PHP로 구현된 Curl 캡슐화 클래스 Curl.class.php의 사용예 분석
이 기사의 예에서는 PHP에서 구현된 Curl 캡슐화 클래스 Curl.class.php의 사용법을 설명합니다. 참고할 수 있도록 모든 사람과 공유하세요. 자세한 내용은 다음과 같습니다.
<?php //curl类 class Curl { function Curl(){ return true; } function execute($method, $url, $fields='', $userAgent='', $httpHeaders='', $username='', $password=''){ $ch = Curl::create(); if(false === $ch){ return false; } if(is_string($url) && strlen($url)){ $ret = curl_setopt($ch, CURLOPT_URL, $url); }else{ return false; } //是否显示头部信息 curl_setopt($ch, CURLOPT_HEADER, false); // curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); if($username != ''){ curl_setopt($ch, CURLOPT_USERPWD, $username . ':' . $password); } $method = strtolower($method); if('post' == $method){ curl_setopt($ch, CURLOPT_POST, true); if(is_array($fields)){ $sets = array(); foreach ($fields AS $key => $val){ $sets[] = $key . '=' . urlencode($val); } $fields = implode('&',$sets); } curl_setopt($ch, CURLOPT_POSTFIELDS, $fields); }else if('put' == $method){ curl_setopt($ch, CURLOPT_PUT, true); } //curl_setopt($ch, CURLOPT_PROGRESS, true); //curl_setopt($ch, CURLOPT_VERBOSE, true); //curl_setopt($ch, CURLOPT_MUTE, false); curl_setopt($ch, CURLOPT_TIMEOUT, 10);//设置curl超时秒数 if(strlen($userAgent)){ curl_setopt($ch, CURLOPT_USERAGENT, $userAgent); } if(is_array($httpHeaders)){ curl_setopt($ch, CURLOPT_HTTPHEADER, $httpHeaders); } $ret = curl_exec($ch); if(curl_errno($ch)){ curl_close($ch); return array(curl_error($ch), curl_errno($ch)); }else{ curl_close($ch); if(!is_string($ret) || !strlen($ret)){ return false; } return $ret; } } function post($url, $fields, $userAgent = '', $httpHeaders = '', $username = '', $password = ''){ $ret = Curl::execute('POST', $url, $fields, $userAgent, $httpHeaders, $username, $password); if(false === $ret){ return false; } if(is_array($ret)){ return false; } return $ret; } function get($url, $userAgent = '', $httpHeaders = '', $username = '', $password = ''){ $ret = Curl::execute('GET', $url, '', $userAgent, $httpHeaders, $username, $password); if(false === $ret){ return false; } if(is_array($ret)){ return false; } return $ret; } function create(){ $ch = null; if(!function_exists('curl_init')){ return false; } $ch = curl_init(); if(!is_resource($ch)){ return false; } return $ch; } } ?>
GET 사용법:
$curl = new Curl(); $curl->get('http://www.XXX.com/');
POST 사용법:
$curl = new Curl(); $curl->get('http://www.XXX.com/', 'p=1&time=0');
이 글이 모든 분들의 PHP 프로그래밍에 도움이 되기를 바랍니다. 설계.
PHP에서 구현한 Curl 캡슐화 클래스 Curl.class.php의 사용예 분석에 대한 자세한 관련 글은 PHP 중국어 홈페이지를 주목해주세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
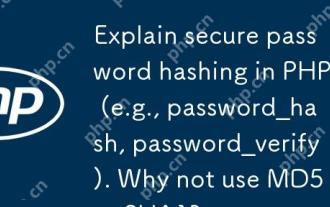
PHP에서 Password_hash 및 Password_Verify 기능을 사용하여 보안 비밀번호 해싱을 구현해야하며 MD5 또는 SHA1을 사용해서는 안됩니다. 1) Password_hash는 보안을 향상시키기 위해 소금 값이 포함 된 해시를 생성합니다. 2) Password_verify 암호를 확인하고 해시 값을 비교하여 보안을 보장합니다. 3) MD5 및 SHA1은 취약하고 소금 값이 부족하며 현대 암호 보안에는 적합하지 않습니다.
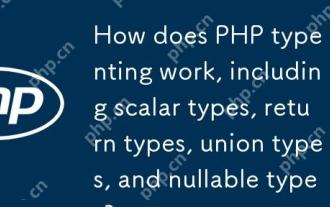
PHP 유형은 코드 품질과 가독성을 향상시키기위한 프롬프트입니다. 1) 스칼라 유형 팁 : PHP7.0이므로 int, float 등과 같은 기능 매개 변수에 기본 데이터 유형을 지정할 수 있습니다. 2) 반환 유형 프롬프트 : 기능 반환 값 유형의 일관성을 확인하십시오. 3) Union 유형 프롬프트 : PHP8.0이므로 기능 매개 변수 또는 반환 값에 여러 유형을 지정할 수 있습니다. 4) Nullable 유형 프롬프트 : NULL 값을 포함하고 널 값을 반환 할 수있는 기능을 포함 할 수 있습니다.
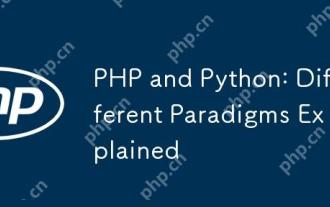
PHP는 주로 절차 적 프로그래밍이지만 객체 지향 프로그래밍 (OOP)도 지원합니다. Python은 OOP, 기능 및 절차 프로그래밍을 포함한 다양한 패러다임을 지원합니다. PHP는 웹 개발에 적합하며 Python은 데이터 분석 및 기계 학습과 같은 다양한 응용 프로그램에 적합합니다.
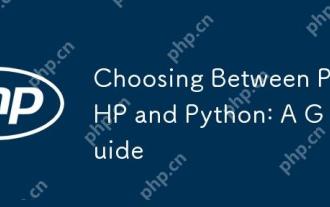
PHP는 웹 개발 및 빠른 프로토 타이핑에 적합하며 Python은 데이터 과학 및 기계 학습에 적합합니다. 1.PHP는 간단한 구문과 함께 동적 웹 개발에 사용되며 빠른 개발에 적합합니다. 2. Python은 간결한 구문을 가지고 있으며 여러 분야에 적합하며 강력한 라이브러리 생태계가 있습니다.
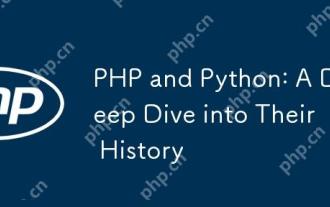
PHP는 1994 년에 시작되었으며 Rasmuslerdorf에 의해 개발되었습니다. 원래 웹 사이트 방문자를 추적하는 데 사용되었으며 점차 서버 측 스크립팅 언어로 진화했으며 웹 개발에 널리 사용되었습니다. Python은 1980 년대 후반 Guidovan Rossum에 의해 개발되었으며 1991 년에 처음 출시되었습니다. 코드 가독성과 단순성을 강조하며 과학 컴퓨팅, 데이터 분석 및 기타 분야에 적합합니다.
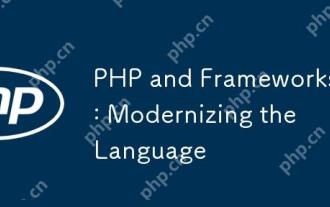
PHP는 현대화 프로세스에서 많은 웹 사이트 및 응용 프로그램을 지원하고 프레임 워크를 통해 개발 요구에 적응하기 때문에 여전히 중요합니다. 1.PHP7은 성능을 향상시키고 새로운 기능을 소개합니다. 2. Laravel, Symfony 및 Codeigniter와 같은 현대 프레임 워크는 개발을 단순화하고 코드 품질을 향상시킵니다. 3. 성능 최적화 및 모범 사례는 응용 프로그램 효율성을 더욱 향상시킵니다.
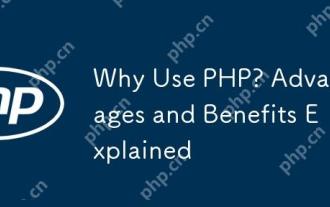
PHP의 핵심 이점에는 학습 용이성, 강력한 웹 개발 지원, 풍부한 라이브러리 및 프레임 워크, 고성능 및 확장 성, 크로스 플랫폼 호환성 및 비용 효율성이 포함됩니다. 1) 배우고 사용하기 쉽고 초보자에게 적합합니다. 2) 웹 서버와 우수한 통합 및 여러 데이터베이스를 지원합니다. 3) Laravel과 같은 강력한 프레임 워크가 있습니다. 4) 최적화를 통해 고성능을 달성 할 수 있습니다. 5) 여러 운영 체제 지원; 6) 개발 비용을 줄이기위한 오픈 소스.
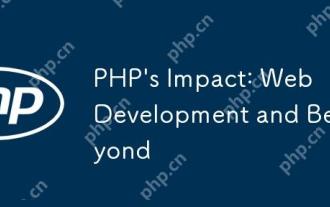
phphassignificallyimpactedwebdevelopmentandextendsbeyondit
