }, { id: 4, type: "Play" , name: "Dancing" } ];
명령어는 다음과 같은 형식으로 작성할 수 있습니다
<select ng-model = "engineer.currentActivityId" class="form-control" ng-options = "a.id as a.name group by a.type for a in activities" > </select >
as 앞의 값으로 유일한 옵션을 결정할 수 있습니다
모든 코드는 다음과 같습니다.
current is: {{ engineer.currentActivityId}}<select ng-model = "engineer.currentActivityId" class="form-control" ng-options = "a.id as a.name group by a.type for a in activities" > </select >
위는 ngOption을 사용하여 AngularJS에서 드롭다운 목록을 구현하는 방법에 대해 편집자가 공유한 예제 코드입니다. 도움이 되길 바랍니다.

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










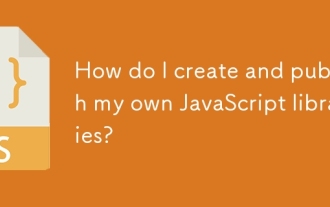
기사는 JavaScript 라이브러리 작성, 게시 및 유지 관리, 계획, 개발, 테스트, 문서 및 홍보 전략에 중점을 둡니다.
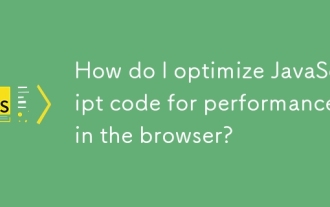
이 기사는 브라우저에서 JavaScript 성능을 최적화하기위한 전략에 대해 설명하고 실행 시간을 줄이고 페이지로드 속도에 미치는 영향을 최소화하는 데 중점을 둡니다.
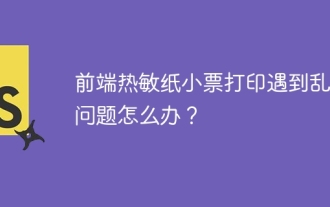
프론트 엔드 개발시 프론트 엔드 열지대 티켓 인쇄를위한 자주 묻는 질문과 솔루션, 티켓 인쇄는 일반적인 요구 사항입니다. 그러나 많은 개발자들이 구현하고 있습니다 ...
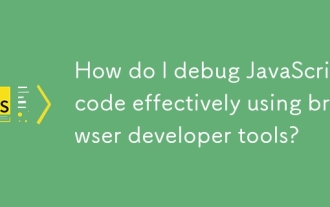
이 기사는 브라우저 개발자 도구를 사용하여 효과적인 JavaScript 디버깅, 중단 점 설정, 콘솔 사용 및 성능 분석에 중점을 둡니다.
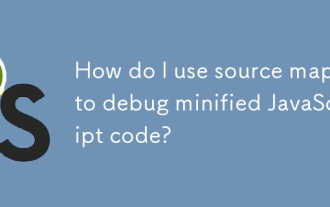
이 기사는 소스 맵을 사용하여 원래 코드에 다시 매핑하여 미니어링 된 JavaScript를 디버그하는 방법을 설명합니다. 소스 맵 활성화, 브레이크 포인트 설정 및 Chrome Devtools 및 Webpack과 같은 도구 사용에 대해 설명합니다.
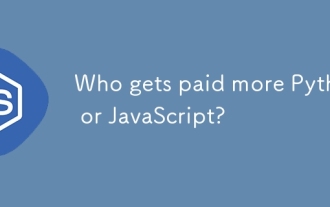
기술 및 산업 요구에 따라 Python 및 JavaScript 개발자에 대한 절대 급여는 없습니다. 1. 파이썬은 데이터 과학 및 기계 학습에서 더 많은 비용을 지불 할 수 있습니다. 2. JavaScript는 프론트 엔드 및 풀 스택 개발에 큰 수요가 있으며 급여도 상당합니다. 3. 영향 요인에는 경험, 지리적 위치, 회사 규모 및 특정 기술이 포함됩니다.

이 튜토리얼은 Chart.js를 사용하여 파이, 링 및 버블 차트를 만드는 방법을 설명합니다. 이전에는 차트 유형의 차트 유형을 배웠습니다. JS : 라인 차트 및 막대 차트 (자습서 2)와 레이더 차트 및 극지 지역 차트 (자습서 3)를 배웠습니다. 파이 및 링 차트를 만듭니다 파이 차트와 링 차트는 다른 부분으로 나뉘어 진 전체의 비율을 보여주는 데 이상적입니다. 예를 들어, 파이 차트는 사파리에서 남성 사자, 여성 사자 및 젊은 사자의 비율 또는 선거에서 다른 후보자가받는 투표율을 보여주는 데 사용될 수 있습니다. 파이 차트는 단일 매개 변수 또는 데이터 세트를 비교하는 데만 적합합니다. 파이 차트의 팬 각도는 데이터 포인트의 숫자 크기에 의존하기 때문에 원형 차트는 값이 0 인 엔티티를 그릴 수 없습니다. 이것은 비율이 0 인 모든 엔티티를 의미합니다
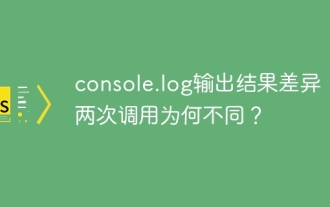
Console.log 출력의 차이의 근본 원인에 대한 심층적 인 논의. 이 기사에서는 Console.log 함수의 출력 결과의 차이점을 코드에서 분석하고 그에 따른 이유를 설명합니다. � ...
