JS로 시뮬레이터를 작성하는 방법
이번에는 JS를 사용하여 시뮬레이터를 작성하는 방법과 JS에서 시뮬레이터를 작성할 때 어떤 노트를 사용해야 하는지 보여드리겠습니다. 다음은 실제 사례입니다.
0x00 CHIP8 소개
CHIP8 Wiki에서 CHIP8이 해석된 프로그래밍 언어라는 것을 알 수 있습니다. 1970년대 중반에 처음 사용됐다. CHIP8 프로그램은 CHIP8 가상 머신에서 실행되며, 그 출현으로 인해 비디오 게임 프로그래밍이 그 시대에 비해 더 단순해졌습니다. CHIP8로 구현된 전자 게임에는 Bee, Tetris, Pac-Man 등이 있습니다. CHIP8의 위키에 대해 더 자세히 알아볼 수 있습니다.
0x01 CHIP8 개체 만들기
CHIP8이 프로세서, 키보드, 디스플레이 및 스피커로 구성되어 있고 CPU가 CHIP8의 핵심이라고 가정하면 코드는 다음과 같아야 합니다.
<!DOCTYPE html><html><head> <title>创建Chip8对象</title></head><body> <script> (function () { function CPU() {/*...*/ }; function Screen() {/*...*/ }; function Keyboard() {/*...*/ }; function Speaker(){/*...*/ }; window.CHIP8 = function () { var c8 = new CPU(); c8.screen = new Screen(); c8.speaker = new Speaker(); c8.input = new Keyboard(); return c8; }; })(); </script></body></html>
0x02 간단한 디스플레이 작성
CHIP8에 따르면 Wiki에 따르면 CHIP8 디스플레이 해상도는 64X32픽셀이며 흑백입니다. 픽셀이 1이면 해당 픽셀이 화면에 표시되고, 0이면 표시되지 않습니다. 그러나 특정 픽셀이 존재했다가 존재하지 않는 경우 캐리 플래그가 1로 설정되어 충돌 감지에 사용될 수 있습니다.
그러면 코드는 다음과 같아야 합니다.
function Screen() { this.rows = 32;//32行 this.columns = 64;//64列 this.resolution = this.rows * this.columns;//分辨率 this.bitMap = new Array(this.resolution);//像素点阵 this.clear = function () { this.bitMap = new Array(this.resolution); } this.render = function () { };//显示渲染 this.setPixel = function (x, y) {//在屏幕坐标(x,y)进行计算与显示 // 显示溢出处理 if (x > this.columns - 1) while (x > this.columns - 1) x -= this.columns; if (x < 0) while (x < 0) x += this.columns; if (y > this.rows - 1) while (y > this.rows - 1) y -= this.rows; if (y < 0) while (y < 0) y += this.rows; //获取点阵索引 var location = x + (y * this.columns); //反向显示,假设二值颜色黑白分别用1、0代表,那么值为1那么就将值设置成0,同理0的话变成1 this.bitMap[location] = this.bitMap[location] ^ 1; return !this.bitMap[location]; } };
디스플레이 모듈을 작성한 후 디스플레이 모듈을 테스트하기 위해 디스플레이 화면을 작성합니다(온라인으로 화면 테스트 보기):
var chip8 = CHIP8(); chip8.screen.render = function () {//自定义实现显示渲染 var boxs = document.getElementById("boxs"); boxs.innerHTML = ""; for (var i of this.bitMap) { var d = document.createElement("span"); d.style = "width: 5px;height: 5px;float: left;"; d.style.backgroundColor = i ? "#000" : "#fff"; boxs.appendChild(d); } };/** 测试 **/chip8.screen.setPixel(2, 2);//设置x,y坐标像素chip8.screen.render(); chip8.screen.setPixel(2, 2);//设置x,y坐标像素
0x03 스피커 작성
을 참조해야 합니다. 여기에 웹 API가 있습니다:
API https://developer.mozilla.org/en-US/docs/Web/API/AudioContext API https://developer.mozilla.org/en-US/docs/Web/API/OscillatorNode
예제 https:/ /mdn.github.io/violent-theremin/
예제 https://codepen.io/gregh/pen/LxJEaj
스피커도 매우 간단합니다:
function Speaker() { var contextClass = (window.AudioContext || window.webkitAudioContext || window.mozAudioContext || window.oAudioContext || window.msAudioContext) , context , oscillator , gain; if (contextClass) { context = new contextClass(); gain = context.createGain(); gain.connect(context.destination); } //播放声音 this.play = function (frequency) { //API https://developer.mozilla.org/en-US/docs/Web/API/OscillatorNode //示例 https://mdn.github.io/violent-theremin/ if (context && !oscillator) { oscillator = context.createOscillator(); oscillator.frequency.value = frequency || 440;//声音频率 oscillator.type = oscillator.TRIANGLE;//波形这里用的是三角波 查看示例:https://codepen.io/gregh/pen/LxJEaj oscillator.connect(gain); oscillator.start(0); } } //停止播放 this.clear = this.stop = function () { if (oscillator) { oscillator.stop(0); oscillator.disconnect(0); oscillator = null; } } };
스피커를 작성한 후 스피커를 테스트할 수 있습니다(스피커 온라인 테스트 보기):
<!DOCTYPE html><html><head> <title>编写扬声器</title></head><body> 频率: <input type="range" id="frequency" value="440" min="100" max="1000"> <label id="showfv">(440)</label> <button id="play_btn">播放</button> <script> (function () { function CPU() {/*...*/ }; function Screen() {/*...*/ };//略... function Keyboard() {/*...*/ }; function Speaker() {/*...*/};//略... window.CHIP8 = function () { var c8 = new CPU(); c8.screen = new Screen(); c8.speaker = new Speaker(); c8.input = new Keyboard(); return c8; }; })(); var chip8 = CHIP8(); //======= var f = document.getElementById("frequency"); var isPlay = false; var play_btn = document.getElementById("play_btn"); f.onchange = function () { var v = Number(this.value); document.getElementById("showfv").innerHTML = "(" + v + ")"; if (isPlay) { chip8.speaker.stop(); chip8.speaker.play(v); } }; play_btn.onclick = function () { isPlay = !isPlay; this.innerHTML = isPlay ? '停止' : '播放'; if (!isPlay) chip8.speaker.stop(); else chip8.speaker.play(f.value); }; </script></body></html>
이 기사의 사례를 읽은 후 방법을 마스터했다고 생각합니다. 더 흥미로운 정보를 보려면 PHP의 다른 관련 기사에 주목하세요. 중국사이트!
관련 읽기:
플러그인 도구를 사용하여 ES6 코드를 ES5로 변환하는 방법
위 내용은 JS로 시뮬레이터를 작성하는 방법의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)
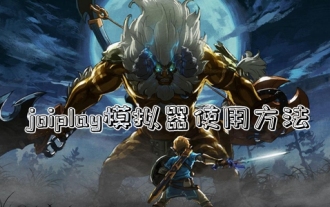
jojplay 시뮬레이터는 매우 사용하기 쉬운 휴대폰 시뮬레이터입니다. 컴퓨터 게임을 지원하고 휴대폰에서 실행할 수 있으며 호환성이 매우 좋습니다. 아래 편집기에서 소개하겠습니다. 사용 방법. joiplay 시뮬레이터 사용 방법 1. 먼저 Joiplay 본체와 RPGM 플러그인을 다운로드해야 합니다. 본체 - 플러그인 순서대로 설치하는 것이 가장 좋습니다. apk 패키지는 Joiplay 바에서 얻을 수 있습니다. >>>를 얻으려면 클릭하세요). 2. 안드로이드가 완성되면 왼쪽 하단에서 게임을 추가할 수 있습니다. 3. 이름을 아무렇게나 입력하고, 실행 파일에서 CHOOSE를 누르면 해당 게임의 game.exe 파일이 선택됩니다. 4. 아이콘을 비워두거나 좋아하는 사진을 선택할 수 있습니다.
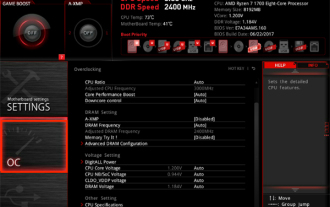
MSI 마더보드에서 VT를 활성화하는 방법은 무엇입니까? 방법은 무엇입니까? 이 사이트는 대부분의 사용자를 위해 MSI 마더보드 VT 활성화 방법을 주의 깊게 편집했습니다. 읽고 공유해 보세요! 첫 번째 단계는 컴퓨터를 다시 시작하고 BIOS로 들어가는 것입니다. 시작 속도가 너무 빨라서 BIOS로 들어갈 수 없으면 어떻게 해야 합니까? 화면이 켜진 후 "Del"을 계속 눌러 BIOS 페이지로 들어갑니다. 두 번째 단계는 메뉴에서 VT 옵션을 찾아서 켜는 것입니다. 컴퓨터 모델마다 BIOS 인터페이스가 다르며 상황 1이 다릅니다. : 1. 진입 BIOS 페이지에 진입한 후, "OC (또는 오버클러킹)" - "CPU 기능" - "SVMMode (또는 Intel Virtualization Technology)" 옵션을 찾아 "Disabled"로 변경하세요.
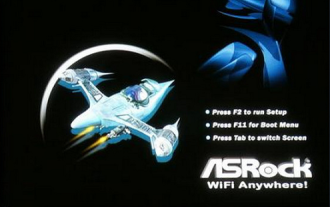
ASRock 마더보드에서 VT를 활성화하는 방법, 방법 및 작동 방법은 무엇입니까? 이 웹사이트는 사용자가 읽고 공유할 수 있도록 ASRock 마더보드 vt 활성화 방법을 편집했습니다! 첫 번째 단계는 컴퓨터를 다시 시작하는 것입니다. 화면이 켜진 후 "F2" 키를 계속 눌러 BIOS 페이지로 들어갑니다. 시작 속도가 너무 빨라서 BIOS로 들어갈 수 없으면 어떻게 해야 합니까? 두 번째 단계는 메뉴에서 VT 옵션을 찾아서 켜는 것입니다. 마더보드 모델마다 BIOS 인터페이스가 다르며 VT에 대한 이름도 다릅니다. 1. BIOS 페이지에 들어간 후 "Advanced(Advanced)" - "CPU Configuration"을 찾습니다. (CPU) 구성)" - "SVMMOD(가상화 기술)" 옵션에서 "비활성화"를 "활성화"로 변경합니다.
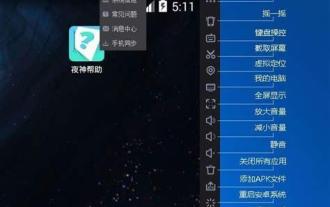
Android 에뮬레이터는 컴퓨터에서 Android 시스템의 실행을 시뮬레이션할 수 있는 소프트웨어입니다. 시중에는 다양한 종류의 Android 에뮬레이터가 있으며 품질도 다양합니다. 독자가 자신에게 가장 적합한 에뮬레이터를 선택할 수 있도록 돕기 위해 이 기사에서는 부드럽고 사용하기 쉬운 Android 에뮬레이터에 중점을 둘 것입니다. 1. BlueStacks: 빠른 실행 속도 뛰어난 실행 속도와 원활한 사용자 경험을 갖춘 BlueStacks는 인기 있는 Android 에뮬레이터입니다. 사용자가 다양한 모바일 게임과 애플리케이션을 플레이할 수 있도록 하여 매우 높은 성능으로 컴퓨터에서 Android 시스템을 에뮬레이트할 수 있습니다. 2. NoxPlayer: 다중 열기를 지원하여 여러 에뮬레이터에서 동시에 다양한 게임을 실행할 수 있습니다.
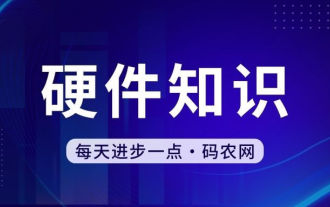
BBK 태블릿에서 Windows 시스템을 플래시하는 방법 첫 번째 방법은 시스템을 하드 디스크에 설치하는 것입니다. 컴퓨터 시스템이 충돌하지 않는 한 시스템에 들어가서 다운로드할 수 있으며 컴퓨터 하드 드라이브를 사용하여 시스템을 설치할 수 있습니다. 방법은 다음과 같습니다. 컴퓨터 구성에 따라 WIN7 운영 체제를 설치할 수 있습니다. 먼저, 귀하의 컴퓨터에 적합한 시스템 버전을 선택하고 다음 단계로 "이 시스템 설치"를 클릭하십시오. 그런 다음 설치 리소스가 다운로드될 때까지 인내심을 갖고 기다린 다음 환경이 배포되고 다시 시작될 때까지 기다립니다. vivopad에 win11을 설치하는 단계는 다음과 같습니다. 먼저 소프트웨어를 사용하여 win11을 설치할 수 있는지 확인합니다. 시스템 감지를 통과한 후 시스템 설정을 입력합니다. 거기에서 업데이트 및 보안 옵션을 선택하세요. 딸깍 하는 소리
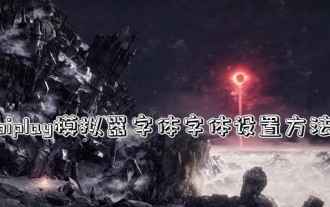
jojplay 시뮬레이터는 실제로 게임 글꼴을 사용자 정의할 수 있으며 텍스트에서 누락된 문자 및 상자 문자 문제를 해결할 수 있습니다. 아마도 많은 플레이어가 이를 작동하는 방법을 모르는 것 같습니다. jojplay 시뮬레이터의 글꼴을 소개합니다. joiplay 시뮬레이터 글꼴 설정 방법 1. 먼저 joiplay 시뮬레이터를 열고 오른쪽 상단에 있는 설정(점 3개)을 클릭하여 찾습니다. 2. RPGMSettings 열의 세 번째 행에서 CustomFont 사용자 정의 글꼴을 클릭하여 선택합니다. 3. 글꼴 파일을 선택하고 확인을 클릭합니다. 오른쪽 하단에 있는 "저장" 아이콘을 클릭하지 않도록 주의하세요. 그렇지 않으면 기본 설정이 복원됩니다. 4. 설립자 및 준원 간체 한자를 권장합니다(이미 Fuxing 및 Rebirth 게임 폴더에 있음). 조이
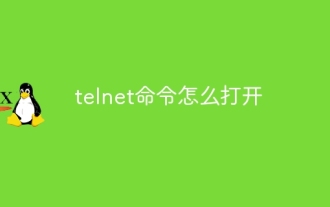
명령줄이나 기타 소프트웨어(PuTTY, Android용 Putty, iTerm2 등)를 사용하여 Telnet 명령을 열 수 있습니다. 명령줄에 "telnet"을 입력하고 Enter를 눌러 연 다음 "telnet [호스트 이름 또는 IP 주소] [포트]"를 사용하여 원격 장치에 연결하면 원격 장치의 명령 프롬프트를 볼 수 있습니다. 장치.
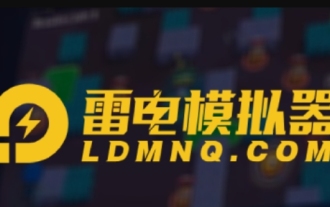
Thunderbolt Simulator의 공식 버전은 매우 전문적인 Android 에뮬레이터 도구입니다. 그렇다면 천둥 및 번개 시뮬레이터 애플리케이션을 삭제하는 방법은 무엇입니까? Thunderbolt Simulator에서 애플리케이션을 삭제하는 방법은 무엇입니까? 편집자가 아래 답변을 드리겠습니다! 천둥 및 번개 시뮬레이터 애플리케이션을 삭제하는 방법은 무엇입니까? 1. 삭제하려는 앱의 아이콘을 길게 클릭하세요. 2. 앱 제거 또는 삭제 옵션이 나타날 때까지 잠시 기다립니다. 3. 앱을 제거 옵션으로 드래그하세요. 4. 팝업되는 확인창에서 확인을 클릭하시면 애플리케이션 삭제가 완료됩니다.
