Kelas SortedDictionary dalam C# diwakili sebagai SortedDictionary yang terdiri daripada kunci dan koleksi nilai dengan kunci mewakili perkataan dan nilai mewakili definisi dan pasangan kunci dan nilai ini diisih berdasarkan kunci dan SortedDictionary kelas kepunyaan ruang nama System.Collection.Generics dan kekunci dalam SortedDictionary sentiasa berbeza, tidak berubah dan tidak akan menjadi batal tetapi nilainya boleh menjadi batal jika jenis nilai adalah jenis, rujukan dan operasi sisipan dan pengambilan adalah lebih pantas menggunakan SortedDictionary kelas dan mendapatkan semula pasangan nilai kunci dalam kelas SortedDictionary dilakukan menggunakan KeyValuePair Structure.
Sintaks:
SortedDictionary<TKey, TValue>variable_name = new SortedDictionary<TKey, TValue>();
Salin selepas log masuk
Kerja Kelas SortedDictionary dalam C#
- ICollection>, IEnumerable, IReadOnlyCollection>, TValue>>, IEnumerable> ;, ICollection, IDictionary, IDictionary, IEnumerable, IReadOnlyDictionary Antara muka dilaksanakan oleh kelas SortedDictionary.
- Operasi memasukkan unsur dan mengalih keluar unsur boleh menjadi lebih pantas menggunakan kelas SortedDictionary.
- Kunci mestilah berbeza dan tidak boleh ada kunci pendua dalam kelas SortedDictionary.
- Kunci adalah unik dan tidak akan menjadi batal dalam kelas SortedDictionary.
- Jika jenis nilai adalah rujukan jenis, nilai tersebut dibenarkan menjadi batal.
- Jenis pasangan kunci dan nilai yang sama boleh disimpan menggunakan kelas SortedDictionary.
- Kamus Sorted dalam C# bersifat dinamik yang bermaksud saiz SortedDictionary bertambah mengikut keperluan.
- Pengisihan dilakukan dalam susunan menurun mengikut kelas SortedDictionary.
- Jumlah bilangan pasangan kunci dan nilai yang boleh dipegang oleh kelas SortedDictionary ialah kapasiti kelas SortedDictionary.
Pembina C# SortedDictionary
Diberikan di bawah ialah pembina C# SortedDictionary:
1. SortedDictionary()
Satu contoh kelas SortedDictionary dimulakan yang kosong dan pelaksanaan IComparer digunakan secara lalai untuk jenis, kunci.
2. SortedDictionary(ICcomparer)
Satu contoh kelas SortedDictionary dimulakan yang kosong dan pelaksanaan IComparer yang ditentukan digunakan untuk perbandingan utama.
3. SortedDictionary(IDictionary)
Satu contoh kelas SortedDictionary dimulakan yang terdiri daripada elemen yang diambil daripada IDictionary yang ditentukan sebagai parameter dan pelaksanaan ICompareris yang digunakan secara lalai untuk jenis, kunci.
4. SortedDictionary(IDictionary, IComparer)
Satu contoh kelas SortedDictionary dimulakan yang terdiri daripada elemen yang disalin daripada IDictionary yang ditentukan sebagai parameter dan pelaksanaan IComparer yang ditentukan digunakan untuk perbandingan utama.
Kaedah C# SortedDictionary
Diberikan di bawah adalah kaedahnya :
-
Add(TKey, TValue): An element with key and value specified as parameters is added into the SortedDictionary using Add(TKey, TValue) method.
-
Remove(Tkey): An element with key specified as parameter is removed from the SortedDictionary using Remove(TKey) method.
-
ContainsKey(TKey): The ContainsKey(TKey) method is used to determine if the key specified as parameter is present in the SortedDictionary.
-
ContainsValue(TValue): The ContainsKey(TValue) method is used to determine if the value specified as parameter is present in the SortedDictionary.
-
Clear(): The clear() method is used to clear all the objects from the SortedDictionary.
-
CopyTo(KeyValuePair[], Int32): The CopyTo(KeyValuePair[], Int32) method is used to copy the elements of the SortedDictionary to the array of KeyValuePair structures specified as the parameter with the array starting from the index specified in the parameter.
-
Equals(Object): The Equals(Object) method is used to determine if the object specified as the parameter is equal to the current object.
-
GetEnumerator(): The GetEnumerator() method is used to return an enumerator which loops through the SortedDictionary.
-
GetHashCode(): The GetHashCode() method is the hash function by default.
-
GetType(): The GetType() method returns the current instance type.
-
MemberwiseClone(): The MemberwiseClone() method is used to create a shallow copy of the current object.
-
ToString():The ToString() method is used to return a string which represents the current object.
-
TryGetValue(TKey, TValue): The TryGetValue(TKey, TValue) method is used to obtain the value associated with key specified as parameter.
Examples
Given below are the examples mentioned:
Example #1
C# program to demonstrate Add method, Remove method, ContainsKey method, ContainsValue method and TryGetValue method of Sorted Dictionary class.
Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
//a class called program is defined
public class program
{
//main method is called
public static void Main()
{
//a new sorted dictionary is created with key type int and value type string
SortedDictionary<int, string>st = new SortedDictionary<int, string>();
//Add method is used to add objects to the dictionary
st.Add(30,"India");
st.Add(10,"China");
st.Add(20,"Nepal");
st.Remove(10);
Console.WriteLine("If the key 30 is present?{0}", st.ContainsKey(30));
Console.WriteLine("If the key 20 is present? {0}", st.Contains(new KeyValuePair<int, string>(20, "Nepal")));
//new sorted dictionary of both string key and string value types is defined
SortedDictionary<string, string> st1 = new SortedDictionary<string, string>();
st1.Add("Flag","India");
Console.WriteLine("If the value India is present?{0}", st1.ContainsValue("India"));
string rest;
if(st.TryGetValue(30, out rest))
{
Console.WriteLine("The value of the specified key is {0}", rest);
}
else
{
Console.WriteLine("The specified key is not present.");
}
}
}
Salin selepas log masuk
Output:
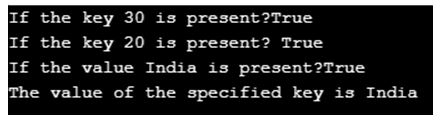
Explanation:
- In the above program, a class called program is defined. Then the main method is called. Then a new sorted dictionary is created with key type int and value type string. Then Add method is used to add objects to the sorted dictionary. Then Remove method is used to remove objects from the sorted dictionary.
- Then new sorted dictionary of both string key and string value types is defined. Then contains value method is used to determine if a certain value is present in the sorted dictionary. Then trygetvalue method is used to obtain the value of a specified key.
Example #2
C# program to demonstrate Add method and Clear method of sorted dictionary class.
Code:
using System;
using System.Collections.Generic;
//a class called check is defined
class check
{
// main method is called
public static void Main()
{
// a new sorted dictionary is created with key type string and value type string
SortedDictionary<string, string> tam = new SortedDictionary<string, string>();
// using add method in dictionary to add the objects to the dictionary
tam.Add("R", "Red");
tam.Add("G", "Green");
tam.Add("Y", "Yellow");
// a foreach loop is used to loop around every key in the dictionary and to obtain each key value
foreach(KeyValuePair<string,string>ra in tam)
{
Console.WriteLine("The key and value pairs is SortedDictionary are = {0} and {1}", ra.Key, ra.Value);
}
//using clear method to remove all the objects from sorted dictionary
tam.Clear();
foreach(KeyValuePair<string,string>tr in tam)
{
Console.WriteLine("The key and value pairs is SortedDictionary are = {0} and {1}", tr.Key, tr.Value);
}
}
}
Salin selepas log masuk
Output:

Explanation:
- In the above program, check is the class defined. Then main method is called. Then a new sorted dictionary is created with key type string and value type string. Then we have used add method to add the objects to the sorted dictionary.
- Then a for each loop is used to loop around every key in the sorted dictionary to obtain each key value. Then clear method is used to clear the console.
Atas ialah kandungan terperinci C# SortedDictionary. Untuk maklumat lanjut, sila ikut artikel berkaitan lain di laman web China PHP!