h5 게임 개발에 대한 자세한 설명
이번에는 h5 게임 개발에 대한 자세한 설명을 가져오겠습니다. h5 게임 개발 시 주의 사항은 무엇인가요? 실제 사례를 살펴보겠습니다.
저는 항상 HMTL5로 게임을 만드는 데 관심이 있었는데, 이 책은 HTML5 2 게임에 대한 소개일 뿐입니다. 데모는 간단하게 주석을 달고 상세하게 설명되어 있어 연습에 활용할 수 있으며, 일주일 정도 지나면 읽을 수 있습니다. 멋지고 고급스러운 효과를 찾고 있다면 이 책은 아마도 실망스러울 것입니다. 하지만 입문용 책으로는 여전히 좋습니다.
http://pan.baidu.com/s/1dD29Nhf
총 10개의 챕터가 있으며, 모두 얕은 것부터 깊은 것까지 아래의 미니 게임과 유사합니다. 데모 다운로드
그래픽과 그림 그리기는 매우 간단합니다. 핵심은 배열과 타이머를 사용하여 게임의 비즈니스 로직과 효과를 구현하는 것입니다. 간단한 로컬 저장, 사운드 및 비디오 재생. 그러나 게임 학습에 대한 욕구를 충족시키기에는 금 함량이 너무 적습니다. 당당은 위에서 좋은 평가를 받았습니다. 책의 출발점은 기본적인 소개이기도 하다. Html5 필수 가이드
1. 기본 그래픽:
//ball 球function Ball(sx, sy, rad, stylestring) { this.sx = sx; this.sy = sy; this.rad = rad; this.draw = drawball; this.moveit = moveball; this.fillstyle = stylestring; }function drawball() { ctx.fillStyle = this.fillstyle; ctx.beginPath(); //ctx.fillStyle= rgb(0,0,0); ctx.arc(this.sx, this.sy, this.rad, 0, Math.PI * 2, true); ctx.fill(); }function moveball(dx, dy) { this.sx += dx; this.sy += dy; }//Rect 方形function Myrectangle(sx, sy, swidth, sheight, stylestring) { this.sx = sx; this.sy = sy; this.swidth = swidth; this.sheight = sheight; this.fillstyle = stylestring; this.draw = drawrects; this.moveit = moveball;//move方法是一样的}function drawrects() { ctx.fillStyle = this.fillstyle; ctx.fillRect(this.sx, this.sy, this.swidth, this.sheight); }//多边形function Polycard(sx, sy, rad, n, frontbgcolor, backcolor, polycolor) { this.sx = sx; this.sy = sy; this.rad = rad; this.draw = drawpoly; this.frontbgcolor = frontbgcolor; this.backcolor = backcolor; this.polycolor = polycolor; this.n = n; this.angle = (2 * Math.PI) / n; //parens may not be needed. this.moveit = generalmove; }//画多边形function drawpoly() { ctx.fillStyle = this.frontbgcolor; ctx.strokeStyle = this.backcolor; ctx.fillRect(this.sx - 2 * this.rad, this.sy - 2 * this.rad, 4 * this.rad, 4 * this.rad); ctx.beginPath(); ctx.fillStyle = this.polycolor; var i; var rad = this.rad; ctx.beginPath(); ctx.moveTo(this.sx + rad * Math.cos(-.5 * this.angle), this.sy + rad * Math.sin(-.5 * this.angle)); for (i = 1; i < this.n; i++) { ctx.lineTo(this.sx + rad * Math.cos((i - .5) * this.angle), this.sy + rad * Math.sin((i - .5) * this.angle)); } ctx.fill(); }function generalmove(dx, dy) { this.sx += dx; this.sy += dy; }//图像function Picture(sx, sy, swidth, sheight, imga) { this.sx = sx; this.sy = sy; this.img = imga; this.swidth = swidth; this.sheight = sheight; this.draw = drawAnImage; }function drawAnImage() { ctx.drawImage(this.img, this.sx, this.sy, this.swidth, this.sheight); }
코드 보기
2. 마우스 위치 가져오기:
(ev.layerX || ev.layerX == 0) { mx == (ev.offsetX || ev.offsetX == 0) { mx ==
3. 키 입력 가져오기:
function getkey(event) { var keyCode; if(event == null) { keyCode = window.event.keyCode; window.event.preventDefault(); } else { keyCode = event.keyCode; event.preventDefault(); } switch(keyCode) { case 68: //按下D deal(); break; case 72: //按下H playerdone(); break; case 78: //按下N newgame(); break; default: alert("Press d, h, or n."); } }
4 이벤트 수신 추가:
var canvas1 = document.getElementById('canvas'); canvas1.addEventListener('mousedown', startwall, false);//false表示事件冒泡的顺序。 canvas1.addEventListener('mousemove', stretchwall, false); canvas1.addEventListener('mouseup', finish, false);
5. 일반적으로 배열에 균일하게 로드되며 타이머가 트리거될 때마다 다시 그려집니다. 모든 객체에는 그리기 방법이 있습니다.
var mypent = new Token(100, 100, 20, "rgb(0,0,250)", 5); everything.push(mypent); function drawall() { ctx.clearRect(0, 0, cwidth, cheight); var i; for (i = 0; i < everything.length; i++) { everything[i].draw(); } }
6.javascript객체 지향은 고급 언어만큼 강력하지 않으며 배열을 영리하게 사용하여 많은 기능을 구현합니다. 예를 들어, 카드를 섞는 동작입니다.
//洗牌就是更换了牌的位置 function shuffle() { var i = deck.length - 1;//deck代表一副牌 var s; while (i>0) {//这里循环一次 每张牌平均更换了两次位置 s = Math.floor(Math.random()*(i+1));//随机范围是0-i (包括i) swapindeck(s,i);//交换位置 i--; } } function swapindeck(j,k) { var hold = new MCard(deck[j].num,deck[j].suit,deck[j].picture.src); //MCard 是一张牌的对象。 deck[j] = deck[k]; deck[k] = hold; }
7. 수학적 지식은 여러 곳에서 필요합니다. 예를 들어 공이 충돌하면 x와 y 이동 방향을 바꿔야 합니다. 목표물이 맞았는지 확인합니다. xy가 특정 간격 내에 있는지 확인하는 것입니다. 그러나 움직이는 물체가 앞의 도로를 통과할 수 있는지, 벽을 통과할 수 없는지 여부를 결정합니다. 조금 복잡합니다. 미로 게임처럼요. 핵심은 선분에서 구 중심까지의 거리가 구의 반경보다 작지 않다는 것을 결정하는 것입니다.
.sx +=.sy += (i = 0; i < walls.length; i++= (intersect(wall.sx, wall.sy, wall.fx, wall.fy, .sx, .sy, .sx -=.sy -== fx -= fy -= 0.0 - ((sx - cx) * dx + (sy - cy) * dy) / ((dx * dx) + (dy * (t < 0.0= 0.0 (t > 1.0= 1.0= (sx+t*(fx-sx))-= (sy +t*(fy-sy))-= (dx*dx) +(dy* (rt<(rad*
이 기사의 사례를 읽으신 후 방법을 마스터하셨다고 생각합니다. 더 흥미로운 정보를 보려면 PHP 중국어 웹사이트의 다른 관련 기사를 주목하세요!
추천 도서:
위 내용은 h5 게임 개발에 대한 자세한 설명의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










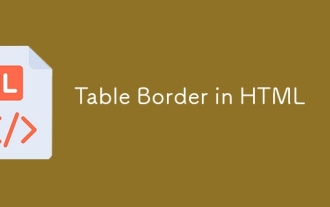
HTML의 테이블 테두리 안내. 여기에서는 HTML의 테이블 테두리 예제를 사용하여 테이블 테두리를 정의하는 여러 가지 방법을 논의합니다.
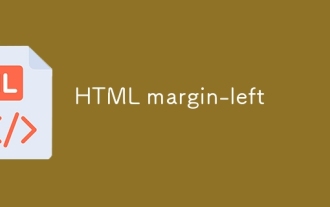
HTML 여백-왼쪽 안내. 여기에서는 HTML margin-left에 대한 간략한 개요와 코드 구현과 함께 예제를 논의합니다.
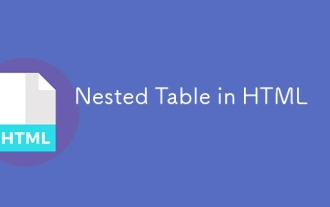
HTML의 Nested Table에 대한 안내입니다. 여기에서는 각 예와 함께 테이블 내에 테이블을 만드는 방법을 설명합니다.
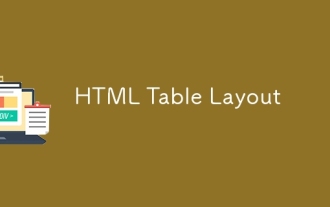
HTML 테이블 레이아웃 안내. 여기에서는 HTML 테이블 레이아웃의 값에 대해 예제 및 출력 n 세부 사항과 함께 논의합니다.
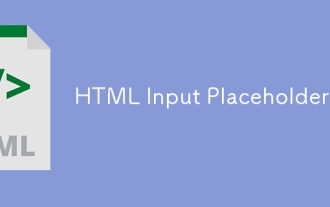
HTML 입력 자리 표시자 안내. 여기서는 코드 및 출력과 함께 HTML 입력 자리 표시자의 예를 논의합니다.
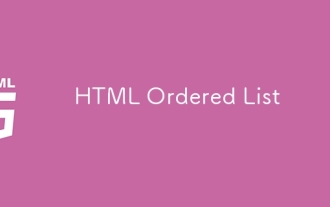
HTML 순서 목록에 대한 안내입니다. 여기서는 HTML Ordered 목록 및 유형에 대한 소개와 각각의 예에 대해서도 설명합니다.
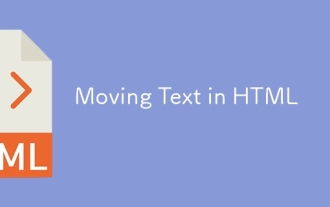
HTML에서 텍스트 이동 안내. 여기서는 Marquee 태그가 구문과 함께 작동하는 방식과 구현할 예제에 대해 소개합니다.
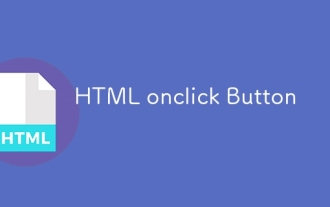
HTML onclick 버튼에 대한 안내입니다. 여기에서는 각각의 소개, 작업, 예제 및 다양한 이벤트의 onclick 이벤트에 대해 설명합니다.
