Laravel8 ES 패키징 및 사용 방법에 대한 자세한 설명
이 글은 Laravel8 ES 패키징 및 사용 방법을 포함하여 Laravel8에 대한 관련 지식을 소개합니다.
【관련 추천: laravel 비디오 튜토리얼】
composer 설치
composer require elasticsearch/elasticsearch
ES package
<?php namespace App\Es; use Elasticsearch\ClientBuilder; class MyEs { //ES客户端链接 private $client; /** * 构造函数 * MyElasticsearch constructor. */ public function __construct() { $this->client = ClientBuilder::create()->setHosts(['127.0.0.1:9200'])->build(); } /** * 判断索引是否存在 * @param string $index_name * @return bool|mixed|string */ public function exists_index($index_name = 'test_ik') { $params = [ 'index' => $index_name ]; try { return $this->client->indices()->exists($params); } catch (\Elasticsearch\Common\Exceptions\BadRequest400Exception $e) { $msg = $e->getMessage(); $msg = json_decode($msg,true); return $msg; } } /** * 创建索引 * @param string $index_name * @return array|mixed|string */ public function create_index($index_name = 'test_ik') { // 只能创建一次 $params = [ 'index' => $index_name, 'body' => [ 'settings' => [ 'number_of_shards' => 5, 'number_of_replicas' => 1 ] ] ]; try { return $this->client->indices()->create($params); } catch (\Elasticsearch\Common\Exceptions\BadRequest400Exception $e) { $msg = $e->getMessage(); $msg = json_decode($msg,true); return $msg; } } /** * 删除索引 * @param string $index_name * @return array */ public function delete_index($index_name = 'test_ik') { $params = ['index' => $index_name]; $response = $this->client->indices()->delete($params); return $response; } /** * 添加文档 * @param $id * @param $doc ['id'=>100, 'title'=>'phone'] * @param string $index_name * @param string $type_name * @return array */ public function add_doc($id,$doc,$index_name = 'test_ik',$type_name = 'goods') { $params = [ 'index' => $index_name, 'type' => $type_name, 'id' => $id, 'body' => $doc ]; $response = $this->client->index($params); return $response; } /** * 判断文档存在 * @param int $id * @param string $index_name * @param string $type_name * @return array|bool */ public function exists_doc($id = 1,$index_name = 'test_ik',$type_name = 'goods') { $params = [ 'index' => $index_name, 'type' => $type_name, 'id' => $id ]; $response = $this->client->exists($params); return $response; } /** * 获取文档 * @param int $id * @param string $index_name * @param string $type_name * @return array */ public function get_doc($id = 1,$index_name = 'test_ik',$type_name = 'goods') { $params = [ 'index' => $index_name, 'type' => $type_name, 'id' => $id ]; $response = $this->client->get($params); return $response; } /** * 更新文档 * @param int $id * @param string $index_name * @param string $type_name * @param array $body ['doc' => ['title' => '苹果手机iPhoneX']] * @return array */ public function update_doc($id = 1,$index_name = 'test_ik',$type_name = 'goods', $body=[]) { // 可以灵活添加新字段,最好不要乱添加 $params = [ 'index' => $index_name, 'type' => $type_name, 'id' => $id, 'body' => $body ]; $response = $this->client->update($params); return $response; } /** * 删除文档 * @param int $id * @param string $index_name * @param string $type_name * @return array */ public function delete_doc($id = 1,$index_name = 'test_ik',$type_name = 'goods') { $params = [ 'index' => $index_name, 'type' => $type_name, 'id' => $id ]; $response = $this->client->delete($params); return $response; } /** * 搜索文档 (分页,排序,权重,过滤) * @param string $index_name * @param string $type_name * @param array $body * $body = [ 'query' => [ 'match' => [ 'fang_name' => [ 'query' => $fangName ] ] ], 'highlight'=>[ 'fields'=>[ 'fang_name'=>[ 'pre_tags'=>[ '<span style="color: red">' ], 'post_tags'=>[ '</span>' ] ] ] ] ]; * @return array */ public function search_doc($index_name = "test_ik",$type_name = "goods",$body=[]) { $params = [ 'index' => $index_name, 'type' => $type_name, 'body' => $body ]; $results = $this->client->search($params); return $results; } }
데이터 테이블의 모든 데이터를 ES
public function esAdd() { $data = Good::get()->toArray(); $es = new MyEs(); if (!$es->exists_index('goods')) { //创建es索引,es的索引相当于MySQL的数据库 $es->create_index('goods'); } foreach ($data as $model) { $es->add_doc($model['id'], $model, 'goods', '_doc'); } }
에 추가하세요. MySQL에 추가되고 es에도 한 줄 추가
데이터베이스에 MySQL을 추가하는 논리 메서드에 코드를 직접 추가
//添加至MySQL $res=Good::insertGetId($arr); $es = new MyEs(); if (!$es->exists_index('goods')) { $es->create_index('goods'); } //添加至es $es->add_doc($res, $arr, 'goods', '_doc'); return $res;
MySQL 데이터를 수정하면 es의 데이터도 업데이트됨
데이터를 수정하는 MySQL의 로직 그 방법에는 ES를 통해 검색 기능을 구현할 수 있습니다
//修改MySQL的数据 $res=Good::where('id',$id)->update($arr); $es = new MyEs(); if (!$es->exists_index('goods')) { $es->create_index('goods'); } //修改es的数据 $es->update_doc($id, 'goods', '_doc',['doc'=>$arr]); return $res;
추가로 es 페이징 검색도 추가
위챗 애플릿에서 사용한다면 풀업 하단 이벤트를 이용하면 됩니다
이것 function은 위의 코드를 추가하여 구현한 것입니다.
1. 프론트엔드 애플릿이 전달한 현재 페이지를 받습니다.
2. es 패키지 클래스의 검색 메소드를 호출할 때 두 개의 매개변수를 추가합니다. es 패키지 클래스의 검색 방법 두 가지 형식 매개변수
검색 후 검색 값이 강조 표시됩니다
WeChat 애플릿에서 사용하면 레이블과 값이 함께 페이지에 직접 출력됩니다. 리치 텍스트를 구문 분석할 수 있습니다. 하이라이트 효과를 얻기 위해 라벨을 형식으로 변환하세요
public function search() { //获取搜索值 $search = \request()->get('search'); if (!empty($search)) { $es = new MyEs(); $body = [ 'query' => [ 'match' => [ 'title' => [ 'query' => $search ] ] ], 'highlight'=>[ 'fields'=>[ 'title'=>[ 'pre_tags'=>[ '<span style="color: red">' ], 'post_tags'=>[ '</span>' ] ] ] ] ]; $res = $es->search_doc('goods', '_doc', $body); $data = array_column($res['hits']['hits'], '_source'); foreach ($data as $key=>&$v){ $v['title'] = $res['hits']['hits'][$key]['highlight']['title'][0]; } unset($v); return $data; } $data = Good::get(); return $data; }
위 내용은 Laravel8 ES 패키징 및 사용 방법에 대한 자세한 설명의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










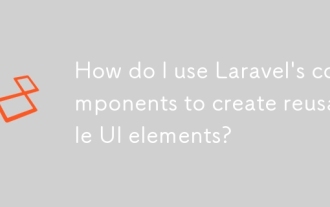
이 기사는 구성 요소를 사용하여 Laravel에서 재사용 가능한 UI 요소를 작성하고 사용자 정의하여 조직을위한 모범 사례를 제공하고 패키지 강화를 제안합니다.
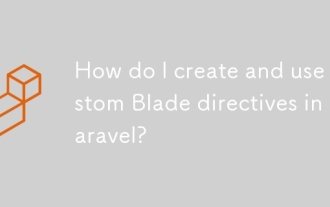
이 기사는 Laravel에서 사용자 정의 블레이드 지시문을 만들고 사용하여 템플릿을 향상시키는 것에 대해 설명합니다. 지침 정의, 템플릿에서이를 사용하고 대규모 프로젝트에서 관리하고 개선 된 코드 재사용 성 및 R과 같은 이점을 강조합니다.
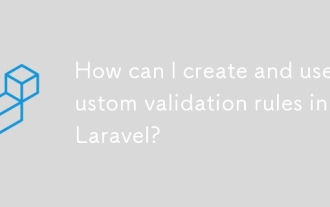
이 기사에서는 Laravel에서 사용자 정의 검증 규칙을 작성하고 사용하여이를 정의하고 구현하는 단계를 제공합니다. 재사용 성과 특이성과 같은 이점을 강조하고 Laravel의 검증 시스템을 확장하는 방법을 제공합니다.
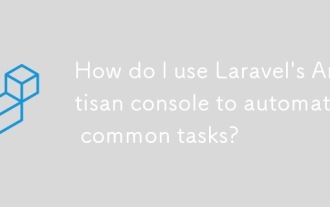
Laravel의 Artisan Console은 코드 생성, 마이그레이션 실행 및 스케줄링과 같은 작업을 자동화합니다. 주요 명령에는 Make : Controller, Migrate 및 DB : SEED가 포함됩니다. 특정 요구에 대해 사용자 정의 명령을 작성할 수 있으며 워크 플로 효율 향상.
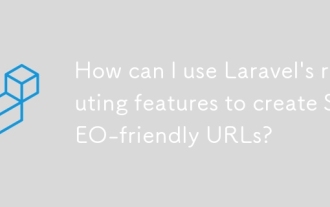
이 기사는 Laravel의 라우팅을 사용하여 SEO 친화적 인 URL을 생성, 모범 사례, 표준 URL 및 SEO 최적화 도구를 다루는 것에 대해 설명합니다.

Django와 Laravel은 모두 풀 스택 프레임 워크입니다. Django는 Python 개발자 및 복잡한 비즈니스 논리에 적합한 반면 Laravel은 PHP 개발자 및 우아한 구문에 적합합니다. 1. Django는 파이썬을 기반으로하며 빠른 개발 및 높은 동시성에 적합한 "배터리 완성"철학을 따릅니다. 2. Laravel은 PHP를 기반으로하며 개발자 경험을 강조하며 중소형 프로젝트에 적합합니다.
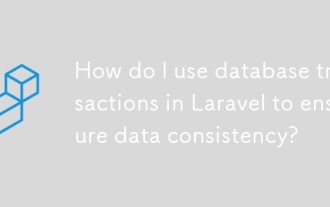
이 기사에서는 Laravel에서 데이터베이스 트랜잭션을 사용하여 데이터 일관성을 유지하고 DB Facade 및 Eloquent 모델, 모범 사례, 예외 처리 및 트랜잭션을 모니터링 및 디버깅하기위한 도구를 자세히 설명하는 데이터 일관성을 유지합니다.
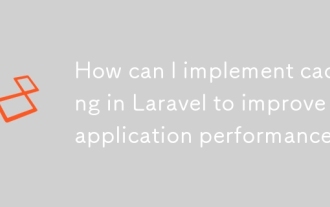
이 기사에서는 캐시 페이스, 캐시 태그 및 원자 연산을 사용하여 성능을 높이고 구성을 다루기 위해 Laravel에서 캐싱 구현에 대해 논의합니다. 또한 캐시 구성에 대한 모범 사례를 간략하게 설명하고 캐시에 대한 데이터 유형을 제안합니다.
