http 서버를 구현하기 위한 js의 콜백 함수
이 글에서 공유한 내용은 http 서버를 구현하기 위한 js의 콜백 기능에 관한 내용입니다. 다음으로 구체적인 내용을 살펴보겠습니다.
네트워크 운영
먼저 http 모듈을 사용하여 http 서버를 구현합니다
var http = require('http'); // 使用http模块 http.createServer ( function (request, response) { response.writeHead(200, {'Content-Type': 'text-plain'}); // http响应头部 response.end('hello word\n'); // 返回的内容 } ).listen(8124); // 监听8124端口
PS C:\Users\mingm\Desktop\test> node main.js
http://127.0.0.1:8124/를 방문하여 안녕하세요 단어를 반환합니다
일부 API
http 모듈
둘 이렇게
을 서버로 사용하게 되면 http 서버를 생성하고 http 클라이언트 요청을 듣고 응답을 돌려준다.
클라이언트로 사용하는 경우 http 클라이언트 요청을 시작하여 서버로부터 응답을 얻습니다.
서버 측은 이벤트 중심이며 서버 생성 시 콜백 함수는 한 번 호출됩니다. 이것은 이벤트 중심의
http 요청 헤더
입니다. http 요청의 본질은 요청 헤더와 요청 본문으로 구성된 데이터 스트림입니다.
브라우저의 개발자 도구를 열고 네트워크 패널을 선택한 다음 페이지를 새로 고치고 파일을 다시 선택하면 헤더 창에 현재 파일 요청의 http 헤더 정보가 표시됩니다
요청 헤더가 먼저 오고 그 다음이 요청 본문입니다
http 요청은 서버를 사용할 때 전체 요청 헤더를 수신한 후 1바이트의 데이터 스트림으로 전송되며, http 모듈에 의해 생성된 http 서버는
var http = require('http'); // 使用http模块 http.createServer ( function (request, response) { var body = []; console.log(request.method); console.log("--------------"); console.log(request.headers); console.log("---------------"); } ).listen(8124); // 监听8124端口
PS C:\Users\mingm\Desktop\test> node main.js GET -------------- { host: '127.0.0.1:8124', connection: 'keep-alive', 'cache-control': 'max-age=0', 'upgrade-insecure-requests': '1', dnt: '1', 'user-agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/67.0.3396.99 Safari/537.36', accept: 'text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,image/apng,*/*;q=0.8', 'accept-encoding': 'gzip, deflate, br', 'accept-language': 'zh-CN,zh;q=0.9' } ---------------
Callback이라는 콜백 기능을 수행합니다. function
var fs = require("fs"); fs.readFile('input.txt', function (err, data) { console.log("3333"); console.log(err); console.log(data.toString()); console.log("3333"); }); console.log("程序执行结束!");
PS C:\Users\mingm\Desktop\test> node main.js 程序执行结束! 3333 null 33333333333333333333333333 3333 PS C:\Users\mingm\Desktop\test>
필요할 때 /o 작업 중에는 먼저 실행을 건너뛰고 현재 내용이 실행됩니다. 그래서 결과는 이렇고 그 다음 실행 결과는 매개변수 목록의 마지막 함수에 전달되므로 마지막 함수는 콜백
http 콜백 함수,
var http = require('http'); http.createServer( function (request, response) { var body = []; console.log(request.method); console.log(request.headers); console.log(1111111111); console.log(body); request.on('end', function () { body = Buffer.concat(body); console.log(222222222222222); console.log(body.toString()); }); console.log(4444444444444); response.writeHead(200, {'Content-Type': 'text-plain'}); response.end('hello word\n'); console.log(55555555555); } ).listen(8124);
실행 결과
PS C:\Users\mingm\Desktop\test> node main.js GET { host: '127.0.0.1:8124', connection: 'keep-alive', 'cache-control': 'max-age=0', 'upgrade-insecure-requests': '1', 'user-agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/67.0.3396.99 Safari/537.36', dnt: '1', accept: 'text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,image/apng,*/*;q=0.8', 'accept-encoding': 'gzip, deflate, br', 'accept-language': 'zh-CN,zh;q=0.9' } 1111111111 [] 4444444444444 55555555555 222222222222222 GET { host: '127.0.0.1:8124', connection: 'keep-alive', pragma: 'no-cache', 'cache-control': 'no-cache', 'user-agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/67.0.3396.99 Safari/537.36', dnt: '1', accept: 'image/webp,image/apng,image/*,*/*;q=0.8', referer: 'http://127.0.0.1:8124/', 'accept-encoding': 'gzip, deflate, br', 'accept-language': 'zh-CN,zh;q=0.9' } 1111111111 [] 4444444444444 55555555555 222222222222222
를 요청하는 함수입니다. 이 실행은 비동기 실행이고, first Execu to
console.log(body);
request.on은 return을 기다려야 하기 때문에 다음의
console.log(444);
문을 비동기적으로 실행한 후 내용을 return한 후 request.on을 실행하고 그 결과를 다시 함수의 마지막 매개변수를 입력하면 실행이 완료됩니다.
http response
클라이언트가 요청한 요청 본문을 서버가 클라이언트에게 그대로 돌려준다
PS C:\Users\mingm\Desktop\test> node main.js 444444444444 22222222 33333333 555555
var http = require("http"); http.createServer(function (request, response){ console.log(444444444444); response.writeHead(200, { 'Content-Type': 'text/plain' }); // 为响应头,即原路发送给客户端 request.on( "data", function (chunk) { response.write(chunk); console.log(111111); }); console.log(22222222); request.on('end', function() {response.end();console.log(555555)}); console.log(33333333); } ).listen(8124);
글이 좀 지저분하다
http client
node가 http 클라이언트 요청을 보낸다
var options = { hostname: 'www.iming.info', port: 80, // 端口为80 path: '/upload', // 请求的路径 method: 'POST', // 请求的方法为post方法 headers: { 'Content-Type': 'application/x-www-form-urlencoded' // 头部信息 }, } var http = require('http'); var request = http.request(options, function (response) {}); request.write('hello word!'); request.end();
위는 http 물어보세요.
관련 권장 사항:
axios 소스 코드 분석 HTTP 요청 라이브러리 구현 방법
위 내용은 http 서버를 구현하기 위한 js의 콜백 함수의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제








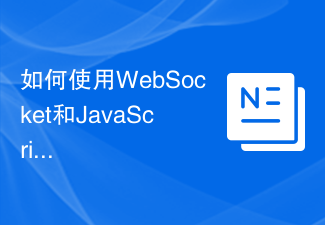
WebSocket 및 JavaScript를 사용하여 온라인 음성 인식 시스템을 구현하는 방법 소개: 지속적인 기술 개발로 음성 인식 기술은 인공 지능 분야의 중요한 부분이 되었습니다. WebSocket과 JavaScript를 기반으로 한 온라인 음성 인식 시스템은 낮은 대기 시간, 실시간, 크로스 플랫폼이라는 특징을 갖고 있으며 널리 사용되는 솔루션이 되었습니다. 이 기사에서는 WebSocket과 JavaScript를 사용하여 온라인 음성 인식 시스템을 구현하는 방법을 소개합니다.
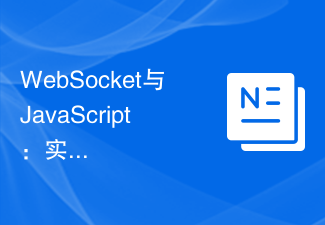
WebSocket과 JavaScript: 실시간 모니터링 시스템 구현을 위한 핵심 기술 서론: 인터넷 기술의 급속한 발전과 함께 실시간 모니터링 시스템이 다양한 분야에서 널리 활용되고 있다. 실시간 모니터링을 구현하는 핵심 기술 중 하나는 WebSocket과 JavaScript의 조합입니다. 이 기사에서는 실시간 모니터링 시스템에서 WebSocket 및 JavaScript의 적용을 소개하고 코드 예제를 제공하며 구현 원칙을 자세히 설명합니다. 1. 웹소켓 기술
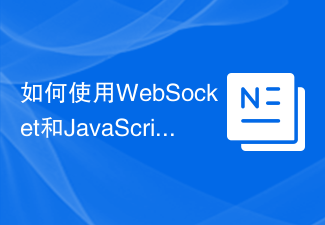
WebSocket과 JavaScript를 사용하여 온라인 예약 시스템을 구현하는 방법 오늘날의 디지털 시대에는 점점 더 많은 기업과 서비스에서 온라인 예약 기능을 제공해야 합니다. 효율적인 실시간 온라인 예약 시스템을 구현하는 것이 중요합니다. 이 기사에서는 WebSocket과 JavaScript를 사용하여 온라인 예약 시스템을 구현하는 방법을 소개하고 구체적인 코드 예제를 제공합니다. 1. WebSocket이란 무엇입니까? WebSocket은 단일 TCP 연결의 전이중 방식입니다.
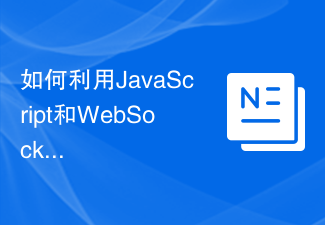
JavaScript 및 WebSocket을 사용하여 실시간 온라인 주문 시스템을 구현하는 방법 소개: 인터넷의 대중화와 기술의 발전으로 점점 더 많은 레스토랑에서 온라인 주문 서비스를 제공하기 시작했습니다. 실시간 온라인 주문 시스템을 구현하기 위해 JavaScript 및 WebSocket 기술을 사용할 수 있습니다. WebSocket은 TCP 프로토콜을 기반으로 하는 전이중 통신 프로토콜로 클라이언트와 서버 간의 실시간 양방향 통신을 실현할 수 있습니다. 실시간 온라인 주문 시스템에서는 사용자가 요리를 선택하고 주문을 하면
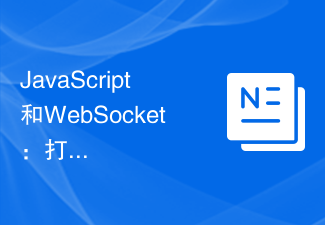
JavaScript 및 WebSocket: 효율적인 실시간 일기 예보 시스템 구축 소개: 오늘날 일기 예보의 정확성은 일상 생활과 의사 결정에 매우 중요합니다. 기술이 발전함에 따라 우리는 날씨 데이터를 실시간으로 획득함으로써 보다 정확하고 신뢰할 수 있는 일기예보를 제공할 수 있습니다. 이 기사에서는 JavaScript 및 WebSocket 기술을 사용하여 효율적인 실시간 일기 예보 시스템을 구축하는 방법을 알아봅니다. 이 문서에서는 특정 코드 예제를 통해 구현 프로세스를 보여줍니다. 우리
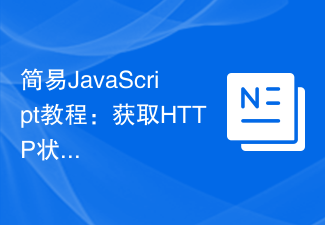
JavaScript 튜토리얼: HTTP 상태 코드를 얻는 방법, 특정 코드 예제가 필요합니다. 서문: 웹 개발에서는 서버와의 데이터 상호 작용이 종종 포함됩니다. 서버와 통신할 때 반환된 HTTP 상태 코드를 가져와서 작업의 성공 여부를 확인하고 다양한 상태 코드에 따라 해당 처리를 수행해야 하는 경우가 많습니다. 이 기사에서는 JavaScript를 사용하여 HTTP 상태 코드를 얻는 방법과 몇 가지 실용적인 코드 예제를 제공합니다. XMLHttpRequest 사용

사용법: JavaScript에서 insertBefore() 메서드는 DOM 트리에 새 노드를 삽입하는 데 사용됩니다. 이 방법에는 삽입할 새 노드와 참조 노드(즉, 새 노드가 삽입될 노드)라는 두 가지 매개 변수가 필요합니다.
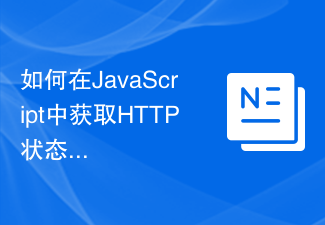
JavaScript에서 HTTP 상태 코드를 얻는 방법 소개: 프런트 엔드 개발에서 우리는 종종 백엔드 인터페이스와의 상호 작용을 처리해야 하며 HTTP 상태 코드는 매우 중요한 부분입니다. HTTP 상태 코드를 이해하고 얻는 것은 인터페이스에서 반환된 데이터를 더 잘 처리하는 데 도움이 됩니다. 이 기사에서는 JavaScript를 사용하여 HTTP 상태 코드를 얻는 방법을 소개하고 구체적인 코드 예제를 제공합니다. 1. HTTP 상태 코드란 무엇입니까? HTTP 상태 코드는 브라우저가 서버에 요청을 시작할 때 서비스가
