Laravel 학습의 기본
이 글은 주로 Laravel 학습에 대한 기본 지식을 소개하며, 이는 어느 정도 참고할만한 가치가 있습니다. 이제 도움이 필요한 친구들이 참고할 수 있도록 하겠습니다.
1.MVC 소개#🎜🎜 #
MVC의 전체 이름은 Model View Controller, 즉 model-view-controller의 약자입니다.2.laravel 코어 디렉터리 파일모델은 애플리케이션의 데이터 로직을 처리하는 데 사용되는 부분입니다
View는 데이터 표시를 처리하는 애플리케이션의 일부입니다
Controller는 사용자 상호 작용을 처리하는 애플리케이션의 일부입니다
#🎜 🎜##🎜 🎜#
- booststrap에는 프레임워크 시작 및 구성이 포함되어 있습니다. 파일 로드 중# 🎜🎜#
config에는 모든 구성 파일이 포함되어 있습니다.
-
데이터베이스에는 데이터베이스 채우기 및 마이그레이션 파일이 포함되어 있습니다.
#🎜 🎜# - public에는 프로젝트 항목 정적 리소스 파일이 포함되어 있습니다.
- resource에는 뷰와 원본 리소스 파일이 포함되어 있습니다.
- stroage에는 컴파일된 템플릿 파일과 파일 기반 세션 및 파일 캐시, 로그 및 프레임워크 파일이 포함되어 있습니다.
- 테스트 단위 테스트 파일#🎜 🎜# wendor에는 compose
- 3의 종속성 파일이 포함되어 있습니다. ###
- 라우팅 출력보기#########
4.Controller
컨트롤러 생성
Route::match(['get', 'post']), 'match', funtion() { return 'match'; }); Route::any(['get', 'post']), funtion() { return 'any'; });
Route::get('user/{name}', funtion($name) { return $id; })->where('name', '[A-Za-z]+'); Route::get('user/{id}/{name?}', funtion($id, $name='phyxiao') { return $id. $name; })->where(['id' => '[0-9]+', 'name'=> '[A-Za-z]+']);
Route::get('user/home', ['as' => 'home', funtion() { return route('home'); }]);
Route::group(['prefix' => 'user'], funtion() { Route::get('home', funtion() { return 'home'; }); Route::get('about', funtion() { return 'about'; }); });
경로 관련 컨트롤러
Route::get('index', funtion() { return view('welcome'); });
5.모델
php artisan make:controller UserController php artisan make:controller UserController --plain
6. 데이터베이스
세 가지 방법:DB facode 원본 검색
,Query builder
및 #🎜 🎜#Eloquent ORM#🎜 🎜#관련 파일
config/database.php,
.env 쿼리 생성자 Route::get('index', 'UserController@index');
로그인 후 복사php artisan make:model User
로그인 후 복사$bool = DB::table('user')->insert(['name => phyxiao', 'age' => 18]);
$id = DB::table('user')->insertGetId(['name => phyxiao', 'age' => 18]);
$bool = DB::table('user')->insert([
['name => phyxiao', 'age' => 18],
['name => aoteman', 'age' => 19],
);
var_dump($bool);
로그인 후 복사$num= DB::table('user')->where('id', 12)->update(['age' => 30]);
$num= DB::table('user')->increment('age', 3);
$num= DB::table('user')->decrement('age', 3);
$num= DB::table('user')->where('id', 12)->increment('age', 3);
$num= DB::table('user')->where('id', 12)->increment('age', 3, ['name' =>'handsome']);
로그인 후 복사$num= DB::table('user')->where('id', 12)->delete();
$num= DB::table('user')->where('id', '>=', 12)->delete();
DB::table('user')->truncate();
로그인 후 복사
Eloquent ORMRoute::get('index', 'UserController@index');
php artisan make:model User
$bool = DB::table('user')->insert(['name => phyxiao', 'age' => 18]); $id = DB::table('user')->insertGetId(['name => phyxiao', 'age' => 18]); $bool = DB::table('user')->insert([ ['name => phyxiao', 'age' => 18], ['name => aoteman', 'age' => 19], ); var_dump($bool);
$num= DB::table('user')->where('id', 12)->update(['age' => 30]); $num= DB::table('user')->increment('age', 3); $num= DB::table('user')->decrement('age', 3); $num= DB::table('user')->where('id', 12)->increment('age', 3); $num= DB::table('user')->where('id', 12)->increment('age', 3, ['name' =>'handsome']);
$num= DB::table('user')->where('id', 12)->delete(); $num= DB::table('user')->where('id', '>=', 12)->delete(); DB::table('user')->truncate();
$users= DB::table('user')->get(); $users= DB::table('user')->where('id', '>=', 12)->get(); $users= DB::table('user')->whereRaw('id >= ? and age > ?', [12, 18])->get(); dd(users); $user= DB::table('user')->orderBy('id', 'desc')->first(); $names = DB::table('user')->pluck('name'); $names = DB::table('user')->lists('name', 'id'); $users= DB::table('user')->select('id', 'age', 'name')->get(); $users= DB::table('user')->chunk(100, function($user){ dd($user); if($user->name == 'phyxiao') return false; });로그인 후 복사$num= DB::table('user')->count(); $max= DB::table('user')->max('age'); $min= DB::table('user')->min('age'); $avg= DB::table('user')->avg('age'); $sum= DB::table('user')->avg('sum');로그인 후 복사7.Blade 템플릿 엔진
// 建立模型 // app/user.php <?php namespace App; use Illuminate\Database\Eloquent\Model; class User extends Model { //指定表名 protected $table = 'user'; //指定id protected $primaryKey= 'id'; //指定允许批量赋值的字段 protected $fillable= ['name', 'age']; //指定不允许批量赋值的字段 protected $guarded= []; //自动维护时间戳 public $timestamps = true; protected function getDateFormat() { return time(); } protected function asDateTime($val) { return val; } }로그인 후 복사위 내용은 이 기사의 전체 내용입니다. 모든 사람의 학습에 도움이 되기를 바랍니다. 도움이 필요하시면 PHP 중국어 웹사이트에서 더 많은 관련 콘텐츠를 확인하세요! 관련 권장 사항:// ORM操作 // app/Http/Contollers/userController.php public function orm() { //all $students = Student::all(); //find $student = Student::find(12); //findOrFail $student = Student::findOrFail(12); // 结合查询构造器 $students = Student::get(); $students = Student::where('id', '>=', '10')->orderBy('age', 'desc')->first(); $num = Student::count(); //使用模型新增数据 $students = new Student(); $students->name = 'phyxiao'; $students->age= 18; $bool = $student->save(); $student = Student::find(20); echo date('Y-m-d H:i:s', $student->created_at); //使用模型的Create方法新增数据 $students = Student::create( ['name' => 'phyxiao', 'age' => 18] ); //firstOrCreate() $student = Student::firstOrCreate( ['name' => 'phyxiao'] ); //firstOrNew() $student = Student::firstOrNew( ['name' => 'phyxiao'] ); $bool= $student->save(); //使用模型更新数据 $student = Student::find(20); $student->name = 'phyxiao'; $student->age= 18; $bool = $student->save(); $num = Student::where('id', '>', 20)->update(['age' => 40]); //使用模型删除数据 $student = Student::find(20); $bool = $student->delete(); //使用主见删除数据 $num= Student::destroy(20); $num= Student::destroy([20, 21]); $num= Student::where('id', '>', 20)->delete; }로그인 후 복사
laravel의 디렉터리 구조위 내용은 Laravel 학습의 기본의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










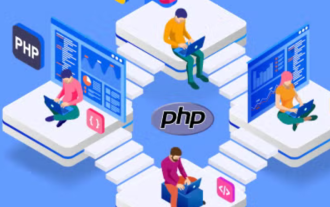
PHP 로깅은 웹 애플리케이션을 모니터링하고 디버깅하고 중요한 이벤트, 오류 및 런타임 동작을 캡처하는 데 필수적입니다. 시스템 성능에 대한 귀중한 통찰력을 제공하고 문제를 식별하며 더 빠른 문제 해결을 지원합니다.
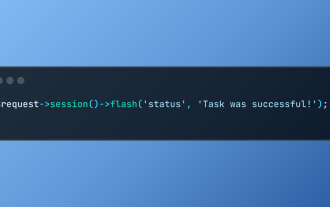
Laravel은 직관적 인 플래시 방법을 사용하여 임시 세션 데이터 처리를 단순화합니다. 응용 프로그램에 간단한 메시지, 경고 또는 알림을 표시하는 데 적합합니다. 데이터는 기본적으로 후속 요청에만 지속됩니다. $ 요청-
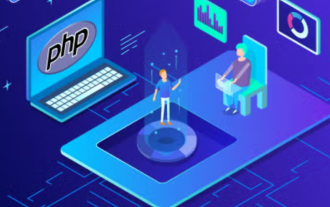
PHP 클라이언트 URL (CURL) 확장자는 개발자를위한 강력한 도구이며 원격 서버 및 REST API와의 원활한 상호 작용을 가능하게합니다. PHP CURL은 존경받는 다중 프로모토콜 파일 전송 라이브러리 인 Libcurl을 활용하여 효율적인 execu를 용이하게합니다.
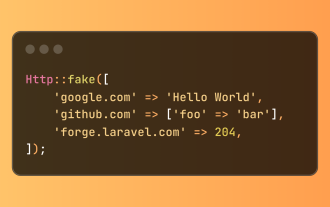
Laravel은 간결한 HTTP 응답 시뮬레이션 구문을 제공하여 HTTP 상호 작용 테스트를 단순화합니다. 이 접근법은 테스트 시뮬레이션을보다 직관적으로 만들면서 코드 중복성을 크게 줄입니다. 기본 구현은 다양한 응답 유형 단축키를 제공합니다. Illuminate \ support \ Facades \ http를 사용하십시오. http :: 가짜 ([ 'google.com'=> 'Hello World', 'github.com'=> [ 'foo'=> 'bar'], 'forge.laravel.com'=>
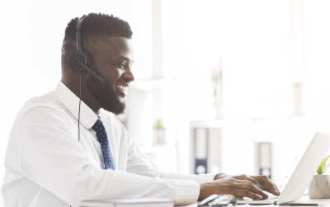
고객의 가장 긴급한 문제에 실시간 인스턴트 솔루션을 제공하고 싶습니까? 라이브 채팅을 통해 고객과 실시간 대화를 나누고 문제를 즉시 해결할 수 있습니다. 그것은 당신이 당신의 관습에 더 빠른 서비스를 제공 할 수 있도록합니다.
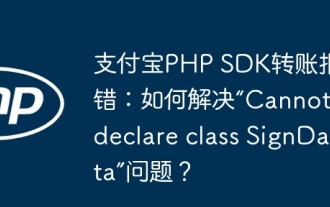
Alipay PHP ...
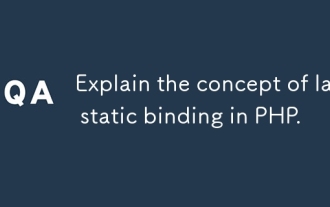
기사는 PHP 5.3에 도입 된 PHP의 LSB (Late STATIC BING)에 대해 논의하여 정적 방법의 런타임 해상도가보다 유연한 상속을 요구할 수있게한다. LSB의 실제 응용 프로그램 및 잠재적 성능
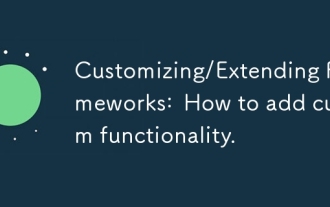
이 기사에서는 프레임 워크에 사용자 정의 기능 추가, 아키텍처 이해, 확장 지점 식별 및 통합 및 디버깅을위한 모범 사례에 중점을 둡니다.
