PHP 7.1의 새로운 기능 한눈에 보기
Nullable 유형
Nullable 유형은 주로 매개변수 유형 선언 및 함수 반환값 선언에 사용됩니다.
주요 두 가지 형식은 다음과 같습니다.
<?phpfunction answer(): ?int { return null; //ok}function answer(): ?int { return 42; // ok}function say(?string $msg) { if ($msg) { echo $msg; }}
예제를 보면 이해하기 쉽습니다. 함수 매개변수의 유형을 나타내기 위해 ? 반환 값은 지정된 유형이거나 null 입니다.
이 방법은 인터페이스 함수 정의에도 사용할 수 있습니다.
<?php interface Fooable { function foo(?Fooable $f);}
그러나 한 가지 주의할 점은 함수 자체가 매개변수 유형을 정의하고 기본값이 없는 경우입니다. , nullable인 경우에도 생략할 수 없습니다. 그렇지 않으면 오류가 발생합니다. 다음과 같습니다:
<?php function foo_nullable(?Bar $bar) {} foo_nullable(new Bar); // 可行foo_nullable(null); // 可行foo_nullable(); // 不可行
그러나 위 함수의 매개변수를 ?Bar $bar = null 형식으로 정의하면 세 번째 작성 방법도 가능합니다. = null은 실제로 ? 의 상위 집합과 동일하므로 null 허용 형식 매개 변수의 경우 null을 기본값으로 설정할 수 있습니다.
list의 대괄호 약어
PHP5.4 이전에는 array()를 통해서만 배열을 정의할 수 있다는 것을 알고 있습니다. 5.4 이후에 추가됨 []의 단순화된 표기(아직도 5자를 생략하는 것이 매우 실용적임).
<?php // 5.4 之前$array = array(1, 2, 3);$array = array("a" => 1, "b" => 2, "c" => 3); // 5.4 及之后$array = [1, 2, 3];$array = ["a" => 1, "b" => 2, "c" => 3];
는 또 다른 질문으로 확장됩니다. 배열의 값을 다른 변수에 할당하려면 목록을 통해 달성할 수 있습니다.
<?php list($a, $b, $c) = $array;
[의 약어를 통해서도 달성할 수 있나요? ] 모직물?
<?php [$a, $b, $c] = $array;
및 다음 기능에서 언급되는 목록 지정 키:
<?php ["a" => $a, "b" => $b, "c" => $c] = $array;
PHP7.1은 이 기능을 구현합니다. 다만, lvalue에 나타나는 []는 array의 약자가 아니고 list()의 약자라는 점에 유의해야 한다.
그러나 이것이 전부는 아닙니다. 새로운 list() 구현은 lvalue에 나타날 수 있을 뿐만 아니라 foreach 루프에서도 사용할 수 있습니다.
<?php foreach ($points as ["x" => $x, "y" => $y]) { var_dump($x, $y);
그러나 구현 문제로 인해 list()와 []를 서로 중첩하여 사용할 수 없습니다.
<?php // 不合法 list([$a, $b], [$c, $d]) = [[1, 2], [3, 4]]; // 不合法 [list($a, $b), list($c, $d)] = [[1, 2], [3, 4]]; // 合法 [[$a, $b], [$c, $d]] = [[1, 2], [3, 4]];
목록에 키를 지정할 수 있습니다
위에서 언급했듯이 new 키는 list() 구현에서 지정할 수 있습니다:
<?php $array = ["a" => 1, "b" => 2, "c" => 3];["a" => $a, "b" => $b, "c" => $c] = $array;
이는
<?php $a = $array['a'];$b = $array['b'];$c = $array['c'];
와 동일합니다. 이전과의 차이점은 list()의 이전 구현이 다음과 동일하다는 것입니다. 키는 0, 1, 2, 3 뿐입니다. 순서는 조정할 수 없습니다. 다음 명령문을 실행하면
<?php list($a, $b) = [1 => '1', 2 => '2'];
오류가 발생합니다. PHP error: Undefine offset: 0... .
새 구현에서는 다음과 같은 방법으로 할당을 조정할 수 있습니다.
<?php list(1 => $a, 2 => $b) = [1 => '1', 2 => '2'];
배열과 달리 목록은 혼합 키를 지원하지 않습니다. 다음 작성은 구문 분석 오류를 유발합니다. >
<?php // Parse error: syntax error, ...list($unkeyed, "key" => $keyed) = $array;
<?php $points = [ ["x" => 1, "y" => 2], ["x" => 2, "y" => 1]]; list(list("x" => $x1, "y" => $y1), list("x" => $x2, "y" => $y2)) = $points; $points = [ "first" => [1, 2], "second" => [2, 1]]; list("first" => list($x1, $y1), "second" => list($x2, $y2)) = $points;
<?php $points = [ ["x" => 1, "y" => 2], ["x" => 2, "y" => 1]]; foreach ($points as list("x" => $x, "y" => $y)) { echo "Point at ($x, $y)", PHP_EOL;}
<?php function should_return_nothing(): void { return 1; // Fatal error: A void function must not return a value}
<?php function lacks_return(): void { // valid} function returns_nothing(): void { return; // valid}
<?php function returns_one(): void { return 1; // Fatal error: A void function must not return a value} function returns_null(): void { return null; // Fatal error: A void function must not return a value}
<?php function foobar(void $foo) { // Fatal error: void cannot be used as a parameter type}
<?php class Foo{ public function bar(): void { } } class Foobar extends Foo{ public function bar(): array { // Fatal error: Declaration of Foobar::bar() must be compatible with Foo::bar(): void }}
이 기능은 상대적으로 간단합니다. 즉, 클래스의 상수는 이제 공개, 비공개 및 보호 수정을 지원합니다.
<?php class Token { // 常量默认为 public const PUBLIC_CONST = 0; // 可以自定义常量的可见范围 private const PRIVATE_CONST = 0; protected const PROTECTED_CONST = 0; public const PUBLIC_CONST_TWO = 0; // 多个常量同时声明只能有一个属性 private const FOO = 1, BAR = 2;}
<?php interface ICache { public const PUBLIC = 0; const IMPLICIT_PUBLIC = 1;}
<?php class testClass { const TEST_CONST = 'test'; } $obj = new ReflectionClass( "testClass" ); $const = $obj->getReflectionConstant( "TEST_CONST" ); $consts = $obj->getReflectionConstants();
<?php try { // Some code... } catch (ExceptionType1 $e) { // 处理 ExceptionType1} catch (ExceptionType2 $e) { // 处理 ExceptionType2} catch (Exception $e) { // ...}
<?php try { // Some code...} catch (ExceptionType1 | ExceptionType2 $e) { // 对于 ExceptionType1 和 ExceptionType2 的处理} catch (Exception $e) { // ...}

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










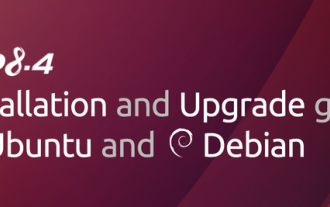
PHP 8.4는 상당한 양의 기능 중단 및 제거를 통해 몇 가지 새로운 기능, 보안 개선 및 성능 개선을 제공합니다. 이 가이드에서는 Ubuntu, Debian 또는 해당 파생 제품에서 PHP 8.4를 설치하거나 PHP 8.4로 업그레이드하는 방법을 설명합니다.
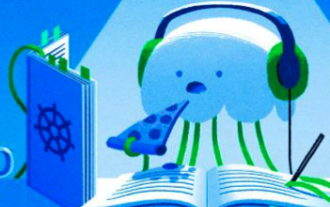
VS Code라고도 알려진 Visual Studio Code는 모든 주요 운영 체제에서 사용할 수 있는 무료 소스 코드 편집기 또는 통합 개발 환경(IDE)입니다. 다양한 프로그래밍 언어에 대한 대규모 확장 모음을 통해 VS Code는
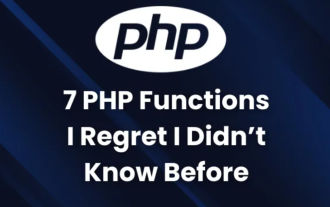
숙련된 PHP 개발자라면 이미 그런 일을 해왔다는 느낌을 받을 것입니다. 귀하는 상당한 수의 애플리케이션을 개발하고, 수백만 줄의 코드를 디버깅하고, 여러 스크립트를 수정하여 작업을 수행했습니다.
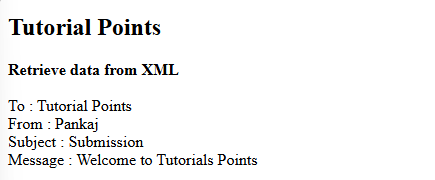
이 튜토리얼은 PHP를 사용하여 XML 문서를 효율적으로 처리하는 방법을 보여줍니다. XML (Extensible Markup Language)은 인간의 가독성과 기계 구문 분석을 위해 설계된 다목적 텍스트 기반 마크 업 언어입니다. 일반적으로 데이터 저장 AN에 사용됩니다
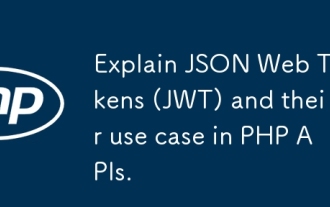
JWT는 주로 신분증 인증 및 정보 교환을 위해 당사자간에 정보를 안전하게 전송하는 데 사용되는 JSON을 기반으로 한 개방형 표준입니다. 1. JWT는 헤더, 페이로드 및 서명의 세 부분으로 구성됩니다. 2. JWT의 작업 원칙에는 세 가지 단계가 포함됩니다. JWT 생성, JWT 확인 및 Parsing Payload. 3. PHP에서 인증에 JWT를 사용하면 JWT를 생성하고 확인할 수 있으며 사용자 역할 및 권한 정보가 고급 사용에 포함될 수 있습니다. 4. 일반적인 오류에는 서명 검증 실패, 토큰 만료 및 대형 페이로드가 포함됩니다. 디버깅 기술에는 디버깅 도구 및 로깅 사용이 포함됩니다. 5. 성능 최적화 및 모범 사례에는 적절한 시그니처 알고리즘 사용, 타당성 기간 설정 합리적,
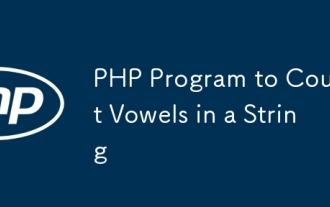
문자열은 문자, 숫자 및 기호를 포함하여 일련의 문자입니다. 이 튜토리얼은 다른 방법을 사용하여 PHP의 주어진 문자열의 모음 수를 계산하는 방법을 배웁니다. 영어의 모음은 A, E, I, O, U이며 대문자 또는 소문자 일 수 있습니다. 모음이란 무엇입니까? 모음은 특정 발음을 나타내는 알파벳 문자입니다. 대문자와 소문자를 포함하여 영어에는 5 개의 모음이 있습니다. a, e, i, o, u 예 1 입력 : String = "Tutorialspoint" 출력 : 6 설명하다 문자열의 "Tutorialspoint"의 모음은 u, o, i, a, o, i입니다. 총 6 개의 위안이 있습니다
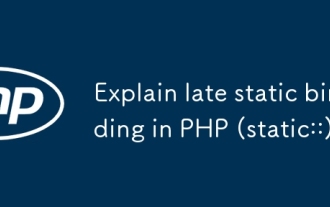
정적 바인딩 (정적 : :)는 PHP에서 늦은 정적 바인딩 (LSB)을 구현하여 클래스를 정의하는 대신 정적 컨텍스트에서 호출 클래스를 참조 할 수 있습니다. 1) 구문 분석 프로세스는 런타임에 수행됩니다. 2) 상속 관계에서 통화 클래스를 찾아보십시오. 3) 성능 오버 헤드를 가져올 수 있습니다.

PHP의 마법 방법은 무엇입니까? PHP의 마법 방법은 다음과 같습니다. 1. \ _ \ _ Construct, 객체를 초기화하는 데 사용됩니다. 2. \ _ \ _ 파괴, 자원을 정리하는 데 사용됩니다. 3. \ _ \ _ 호출, 존재하지 않는 메소드 호출을 처리하십시오. 4. \ _ \ _ get, 동적 속성 액세스를 구현하십시오. 5. \ _ \ _ Set, 동적 속성 설정을 구현하십시오. 이러한 방법은 특정 상황에서 자동으로 호출되어 코드 유연성과 효율성을 향상시킵니다.
