Go 언어의 성능 테스트를 위한 측정항목
In Go 언어 성능 테스트에는 다음을 포함한 공통 측정항목이 사용됩니다. 처리량(TPS): 동시 요청을 처리하는 애플리케이션의 능력을 반영하여 단위 시간당 처리된 요청 수를 측정합니다. 응답 시간(RT): 요청을 보낸 후 응답을 받는 데 걸리는 시간으로, 사용자 경험과 애플리케이션 민감도를 측정합니다. 동시성(C): 동시에 처리되는 요청 수로, 병렬 작업을 처리하는 애플리케이션의 능력을 반영합니다. 리소스 소비(M): 애플리케이션에서 소비하는 시스템 리소스로, 애플리케이션이 리소스를 효율적으로 사용하고 있는지 확인하는 데 도움이 됩니다. 오류율(E): 요청을 처리하는 동안 발생한 오류 수로, 애플리케이션의 안정성과 신뢰성을 측정합니다.
Go 언어의 성능 테스트를 위한 메트릭
Go 언어로 성능 테스트를 수행할 때 애플리케이션 성능을 깊이 이해하려면 적절한 메트릭을 사용하는 것이 중요합니다. 다음은 몇 가지 일반적인 측정항목과 그 의미입니다.
처리량(TPS)
- 은 단위 시간당 처리된 요청 수를 측정합니다.
- 애플리케이션의 전체 용량과 동시 요청 처리 능력을 반영합니다.
응답 시간(RT)
- 요청을 보낸 후 응답을 받는 데 걸리는 시간입니다.
- 사용자 경험 및 애플리케이션 민감도 측정.
동시성(C)
- 동시에 처리되는 요청 수입니다.
- 병렬 작업을 처리하는 애플리케이션의 능력을 반영합니다.
리소스 소비(M)
- CPU, 메모리, 네트워크 대역폭 등 애플리케이션에서 소비하는 시스템 리소스입니다.
- 애플리케이션이 리소스를 효율적으로 활용하고 있는지 확인하는 데 도움이 됩니다.
오류율(E)
- 요청을 처리하는 동안 발생한 오류 수입니다.
- 애플리케이션 안정성과 신뢰성을 측정합니다.
실제 사례
다음은 Go 언어에서 성능 테스트를 위해 이러한 메트릭을 사용하는 예입니다.
import ( "context" "fmt" "net/http" "sync/atomic" "testing" "time" ) func TestPerformance(t *testing.T) { // 计数器 var totalRequests, totalTPS, totalRT int64 var maxConcurrency int32 // 创建HTTP服务器 server := http.Server{ Addr: ":8080", Handler: http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { // 处理请求 time.Sleep(time.Millisecond * 100) w.Write([]byte("Hello, world!")) }), } // 启动HTTP服务器 go server.ListenAndServe() // 启动性能测试 for i := 0; i < 10000; i++ { go func() { // 发起HTTP请求 resp, err := http.Get("http://localhost:8080") if err != nil { return } resp.Body.Close() // 更新计数器 atomic.AddInt64(&totalRequests, 1) atomic.AddInt64(&totalRT, time.Since(time.Now()).Nanoseconds()) if currentConcurrency := atomic.AddInt32(&maxConcurrency, 1); currentConcurrency > maxConcurrency { maxConcurrency = currentConcurrency } atomic.AddInt32(&maxConcurrency, -1) }() } // 停止性能测试 time.Sleep(time.Second * 10) server.Shutdown(context.Background()) // 计算度量标准 averageRT := float64(totalRT) / float64(totalRequests) / 1000000.0 averageTPS := float64(totalRequests) / float64(time.Second * 10) // 打印结果 fmt.Printf("Total requests: %d\n", totalRequests) fmt.Printf("Average response time: %.2f ms\n", averageRT) fmt.Printf("Average TPS: %.2f\n", averageTPS) fmt.Printf("Maximum concurrency: %d\n", maxConcurrency) }
위 내용은 Go 언어의 성능 테스트를 위한 측정항목의 상세 내용입니다. 자세한 내용은 PHP 중국어 웹사이트의 기타 관련 기사를 참조하세요!

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

AI Hentai Generator
AI Hentai를 무료로 생성하십시오.

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










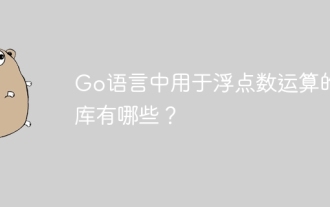
Go Language의 부동 소수점 번호 작동에 사용되는 라이브러리는 정확도를 보장하는 방법을 소개합니다.
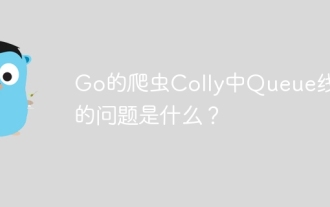
Go Crawler Colly의 대기열 스레딩 문제는 Colly Crawler 라이브러리를 GO 언어로 사용하는 문제를 탐구합니다. � ...
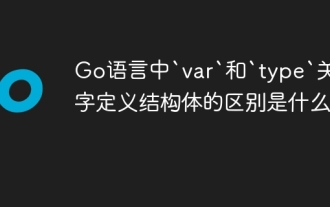
GO 언어에서 구조를 정의하는 두 가지 방법 : VAR과 유형 키워드의 차이. 구조를 정의 할 때 Go Language는 종종 두 가지 다른 글쓰기 방법을 본다 : 첫째 ...
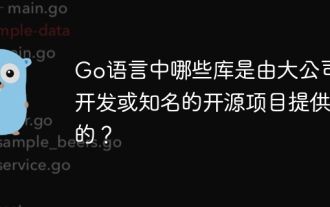
GO의 어떤 라이브러리가 대기업이나 잘 알려진 오픈 소스 프로젝트에서 개발 했습니까? GO에 프로그래밍 할 때 개발자는 종종 몇 가지 일반적인 요구를 만납니다.
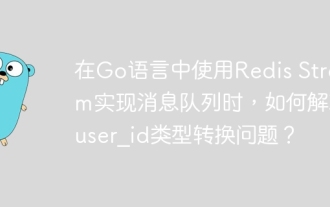
Go Language에서 메시지 대기열을 구현하기 위해 Redisstream을 사용하는 문제는 Go Language와 Redis를 사용하는 것입니다 ...
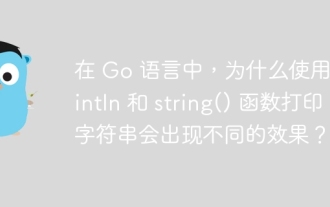
Go Language의 문자열 인쇄의 차이 : println 및 String () 함수 사용 효과의 차이가 진행 중입니다 ...
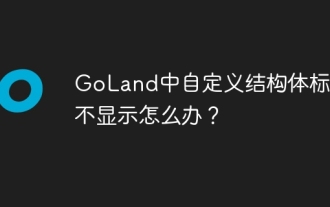
골란드의 사용자 정의 구조 레이블이 표시되지 않으면 어떻게해야합니까? Go Language 개발을 위해 Goland를 사용할 때 많은 개발자가 사용자 정의 구조 태그를 만날 것입니다 ...
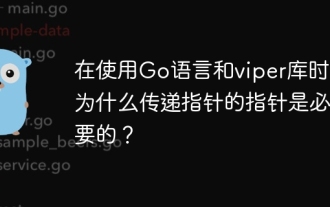
Go Pointer Syntax 및 Viper Library 사용의 문제 해결 GO 언어로 프로그래밍 할 때 특히 포인터의 구문 및 사용법을 이해하는 것이 중요합니다.
