JavaScript 디자인 패턴_javascript 기술의 상태 패턴을 알아보세요.
상태 모델의 핵심은 사물의 내부 상태를 구별하는 것입니다. 사물의 내부 상태 변화는 종종 사물의 행동 변화로 이어집니다.
조명이 켜져 있을 때 스위치를 누르면 조명이 꺼진 상태로 전환되고, 스위치를 다시 누르면 조명이 다시 켜집니다. 동일한 스위치는 상태에 따라 다르게 작동합니다.
1. 유한 상태 기계
- 스테이트(State)의 총 개수는 제한되어 있습니다.
- 어떤 순간에도 당신은 오직 하나의 상태에 있을 뿐입니다.
-
특정 조건에서는 한 상태에서 다른 상태로 전환이 발생합니다.
내부 상태가 변경될 때 개체의 동작을 변경하여 개체가 해당 클래스를 수정하는 것처럼 보이도록 합니다.
설명:
(1) 상태를 독립 클래스로 캡슐화하고 요청을 현재 상태 객체에 위임합니다. 객체의 내부 상태가 변경되면 다른 동작 변경이 발생합니다.
(2) 사용된 객체는 상태에 따라 완전히 다른 동작(위임 효과)을 갖습니다
캡슐화의 경우 일반적으로 객체의 상태보다는 객체의 동작을 캡슐화하는 것이 우선시됩니다.
그러나 상태 패턴에서는 그 반대입니다. 상태 패턴의 핵심은 사물의 각 상태를 별도의 클래스로 캡슐화하는 것입니다.
2. 예시
조명 프로그램(약한 조명 –> 강한 조명 –> 조명 꺼짐) 주기
// 关灯 var OffLightState = function(light) { this.light = light; }; // 弱光 var OffLightState = function(light) { this.light = light; }; // 强光 var StrongLightState = function(light) { this.light = light; }; var Light = function(){ /* 开关状态 */ this.offLight = new OffLightState(this); this.weakLight = new WeakLightState(this); this.strongLight = new StrongLightState(this); /* 快关按钮 */ this.button = null; }; Light.prototype.init = function() { var button = document.createElement("button"), self = this; this.button = document.body.appendChild(button); this.button.innerHTML = '开关'; this.currentState = this.offLight; this.button.click = function() { self.currentState.buttonWasPressed(); } }; // 让抽象父类的抽象方法直接抛出一个异常(避免状态子类未实现buttonWasPressed方法) Light.prototype.buttonWasPressed = function() { throw new Error("父类的buttonWasPressed方法必须被重写"); }; Light.prototype.setState = function(newState) { this.currentState = newState; }; /* 关灯 */ OffLightState.prototype = new Light(); // 继承抽象类 OffLightState.prototype.buttonWasPressed = function() { console.log("关灯!"); this.light.setState(this.light.weakLight); } /* 弱光 */ WeakLightState.prototype = new Light(); WeakLightState.prototype.buttonWasPressed = function() { console.log("弱光!"); this.light.setState(this.light.strongLight); }; /* 强光 */ StrongLightState.prototype = new Light(); StrongLightState.prototype.buttonWasPressed = function() { console.log("强光!"); this.light.setState(this.light.offLight); };
PS: 추가 설명
OffLightState, WeakLightState, StrongLightState 생성자는 고급이어야 합니다.
new A("a"); var A = function(a) { console.log(a) } new B("b"); function B(b) { console.log(b); }
함수 선언은 일반 변수보다 먼저 호이스팅됩니다.
3. 성능 최적화 포인트
(1) 상태 객체의 생성과 소멸을 어떻게 관리하나요?
첫 번째는 상태 객체가 필요할 때만 생성된 다음 소멸됩니다(상태 객체는 상대적으로 크므로 선호됨).
다른 하나는 처음에 모든 상태 객체를 생성하고 절대 파괴하지 않는 것입니다(상태는 자주 변경됩니다).
(2) 플라이웨이트 모드를 사용하여 상태 개체를 공유합니다.
4. 상태 머신의 JavaScript 버전
(1) Function.prototype.call 메소드를 통해 실행을 위해 리터럴 객체에 요청을 직접 위임합니다
// 状态机 var FSM = { off: { buttonWasPressed: function() { console.log("关灯"); this.button.innerHTML = "下一次按我是开灯"; // 这是Light上的属性!!! this.currState = FSM.on; // 这是Light上的属性!!! } }, on: { buttonWasPressed: function() { console.log("开灯"); this.button.innerHTML = "下一次按我是关灯"; this.currState = FSM.off; } }, }; var Light = function() { this.currState = FSM.off; // 设置当前状态 this.button = null; }; Light.prototype.init = function() { var button = document.createElement("button"); self = this; button.innerHTML = "已关灯"; this.button = document.body.appendChild(button); this.button.onclick = function() { // 请求委托给FSM状态机 self.currState.buttonWasPressed.call(self); } } var light = new Light(); light.init();
(2) 위임 기능 사용
var delegate = function(client, delegation) { return { buttonWasPressed: function() { return delegation.buttonWasPressed.apply(client, arguments); } }; }; // 状态机 var FSM = { off: { buttonWasPressed: function() { console.log("关灯"); this.button.innerHTML = "下一次按我是开灯"; this.currState = this.onState; } }, on: { buttonWasPressed: function() { console.log("开灯"); this.button.innerHTML = "下一次按我是关灯"; this.currState = this.offState; } }, }; var Light = function() { this.offState = delegate(this, FSM.off); this.onState = delegate(this, FSM.on); this.currState = this.offState; // 设置当前状态 this.button = null; }; Light.prototype.init = function() { var button = document.createElement("button"); self = this; button.innerHTML = "已关灯"; this.button = document.body.appendChild(button); this.button.onclick = function() { // 请求委托给FSM状态机 self.currState.buttonWasPressed(); } } var light = new Light(); light.init();
이 기사가 JavaScript 프로그래밍을 배우는 모든 사람에게 도움이 되기를 바랍니다.

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










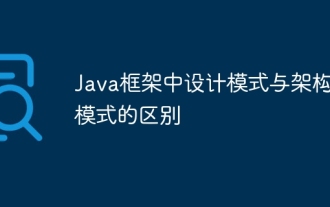
Java 프레임워크에서 디자인 패턴과 아키텍처 패턴의 차이점은 디자인 패턴이 클래스와 객체(예: 팩토리 패턴) 간의 상호 작용에 중점을 두고 소프트웨어 디자인의 일반적인 문제에 대한 추상적인 솔루션을 정의한다는 것입니다. 아키텍처 패턴은 계층화된 아키텍처와 같은 시스템 구성 요소의 구성 및 상호 작용에 중점을 두고 시스템 구조와 모듈 간의 관계를 정의합니다.
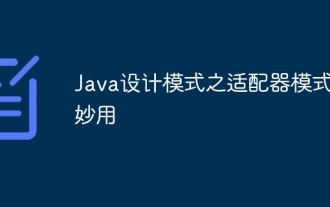
어댑터 패턴은 호환되지 않는 개체가 함께 작동할 수 있도록 하는 구조적 디자인 패턴입니다. 이는 개체가 원활하게 상호 작용할 수 있도록 하나의 인터페이스를 다른 인터페이스로 변환합니다. 개체 어댑터는 적응된 개체를 포함하는 어댑터 개체를 만들고 대상 인터페이스를 구현하여 어댑터 패턴을 구현합니다. 실제적인 경우 클라이언트(예: MediaPlayer)는 어댑터 모드를 통해 고급 형식 미디어(예: VLC)를 재생할 수 있지만 클라이언트 자체는 일반 미디어 형식(예: MP3)만 지원합니다.
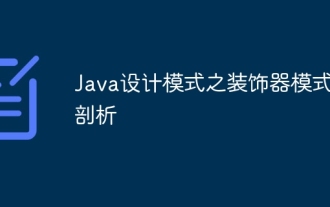
데코레이터 패턴은 원래 클래스를 수정하지 않고도 객체 기능을 동적으로 추가할 수 있는 구조적 디자인 패턴입니다. 추상 컴포넌트, 콘크리트 컴포넌트, 추상 데코레이터, 콘크리트 데코레이터의 협업을 통해 구현되며, 변화하는 요구에 맞게 클래스 기능을 유연하게 확장할 수 있습니다. 이 예에서는 우유와 모카 데코레이터가 총 $2.29의 가격으로 Espresso에 추가되어 객체의 동작을 동적으로 수정하는 데코레이터 패턴의 힘을 보여줍니다.
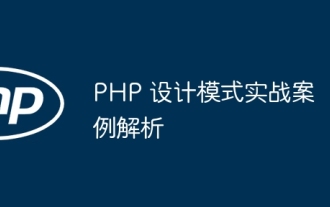
1. 팩토리 패턴: 객체 생성과 비즈니스 로직을 분리하고, 팩토리 클래스를 통해 지정된 형태의 객체를 생성합니다. 2. 관찰자 패턴: 주체 개체가 관찰자 개체에 상태 변경을 알리도록 허용하여 느슨한 결합 및 관찰자 패턴을 달성합니다.
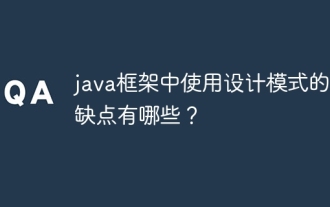
Java 프레임워크에서 디자인 패턴을 사용하면 향상된 코드 가독성, 유지 관리성 및 확장성이 향상된다는 이점이 있습니다. 단점으로는 복잡성, 성능 오버헤드, 과도한 사용으로 인한 가파른 학습 곡선 등이 있습니다. 실제 사례: 프록시 모드는 개체를 지연 로드하는 데 사용됩니다. 디자인 패턴을 현명하게 사용하여 장점을 활용하고 단점을 최소화하세요.
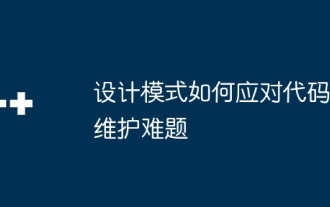
디자인 패턴은 재사용 및 확장 가능한 솔루션을 제공하여 코드 유지 관리 문제를 해결합니다. 관찰자 패턴: 개체가 이벤트를 구독하고 이벤트가 발생할 때 알림을 받을 수 있도록 합니다. 팩토리 패턴: 구체적인 클래스에 의존하지 않고 객체를 생성하는 중앙 집중식 방법을 제공합니다. 싱글톤 패턴: 클래스에 전역적으로 액세스 가능한 개체를 만드는 데 사용되는 인스턴스가 하나만 있는지 확인합니다.
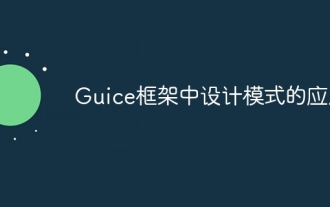
Guice 프레임워크는 다음을 포함한 다양한 디자인 패턴을 적용합니다. 싱글톤 패턴: @Singleton 주석을 통해 클래스에 인스턴스가 하나만 있는지 확인합니다. 팩토리 메소드 패턴: @Provides 주석을 통해 팩토리 메소드를 생성하고 종속성 주입 중에 객체 인스턴스를 얻습니다. 전략 모드: 알고리즘을 다양한 전략 클래스로 캡슐화하고 @Named 주석을 통해 특정 전략을 지정합니다.
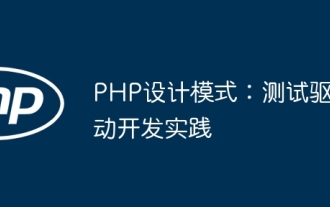
TDD는 고품질 PHP 코드를 작성하는 데 사용됩니다. 단계에는 테스트 사례 작성, 예상 기능 설명 및 실패 만들기가 포함됩니다. 과도한 최적화나 세부 설계 없이 테스트 케이스만 통과하도록 코드를 작성합니다. 테스트 케이스를 통과한 후 코드를 최적화하고 리팩터링하여 가독성, 유지 관리성 및 확장성을 향상시킵니다.
