스레드 풀 구현
1. 스레드의 세 가지 주요 동기화 메커니즘
2. 뮤텍스 잠금
2. 구현 세 가지 동기화 메커니즘 각각에 대한 래퍼 클래스
3. 스레드 풀 구현스레드 풀이 있는 경우 동적으로 스레드를 생성하는 데는 시간이 많이 걸립니다. 사용자 요청이 오면 스레드 풀에서 유휴 스레드를 가져와서 사용자의 요청을 처리합니다. 다시 유휴 상태가 되어 다음 사용 시간을 기다립니다.
#ifdef LOCKER_H #define LOCKER_H #include <pthread.h> #include <semaphore.h> /*信号量的封装*/ class sem { public: sem() { if( sem_init( &sem_like, 0, 0)) { throw std::exception(); } } ~sem() { sem_destroy( &sem_like); } bool wait() { return sem_wait( &sem_like)== 0; } bool post() { return sem_post( &sem_like)== 0; } private: sem_t sem_like; } /*互斥锁的封装*/ class locker { public: locker() { if( pthread_mutex_init( &mutex_like,NULL) !=0) { throw std::exception(); } } ~locker() { pthread_mutex_destroy( &mutex_like); } bool lock() { return pthread_mutex_lock( &mutex_like)== 0; } bool unlock() { return pthread_mutex_unlock( &mutex_like); } private: pthread_mutex_t mutex_like; } /*条件变量的封装*/ class cond { public: cond() { if( pthread_mutex_init( &mutex_like,NULL)!= 0) { throw std::exception; } if( pthread_cond_init( &cond_like, NULL)!= 0) { //释放对应的互斥锁 pthread_mutex_destroy( &mutex_like); throw std::exception; } } ~cond() { pthread_mutex_destroy( &mutex_like); pthread_cond_destroy( &cond_like); } bool wait() { int flag= 0; pthread_mutex_lock( &mutex_like); flag= pthread_cond_wait( &cond_like, &mutex_like); pthread_mutex_unlock( &mutex_like); return flag== 0; } bool signal() { return pthread_cond_signal( &cond_like)== 0; } private: pthread_mutex_t mutex_like; pthread_cond_t cond_like; } #endif
두 가지 핵심 데이터 구조가 있습니다: 스레드 컨테이너와 요청 큐
벡터가 사용됩니다. here 스레드 풀의 모든 스레드 ID를 저장하는 컨테이너
여기서 목록 컨테이너는 모든 요청을 저장하는 데 사용되며 요청 처리는 FIFO 순서입니다.
#ifndef THREADPOOL_H #define THREADPOOL_H #include <list> #include <cstdio> #include <exception> #include <pthread.h> #include "locker.h" template< typename T > class threadpool { public: threadpool( int thread_number = 8, int max_requests = 10000 ); ~threadpool(); bool append( T* request ); private: static void* worker( void* arg ); void run(); private: int thread_number_like;//当前线程池中的线程个数 int max_requests_like;//最大请求数 //pthread_t* threads_like; vector< pthread> threads_like;//线程容器 std::list< T* > workqueue_like;//请求队列 locker queuelocker_like;//请求队列的访问互斥锁 sem queuestat_like;//用于请求队列与空闲线程同步的信号量 bool stop_like;//结束所有线程,线程池此时没有线程 }; template< typename T > threadpool< T >::threadpool( int thread_number, int max_requests ) : m_thread_number( thread_number ), m_max_requests( max_requests ), m_stop( false ), m_threads( NULL ) { if( ( thread_number <= 0 ) || ( max_requests <= 0 ) ) { throw std::exception(); } threads_like.resize( thread_number_like); if( thread_number_like!= threads_like.size() ) { throw std::exception(); } for ( int i = 0; i < thread_number_like; ++i ) { printf( "create the %dth thread\n", i ); if( pthread_create( &threads_like [i], NULL, worker, this ) != 0 )//创建线程 { threads_like.resize(0); throw std::exception(); } if( pthread_detach( m_threads[i] ) )//设置为脱离线程 { threads_like.resize(0); throw std::exception(); } } } template< typename T > threadpool< T >::~threadpool() { stop_like = true; } template< typename T > bool threadpool< T >::append( T* request ) { queuelocker_like.lock(); if ( workqueue_like.size() > max_requests_like ) { queuelocker_like.unlock(); return false; } workqueue_like.push_back( request ); queuelocker_like.unlock(); queuestat_like.post(); return true; } template< typename T > void* threadpool< T >::worker( void* arg ) { threadpool* pool = ( threadpool* )arg;//静态函数要调用动态成员run,必须通过参数arg得到 pool->run();//线程的执行体 return pool; } template< typename T > void threadpool< T >::run() { while ( ! m_stop ) { queuestat_like.wait(); queuelocker_like.lock(); if ( workqueue_like.empty() ) { queuelocker_like.unlock(); continue; } T* request = workqueue_like.front(); workqueue_like.pop_front(); queuelocker_like.unlock(); if ( ! request ) { continue; } request->process();//执行当前请求所对应的处理函数 } } #endif
3. 이 방법은 다중 사용자 요청에 응답하는 nginx와 유사한 프로세스가 더 유리합니다. 1. 프로세스 수가 고정되어 있습니다. 동시에 많은 스레드나 프로세스가 있기 때문에 너무 많은 메모리를 차지하지 않습니다. 2: nginx 작업 프로세스 수는 일반적으로 CPU 코어 수와 일치하며 프로세스를 코어에 바인딩할 수 있으므로 프로세스 전환이나 스레드 전환으로 인한 시스템 오버헤드가 절약됩니다

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










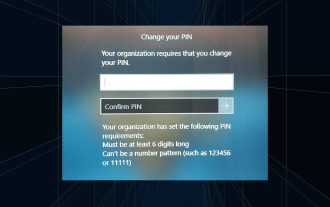
로그인 화면에 "귀하의 조직에서 PIN 변경을 요구합니다"라는 메시지가 나타납니다. 이는 개인 장치를 제어할 수 있는 조직 기반 계정 설정을 사용하는 컴퓨터에서 PIN 만료 제한에 도달한 경우 발생합니다. 그러나 개인 계정을 사용하여 Windows를 설정하는 경우 이상적으로는 오류 메시지가 나타나지 않습니다. 항상 그런 것은 아니지만. 오류가 발생한 대부분의 사용자는 개인 계정을 사용하여 신고합니다. 조직에서 Windows 11에서 PIN을 변경하도록 요청하는 이유는 무엇입니까? 귀하의 계정이 조직과 연결되어 있을 수 있으므로 이를 확인하는 것이 기본 접근 방식입니다. 도메인 관리자에게 문의하면 도움이 될 수 있습니다! 또한 잘못 구성된 로컬 정책 설정이나 잘못된 레지스트리 키로 인해 오류가 발생할 수 있습니다. 지금 바로
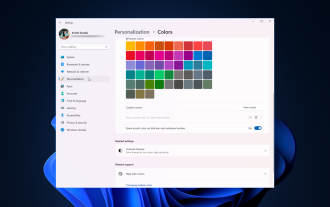
Windows 11은 신선하고 우아한 디자인을 전면에 내세웠습니다. 현대적인 인터페이스를 통해 창 테두리와 같은 미세한 세부 사항을 개인화하고 변경할 수 있습니다. 이 가이드에서는 Windows 운영 체제에서 자신의 스타일을 반영하는 환경을 만드는 데 도움이 되는 단계별 지침을 설명합니다. 창 테두리 설정을 변경하는 방법은 무엇입니까? +를 눌러 설정 앱을 엽니다. Windows개인 설정으로 이동하여 색상 설정을 클릭합니다. 색상 변경 창 테두리 설정 창 11" Width="643" Height="500" > 제목 표시줄 및 창 테두리에 강조 색상 표시 옵션을 찾아 옆에 있는 스위치를 토글합니다. 시작 메뉴 및 작업 표시줄에 강조 색상을 표시하려면 시작 메뉴와 작업 표시줄에 테마 색상을 표시하려면 시작 메뉴와 작업 표시줄에 테마 표시를 켭니다.
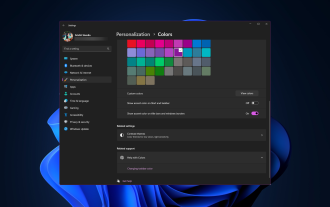
기본적으로 Windows 11의 제목 표시줄 색상은 선택한 어두운/밝은 테마에 따라 다릅니다. 그러나 원하는 색상으로 변경할 수 있습니다. 이 가이드에서는 이를 변경하고 데스크톱 환경을 개인화하여 시각적으로 매력적으로 만드는 세 가지 방법에 대한 단계별 지침을 논의합니다. 활성 창과 비활성 창의 제목 표시줄 색상을 변경할 수 있습니까? 예, 설정 앱을 사용하여 활성 창의 제목 표시줄 색상을 변경하거나 레지스트리 편집기를 사용하여 비활성 창의 제목 표시줄 색상을 변경할 수 있습니다. 이러한 단계를 알아보려면 다음 섹션으로 이동하세요. Windows 11에서 제목 표시줄 색상을 변경하는 방법은 무엇입니까? 1. 설정 앱을 사용하여 +를 눌러 설정 창을 엽니다. Windows"개인 설정"으로 이동한 다음
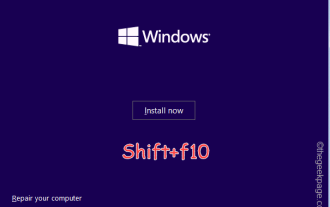
Windows Installer 페이지에 "OOBELANGUAGE" 문과 함께 "문제가 발생했습니다."가 표시됩니까? 이러한 오류로 인해 Windows 설치가 중단되는 경우가 있습니다. OOBE는 즉시 사용 가능한 경험을 의미합니다. 오류 메시지에서 알 수 있듯이 이는 OOBE 언어 선택과 관련된 문제입니다. 걱정할 필요가 없습니다. OOBE 화면 자체에서 레지스트리를 편집하면 이 문제를 해결할 수 있습니다. 빠른 수정 – 1. OOBE 앱 하단에 있는 “다시 시도” 버튼을 클릭하세요. 그러면 더 이상의 문제 없이 프로세스가 계속됩니다. 2. 전원 버튼을 사용하여 시스템을 강제 종료합니다. 시스템이 다시 시작된 후 OOBE가 계속되어야 합니다. 3. 인터넷에서 시스템 연결을 끊습니다. 오프라인 모드에서 OOBE의 모든 측면을 완료하세요.
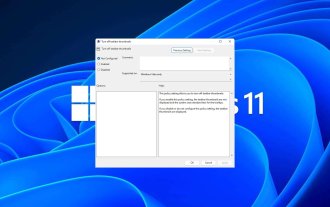
작업 표시줄 축소판은 재미있을 수도 있지만 주의를 산만하게 하거나 짜증나게 할 수도 있습니다. 이 영역 위로 얼마나 자주 마우스를 가져가는지 고려하면 실수로 중요한 창을 몇 번 닫았을 수도 있습니다. 또 다른 단점은 더 많은 시스템 리소스를 사용한다는 것입니다. 따라서 리소스 효율성을 높일 수 있는 방법을 찾고 있다면 비활성화하는 방법을 알려드리겠습니다. 그러나 하드웨어 사양이 이를 처리할 수 있고 미리 보기가 마음에 들면 활성화할 수 있습니다. Windows 11에서 작업 표시줄 축소판 미리 보기를 활성화하는 방법은 무엇입니까? 1. 설정 앱을 사용하여 키를 탭하고 설정을 클릭합니다. Windows에서는 시스템을 클릭하고 정보를 선택합니다. 고급 시스템 설정을 클릭합니다. 고급 탭으로 이동하여 성능 아래에서 설정을 선택합니다. "시각 효과"를 선택하세요.
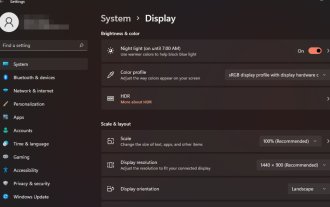
Windows 11의 디스플레이 크기 조정과 관련하여 우리 모두는 서로 다른 선호도를 가지고 있습니다. 큰 아이콘을 좋아하는 사람도 있고, 작은 아이콘을 좋아하는 사람도 있습니다. 그러나 올바른 크기 조정이 중요하다는 점에는 모두가 동의합니다. 잘못된 글꼴 크기 조정이나 이미지의 과도한 크기 조정은 작업 시 생산성을 저하시킬 수 있으므로 시스템 기능을 최대한 활용하려면 이를 사용자 정의하는 방법을 알아야 합니다. Custom Zoom의 장점: 화면의 텍스트를 읽기 어려운 사람들에게 유용한 기능입니다. 한 번에 화면에서 더 많은 것을 볼 수 있도록 도와줍니다. 특정 모니터 및 응용 프로그램에만 적용되는 사용자 정의 확장 프로필을 생성할 수 있습니다. 저사양 하드웨어의 성능을 향상시키는 데 도움이 될 수 있습니다. 이를 통해 화면의 내용을 더 효과적으로 제어할 수 있습니다. 윈도우 11을 사용하는 방법
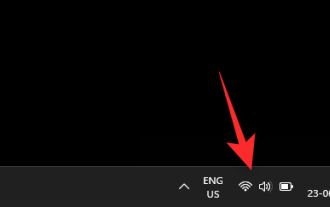
화면 밝기는 최신 컴퓨팅 장치를 사용할 때 필수적인 부분이며, 특히 화면을 장시간 볼 때 더욱 그렇습니다. 눈의 피로를 줄이고, 가독성을 높이며, 콘텐츠를 쉽고 효율적으로 보는 데 도움이 됩니다. 그러나 설정에 따라 밝기 관리가 어려울 수 있으며, 특히 새로운 UI 변경이 적용된 Windows 11에서는 더욱 그렇습니다. 밝기를 조정하는 데 문제가 있는 경우 Windows 11에서 밝기를 관리하는 모든 방법은 다음과 같습니다. Windows 11에서 밝기를 변경하는 방법 [10가지 설명] 단일 모니터 사용자는 다음 방법을 사용하여 Windows 11에서 밝기를 조정할 수 있습니다. 여기에는 단일 모니터를 사용하는 데스크탑 시스템과 노트북이 포함됩니다. 시작하자. 방법 1: 알림 센터 사용 알림 센터에 액세스할 수 있습니다.
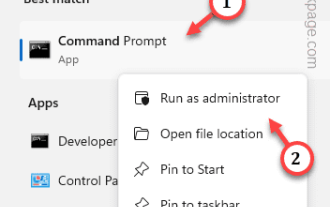
Windows의 정품 인증 프로세스에서 갑자기 이 오류 코드 0xc004f069가 포함된 오류 메시지가 표시되는 경우가 있습니다. 활성화 프로세스가 온라인으로 진행되더라도 Windows Server를 실행하는 일부 이전 시스템에서 이 문제가 발생할 수 있습니다. 이러한 초기 점검을 수행하고 시스템 활성화에 도움이 되지 않으면 기본 해결 방법으로 이동하여 문제를 해결하십시오. 해결 방법 - 오류 메시지와 활성화 창을 닫습니다. 그런 다음 컴퓨터를 다시 시작하십시오. Windows 정품 인증 프로세스를 처음부터 다시 시도하세요. 수정 1 – 터미널에서 활성화 cmd 터미널에서 Windows Server Edition 시스템을 활성화합니다. 1단계 – Windows Server 버전 확인 현재 사용하고 있는 W 종류를 확인해야 합니다.
