JS 정규식 수정자 global(/g) 사용 분석
이 기사의 예에서는 JS 정규식 수정자 전역(/g)의 사용법을 설명합니다. 참고용으로 모든 사람과 공유하세요. 세부 사항은 다음과 같습니다.
/g 수정자는 전역 일치를 나타내며 첫 번째 일치를 찾은 후 중지하는 대신 모든 일치를 검색합니다. 먼저 고전적인 코드를 살펴보겠습니다.
var str = "123#abc"; var noglobal = /abc/i;//非全局匹配模式 console.log(re.test(str)); //输出ture console.log(re.test(str)); //输出ture console.log(re.test(str)); //输出ture console.log(re.test(str)); //输出ture var re = /abc/ig;//全局匹配 console.log(re.test(str)); //输出ture console.log(re.test(str)); //输出false console.log(re.test(str)); //输出ture console.log(re.test(str)); //输出false
다음을 볼 수 있습니다. /g 모드를 사용할 때 RegExp.test의 출력 결과 ()를 여러 번 실행하면 차이가 있습니다.
정규식 개체를 생성할 때 "g" 식별자가 사용되거나 해당 ?global 속성 값이 true로 설정된 경우 새로 생성된 정규식 개체는 패턴을 사용하여 문자열을 전역적으로 일치시킵니다. 전역 일치 모드에서는 지정된 문자열에 대해 여러 일치 항목을 수행하여 찾을 수 있습니다. 각 일치 항목은 현재 일반 개체의 lastIndex 속성 값을 대상 문자열에서 검색을 시작하는 시작 위치로 사용합니다. lastIndex 속성의 초기값은 0이다. 일치하는 항목을 찾은 후 lastIndex 값은 문자열에서 일치하는 내용의 다음 문자의 위치 인덱스로 재설정되어 검색을 시작할 위치를 식별하는 데 사용됩니다. 다음 경기가 열릴 때. 일치하는 항목이 없으면 lastIndex 값이 0으로 설정됩니다. 일반 객체의 전역 일치 플래그가 설정되지 않은 경우 lastIndex 속성의 값은 항상 0이며, 일치가 수행될 때마다 문자열에서 첫 번째로 일치하는 항목만 찾습니다.
다음 코드를 통해 /g 모드에서 lastIndex의 성능을 확인할 수 있습니다.
var str = "012345678901234567890123456789"; var re = /123/g; console.log(re.lastIndex); //输出0,正则表达式刚开始创建 console.log(re.test(str)); //输出ture console.log(re.lastIndex); //输出4 console.log(re.test(str)); //输出true console.log(re.lastIndex); //输出14 console.log(re.test(str)); //输出ture console.log(re.lastIndex); //输出24 console.log(re.test(str)); //输出false console.log(re.lastIndex); //输出0,没有找到匹配项被重置
위에서 RegExp.test() 함수에 대한 /g 모드의 영향을 확인했습니다. 이제 RegExp.exec() 함수에 미치는 영향을 살펴보겠습니다. RegExp.exec()는 일치하는 결과가 포함된 배열을 반환합니다. 일치하는 항목이 없으면 반환 값은 null입니다.
var str = "012345678901234567890123456789"; var re = /abc/; //exec没有找到匹配 console.log(re.exec(str));//null console.log(re.lastIndex);//0
var str = "012345678901234567890123456789"; var re = /123/; var resultArray = re.exec(str); console.log(resultArray[0]);//匹配结果123 console.log(resultArray.input);//目标字符串str console.log(resultArray.index);//匹配结果在原始字符串str中的索引
RegExp.exec 메서드의 경우 g를 추가하지 않으면 횟수에 관계없이 첫 번째 일치 항목만 반환됩니다. g가 추가되면 첫 번째 실행도 첫 번째 일치 항목을 반환하고 두 번째 실행에서는 두 번째 일치 항목을 반환하는 식으로 진행됩니다.
var str = "012345678901234567890123456789"; var re = /123/; var globalre = /123/g; //非全局匹配 var resultArray = re.exec(str); console.log(resultArray[0]);//输出123 console.log(resultArray.index);//输出1 console.log(globalre.lastIndex);//输出0 var resultArray = re.exec(str); console.log(resultArray[0]);//输出123 console.log(resultArray.index);//输出1 console.log(globalre.lastIndex);//输出0 //全局匹配 var resultArray = globalre.exec(str); console.log(resultArray[0]);//输出123 console.log(resultArray.index);//输出1 console.log(globalre.lastIndex);//输出4 var resultArray = globalre.exec(str); console.log(resultArray[0]);//输出123 console.log(resultArray.index);//输出11 console.log(globalre.lastIndex);//输出14
/g 일치 모드를 사용하면 루프를 통해 모든 일치 항목을 얻을 수 있습니다.
var str = "012345678901234567890123456789"; var globalre = /123/g; //循环遍历,获取所有匹配 var result = null; while ((result = globalre.exec(str)) != null) { console.log(result[0]); console.log(globalre.lastIndex); }
이 글이 JavaScript 프로그래밍에 종사하는 모든 분들께 도움이 되기를 바랍니다.
JS 정규식 수정자 global(/g) 사용 분석에 관한 더 많은 기사를 보려면 PHP 중국어 사이트를 주목하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










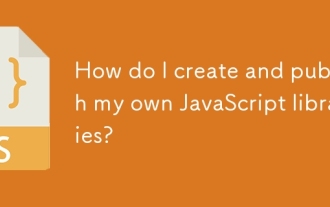
기사는 JavaScript 라이브러리 작성, 게시 및 유지 관리, 계획, 개발, 테스트, 문서 및 홍보 전략에 중점을 둡니다.
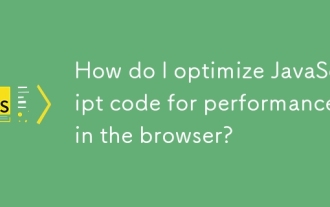
이 기사는 브라우저에서 JavaScript 성능을 최적화하기위한 전략에 대해 설명하고 실행 시간을 줄이고 페이지로드 속도에 미치는 영향을 최소화하는 데 중점을 둡니다.
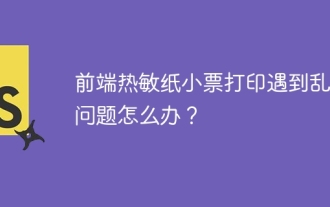
프론트 엔드 개발시 프론트 엔드 열지대 티켓 인쇄를위한 자주 묻는 질문과 솔루션, 티켓 인쇄는 일반적인 요구 사항입니다. 그러나 많은 개발자들이 구현하고 있습니다 ...
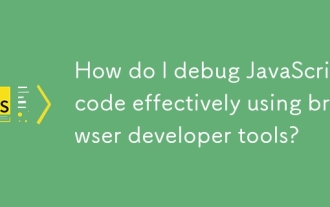
이 기사는 브라우저 개발자 도구를 사용하여 효과적인 JavaScript 디버깅, 중단 점 설정, 콘솔 사용 및 성능 분석에 중점을 둡니다.
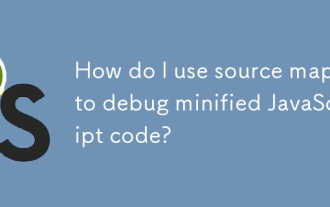
이 기사는 소스 맵을 사용하여 원래 코드에 다시 매핑하여 미니어링 된 JavaScript를 디버그하는 방법을 설명합니다. 소스 맵 활성화, 브레이크 포인트 설정 및 Chrome Devtools 및 Webpack과 같은 도구 사용에 대해 설명합니다.
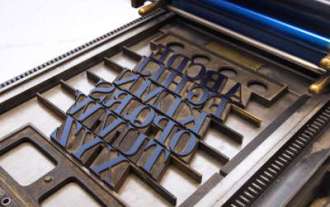
엔트리 레벨 타입 스크립트 자습서를 마스터 한 후에는 TypeScript를 지원하고 JavaScript로 컴파일하는 IDE에서 자신의 코드를 작성할 수 있어야합니다. 이 튜토리얼은 TypeScript의 다양한 데이터 유형으로 뛰어납니다. JavaScript에는 NULL, UNDEFINED, BOOLEAN, 번호, 문자열, 기호 (ES6에 의해 소개 됨) 및 객체의 7 가지 데이터 유형이 있습니다. TypeScript는이 기반으로 더 많은 유형을 정의 하며이 튜토리얼은이 모든 튜토리얼을 자세히 다룹니다. 널 데이터 유형 JavaScript와 마찬가지로 Null in TypeScript
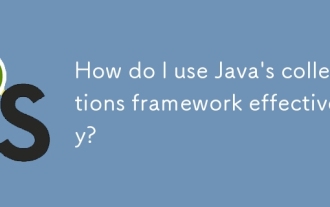
이 기사는 Java의 컬렉션 프레임 워크의 효과적인 사용을 탐구합니다. 데이터 구조, 성능 요구 및 스레드 안전을 기반으로 적절한 컬렉션 (목록, 세트, 맵, 큐)을 선택하는 것을 강조합니다. 효율적인 수집 사용을 최적화합니다

이 튜토리얼은 Chart.js를 사용하여 파이, 링 및 버블 차트를 만드는 방법을 설명합니다. 이전에는 차트 유형의 차트 유형을 배웠습니다. JS : 라인 차트 및 막대 차트 (자습서 2)와 레이더 차트 및 극지 지역 차트 (자습서 3)를 배웠습니다. 파이 및 링 차트를 만듭니다 파이 차트와 링 차트는 다른 부분으로 나뉘어 진 전체의 비율을 보여주는 데 이상적입니다. 예를 들어, 파이 차트는 사파리에서 남성 사자, 여성 사자 및 젊은 사자의 비율 또는 선거에서 다른 후보자가받는 투표율을 보여주는 데 사용될 수 있습니다. 파이 차트는 단일 매개 변수 또는 데이터 세트를 비교하는 데만 적합합니다. 파이 차트의 팬 각도는 데이터 포인트의 숫자 크기에 의존하기 때문에 원형 차트는 값이 0 인 엔티티를 그릴 수 없습니다. 이것은 비율이 0 인 모든 엔티티를 의미합니다
