Python으로 작성된 클라이언트는 해당 명령을 실행하고 결과를 반환하라는 명령을 서버에 보냅니다.
아무것도 없이 Python을 사용하여 클라이언트와 서버 응답을 위한 프로그램을 작성합니다. 주요 원칙은 클라이언트가 tcp 프로토콜을 통해 서버와 통신하고, 서버가 이를 실행하는 것입니다. 지시사항을 전달하고 해당 결과를 클라이언트로 보내면 클라이언트가 결과를 인쇄합니다. 코드는 비교적 간단하므로 자세히 소개하지 않습니다. 단지 오락을 위해서입니다.
서버측 코드, server_tcp.py
#!/usr/bin/env python # -*- coding:utf-8 -*- # #执行客户端发送过来的命令,并把执行结果返回给客户端 import socket, traceback, subprocess host = '' port = 51888 s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1) s.bind((host, port)) s.listen(1) while 1: try: client_socket, client_addr = s.accept() except Exception, e: traceback.print_exc() continue try: print 'From host:', client_socket.getpeername() while 1: command = client_socket.recv(4096) if not len(command): break print client_socket.getpeername()[0] + ':' + str(command) # 执行客户端传递过来的命令 handler = subprocess.Popen(command, shell=True, stdout=subprocess.PIPE) output = handler.stdout.readlines() if output is None: output = [] for one_line in output: client_socket.sendall(one_line) client_socket.sendall("\n") client_socket.sendall("ok") except Exception, e: traceback.print_exc() try: client_socket.close() except Exception, e: traceback.print_exc()
2. 클라이언트측 코드 client_tcp.py
#!/usr/bin/env python # -*- coding:utf-8 -*- # #给server端发送命令 import socket, sys, traceback host = '127.0.0.1' port = 51888 s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) try: s.connect((host, port)) except Exception, e: msg = traceback.format_exc() print '连接错误:', msg input_command = raw_input('Input command:') s.send(input_command) # 利用shutdown()函数使socket双向数据传输变为单向数据传输 # 该参数表示了如何关闭socket。具体为:0表示禁止将来读;1表示禁止将来写;2表示禁止将来读和写 s.shutdown(1) print '发送完成.' print '收到内容:\n' while 1: buff = s.recv(4096) if not len(buff): break sys.stdout.write(buff)
3. server_tcp.py 스크립트를 시작하고 로컬 포트 51888을 수신한 다음 client_tcp.py를 시작합니다.
(1) 클라이언트 콘텐츠:
/usr/bin/python2.7 /home/wuguowei/PycharmProjects/xplan_script/test_process/client_tcp.py Input command:ls -l 发送完成. 收到内容: 总用量 20 -rw-r--r-- 1 root root 744 2月 10 14:44 client_tcp.py -rw-r--r-- 1 root root 877 2月 10 14:18 my_sub_process.py -rw-r--r-- 1 root root 1290 2月 10 14:45 server_tcp.py -rw-r--r-- 1 root root 493 2月 10 10:43 tcpclient.py -rw-r--r-- 1 root root 1168 2月 10 11:51 tcpserver.py ok Process finished with exit code 0
(2) 서버 정보
/usr/bin/python2.7 /home/wuguowei/PycharmProjects/xplan_script/test_process/server_tcp.py From host: ('127.0.0.1', 46993) 127.0.0.1:ls -l

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










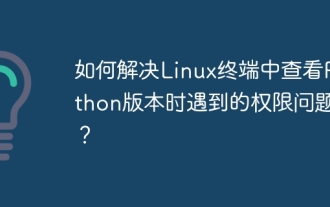
Linux 터미널에서 Python 버전을 보려고 할 때 Linux 터미널에서 Python 버전을 볼 때 권한 문제에 대한 솔루션 ... Python을 입력하십시오 ...
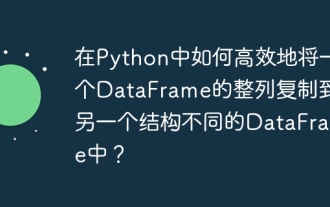
Python의 Pandas 라이브러리를 사용할 때는 구조가 다른 두 데이터 프레임 사이에서 전체 열을 복사하는 방법이 일반적인 문제입니다. 두 개의 dats가 있다고 가정 해
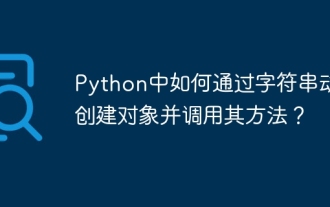
파이썬에서 문자열을 통해 객체를 동적으로 생성하고 메소드를 호출하는 방법은 무엇입니까? 특히 구성 또는 실행 해야하는 경우 일반적인 프로그래밍 요구 사항입니다.
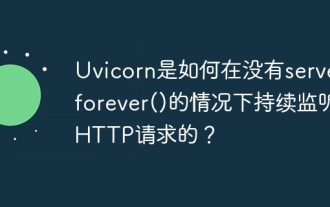
Uvicorn은 HTTP 요청을 어떻게 지속적으로 듣습니까? Uvicorn은 ASGI를 기반으로 한 가벼운 웹 서버입니다. 핵심 기능 중 하나는 HTTP 요청을 듣고 진행하는 것입니다 ...
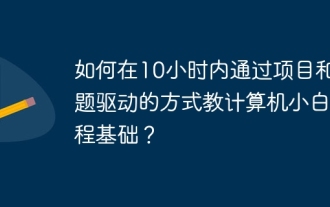
10 시간 이내에 컴퓨터 초보자 프로그래밍 기본 사항을 가르치는 방법은 무엇입니까? 컴퓨터 초보자에게 프로그래밍 지식을 가르치는 데 10 시간 밖에 걸리지 않는다면 무엇을 가르치기로 선택 하시겠습니까?
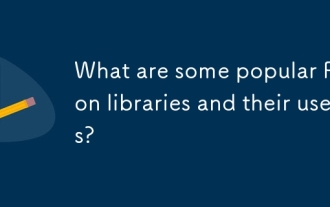
이 기사는 Numpy, Pandas, Matplotlib, Scikit-Learn, Tensorflow, Django, Flask 및 요청과 같은 인기있는 Python 라이브러리에 대해 설명하고 과학 컴퓨팅, 데이터 분석, 시각화, 기계 학습, 웹 개발 및 H에서의 사용에 대해 자세히 설명합니다.
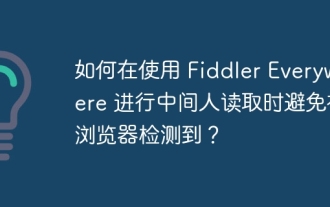
Fiddlerevery Where를 사용할 때 Man-in-the-Middle Reading에 Fiddlereverywhere를 사용할 때 감지되는 방법 ...
