PHP와 SOAP를 사용하여 데이터를 압축 및 압축 해제하는 방법
PHP 및 SOAP를 사용하여 데이터를 압축 및 압축 해제하는 방법
소개:
현대 인터넷 응용 프로그램에서 데이터 전송은 매우 일반적인 작업입니다. 그러나 인터넷 응용 프로그램의 지속적인 개발로 인해 데이터 양이 증가하고 전송 속도가 빨라집니다. 요구 사항, 데이터 압축 및 압축 해제 기술의 합리적인 사용이 매우 중요한 주제가 되었습니다. PHP 개발에서는 SOAP(Simple Object Access Protocol) 프로토콜을 사용하여 데이터를 압축하고 압축을 풀 수 있습니다. 이 기사에서는 PHP와 SOAP를 사용하여 데이터를 압축 및 압축 해제하는 방법을 소개하고 코드 예제를 제공합니다.
1. SOAP 소개
SOAP는 RPC(원격 프로시저 호출) 및 네트워크 서비스 게시에 사용되는 XML 기반 프로토콜입니다. HTTP 및 기타 프로토콜을 전송 계층으로 사용하여 서로 다른 시스템 간에 통신합니다. SOAP는 데이터의 직렬화 및 역직렬화를 지원하므로 데이터를 압축하고 압축을 풀 수 있습니다.
2. PHP에서 SOAP 확장 사용
PHP에서는 SOAP 확장을 사용하여 SOAP 프로토콜의 기능을 구현할 수 있습니다. 먼저, PHP에 SOAP 확장이 설치되어 있는지 확인해야 합니다. 다음 코드를 사용하여 SOAP 확장이 설치되었는지 확인할 수 있습니다.
<?php if (extension_loaded('soap')) { echo 'SOAP extension is loaded'; } else { echo 'SOAP extension is not loaded'; }
출력 결과가 "SOAP 확장이 로드되었습니다"이면 SOAP 확장이 설치되었음을 의미합니다.
다음으로 SOAP 클라이언트와 서버를 사용하여 데이터를 압축하고 압축을 풀어야 합니다.
3. SOAP를 사용하여 데이터 압축 달성
다음은 SOAP를 사용하여 데이터 압축을 달성하기 위한 샘플 코드입니다.
<?php // 定义需要压缩的数据 $data = 'Hello, World!'; // 创建SOAP客户端 $client = new SoapClient(null, array( 'compression' => SOAP_COMPRESSION_ACCEPT | SOAP_COMPRESSION_GZIP )); // 调用压缩方法 $compressed_data = $client->compressData($data); // 输出压缩后的数据 echo 'Compressed Data: ' . $compressed_data; ?> <?xml version="1.0" encoding="UTF-8"?> <definitions xmlns:soap="http://schemas.xmlsoap.org/wsdl/soap/" xmlns="http://schemas.xmlsoap.org/wsdl/" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:soapenc="http://schemas.xmlsoap.org/soap/encoding/" xmlns:wsdl="http://schemas.xmlsoap.org/wsdl/" xmlns:tns="urn:compress-service" name="compressService" targetNamespace="urn:compress-service"> <types> <xsd:schema targetNamespace="urn:compress-service"></xsd:schema> </types> <portType name="compress"> <operation name="compressData"> <input message="tns:compressDataRequest"></input> <output message="tns:compressDataResponse"></output> </operation> </portType> <binding name="compressBinding" type="tns:compress"> <soap:binding style="rpc" transport="http://schemas.xmlsoap.org/soap/http"></soap:binding> <operation name="compressData"> <soap:operation soapAction="urn:compressData"></soap:operation> <input> <soap:body use="encoded" namespace="urn:compress-service" encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"></soap:body> </input> <output> <soap:body use="encoded" namespace="urn:compress-service" encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"></soap:body> </output> </operation> </binding> <service name="compressService"> <port name="compressPort" binding="tns:compressBinding"> <soap:address location="http://example.com/compress-service"></soap:address> </port> </service> </definitions>
위 코드에서는 먼저 압축해야 할 데이터를 정의한 다음 SOAP 클라이언트를 생성하고 SOAP 옵션의 압축을 설정하고 마지막으로 압축 방법이 호출됩니다. 서버 측에서는 압축 요청을 처리하기 위해 해당 SOAP 서비스를 제공해야 합니다. 구체적인 SOAP 서비스 정의는 위의 샘플 코드를 참조하세요.
4. SOAP를 사용하여 데이터 압축 해제
다음은 SOAP를 사용하여 데이터 압축을 풀기 위한 샘플 코드입니다.
<?php // 定义需要解压缩的数据 $compressed_data = 'compressed data'; // 创建SOAP客户端 $client = new SoapClient(null, array( 'compression' => SOAP_COMPRESSION_ACCEPT | SOAP_COMPRESSION_GZIP )); // 调用解压缩方法 $data = $client->decompressData($compressed_data); // 输出解压缩后的数据 echo 'Decompressed Data: ' . $data; ?> <?xml version="1.0" encoding="UTF-8"?> <definitions xmlns:soap="http://schemas.xmlsoap.org/wsdl/soap/" xmlns="http://schemas.xmlsoap.org/wsdl/" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:soapenc="http://schemas.xmlsoap.org/soap/encoding/" xmlns:wsdl="http://schemas.xmlsoap.org/wsdl/" xmlns:tns="urn:compress-service" name="compressService" targetNamespace="urn:compress-service"> <types> <xsd:schema targetNamespace="urn:compress-service"></xsd:schema> </types> <portType name="compress"> <operation name="decompressData"> <input message="tns:decompressDataRequest"></input> <output message="tns:decompressDataResponse"></output> </operation> </portType> <binding name="compressBinding" type="tns:compress"> <soap:binding style="rpc" transport="http://schemas.xmlsoap.org/soap/http"></soap:binding> <operation name="decompressData"> <soap:operation soapAction="urn:decompressData"></soap:operation> <input> <soap:body use="encoded" namespace="urn:compress-service" encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"></soap:body> </input> <output> <soap:body use="encoded" namespace="urn:compress-service" encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"></soap:body> </output> </operation> </binding> <service name="compressService"> <port name="compressPort" binding="tns:compressBinding"> <soap:address location="http://example.com/compress-service"></soap:address> </port> </service> </definitions>
데이터 압축과 유사하게 먼저 압축을 풀어야 하는 데이터를 정의한 다음 SOAP 클라이언트를 생성하고 SOAP 압축 옵션이 선택되고 마지막으로 압축 해제 방법이 호출됩니다. 서버 측에서는 압축 해제 요청을 처리하기 위해 해당 SOAP 서비스를 제공해야 합니다. 구체적인 SOAP 서비스 정의는 위의 샘플 코드를 참조하세요.
요약:
이 글에서는 PHP와 SOAP를 사용하여 데이터를 압축하고 압축을 푸는 방법을 소개합니다. SOAP 프로토콜의 지원을 통해 PHP 개발에서 데이터 압축 및 압축 해제 기술을 쉽게 사용할 수 있습니다. SOAP를 사용하면 데이터 전송량을 효과적으로 줄이고 전송 효율성을 높일 수 있어 네트워크 개발 및 빅데이터 전송에 매우 유용합니다. 이 기사가 도움이 되기를 바랍니다.
위 내용은 PHP와 SOAP를 사용하여 데이터를 압축 및 압축 해제하는 방법의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










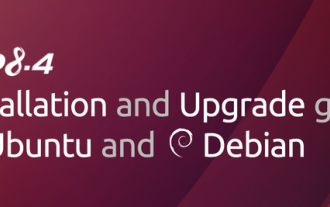
PHP 8.4는 상당한 양의 기능 중단 및 제거를 통해 몇 가지 새로운 기능, 보안 개선 및 성능 개선을 제공합니다. 이 가이드에서는 Ubuntu, Debian 또는 해당 파생 제품에서 PHP 8.4를 설치하거나 PHP 8.4로 업그레이드하는 방법을 설명합니다.
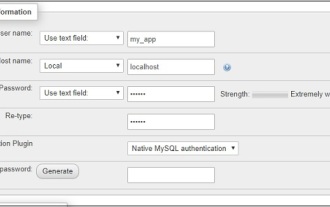
CakePHP에서 데이터베이스 작업은 매우 쉽습니다. 이번 장에서는 CRUD(생성, 읽기, 업데이트, 삭제) 작업을 이해하겠습니다.
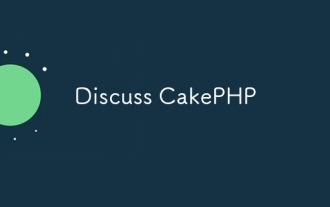
CakePHP는 PHP용 오픈 소스 프레임워크입니다. 이는 애플리케이션을 훨씬 쉽게 개발, 배포 및 유지 관리할 수 있도록 하기 위한 것입니다. CakePHP는 강력하고 이해하기 쉬운 MVC와 유사한 아키텍처를 기반으로 합니다. 모델, 뷰 및 컨트롤러 gu
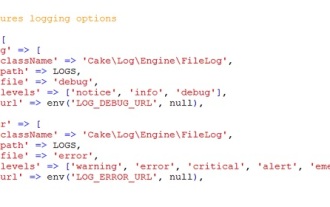
CakePHP에 로그인하는 것은 매우 쉬운 작업입니다. 한 가지 기능만 사용하면 됩니다. cronjob과 같은 백그라운드 프로세스에 대해 오류, 예외, 사용자 활동, 사용자가 취한 조치를 기록할 수 있습니다. CakePHP에 데이터를 기록하는 것은 쉽습니다. log() 함수는 다음과 같습니다.
