자주 사용하는 JavaScript WEB 운용방법_javascript 기술 공유
数组方法集
Angela.array = { //# 数组方法 // index, 返回位置! 不存在则返回 -1; index: function (t, arr) { //# 返回当前值所在数组的位置 if (arr.indexOf) { return arr.indexOf(t); } for (var i = arr.length ; i--;) { if (arr[i] === t) { return i * 1; } }; return -1; } //返回对象 的 键值! 返回值 类型为数组。 , getKey: function (data) { //# 返回对象所有的键值 var arr = [] , k ; for (k in data) { arr.push(k); }; return arr; } //从数组中 随机取出 一个值 , random: function (arrays) { //# 从数组中 随机取出 一个值 arrays = arrays || []; var len = arrays.length , index = Tydic.math.randInt(0, len - 1) ; return arrays[index] || ''; } // 一维 数组去重 , unique: function (array) { //#一维数组去重 array = array || []; for (var i = 0, len = array.length; i < len; i++) { for (var j = i + 1; j < array.length; j++) { if (array[i] === array[j]) { array.splice(j, 1); j--; } } } return array; } // max , 数组中最大的项 , max: function (array) {//#求数组中最大的项 return Math.max.apply(null, array); } // min , 数组中最小的项 , min: function (array) { //#求数组中最小的项 return Math.min.apply(null, array); } // remove , 移除 , remove: function (array, value) { //#移除数组中某值 var length = array.length; while (length--) { if (value === array[length]) { array.splice(length, 1); } } return array; } //清空数组 , empty: function (array) { //# 清空数组 (array || []).length = 0; return array; } // removeAt ,删除指定位置的 值 //@index , 索引. 不传递 index ,会删除第一个 , removeAt: function (array, index) { //#删除数组中 指定位置的值 array.splice(index, 1); return array; } //打乱数组排序 , shuffle: function (arr) { //#打乱数组排序 var array = (arr || []).concat() , length = array.length , i = length //遍历 , tmp = null // 临时 , rand = Tydic.math.randInt //位置 , pos = 0 ; while (i--) { pos = rand(0, length); //交换随机位置 tmp = array[pos]; array[pos] = array[i]; array[i] = tmp; } return array; } };
cookie方法集
Angela.cookie = { //# Cookie // 浏览器是够支持 cookie enable: !!navigator.cookieEnabled //读取COOKIE , get: function (name) { //#读取 cookie var reg = new RegExp("(^| )" + name + "(?:=([^;]*))?(;|$)") , val = document.cookie.match(reg) ; return val ? (val[2] ? unescape(val[2]) : "") : ''; } //写入COOKIES , set: function (name, value, expires, path, domain, secure) { //# 写入 cookie var exp = new Date() , expires = arguments[2] || null , path = arguments[3] || "/" , domain = arguments[4] || null , secure = arguments[5] || false ; expires ? exp.setMinutes(exp.getMinutes() + parseInt(expires)) : ""; document.cookie = name + '=' + escape(value) + (expires ? ';expires=' + exp.toGMTString() : '') + (path ? ';path=' + path : '') + (domain ? ';domain=' + domain : '') + (secure ? ';secure' : ''); } //删除cookie , del: function (name, path, domain, secure) { //#删除 cookie var value = $getCookie(name); if (value != null) { var exp = new Date(); exp.setMinutes(exp.getMinutes() - 1000); path = path || "/"; document.cookie = name + '=;expires=' + exp.toGMTString() + (path ? ';path=' + path : '') + (domain ? ';domain=' + domain : '') + (secure ? ';secure' : ''); } } };
url方法集
Angela.url = { //#URL //参数:变量名,url为空则表从当前页面的url中取 getQuery: function (name, url) { var u = arguments[1] || window.location.search , reg = new RegExp("(^|&)" + name + "=([^&]*)(&|$)") , r = u.substr(u.indexOf("?") + 1).match(reg) ; return r != null ? r[2] : ""; } , getHash: function (name, url) { //# 获取 hash值 var u = arguments[1] || location.hash; var reg = new RegExp("(^|&)" + name + "=([^&]*)(&|$)"); var r = u.substr(u.indexOf("#") + 1).match(reg); if (r != null) { return r[2]; } return ""; } , parse: function (url) { //# 解析URL var a = document.createElement('a'); url = url || document.location.href; a.href = url; return { source: url , protocol: a.protocol.replace(':', '') , host: a.hostname , port: a.port , query: a.search , file: (a.pathname.match(/([^\/?#]+)$/i) || [, ''])[1] , hash: a.hash.replace('#', '') , path: a.pathname.replace(/^([^\/])/, '/$1') , relative: (a.href.match(/tps?:\/\/[^\/]+(.+)/) || [, ''])[1] , segments: a.pathname.replace(/^\//, '').split('/') }; } };
正则表达式方法集
Angela.regExp = { //# 字符串匹配 //是否为 数字!整数,浮点数 isNum: function (num) { //# 是否为数组 return !isNaN(num); } , isEmail: function (mail) {//# 是否为 邮箱 return /^([a-z0-9]+[_\-\.]?)*[a-z0-9]+@([a-z0-9]+[_\-\.]?)*[a-z0-9]+\.[a-z]{2,5}$/i.test(mail); } , isIdCard: function (card) { //# 是否为 身份证 return /^(\d{14}|\d{17})(\d|[xX])$/.test(card); } , isMobile: function (mobile) { //# 是否为 手机 return /^0*1\d{10}$/.test(mobile); } , isQQ: function (qq) { //# 是否为 QQ return /^[1-9]\d{4,10}$/.test(qq); } , isTel: function (tel) { //# 是否为 电话 return /^\d{3,4}-\d{7,8}(-\d{1,6})?$/.text(tel); } , isUrl: function (url) { //# 是否为 URL return /https?:\/\/[a-z0-9\.\-]{1,255}\.[0-9a-z\-]{1,255}/i.test(url); } , isColor: function (color) { //# 是否为 16进制颜色 return /#([\da-f]{3}){1,2}$/i.test(color); } //@id : 身份证 , // @now : 当前时间 如:new Date('2013/12/12') , '2013/12/12' // @age : 允许的年龄 , isAdult: function (id, allowAge, now) { //# 是否年龄是否成年 var age = 0 // 用户 年月日 , nowDate = 0 //当前年月日 ; allowAge = parseFloat(allowAge) || 18; now = typeof now == 'string' ? new Date(now) : (now || new Date()); if (!this.isIdCard(id)) { return false; } //15位身份证 if (15 == id.length) { age = '19' + id.slice(6, 6); } else { age = id.slice(6, 14); } // 类型转换 整型 age = ~~age; nowDate = ~~(Tydic.date.format('YYYYMMDD', now)); //比较年龄 if (nowDate - age < allowAge * 1e4) { return false; } return true; } //浮点数 , isFloat: function (num) { //# 是否为 浮点数 return /^(([1-9]\d*)|(\d+\.\d+)|0)$/.test(num); } //正整数 , isInt: function (num) { //# 是否为 正整数 return /^[1-9]\d*$/.test(num); } //是否全为汉字 , isChinese: function (str) { //# 是否全为 汉字 return /^([\u4E00-\u9FA5]|[\uFE30-\uFFA0])+$/gi.test(str); } };
字符串方法集
Angela.string = { //# 字符串 codeHtml: function (content) { //# 转义 HTML 字符 return this.replace(content, { '&': "&" , '"': """ , "'": ''' , '<': "<" , '>': ">" , ' ': " " , '\t': "	" , '(': "(" , ')': ")" , '*': "*" , '+': "+" , ',': "," , '-': "-" , '.': "." , '/': "/" , '?': "?" , '\\': "\" , '\n': "<br>" }); } //重复字符串 , repeat: function (word, length, end) { //# 重复字符串 end = end || ''; //加在末位 length = ~~length; return new Array(length * 1 + 1).join(word) + '' + end; } //增加前缀 , addPre: function (pre, word, size) { //# 补齐。如给数字前 加 0 pre = pre || '0'; size = parseInt(size) || 0; word = String(word || ''); var length = Math.max(0, size - word.length); return this.repeat(pre, length, word); } //去除两边空格 , trim: function (text) { //# 去除两边空格 return (text || '').replace(/^\s+|\s$/, ''); } //字符串替换 , replace: function (str, re) { //# 字符串替换 str = str || ''; for (var key in re) { replace(key, re[key]); }; function replace(a, b) { var arr = str.split(a); str = arr.join(b); }; return str; } , xss: function (str, type) { //# XSS 转义 //空过滤 if (!str) { return str === 0 ? "0" : ""; } switch (type) { case "html": //过滤html字符串中的XSS return str.replace(/[&'"<>\/\\\-\x00-\x09\x0b-\x0c\x1f\x80-\xff]/g, function (r) { return "&#" + r.charCodeAt(0) + ";" }).replace(/ /g, " ").replace(/\r\n/g, "<br />").replace(/\n/g, "<br />").replace(/\r/g, "<br />"); break; case "htmlEp": //过滤DOM节点属性中的XSS return str.replace(/[&'"<>\/\\\-\x00-\x1f\x80-\xff]/g, function (r) { return "&#" + r.charCodeAt(0) + ";" }); break; case "url": //过滤url return escape(str).replace(/\+/g, "%2B"); break; case "miniUrl": return str.replace(/%/g, "%25"); break; case "script": return str.replace(/[\\"']/g, function (r) { return "\\" + r; }).replace(/%/g, "\\x25").replace(/\n/g, "\\n").replace(/\r/g, "\\r").replace(/\x01/g, "\\x01"); break; case "reg": return str.replace(/[\\\^\$\*\+\?\{\}\.\(\)\[\]]/g, function (a) { return "\\" + a; }); break; default: return escape(str).replace(/[&'"<>\/\\\-\x00-\x09\x0b-\x0c\x1f\x80-\xff]/g, function (r) { return "&#" + r.charCodeAt(0) + ";" }).replace(/ /g, " ").replace(/\r\n/g, "<br />").replace(/\n/g, "<br />").replace(/\r/g, "<br />"); break; } } // badword , 过滤敏感词 //@text : 要过滤的文本 , 类型 :字符串 //@words : 敏感词 ,类型,数组, 如 : ['你妹', '我丢' ,'我靠'] // 如果 用 正则匹配, text 长度 100万,words 100万,需要 4秒! , badWord: function (text, words) { //# 敏感词过滤 text = String(text || ''); words = words || []; var reg = new RegExp(words.join('|'), 'g') , _self = this; return text.replace(reg, function ($0) { var length = String($0 || '').length; return _self.repeat('*', length); }); } };
加密方法集
Angela.encrypt = { //# 加密 md5: function (words) { //# md5 哈希算法 /* * Crypto-JS 3.1.2 * http://code.google.com/p/crypto-js */ var CryptoJS = function (s, p) { var m = {}, l = m.lib = {}, n = function () { }, r = l.Base = { extend: function (b) { n.prototype = this; var h = new n; b && h.mixIn(b); h.hasOwnProperty("init") || (h.init = function () { h.$super.init.apply(this, arguments) }); h.init.prototype = h; h.$super = this; return h }, create: function () { var b = this.extend(); b.init.apply(b, arguments); return b }, init: function () { }, mixIn: function (b) { for (var h in b) b.hasOwnProperty(h) && (this[h] = b[h]); b.hasOwnProperty("toString") && (this.toString = b.toString) }, clone: function () { return this.init.prototype.extend(this) } }, q = l.WordArray = r.extend({ init: function (b, h) { b = this.words = b || []; this.sigBytes = h != p ? h : 4 * b.length }, toString: function (b) { return (b || t).stringify(this) }, concat: function (b) { var h = this.words, a = b.words, j = this.sigBytes; b = b.sigBytes; this.clamp(); if (j % 4) for (var g = 0; g < b; g++) h[j + g >>> 2] |= (a[g >>> 2] >>> 24 - 8 * (g % 4) & 255) << 24 - 8 * ((j + g) % 4); else if (65535 < a.length) for (g = 0; g < b; g += 4) h[j + g >>> 2] = a[g >>> 2]; else h.push.apply(h, a); this.sigBytes += b; return this }, clamp: function () { var b = this.words, h = this.sigBytes; b[h >>> 2] &= 4294967295 << 32 - 8 * (h % 4); b.length = s.ceil(h / 4) }, clone: function () { var b = r.clone.call(this); b.words = this.words.slice(0); return b }, random: function (b) { for (var h = [], a = 0; a < b; a += 4) h.push(4294967296 * s.random() | 0); return new q.init(h, b) } }), v = m.enc = {}, t = v.Hex = { stringify: function (b) { var a = b.words; b = b.sigBytes; for (var g = [], j = 0; j < b; j++) { var k = a[j >>> 2] >>> 24 - 8 * (j % 4) & 255; g.push((k >>> 4).toString(16)); g.push((k & 15).toString(16)) } return g.join("") }, parse: function (b) { for (var a = b.length, g = [], j = 0; j < a; j += 2) g[j >>> 3] |= parseInt(b.substr(j, 2), 16) << 24 - 4 * (j % 8); return new q.init(g, a / 2) } }, a = v.Latin1 = { stringify: function (b) { var a = b.words; b = b.sigBytes; for (var g = [], j = 0; j < b; j++) g.push(String.fromCharCode(a[j >>> 2] >>> 24 - 8 * (j % 4) & 255)); return g.join("") }, parse: function (b) { for (var a = b.length, g = [], j = 0; j < a; j++) g[j >>> 2] |= (b.charCodeAt(j) & 255) << 24 - 8 * (j % 4); return new q.init(g, a) } }, u = v.Utf8 = { stringify: function (b) { try { return decodeURIComponent(escape(a.stringify(b))) } catch (g) { throw Error("Malformed UTF-8 data"); } }, parse: function (b) { return a.parse(unescape(encodeURIComponent(b))) } }, g = l.BufferedBlockAlgorithm = r.extend({ reset: function () { this._data = new q.init; this._nDataBytes = 0 }, _append: function (b) { "string" == typeof b && (b = u.parse(b)); this._data.concat(b); this._nDataBytes += b.sigBytes }, _process: function (b) { var a = this._data, g = a.words, j = a.sigBytes, k = this.blockSize, m = j / (4 * k), m = b ? s.ceil(m) : s.max((m | 0) - this._minBufferSize, 0); b = m * k; j = s.min(4 * b, j); if (b) { for (var l = 0; l < b; l += k) this._doProcessBlock(g, l); l = g.splice(0, b); a.sigBytes -= j } return new q.init(l, j) }, clone: function () { var b = r.clone.call(this); b._data = this._data.clone(); return b }, _minBufferSize: 0 }); l.Hasher = g.extend({ cfg: r.extend(), init: function (b) { this.cfg = this.cfg.extend(b); this.reset() }, reset: function () { g.reset.call(this); this._doReset() }, update: function (b) { this._append(b); this._process(); return this }, finalize: function (b) { b && this._append(b); return this._doFinalize() }, blockSize: 16, _createHelper: function (b) { return function (a, g) { return (new b.init(g)).finalize(a) } }, _createHmacHelper: function (b) { return function (a, g) { return (new k.HMAC.init(b, g)).finalize(a) } } }); var k = m.algo = {}; return m }(Math); (function (s) { function p(a, k, b, h, l, j, m) { a = a + (k & b | ~k & h) + l + m; return (a << j | a >>> 32 - j) + k } function m(a, k, b, h, l, j, m) { a = a + (k & h | b & ~h) + l + m; return (a << j | a >>> 32 - j) + k } function l(a, k, b, h, l, j, m) { a = a + (k ^ b ^ h) + l + m; return (a << j | a >>> 32 - j) + k } function n(a, k, b, h, l, j, m) { a = a + (b ^ (k | ~h)) + l + m; return (a << j | a >>> 32 - j) + k } for (var r = CryptoJS, q = r.lib, v = q.WordArray, t = q.Hasher, q = r.algo, a = [], u = 0; 64 > u; u++) a[u] = 4294967296 * s.abs(s.sin(u + 1)) | 0; q = q.MD5 = t.extend({ _doReset: function () { this._hash = new v.init([1732584193, 4023233417, 2562383102, 271733878]) }, _doProcessBlock: function (g, k) { for (var b = 0; 16 > b; b++) { var h = k + b, w = g[h]; g[h] = (w << 8 | w >>> 24) & 16711935 | (w << 24 | w >>> 8) & 4278255360 } var b = this._hash.words, h = g[k + 0], w = g[k + 1], j = g[k + 2], q = g[k + 3], r = g[k + 4], s = g[k + 5], t = g[k + 6], u = g[k + 7], v = g[k + 8], x = g[k + 9], y = g[k + 10], z = g[k + 11], A = g[k + 12], B = g[k + 13], C = g[k + 14], D = g[k + 15], c = b[0], d = b[1], e = b[2], f = b[3], c = p(c, d, e, f, h, 7, a[0]), f = p(f, c, d, e, w, 12, a[1]), e = p(e, f, c, d, j, 17, a[2]), d = p(d, e, f, c, q, 22, a[3]), c = p(c, d, e, f, r, 7, a[4]), f = p(f, c, d, e, s, 12, a[5]), e = p(e, f, c, d, t, 17, a[6]), d = p(d, e, f, c, u, 22, a[7]), c = p(c, d, e, f, v, 7, a[8]), f = p(f, c, d, e, x, 12, a[9]), e = p(e, f, c, d, y, 17, a[10]), d = p(d, e, f, c, z, 22, a[11]), c = p(c, d, e, f, A, 7, a[12]), f = p(f, c, d, e, B, 12, a[13]), e = p(e, f, c, d, C, 17, a[14]), d = p(d, e, f, c, D, 22, a[15]), c = m(c, d, e, f, w, 5, a[16]), f = m(f, c, d, e, t, 9, a[17]), e = m(e, f, c, d, z, 14, a[18]), d = m(d, e, f, c, h, 20, a[19]), c = m(c, d, e, f, s, 5, a[20]), f = m(f, c, d, e, y, 9, a[21]), e = m(e, f, c, d, D, 14, a[22]), d = m(d, e, f, c, r, 20, a[23]), c = m(c, d, e, f, x, 5, a[24]), f = m(f, c, d, e, C, 9, a[25]), e = m(e, f, c, d, q, 14, a[26]), d = m(d, e, f, c, v, 20, a[27]), c = m(c, d, e, f, B, 5, a[28]), f = m(f, c, d, e, j, 9, a[29]), e = m(e, f, c, d, u, 14, a[30]), d = m(d, e, f, c, A, 20, a[31]), c = l(c, d, e, f, s, 4, a[32]), f = l(f, c, d, e, v, 11, a[33]), e = l(e, f, c, d, z, 16, a[34]), d = l(d, e, f, c, C, 23, a[35]), c = l(c, d, e, f, w, 4, a[36]), f = l(f, c, d, e, r, 11, a[37]), e = l(e, f, c, d, u, 16, a[38]), d = l(d, e, f, c, y, 23, a[39]), c = l(c, d, e, f, B, 4, a[40]), f = l(f, c, d, e, h, 11, a[41]), e = l(e, f, c, d, q, 16, a[42]), d = l(d, e, f, c, t, 23, a[43]), c = l(c, d, e, f, x, 4, a[44]), f = l(f, c, d, e, A, 11, a[45]), e = l(e, f, c, d, D, 16, a[46]), d = l(d, e, f, c, j, 23, a[47]), c = n(c, d, e, f, h, 6, a[48]), f = n(f, c, d, e, u, 10, a[49]), e = n(e, f, c, d, C, 15, a[50]), d = n(d, e, f, c, s, 21, a[51]), c = n(c, d, e, f, A, 6, a[52]), f = n(f, c, d, e, q, 10, a[53]), e = n(e, f, c, d, y, 15, a[54]), d = n(d, e, f, c, w, 21, a[55]), c = n(c, d, e, f, v, 6, a[56]), f = n(f, c, d, e, D, 10, a[57]), e = n(e, f, c, d, t, 15, a[58]), d = n(d, e, f, c, B, 21, a[59]), c = n(c, d, e, f, r, 6, a[60]), f = n(f, c, d, e, z, 10, a[61]), e = n(e, f, c, d, j, 15, a[62]), d = n(d, e, f, c, x, 21, a[63]); b[0] = b[0] + c | 0; b[1] = b[1] + d | 0; b[2] = b[2] + e | 0; b[3] = b[3] + f | 0 }, _doFinalize: function () { var a = this._data, k = a.words, b = 8 * this._nDataBytes, h = 8 * a.sigBytes; k[h >>> 5] |= 128 << 24 - h % 32; var l = s.floor(b / 4294967296); k[(h + 64 >>> 9 << 4) + 15] = (l << 8 | l >>> 24) & 16711935 | (l << 24 | l >>> 8) & 4278255360; k[(h + 64 >>> 9 << 4) + 14] = (b << 8 | b >>> 24) & 16711935 | (b << 24 | b >>> 8) & 4278255360; a.sigBytes = 4 * (k.length + 1); this._process(); a = this._hash; k = a.words; for (b = 0; 4 > b; b++) h = k[b], k[b] = (h << 8 | h >>> 24) & 16711935 | (h << 24 | h >>> 8) & 4278255360; return a }, clone: function () { var a = t.clone.call(this); a._hash = this._hash.clone(); return a } }); r.MD5 = t._createHelper(q); r.HmacMD5 = t._createHmacHelper(q) })(Math); return CryptoJS.MD5(words).toString(); } // sha1 , sha1: function (words) { //# sha1 哈希算法 var CryptoJS = function (e, m) { var p = {}, j = p.lib = {}, l = function () { }, f = j.Base = { extend: function (a) { l.prototype = this; var c = new l; a && c.mixIn(a); c.hasOwnProperty("init") || (c.init = function () { c.$super.init.apply(this, arguments) }); c.init.prototype = c; c.$super = this; return c }, create: function () { var a = this.extend(); a.init.apply(a, arguments); return a }, init: function () { }, mixIn: function (a) { for (var c in a) a.hasOwnProperty(c) && (this[c] = a[c]); a.hasOwnProperty("toString") && (this.toString = a.toString) }, clone: function () { return this.init.prototype.extend(this) } }, n = j.WordArray = f.extend({ init: function (a, c) { a = this.words = a || []; this.sigBytes = c != m ? c : 4 * a.length }, toString: function (a) { return (a || h).stringify(this) }, concat: function (a) { var c = this.words, q = a.words, d = this.sigBytes; a = a.sigBytes; this.clamp(); if (d % 4) for (var b = 0; b < a; b++) c[d + b >>> 2] |= (q[b >>> 2] >>> 24 - 8 * (b % 4) & 255) << 24 - 8 * ((d + b) % 4); else if (65535 < q.length) for (b = 0; b < a; b += 4) c[d + b >>> 2] = q[b >>> 2]; else c.push.apply(c, q); this.sigBytes += a; return this }, clamp: function () { var a = this.words, c = this.sigBytes; a[c >>> 2] &= 4294967295 << 32 - 8 * (c % 4); a.length = e.ceil(c / 4) }, clone: function () { var a = f.clone.call(this); a.words = this.words.slice(0); return a }, random: function (a) { for (var c = [], b = 0; b < a; b += 4) c.push(4294967296 * e.random() | 0); return new n.init(c, a) } }), b = p.enc = {}, h = b.Hex = { stringify: function (a) { var c = a.words; a = a.sigBytes; for (var b = [], d = 0; d < a; d++) { var f = c[d >>> 2] >>> 24 - 8 * (d % 4) & 255; b.push((f >>> 4).toString(16)); b.push((f & 15).toString(16)) } return b.join("") }, parse: function (a) { for (var c = a.length, b = [], d = 0; d < c; d += 2) b[d >>> 3] |= parseInt(a.substr(d, 2), 16) << 24 - 4 * (d % 8); return new n.init(b, c / 2) } }, g = b.Latin1 = { stringify: function (a) { var c = a.words; a = a.sigBytes; for (var b = [], d = 0; d < a; d++) b.push(String.fromCharCode(c[d >>> 2] >>> 24 - 8 * (d % 4) & 255)); return b.join("") }, parse: function (a) { for (var c = a.length, b = [], d = 0; d < c; d++) b[d >>> 2] |= (a.charCodeAt(d) & 255) << 24 - 8 * (d % 4); return new n.init(b, c) } }, r = b.Utf8 = { stringify: function (a) { try { return decodeURIComponent(escape(g.stringify(a))) } catch (c) { throw Error("Malformed UTF-8 data"); } }, parse: function (a) { return g.parse(unescape(encodeURIComponent(a))) } }, k = j.BufferedBlockAlgorithm = f.extend({ reset: function () { this._data = new n.init; this._nDataBytes = 0 }, _append: function (a) { "string" == typeof a && (a = r.parse(a)); this._data.concat(a); this._nDataBytes += a.sigBytes }, _process: function (a) { var c = this._data, b = c.words, d = c.sigBytes, f = this.blockSize, h = d / (4 * f), h = a ? e.ceil(h) : e.max((h | 0) - this._minBufferSize, 0); a = h * f; d = e.min(4 * a, d); if (a) { for (var g = 0; g < a; g += f) this._doProcessBlock(b, g); g = b.splice(0, a); c.sigBytes -= d } return new n.init(g, d) }, clone: function () { var a = f.clone.call(this); a._data = this._data.clone(); return a }, _minBufferSize: 0 }); j.Hasher = k.extend({ cfg: f.extend(), init: function (a) { this.cfg = this.cfg.extend(a); this.reset() }, reset: function () { k.reset.call(this); this._doReset() }, update: function (a) { this._append(a); this._process(); return this }, finalize: function (a) { a && this._append(a); return this._doFinalize() }, blockSize: 16, _createHelper: function (a) { return function (c, b) { return (new a.init(b)).finalize(c) } }, _createHmacHelper: function (a) { return function (b, f) { return (new s.HMAC.init(a, f)).finalize(b) } } }); var s = p.algo = {}; return p }(Math); (function () { var e = CryptoJS, m = e.lib, p = m.WordArray, j = m.Hasher, l = [], m = e.algo.SHA1 = j.extend({ _doReset: function () { this._hash = new p.init([1732584193, 4023233417, 2562383102, 271733878, 3285377520]) }, _doProcessBlock: function (f, n) { for (var b = this._hash.words, h = b[0], g = b[1], e = b[2], k = b[3], j = b[4], a = 0; 80 > a; a++) { if (16 > a) l[a] = f[n + a] | 0; else { var c = l[a - 3] ^ l[a - 8] ^ l[a - 14] ^ l[a - 16]; l[a] = c << 1 | c >>> 31 } c = (h << 5 | h >>> 27) + j + l[a]; c = 20 > a ? c + ((g & e | ~g & k) + 1518500249) : 40 > a ? c + ((g ^ e ^ k) + 1859775393) : 60 > a ? c + ((g & e | g & k | e & k) - 1894007588) : c + ((g ^ e ^ k) - 899497514); j = k; k = e; e = g << 30 | g >>> 2; g = h; h = c } b[0] = b[0] + h | 0; b[1] = b[1] + g | 0; b[2] = b[2] + e | 0; b[3] = b[3] + k | 0; b[4] = b[4] + j | 0 }, _doFinalize: function () { var f = this._data, e = f.words, b = 8 * this._nDataBytes, h = 8 * f.sigBytes; e[h >>> 5] |= 128 << 24 - h % 32; e[(h + 64 >>> 9 << 4) + 14] = Math.floor(b / 4294967296); e[(h + 64 >>> 9 << 4) + 15] = b; f.sigBytes = 4 * e.length; this._process(); return this._hash }, clone: function () { var e = j.clone.call(this); e._hash = this._hash.clone(); return e } }); e.SHA1 = j._createHelper(m); e.HmacSHA1 = j._createHmacHelper(m) })(); return CryptoJS.SHA1(words).toString(); } // time33 哈希 , time33: function (words) { //# time33 哈希算法 words = words || ''; //哈希time33算法 for (var i = 0, len = words.length, hash = 5381; i < len; ++i) { hash += (hash << 5) + words.charAt(i).charCodeAt(); }; return hash & 0x7fffffff; } }
日期方法集
Angela.date = { //# 日期时间 //@s : 开始时间 //@e : 结束时间 //@n : 当前时间 , n 的格式为 毫秒数 isInArea: function (s, e, n) { //# 判断时间区域 var start = this.parse(s), end = this.parse(e), now = parseFloat(n) || new Date() ; start = Math.min(start, end); end = Math.max(start, end); return now >= start && now <= end ? true : false; } //把 字符窜转化为 毫秒 //@date : 2013-03-02 1:2:2 , parse: function (date) { //# 格式化时间 return Date.parse(date); //.replace(/-/g, '/') } //@time , 时间 , 如 new Date('2013/11/10 0:12:12') //@pre , 星期的 前缀,如:周 ,星期 //@ nums ,如:一二三四五六日 , getWeek: function (time, pre, nums) { //# 获取星期几 time = typeof time == 'string' ? this.parse(time) : (time || new Date()); pre = pre || '星期'; //周 nums = '日一二三四五六'; return pre + nums[time.getDay()]; } //@formatType : YYYY, YY, MM //@ time : new Date('2013/11/12') //@weeks : 日一二三四五六 , format: function (formatType, time, weeks) { //格式化输出时间 var pre = '0' ; formatType = formatType || 'YYYY-MM-DD' weeks = weeks || '日一二三四五六'; time = time || new Date(); //格式化时间 return (formatType || '') .replace(/yyyy|YYYY/g, time.getFullYear()) .replace(/yy|YY/g, Tydic.string.addPre(pre, time.getFullYear() % 100), 2) .replace(/mm|MM/g, Tydic.string.addPre(pre, time.getMonth() + 1, 2)) .replace(/m|M/g, time.getMonth() + 1) .replace(/dd|DD/g, Tydic.string.addPre(pre, time.getDate(), 2)) .replace(/d|D/g, time.getDate()) .replace(/hh|HH/g, Tydic.string.addPre(pre, time.getHours(), 2)) .replace(/h|H/g, time.getHours()) .replace(/ii|II/g, Tydic.string.addPre(pre, time.getMinutes(), 2)) .replace(/i|I/g, time.getMinutes()) .replace(/ss|SS/g, Tydic.string.addPre(pre, time.getSeconds(), 2)) .replace(/s|S/g, time.getSeconds()) .replace(/w/g, time.getDay()) .replace(/W/g, weeks[time.getDay()]) ; } //倒计时 , countDown: function (opt) { //# 倒计时 var option = { nowTime: 0 // 当前时间, ,2013/02/01 18:30:30 , endTime: 0 //截止时间 ,2013/02/01 18:30:30 , interval: 1 //间隔回调时间,秒 , called: function (day, hour, second, minute) { }//每次回调 , finaled: function () { } //完成后回调 } , opts = {} , timer = null ; opts = Tydic.extend(option, opt); //当前时间 if (!opts.nowTime) { opts.nowTime = (new Date()).getTime(); } else { opts.nowTime = this.parse(opts.nowTime); } //当前时间 if (!opts.endTime) { opts.endTime = (new Date()).getTime(); } else { opts.endTime = this.parse(opts.endTime); } timer = setInterval(loop, opts.interval * 1e3); // 循环 function loop() { var ts = opts.endTime - opts.nowTime //计算剩余的毫秒数 , dd = parseInt(ts / 8.64e7) //计算剩余的天数 , hh = parseInt(ts / 3.6e7 % 24)//计算剩余的小时数 , mm = parseInt(ts / 6e4 % 60)//计算剩余的分钟数 , ss = parseInt(ts / 1e3 % 60)//计算剩余的秒数 ; //当前时间递减 opts.nowTime += opts.interval * 1e3; if (ts <= 0) { clearInterval(timer); opts.finaled(); } else { opts.called(dd, hh, mm, ss); } } } };
浏览器检测方法集
Angela.browser = { //#浏览器 browsers: { //# 浏览器内核类别 weixin: /micromessenger(\/[\d\.]+)*/ //微信内置浏览器 , mqq: /mqqbrowser(\/[\d\.]+)*/ //手机QQ浏览器 , uc: /ucbrowser(\/[\d\.]+)*/ //UC浏览器 , chrome: /(?:chrome|crios)(\/[\d\.]+)*/ //chrome浏览器 , firefox: /firefox(\/[\d\.]+)*/ //火狐浏览器 , opera: /opera(\/|\s)([\d\.]+)*/ //欧朋浏览器 , sougou: /sogoumobilebrowser(\/[\d\.]+)*/ //搜狗手机浏览器 , baidu: /baidubrowser(\/[\d\.]+)*/ //百度手机浏览器 , 360: /360browser([\d\.]*)/ //360浏览器 , safari: /safari(\/[\d\.]+)*/ //苹果浏览器 , ie: /msie\s([\d\.]+)*/ // ie 浏览器 } //@errCall : 错误回调 , addFav: function (url, title, errCall) { //#加入收藏夹 try { window.external.addFavorite(url, title); } catch (e) { try { window.sidebar.addPanel(title, url, ''); } catch (e) { errCall(); } } }, //浏览器版本 coreInit: function () { //#noadd var i = null , browsers = this.browsers , ua = window.navigator.userAgent.toLowerCase() , brower = '' , pos = 1 ; for (i in browsers) { if (brower = ua.match(browsers[i])) { if (i == 'opera') { pos = 2; } else { pos = 1; } this.version = (brower[pos] || '').replace(/[\/\s]+/, ''); this.core = i; return i; } } } // 检测IE版本 !仅支持IE: 5,6,7,8,9 版本 , ie: (function () { //# 检测IE版本 !仅支: ie5,6,7,8,9 var v = 3, div = document.createElement('div'), all = div.getElementsByTagName('i'); while ( div.innerHTML = '<!--[if gt IE ' + (++v) + ']><i></i><![endif]-->', all[0] ); return v > 4 ? v : false; })() , isWebkit: /webkit/i.test(navigator.userAgent) };
json方法
Angela.json = { //# json 对象 // 字符串 变为 json 对象 parse: function (data) { //# 格式化字符串,变为 json 对象 var // JSON RegExp rvalidchars = /^[\],:{}\s]*$/ , rvalidbraces = /(?:^|:|,)(?:\s*\[)+/g , rvalidescape = /\\(?:["\\\/bfnrt]|u[\da-fA-F]{4})/g , rvalidtokens = /"[^"\\\r\n]*"|true|false|null|-?(?:\d+\.|)\d+(?:[eE][+-]?\d+|)/g ; if (window.JSON && window.JSON.parse) { return window.JSON.parse(data); } if (data === null) { return data; } if (typeof data === "string") { data = data.replace(/^\s+|\s+$/g, ''); if (data && rvalidchars.test(data.replace(rvalidescape, "@") .replace(rvalidtokens, "]") .replace(rvalidbraces, ""))) { return (new Function("return " + data))(); } } return ''; } };
extend方法
Angela.extend = function () { //# 对象扩展 var target = arguments[0] || {} , i = 1 , length = arguments.length , options ; if (typeof target != "object" && typeof target != "function") target = {}; for (; i < length; i++) { if ((options = arguments[i]) != null) { for (var name in options) { var copy = options[name]; if (target === copy) { continue; } if (copy !== undefined) { target[name] = copy; } } } } return target; };
类型判断的方法
/* *判断变量val是不是整数类型 */ function isNumber(val) { return typeof val === 'number' && isFinite(val); } /* *判断变量val是不是布尔类型 */ function isBoolean(val) { return typeof val === 'boolean'; } /* *判断变量val是不是字符串类型 */ function isString (val) { return typeof val === 'string'; } /* *判断变量val是不是undefined */ function isUndefined(val) { return typeof val === 'undefined'; } /* *判断变量val是不是对象 */ function isObj(str) { if (str===null||typeof str==='undefined') { return false; } return typeof str === 'object'; } /* *判断变量val是不是null */ function isNull(val) { return val === null; } /* *判断变量arr是不是数组 *方法一 */ function isArray1(arr) { return Object.prototype.toString.apply(arr) === '[object Array]'; } /* *判断变量arr是不是数组 *方法二 */ function isArray2(arr) { if (arr === null || typeof arr === 'undefined') { return false; } return arr.constructor === Array; }

핫 AI 도구

Undresser.AI Undress
사실적인 누드 사진을 만들기 위한 AI 기반 앱

AI Clothes Remover
사진에서 옷을 제거하는 온라인 AI 도구입니다.

Undress AI Tool
무료로 이미지를 벗다

Clothoff.io
AI 옷 제거제

Video Face Swap
완전히 무료인 AI 얼굴 교환 도구를 사용하여 모든 비디오의 얼굴을 쉽게 바꾸세요!

인기 기사

뜨거운 도구

메모장++7.3.1
사용하기 쉬운 무료 코드 편집기

SublimeText3 중국어 버전
중국어 버전, 사용하기 매우 쉽습니다.

스튜디오 13.0.1 보내기
강력한 PHP 통합 개발 환경

드림위버 CS6
시각적 웹 개발 도구

SublimeText3 Mac 버전
신 수준의 코드 편집 소프트웨어(SublimeText3)

뜨거운 주제










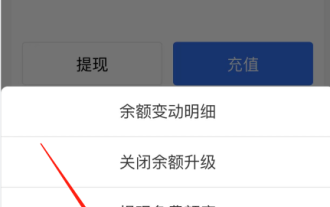
Alipay는 일반적으로 여유 현금을 보관하고 이체하고 결제하는 데 사용됩니다. 그러나 Alipay에 돈이 있다고 표시되지만 Alipay 잔액을 사용할 수 없는 상황이 발생하는 경우 문제를 해결하는 방법은 무엇입니까? 다음으로, 본 사이트의 편집자가 Alipay의 동결된 잔액을 처리하는 방법에 대한 자세한 운영 방법을 알려드릴 것입니다. 이에 관심 있는 친구들은 에디터를 팔로우해서 구경해보세요! Alipay 동결 잔액 도입 및 처리의 세부 운영 방법 Alipay 잔액이 동결되면 Alipay 고객 서비스 전화번호로 직접 전화하여 관련 프롬프트 및 요구 사항에 따라 계정 동결을 해제할 수 있습니다. Alipay 잔액 변경 내역을 어떻게 삭제하나요? Alipay 기본 인터페이스에 들어가면 오른쪽 하단에 "내" 옵션이 표시됩니다. 이 인터페이스에서 "Bill"을 클릭하면 다른 인터페이스로 이동할 수 있습니다.
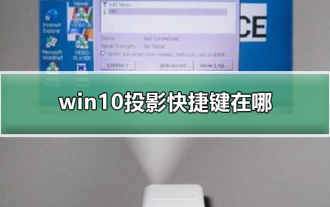
win10 프로젝터를 사용할 때 많은 사람들이 컴퓨터에서 조작하는 것이 매우 복잡하다고 생각합니다. 그렇다면 단축키를 사용하여 조작하는 방법은 무엇입니까? 다음으로 편집자가 함께 볼 수 있도록 안내해 드리겠습니다. Windows 10에서 투영 단축키를 누르는 방법에 대한 자세한 튜토리얼. 1단계: Win+P 키를 동시에 길게 누르세요. 2단계: 컴퓨터 오른쪽에 나타나는 옵션을 선택하세요. win10 프로젝터 관련 질문 win10 프로젝터 설정 위치 >>> win10 프로젝터로 전체 화면을 채우는 방법 >>> win10 프로젝터로 이 컴퓨터에 투사하는 방법 >>>
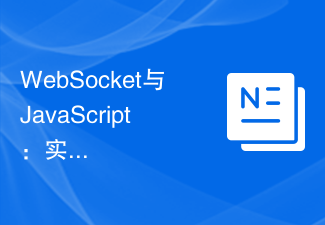
WebSocket과 JavaScript: 실시간 모니터링 시스템 구현을 위한 핵심 기술 서론: 인터넷 기술의 급속한 발전과 함께 실시간 모니터링 시스템이 다양한 분야에서 널리 활용되고 있다. 실시간 모니터링을 구현하는 핵심 기술 중 하나는 WebSocket과 JavaScript의 조합입니다. 이 기사에서는 실시간 모니터링 시스템에서 WebSocket 및 JavaScript의 적용을 소개하고 코드 예제를 제공하며 구현 원칙을 자세히 설명합니다. 1. 웹소켓 기술
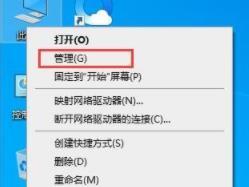
많은 사용자가 매일 컴퓨터를 사용할 때 항상 시스템에서 자동 업데이트를 받기 때문에 컴퓨터 속도가 느려질 뿐만 아니라 작동이 중단되기도 합니다. 이러한 이유로 오늘은 Win11을 사용하지 않는 경우의 작동 방법을 알려드리겠습니다. 자동 업데이트를 원합니다. 자동 업데이트가 계속 영향을 미치는 경우 종료하는 방법을 살펴보겠습니다. Windows 11 시스템의 자동 업데이트를 방지하는 방법 1. 먼저 바탕 화면에서 "내 PC"를 마우스 오른쪽 버튼으로 클릭하고 "관리"를 선택합니다. 2. 열린 "컴퓨터 관리"에서 "서비스" → "응용 프로그램" → "서비스" → "Windows 업데이트"를 클릭합니다. 3. 그런 다음 "Windows 업데이트"를 두 번 클릭하고 "시작 유형"을 "사용 안 함"으로 설정한 다음 서비스 "중지"를 클릭하고 확인합니다. 4. 첫 번째 손실된 데이터를 복원하려면 "복구" 탭을 클릭하세요.
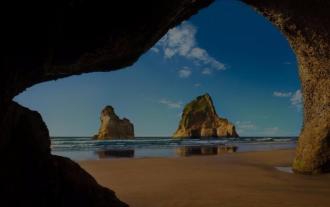
많은 사용자가 컴퓨터를 사용할 때 부팅 모드에서 전원 켜기 암호를 직접 건너뛰고 그대로 두는 상황에 직면하는 경우가 많습니다. 이는 실제로 해결하기 어렵지 않습니다. win10 보안 모드를 건너뛰십시오. win10 안전 모드에 들어갈 수 없습니다: 1. 많은 사용자가 데스크탑에 들어가기 전에 멈춰 있고 비밀번호 인터페이스가 없습니다. 2. 3~4회 강제 종료하면 시작 설정 페이지가 나타나고 "안전 모드 활성화"를 선택합니다. 3. "시작"을 마우스 오른쪽 버튼으로 클릭하고 "실행"을 클릭합니다. 4. 실행창에 "msconfig"를 입력하세요. 5. "일반"을 클릭하고 "정상 시작"을 선택합니다. 6. 비밀번호 인터페이스에 성공적으로 진입했습니다.
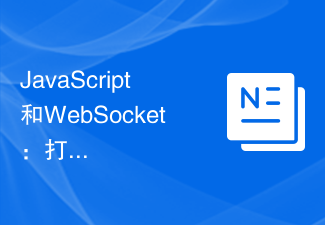
JavaScript 및 WebSocket: 효율적인 실시간 일기 예보 시스템 구축 소개: 오늘날 일기 예보의 정확성은 일상 생활과 의사 결정에 매우 중요합니다. 기술이 발전함에 따라 우리는 날씨 데이터를 실시간으로 획득함으로써 보다 정확하고 신뢰할 수 있는 일기예보를 제공할 수 있습니다. 이 기사에서는 JavaScript 및 WebSocket 기술을 사용하여 효율적인 실시간 일기 예보 시스템을 구축하는 방법을 알아봅니다. 이 문서에서는 특정 코드 예제를 통해 구현 프로세스를 보여줍니다. 우리
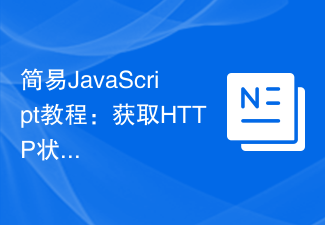
JavaScript 튜토리얼: HTTP 상태 코드를 얻는 방법, 특정 코드 예제가 필요합니다. 서문: 웹 개발에서는 서버와의 데이터 상호 작용이 종종 포함됩니다. 서버와 통신할 때 반환된 HTTP 상태 코드를 가져와서 작업의 성공 여부를 확인하고 다양한 상태 코드에 따라 해당 처리를 수행해야 하는 경우가 많습니다. 이 기사에서는 JavaScript를 사용하여 HTTP 상태 코드를 얻는 방법과 몇 가지 실용적인 코드 예제를 제공합니다. XMLHttpRequest 사용
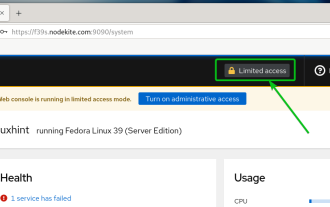
Cockpit은 Linux 서버용 웹 기반 그래픽 인터페이스입니다. 이는 주로 신규/전문가 사용자가 Linux 서버를 보다 쉽게 관리할 수 있도록 하기 위한 것입니다. 이 문서에서는 Cockpit 액세스 모드와 CockpitWebUI에서 Cockpit으로 관리 액세스를 전환하는 방법에 대해 설명합니다. 콘텐츠 항목: Cockpit 입장 모드 현재 Cockpit 액세스 모드 찾기 CockpitWebUI에서 Cockpit에 대한 관리 액세스 활성화 CockpitWebUI에서 Cockpit에 대한 관리 액세스 비활성화 결론 조종석 입장 모드 조종석에는 두 가지 액세스 모드가 있습니다. 제한된 액세스: 이는 조종석 액세스 모드의 기본값입니다. 이 액세스 모드에서는 조종석에서 웹 사용자에 액세스할 수 없습니다.
