mysql에는 데이터가 어떻게 저장되나요?
mysql 데이터베이스가 많은 양의 데이터를 저장할 수 있다는 것은 모두가 알고 있지만, mysql에 데이터가 어떻게 저장되는지 알고 계시나요?
일반적으로 MySQL에 데이터를 저장하는 방법에는 동기 모드와 비동기 모드의 두 가지가 있습니다.
동기화 모드
동기화 모드는 SQL 문을 사용하여 데이터베이스에 데이터를 삽입합니다. 그러나 Scrapy의 파싱 속도는 MySQL의 로깅 속도보다 훨씬 빠르며, 파싱량이 많은 경우 MySQL의 로깅이 차단될 수 있습니다.
import MySQLdbclass MysqlPipeline(object): def __init__(self): self.conn = MySQLdb.connect('127.0.0.1','root','root','article_spider',charset="utf8",use_unicode=True) self.cursor = self.conn.cursor() def process_item(self, item, spider): insert_sql = """ insert into jobbole_article(title,create_date,url,url_object_id) VALUES (%s,%s,%s,%s) """ self.cursor.execute(insert_sql,(item["title"],item["create_date"],item["url"],item["url_object_id"])) self.conn.commit()
비동기 모드
동기 모드를 사용하면 차단이 발생할 수 있으므로 Twisted를 사용하여 MySQL을 저장하고 구문 분석할 수 있습니다. 단순한 실행 및 커밋 동기 작업 대신 비동기 작업을 수행합니다.
MySQL 구성과 관련하여 구성 파일에서 직접 데이터베이스를 구성할 수 있습니다.
MYSQL_HOST = "127.0.0.1" MYSQL_DBNAME = "article_spider" MYSQL_USER = "root"MYSQL_PASSWORD = "root"
설정의 구성에서 from_settings를 정의하여 설정 개체를 얻습니다. 설정 구성 파일에서 값을 직접 가져올 수 있는 파이프라인입니다.
Twisted에서 제공하는 비동기 컨테이너를 사용하여 MySQL에 연결합니다.
import MySQLdb import MySQLdb.cursorsfrom twisted.enterprise import adbapi
adbapi를 사용하면 mysqldb 비동기 작업의 일부 작업을 수행할 수 있습니다.
SQL 문에 커서를 사용하여 실행하고 submit
코드 부분 :
class MysqlTwistedPipline(object): def __init__(self,dbpool): self.dbpool = dbpool @classmethod def from_settings(cls,settings): dbparms = dict( host = settings["MYSQL_HOST"], db = settings["MYSQL_DBNAME"], user = settings["MYSQL_USER"], passwd = settings["MYSQL_PASSWORD"], charset = 'utf8', cursorclass = MySQLdb.cursors.DictCursor, use_unicode=True, ) dbpool = adbapi.ConnectionPool("MySQLdb",**dbparms) return cls(dbpool) def process_item(self, item, spider): #使用Twisted将mysql插入变成异步执行 #runInteraction可以将传入的函数变成异步的 query = self.dbpool.runInteraction(self.do_insert,item) #处理异常 query.addErrback(self.handle_error,item,spider) def handle_error(self,failure,item,spider): #处理异步插入的异常 print(failure) def do_insert(self,cursor,item): #会从dbpool取出cursor #执行具体的插入 insert_sql = """ insert into jobbole_article(title,create_date,url,url_object_id) VALUES (%s,%s,%s,%s) """ cursor.execute(insert_sql, (item["title"], item["create_date"], item["url"], item["url_object_id"])) #拿传进的cursor进行执行,并且自动完成commit操作
do_insert를 제외한 위 코드 부분은 재사용이 가능합니다.
위 내용은 mysql에는 데이터가 어떻게 저장되나요?의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










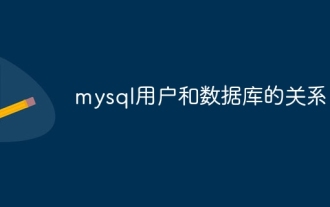
MySQL 데이터베이스에서 사용자와 데이터베이스 간의 관계는 권한과 테이블로 정의됩니다. 사용자는 데이터베이스에 액세스 할 수있는 사용자 이름과 비밀번호가 있습니다. 권한은 보조금 명령을 통해 부여되며 테이블은 Create Table 명령에 의해 생성됩니다. 사용자와 데이터베이스 간의 관계를 설정하려면 데이터베이스를 작성하고 사용자를 생성 한 다음 권한을 부여해야합니다.
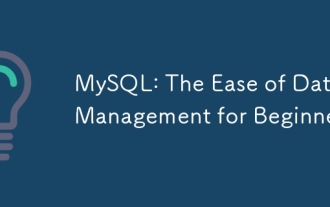
MySQL은 설치가 간단하고 강력하며 데이터를 쉽게 관리하기 쉽기 때문에 초보자에게 적합합니다. 1. 다양한 운영 체제에 적합한 간단한 설치 및 구성. 2. 데이터베이스 및 테이블 작성, 삽입, 쿼리, 업데이트 및 삭제와 같은 기본 작업을 지원합니다. 3. 조인 작업 및 하위 쿼리와 같은 고급 기능을 제공합니다. 4. 인덱싱, 쿼리 최적화 및 테이블 파티셔닝을 통해 성능을 향상시킬 수 있습니다. 5. 데이터 보안 및 일관성을 보장하기위한 지원 백업, 복구 및 보안 조치.
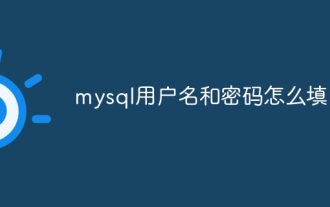
MySQL 사용자 이름 및 비밀번호를 작성하려면 : 1. 사용자 이름과 비밀번호를 결정합니다. 2. 데이터베이스에 연결; 3. 사용자 이름과 비밀번호를 사용하여 쿼리 및 명령을 실행하십시오.
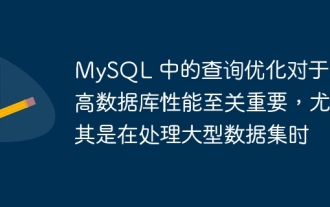
1. 올바른 색인을 사용하여 스캔 한 데이터의 양을 줄임으로써 데이터 검색 속도를 높이십시오. 테이블 열을 여러 번 찾으면 해당 열에 대한 인덱스를 만듭니다. 귀하 또는 귀하의 앱이 기준에 따라 여러 열에서 데이터가 필요한 경우 복합 인덱스 2를 만듭니다. 2. 선택을 피하십시오 * 필요한 열만 선택하면 모든 원치 않는 열을 선택하면 더 많은 서버 메모리를 선택하면 서버가 높은 부하 또는 주파수 시간으로 서버가 속도가 느려지며, 예를 들어 Creation_at 및 Updated_at 및 Timestamps와 같은 열이 포함되어 있지 않기 때문에 쿼리가 필요하지 않기 때문에 테이블은 선택을 피할 수 없습니다.
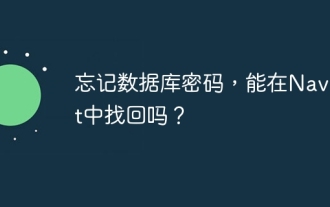
Navicat 자체는 데이터베이스 비밀번호를 저장하지 않으며 암호화 된 암호 만 검색 할 수 있습니다. 솔루션 : 1. 비밀번호 관리자를 확인하십시오. 2. Navicat의 "비밀번호 기억"기능을 확인하십시오. 3. 데이터베이스 비밀번호를 재설정합니다. 4. 데이터베이스 관리자에게 문의하십시오.
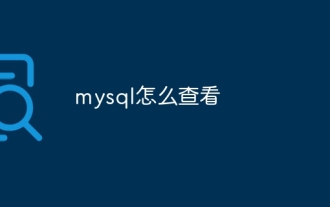
다음 명령으로 MySQL 데이터베이스를보십시오. 서버에 연결하십시오. mysql -u username -p password run show database; 기존의 모든 데이터베이스를 가져 오려는 명령 데이터베이스 선택 : 데이터베이스 이름 사용; 보기 테이블 : 테이블 표시; 테이블 구조보기 : 테이블 이름을 설명합니다. 데이터보기 : 테이블 이름에서 *를 선택하십시오.
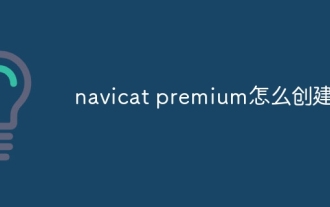
Navicat Premium을 사용하여 데이터베이스 생성 : 데이터베이스 서버에 연결하고 연결 매개 변수를 입력하십시오. 서버를 마우스 오른쪽 버튼으로 클릭하고 데이터베이스 생성을 선택하십시오. 새 데이터베이스의 이름과 지정된 문자 세트 및 Collation의 이름을 입력하십시오. 새 데이터베이스에 연결하고 객체 브라우저에서 테이블을 만듭니다. 테이블을 마우스 오른쪽 버튼으로 클릭하고 데이터 삽입을 선택하여 데이터를 삽입하십시오.
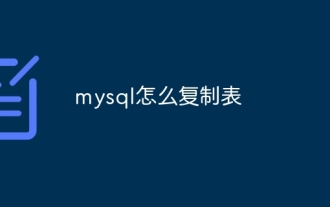
MySQL에서 테이블을 복사하려면 새 테이블을 만들고, 데이터를 삽입하고, 외래 키 설정, 인덱스 복사, 트리거, 저장된 절차 및 기능이 필요합니다. 특정 단계에는 다음이 포함됩니다 : 동일한 구조를 가진 새 테이블 작성. 원래 테이블의 데이터를 새 테이블에 삽입하십시오. 동일한 외래 키 제약 조건을 설정하십시오 (원래 테이블에 하나가있는 경우). 동일한 색인을 만듭니다. 동일한 트리거를 만듭니다 (원래 테이블에 하나가있는 경우). 동일한 저장된 절차 또는 기능을 만듭니다 (원래 테이블이 사용되는 경우).
