Spring Boot 시리즈의 속성 구성 및 사용자 정의 속성 구성에 대한 자세한 소개
스프링 부트를 사용하는 과정에서 해당 기능을 완료하려면 프로젝트에서 최소한의 구성만 필요하다는 것을 알 수 있습니다. 이는 pom.xml에 의존하는 각 스타터에 기본 구성이 있기 때문입니다. . , 이러한 기본 구성은 일반적인 기능 개발에 충분합니다.
사용자 정의 또는 기본 구성을 수정해야 하는 경우 spring boot는 application.properties에서 해당 구성을 추가하고 수정하기만 하면 됩니다. (application.properties의 기본 구성은 spring boot가 시작될 때 읽혀집니다.)
1. 기본 구성 수정
Example 1. spring boot가 웹 애플리케이션을 개발할 때 tomcat의 기본 시작 포트는 8080입니다. 수정이 필요한 경우 기본 포트인 경우 application.properties에 다음 레코드를 추가해야 합니다.
server.port=8888
프로젝트를 다시 시작하면 시작 로그를 볼 수 있습니다. Tomcat이 포트에서 시작됨: 8888(http) 시작 포트는 8888이며 http에 액세스합니다. 브라우저의 ://localhost :8888 에서는 정상적으로 접속이 가능합니다.
예제 2, 스프링 부트 개발 시 데이터베이스 연결 정보 구성(여기에서는 com.alibaba의 druid가 사용됨), application.properties에 다음 레코드를 추가합니다.
druid.url=jdbc:mysql://192.168.0.20:3306/test druid.driver-class=com.mysql.jdbc.Driver druid.username=root druid.password=123456 druid.initial-size=1 druid.min-idle=1 druid.max-active=20 druid.test-on-borrow=true
위 두 예는 스타터에서 기본 설정을 수정하는 방법을 보여줍니다. 모듈 구성, application.properties에서 수정해야 하는 구성을 추가하기만 하면 됩니다.
2. 사용자 정의 속성 구성
application.properties의 기본 구성을 수정하는 것 외에도 여기에서 사용자 정의 속성을 구성하고 엔터티 빈에 로드할 수도 있습니다.
1. application.properties에 사용자 정의 속성 구성 추가
com.sam.name=sam com.sam.age=11 com.sam.desc=magical sam
2. Bean 클래스 작성 및 속성 로드
Sam 클래스는 Spring이 시작될 때 클래스를 스캔하고 이를 Spring에 추가할 수 있도록 @Component 주석을 추가해야 합니다. 컨테이너.
첫 번째: 스프링에서 지원하는 @Value()를 사용하여 로드
package com.sam.demo.conf; import org.springframework.beans.factory.annotation.Value; import org.springframework.stereotype.Component; /** * @author sam * @since 2017/7/15 */ @Component public class Sam { //获取application.properties的属性 @Value("${com.sam.name}") private String name; @Value("${com.sam.age}") private int age; @Value("${com.sam.desc}") private String desc; //getter & setter }
두 번째: @ConfigurationProperties(prefix="")를 사용하여 접두사를 설정하고 속성에는 주석이 필요하지 않습니다.
package com.sam.demo.conf; import org.springframework.stereotype.Component; /** * @author sam * @since 2017/7/15 */ @Component @ConfigurationProperties(prefix = "com.sam") public class Sam { private String name; private int age; private String desc; //getter & setter }
3. 컨트롤러에 샘빈을 주입해서 사용해보세요.
package com.sam.demo.controller; import com.sam.demo.conf.Sam; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; /** * @author sam * @since 2017/7/14 */ @RestController public class IndexController { @Autowired private Sam sam; @RequestMapping("/index") public String index() { System.out.println(sam.getName() + " " + sam.getAge() + " " + sam.getDesc()); return "index"; } }
브라우저 접속: http://localhost:8080/index, 콘솔은 sam의 내용을 정상적으로 출력합니다.
3. application.properties 속성 구성에 대한 자세한 설명
1. 매개변수 참조 및 난수 방식 사용
application.properties에서는 다음과 같이 ${}를 통해 다른 속성의 값을 직접 참조할 수 있습니다.
com.sam.name=sam com.sam.age=11 com.sam.desc=${name} is ${age} years old.
애플리케이션에서 속성에서 난수를 구해야 하는 경우 다음과 같이 ${random}을 사용할 수 있습니다.
#获取随机字符串 com.sam.randomValue=${random.value} #获取随机字符串:${random.value} #获取随机int:${random.int} #获取10以内的随机数:${random.int(10)} #获取10-20的随机数:${random.int[10,20]} #获取随机long:${random.long} #获取随机uuid:${random.uuid}
2. 다중 환경 구성
실제 개발에서는 다른 환경이 있을 수 있습니다. 개발 환경, 테스트 환경, 프로덕션 환경을 포함합니다. 데이터베이스 정보, 포트 구성, 로컬 경로 구성 등 관련 구성은 환경마다 다를 수 있습니다.
다른 환경으로 전환할 때마다 application.properties를 수정해야 한다면 작업이 매우 번거로울 것입니다. Spring Boot에서는 다중 환경 구성이 제공되므로 환경 전환이 쉽습니다.
application.properties와 동일한 디렉터리에 세 개의 새 파일을 만듭니다.
application-dev.properties //开发环境的配置文件 application-test.properties //测试环境的配置文件 application-prod.properties //生产环境的配置文件
위 세 파일은 각각 개발, 테스트 및 프로덕션의 구성 내용에 해당합니다. 다음 단계는 이러한 구성을 선택적으로 참조하는 방법입니다.
application.properties에 추가:
spring.profiles.active=dev #引用测试的配置文件 #spring.profiles.active=test #引用生产的配置文件 #spring.profiles.active=prod
spring.profiles.active=dev를 추가하고 애플리케이션을 시작하면 dev의 구성 정보가 참조되는 것을 확인할 수 있습니다.
위 세 가지 구성 파일은 application-{profile}.properties 형식을 따르고 있으며 application.properties에 추가된 spring.profiles.active=dev의 dev가 바로 위 구성 파일의 프로필임을 알 수 있습니다. 특정 환경에 따라 즉시 전환됩니다.
애플리케이션 시작 명령으로 jar 패키지를 실행할 때 해당 구성을 지정할 수 있습니다.
java -jar demo-0.0.1-SNAPSHOT.jar --spring.profiles.active=dev
첨부: 구성 방법 및 우선순위
这些方式优先级如下: a. 命令行参数 b. 来自java:comp/env的JNDI属性 c. Java系统属性(System.getProperties()) d. 操作系统环境变量 e. RandomValuePropertySource配置的random.*属性值 f. jar外部的application-{profile}.properties或application.yml(带spring.profile)配置文件 g. jar内部的application-{profile}.properties或application.yml(带spring.profile)配置文件 h. jar外部的application.properties或application.yml(不带spring.profile)配置文件 i. jar内部的application.properties或application.yml(不带spring.profile)配置文件 j. @Configuration注解类上的@PropertySource k. 通过SpringApplication.setDefaultProperties指定的默认属性
참고: 명령줄 매개변수 이 방법은 매개변수를 지정하여 애플리케이션을 시작하는 방법입니다. jar 패키지는 안전하지 않으므로 다음과 같이 애플리케이션 시작을 금지하도록 설정할 수 있습니다.
springApplication.setAddCommandLineProperties(false);
package com.sam.demo; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class DemoApplication { public static void main(String[] args) { // SpringApplication.run(DemoApplication.class, args); SpringApplication springApplication = new SpringApplication(DemoApplication.class); //禁止命令行设置参数 springApplication.setAddCommandLineProperties(false); springApplication.run(args); } }
추가:
application.properties를 지원하는 것 외에도, Spring Boot의 구성은 application.yml도 지원합니다. 구성 방법은 다음과 같습니다.
application.properties 대신 새 application.yml을 만듭니다.
server: port: 9999 com: sam: name: sam age: 11 desc: magical sam
참고: 포트 중간에 공백이 있습니다: 9999. yml 구문의 경우 참조: yml 구성 파일 사용법
위 내용은 Spring Boot 시리즈의 속성 구성 및 사용자 정의 속성 구성에 대한 자세한 소개의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










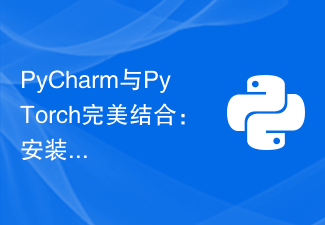
PyCharm은 강력한 통합 개발 환경(IDE)이고, PyTorch는 딥 러닝 분야에서 인기 있는 오픈 소스 프레임워크입니다. 머신러닝과 딥러닝 분야에서 PyCharm과 PyTorch를 개발에 활용하면 개발 효율성과 코드 품질을 크게 향상시킬 수 있습니다. 이 기사에서는 PyCharm에서 PyTorch를 설치 및 구성하는 방법을 자세히 소개하고 독자가 이 두 가지의 강력한 기능을 더 잘 활용할 수 있도록 특정 코드 예제를 첨부합니다. 1단계: PyCharm 및 Python 설치
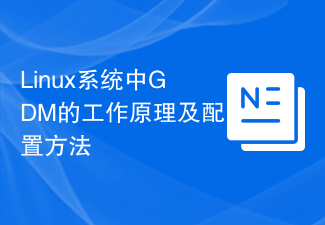
제목: Linux 시스템에서 GDM의 작동 원리 및 구성 방법 Linux 운영 체제에서 GDM(GNOMEDisplayManager)은 그래픽 사용자 인터페이스(GUI) 로그인 및 사용자 세션 관리를 제어하는 데 사용되는 일반적인 디스플레이 관리자입니다. 이 기사에서는 GDM의 작동 원리와 구성 방법을 소개하고 구체적인 코드 예제를 제공합니다. 1. GDM의 작동 원리 GDM은 GNOME 데스크탑 환경의 디스플레이 관리자이며 X 서버를 시작하고 사용자에게 로그인 인터페이스를 제공합니다.
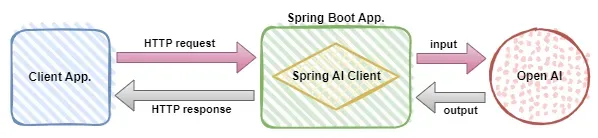
업계 리더인 Spring+AI는 강력하고 유연한 API와 고급 기능을 통해 다양한 산업에 선도적인 솔루션을 제공합니다. 이 주제에서는 다양한 분야의 Spring+AI 적용 사례를 살펴보겠습니다. 각 사례에서는 Spring+AI가 어떻게 특정 요구 사항을 충족하고 목표를 달성하며 이러한 LESSONSLEARNED를 더 넓은 범위의 애플리케이션으로 확장하는지 보여줍니다. 이 주제가 여러분이 Spring+AI의 무한한 가능성을 더 깊이 이해하고 활용하는 데 영감을 줄 수 있기를 바랍니다. Spring 프레임워크는 소프트웨어 개발 분야에서 20년 이상의 역사를 가지고 있으며, Spring Boot 1.0 버전이 출시된 지 10년이 되었습니다. 이제 봄이 왔다는 것에 대해 누구도 이의를 제기할 수 없습니다.
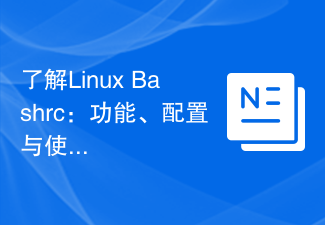
Linux Bashrc 이해: 기능, 구성 및 사용법 Linux 시스템에서 Bashrc(BourneAgainShellruncommands)는 시스템 시작 시 자동으로 실행되는 다양한 명령과 설정이 포함된 매우 중요한 구성 파일입니다. Bashrc 파일은 일반적으로 사용자의 홈 디렉토리에 있으며 숨겨진 파일입니다. 해당 기능은 사용자를 위해 Bashshell 환경을 사용자 정의하는 것입니다. 1. Bashrc 기능 설정 환경
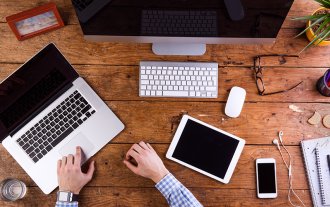
Win11에서 작업 그룹을 구성하는 방법 작업 그룹은 로컬 영역 네트워크에서 여러 컴퓨터를 연결하는 방법으로, 파일, 프린터 및 기타 리소스를 컴퓨터 간에 공유할 수 있습니다. Win11 시스템에서는 작업 그룹을 구성하는 것이 매우 간단합니다. 아래 단계를 따르십시오. 1단계: "설정" 애플리케이션을 엽니다. 먼저 Win11 시스템의 "시작" 버튼을 클릭한 다음 팝업 메뉴에서 "설정" 애플리케이션을 선택합니다. "Win+I" 단축키를 사용하여 "설정"을 열 수도 있습니다. 2단계: "시스템"을 선택하세요. 설정 앱에 여러 옵션이 표시됩니다. 시스템 설정 페이지로 들어가려면 "시스템" 옵션을 클릭하세요. 3단계: "정보"를 선택합니다. "시스템" 설정 페이지에 여러 하위 옵션이 표시됩니다. 클릭하세요
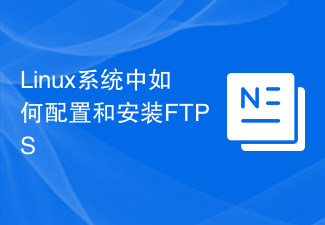
제목: Linux 시스템에서 FTPS를 구성하고 설치하는 방법에는 특정 코드 예제가 필요합니다. Linux 시스템에서 FTPS는 FTP와 비교하여 전송된 데이터를 TLS/SSL 프로토콜을 통해 암호화하므로 성능이 향상됩니다. 데이터 전송의 보안. 이 기사에서는 Linux 시스템에서 FTPS를 구성 및 설치하는 방법을 소개하고 구체적인 코드 예제를 제공합니다. 1단계: vsftpd 설치 터미널을 열고 다음 명령을 입력하여 vsftpd를 설치합니다. sudo
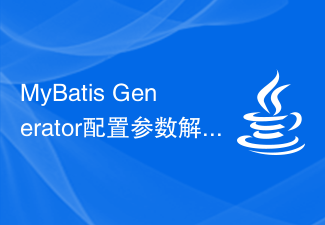
MyBatisGenerator는 MyBatis에서 공식적으로 제공하는 코드 생성 도구로, 개발자가 데이터베이스 테이블 구조에 맞는 JavaBeans, Mapper 인터페이스 및 XML 매핑 파일을 빠르게 생성할 수 있도록 도와줍니다. 코드 생성을 위해 MyBatisGenerator를 사용하는 과정에서 구성 매개변수 설정이 중요합니다. 이 글은 구성 매개변수의 관점에서 시작하여 MyBatisGenerator의 기능을 깊이 탐구할 것입니다.
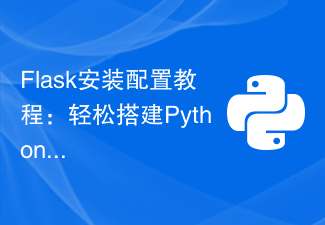
Flask 설치 및 구성 튜토리얼: Python 웹 애플리케이션을 쉽게 구축하기 위한 도구로, 특정 코드 예제가 필요합니다. 소개: Python의 인기가 높아짐에 따라 웹 개발은 Python 프로그래머에게 필요한 기술 중 하나가 되었습니다. Python으로 웹 개발을 수행하려면 적합한 웹 프레임워크를 선택해야 합니다. 많은 Python 웹 프레임워크 중에서 Flask는 개발자가 선호하는 간단하고 사용하기 쉽고 유연한 프레임워크입니다. 이번 글에서는 Flask 프레임워크 설치에 대해 소개하겠습니다.
