


Carian Tatasusunan dalam DSA menggunakan JavaScript: Daripada Asas kepada Lanjutan
Pencarian tatasusunan ialah konsep asas dalam Struktur Data dan Algoritma (DSA). Catatan blog ini akan merangkumi pelbagai teknik carian tatasusunan menggunakan JavaScript, dari peringkat asas hingga lanjutan. Kami akan meneroka 20 contoh, membincangkan kerumitan masa dan menyediakan masalah LeetCode untuk latihan.
Jadual Kandungan
- Carian Linear
- Carian Binari
- Lompat Cari
- Carian Interpolasi
- Carian Eksponen
- Carian Subarray
- Teknik Dua Penunjuk
- Teknik Tingkap Gelongsor
- Teknik Carian Terperinci
- Masalah Amalan LeetCode
1. Carian Linear
Carian linear ialah algoritma carian paling mudah yang berfungsi pada tatasusunan yang diisih dan tidak diisih.
Kerumitan Masa: O(n), dengan n ialah bilangan elemen dalam tatasusunan.
Contoh 1: Carian Linear Asas
function linearSearch(arr, target) { for (let i = 0; i < arr.length; i++) { if (arr[i] === target) { return i; } } return -1; } const arr = [5, 2, 8, 12, 1, 6]; console.log(linearSearch(arr, 8)); // Output: 2 console.log(linearSearch(arr, 3)); // Output: -1
Contoh 2: Cari Semua Kejadian
function findAllOccurrences(arr, target) { const result = []; for (let i = 0; i < arr.length; i++) { if (arr[i] === target) { result.push(i); } } return result; } const arr = [1, 2, 3, 4, 2, 5, 2, 6]; console.log(findAllOccurrences(arr, 2)); // Output: [1, 4, 6]
2. Carian Binari
Carian binari ialah algoritma yang cekap untuk mencari dalam tatasusunan yang diisih.
Kerumitan Masa: O(log n)
Contoh 3: Carian Binari Berulang
function binarySearch(arr, target) { let left = 0; let right = arr.length - 1; while (left <= right) { const mid = Math.floor((left + right) / 2); if (arr[mid] === target) { return mid; } else if (arr[mid] < target) { left = mid + 1; } else { right = mid - 1; } } return -1; } const sortedArr = [1, 3, 5, 7, 9, 11, 13, 15]; console.log(binarySearch(sortedArr, 7)); // Output: 3 console.log(binarySearch(sortedArr, 10)); // Output: -1
Contoh 4: Carian Binari Rekursif
function recursiveBinarySearch(arr, target, left = 0, right = arr.length - 1) { if (left > right) { return -1; } const mid = Math.floor((left + right) / 2); if (arr[mid] === target) { return mid; } else if (arr[mid] < target) { return recursiveBinarySearch(arr, target, mid + 1, right); } else { return recursiveBinarySearch(arr, target, left, mid - 1); } } const sortedArr = [1, 3, 5, 7, 9, 11, 13, 15]; console.log(recursiveBinarySearch(sortedArr, 13)); // Output: 6 console.log(recursiveBinarySearch(sortedArr, 4)); // Output: -1
3. Lompat Carian
Carian lompat ialah algoritma untuk tatasusunan diisih yang berfungsi dengan melangkau beberapa elemen untuk mengurangkan bilangan perbandingan.
Kerumitan Masa: O(√n)
Contoh 5: Pelaksanaan Carian Lompat
function jumpSearch(arr, target) { const n = arr.length; const step = Math.floor(Math.sqrt(n)); let prev = 0; while (arr[Math.min(step, n) - 1] < target) { prev = step; step += Math.floor(Math.sqrt(n)); if (prev >= n) { return -1; } } while (arr[prev] < target) { prev++; if (prev === Math.min(step, n)) { return -1; } } if (arr[prev] === target) { return prev; } return -1; } const sortedArr = [0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377]; console.log(jumpSearch(sortedArr, 55)); // Output: 10 console.log(jumpSearch(sortedArr, 111)); // Output: -1
4. Carian Interpolasi
Carian interpolasi ialah varian carian binari yang dipertingkatkan untuk tatasusunan diisih yang diedarkan secara seragam.
Kerumitan Masa: O(log log n) untuk data teragih seragam, O(n) dalam kes yang paling teruk.
Contoh 6: Pelaksanaan Carian Interpolasi
function interpolationSearch(arr, target) { let low = 0; let high = arr.length - 1; while (low <= high && target >= arr[low] && target <= arr[high]) { if (low === high) { if (arr[low] === target) return low; return -1; } const pos = low + Math.floor(((target - arr[low]) * (high - low)) / (arr[high] - arr[low])); if (arr[pos] === target) { return pos; } else if (arr[pos] < target) { low = pos + 1; } else { high = pos - 1; } } return -1; } const uniformArr = [1, 2, 4, 8, 16, 32, 64, 128, 256, 512]; console.log(interpolationSearch(uniformArr, 64)); // Output: 6 console.log(interpolationSearch(uniformArr, 100)); // Output: -1
5. Carian Eksponen
Carian eksponen berguna untuk carian tanpa sempadan dan berfungsi dengan baik untuk tatasusunan terhad juga.
Kerumitan Masa: O(log n)
Contoh 7: Pelaksanaan Carian Eksponen
function exponentialSearch(arr, target) { if (arr[0] === target) { return 0; } let i = 1; while (i < arr.length && arr[i] <= target) { i *= 2; } return binarySearch(arr, target, i / 2, Math.min(i, arr.length - 1)); } function binarySearch(arr, target, left, right) { while (left <= right) { const mid = Math.floor((left + right) / 2); if (arr[mid] === target) { return mid; } else if (arr[mid] < target) { left = mid + 1; } else { right = mid - 1; } } return -1; } const sortedArr = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15]; console.log(exponentialSearch(sortedArr, 7)); // Output: 6 console.log(exponentialSearch(sortedArr, 16)); // Output: -1
6. Carian Subarray
Mencari subarray dalam tatasusunan yang lebih besar ialah masalah biasa dalam DSA.
Contoh 8: Carian Subarray Naif
Kerumitan Masa: O(n * m), dengan n ialah panjang tatasusunan utama dan m ialah panjang subarray.
function naiveSubarraySearch(arr, subArr) { for (let i = 0; i <= arr.length - subArr.length; i++) { let j; for (j = 0; j < subArr.length; j++) { if (arr[i + j] !== subArr[j]) { break; } } if (j === subArr.length) { return i; } } return -1; } const mainArr = [1, 2, 3, 4, 5, 6, 7, 8, 9]; const subArr = [3, 4, 5]; console.log(naiveSubarraySearch(mainArr, subArr)); // Output: 2
Contoh 9: Algoritma KMP untuk Carian Subarray
Kerumitan Masa: O(n + m)
function kmpSearch(arr, pattern) { const n = arr.length; const m = pattern.length; const lps = computeLPS(pattern); let i = 0, j = 0; while (i < n) { if (pattern[j] === arr[i]) { i++; j++; } if (j === m) { return i - j; } else if (i < n && pattern[j] !== arr[i]) { if (j !== 0) { j = lps[j - 1]; } else { i++; } } } return -1; } function computeLPS(pattern) { const m = pattern.length; const lps = new Array(m).fill(0); let len = 0; let i = 1; while (i < m) { if (pattern[i] === pattern[len]) { len++; lps[i] = len; i++; } else { if (len !== 0) { len = lps[len - 1]; } else { lps[i] = 0; i++; } } } return lps; } const mainArr = [1, 2, 3, 4, 5, 6, 7, 8, 9]; const pattern = [3, 4, 5]; console.log(kmpSearch(mainArr, pattern)); // Output: 2
7. Teknik Dua Penunjuk
Teknik dua mata sering digunakan untuk mencari dalam tatasusunan yang disusun atau apabila berurusan dengan pasangan.
Contoh 10: Cari Pasangan dengan Jumlah Diberi
Kerumitan Masa: O(n)
function findPairWithSum(arr, target) { let left = 0; let right = arr.length - 1; while (left < right) { const sum = arr[left] + arr[right]; if (sum === target) { return [left, right]; } else if (sum < target) { left++; } else { right--; } } return [-1, -1]; } const sortedArr = [1, 2, 3, 4, 5, 6, 7, 8, 9]; console.log(findPairWithSum(sortedArr, 10)); // Output: [3, 7]
Contoh 11: Masalah Jumlah Tiga
Kerumitan Masa: O(n^2)
function threeSum(arr, target) { arr.sort((a, b) => a - b); const result = []; for (let i = 0; i < arr.length - 2; i++) { if (i > 0 && arr[i] === arr[i - 1]) continue; let left = i + 1; let right = arr.length - 1; while (left < right) { const sum = arr[i] + arr[left] + arr[right]; if (sum === target) { result.push([arr[i], arr[left], arr[right]]); while (left < right && arr[left] === arr[left + 1]) left++; while (left < right && arr[right] === arr[right - 1]) right--; left++; right--; } else if (sum < target) { left++; } else { right--; } } } return result; } const arr = [-1, 0, 1, 2, -1, -4]; console.log(threeSum(arr, 0)); // Output: [[-1, -1, 2], [-1, 0, 1]]
8. Teknik Tingkap Gelongsor
Teknik tetingkap gelongsor berguna untuk menyelesaikan masalah tatasusunan/rentetan dengan elemen bersebelahan.
Contoh 12: Subarray Jumlah Maksimum Saiz K
Kerumitan Masa: O(n)
function maxSumSubarray(arr, k) { let maxSum = 0; let windowSum = 0; for (let i = 0; i < k; i++) { windowSum += arr[i]; } maxSum = windowSum; for (let i = k; i < arr.length; i++) { windowSum = windowSum - arr[i - k] + arr[i]; maxSum = Math.max(maxSum, windowSum); } return maxSum; } const arr = [1, 4, 2, 10, 23, 3, 1, 0, 20]; console.log(maxSumSubarray(arr, 4)); // Output: 39
Contoh 13: Subrentetan Terpanjang dengan Aksara K Distinct
Kerumitan Masa: O(n)
function longestSubstringKDistinct(s, k) { const charCount = new Map(); let left = 0; let maxLength = 0; for (let right = 0; right < s.length; right++) { charCount.set(s[right], (charCount.get(s[right]) || 0) + 1); while (charCount.size > k) { charCount.set(s[left], charCount.get(s[left]) - 1); if (charCount.get(s[left]) === 0) { charCount.delete(s[left]); } left++; } maxLength = Math.max(maxLength, right - left + 1); } return maxLength; } const s = "aabacbebebe"; console.log(longestSubstringKDistinct(s, 3)); // Output: 7
9. Teknik Carian Lanjutan
Contoh 14: Cari dalam Tatasusunan Isih Diputar
Kerumitan Masa: O(log n)
function searchRotatedArray(arr, target) { let left = 0; let right = arr.length - 1; while (left <= right) { const mid = Math.floor((left + right) / 2); if (arr[mid] === target) { return mid; } if (arr[left] <= arr[mid]) { if (target >= arr[left] && target < arr[mid]) { right = mid - 1; } else { left = mid + 1; } } else { if (target > arr[mid] && target <= arr[right]) { left = mid + 1; } else { right = mid - 1; } } } return -1; } const rotatedArr = [4, 5, 6, 7, 0, 1, 2]; console.log(searchRotatedArray(rotatedArr, 0)); // Output: 4
Contoh 15: Cari dalam Matriks 2D
Kerumitan Masa: O(log(m * n)), dengan m ialah bilangan baris dan n ialah bilangan lajur
function searchMatrix(matrix, target) { if (!matrix.length || !matrix[0].length) return false; const m = matrix.length; const n = matrix[0].length; let left = 0; let right = m * n - 1; while (left <= right) { const mid = Math.floor((left + right) / 2); const midValue = matrix[Math.floor(mid / n)][mid % n]; if (midValue === target) { return true; } else if (midValue < target) { left = mid + 1; } else { right = mid - 1; } } return false; } const matrix = [ [1, 3, 5, 7], [10, 11, 16, 20], [23, 30, 34, 50] ]; console.log(searchMatrix(matrix, 3)); // Output: true
Contoh 16: Cari Elemen Puncak
Kerumitan Masa: O(log n)
function findPeakElement(arr) { let left = 0; let right = arr.length - 1; while (left < right) { const mid = Math.floor((left + right) / 2); if (arr[mid] > arr[mid + 1]) { right = mid; } else { left = mid + 1; } } return left; } const arr = [1, 2, 1, 3, 5, 6, 4]; console.log(findPeakElement(arr)); // Output: 5
Contoh 17: Cari dalam Susunan Isih Saiz Tidak Diketahui
Kerumitan Masa: O(log n)
function searchUnknownSize(arr, target) { let left = 0; let right = 1; while (arr[right] < target) { left = right; right *= 2; } return binarySearch(arr, target, left, right); } function binarySearch(arr, target, left, right) { while (left <= right) { const mid = Math.floor((left + right) / 2); if (arr[mid] === target) { return mid; } else if (arr[mid] < target) { left = mid + 1; } else { right = mid - 1; } } return -1; } // Assume we have a special array that throws an error when accessing out-of-bounds elements const specialArray = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15]; console.log(searchUnknownSize(specialArray, 7)); // Output: 6
Contoh 18: Cari Minimum dalam Tatasusunan Isih Diputar
Kerumitan Masa: O(log n)
function findMin(arr) { let left = 0; let right = arr.length - 1; while (left < right) { const mid = Math.floor((left + right) / 2); if (arr[mid] > arr[right]) { left = mid + 1; } else { right = mid; } } return arr[left]; } const rotatedArr = [4, 5, 6, 7, 0, 1, 2]; console.log(findMin(rotatedArr)); // Output: 0
Contoh 19: Cari Julat
Kerumitan Masa: O(log n)
function searchRange(arr, target) { const left = findBound(arr, target, true); if (left === -1) return [-1, -1]; const right = findBound(arr, target, false); return [left, right]; } function findBound(arr, target, isLeft) { let left = 0; let right = arr.length - 1; let result = -1; while (left <= right) { const mid = Math.floor((left + right) / 2); if (arr[mid] === target) { result = mid; if (isLeft) { right = mid - 1; } else { left = mid + 1; } } else if (arr[mid] < target) { left = mid + 1; } else { right = mid - 1; } } return result; } const arr = [5, 7, 7, 8, 8, 10]; console.log(searchRange(arr, 8)); // Output: [3, 4]
Contoh 20: Median Dua Tatasusunan Isih
Kerumitan Masa: O(log(min(m, n))), dengan m dan n ialah panjang dua tatasusunan
function findMedianSortedArrays(nums1, nums2) { if (nums1.length > nums2.length) { return findMedianSortedArrays(nums2, nums1); } const m = nums1.length; const n = nums2.length; let left = 0; let right = m; while (left <= right) { const partitionX = Math.floor((left + right) / 2); const partitionY = Math.floor((m + n + 1) / 2) - partitionX; const maxLeftX = partitionX === 0 ? -Infinity : nums1[partitionX - 1]; const minRightX = partitionX === m ? Infinity : nums1[partitionX]; const maxLeftY = partitionY === 0 ? -Infinity : nums2[partitionY - 1]; const minRightY = partitionY === n ? Infinity : nums2[partitionY]; if (maxLeftX <= minRightY && maxLeftY <= minRightX) { if ((m + n) % 2 === 0) { return (Math.max(maxLeftX, maxLeftY) + Math.min(minRightX, minRightY)) / 2; } else { return Math.max(maxLeftX, maxLeftY); } } else if (maxLeftX > minRightY) { right = partitionX - 1; } else { left = partitionX + 1; } } throw new Error("Input arrays are not sorted."); } const nums1 = [1, 3]; const nums2 = [2]; console.log(findMedianSortedArrays(nums1, nums2)); // Output: 2
10. Masalah Amalan LeetCode
Untuk menguji lagi pemahaman dan kemahiran anda dalam pencarian tatasusunan, berikut ialah 15 masalah LeetCode yang boleh anda amalkan:
- Deux sommes
- Recherche dans un tableau trié avec rotation
- Trouver le minimum dans un tableau trié avec rotation
- Rechercher une matrice 2D
- Trouver l'élément de pointe
- Rechercher une plage
- Médiane de deux tableaux triés
- Kième plus grand élément d'un tableau
- Trouver les éléments les plus proches
- Rechercher dans un tableau trié de taille inconnue
- Capacité d'expédition des colis sous les jours J
- Koko mange des bananes
- Trouver le numéro en double
- Sous-chaîne la plus longue avec au plus K caractères distincts
- La somme du sous-tableau est égale à K
Ces problèmes couvrent un large éventail de techniques de recherche de tableaux et vous aideront à consolider votre compréhension des concepts abordés dans cet article de blog.
En conclusion, maîtriser les techniques de recherche de tableaux est crucial pour maîtriser les structures de données et les algorithmes. En comprenant et en mettant en œuvre ces différentes méthodes, vous serez mieux équipé pour résoudre des problèmes complexes et optimiser votre code. N'oubliez pas d'analyser la complexité temporelle et spatiale de chaque approche et de choisir la plus appropriée en fonction des exigences spécifiques de votre problématique.
Bon codage et bonne recherche !
Atas ialah kandungan terperinci Carian Tatasusunan dalam DSA menggunakan JavaScript: Daripada Asas kepada Lanjutan. Untuk maklumat lanjut, sila ikut artikel berkaitan lain di laman web China PHP!

Alat AI Hot

Undresser.AI Undress
Apl berkuasa AI untuk mencipta foto bogel yang realistik

AI Clothes Remover
Alat AI dalam talian untuk mengeluarkan pakaian daripada foto.

Undress AI Tool
Gambar buka pakaian secara percuma

Clothoff.io
Penyingkiran pakaian AI

Video Face Swap
Tukar muka dalam mana-mana video dengan mudah menggunakan alat tukar muka AI percuma kami!

Artikel Panas

Alat panas

Notepad++7.3.1
Editor kod yang mudah digunakan dan percuma

SublimeText3 versi Cina
Versi Cina, sangat mudah digunakan

Hantar Studio 13.0.1
Persekitaran pembangunan bersepadu PHP yang berkuasa

Dreamweaver CS6
Alat pembangunan web visual

SublimeText3 versi Mac
Perisian penyuntingan kod peringkat Tuhan (SublimeText3)

Topik panas










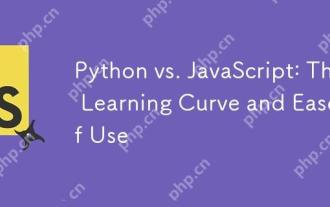
Python lebih sesuai untuk pemula, dengan lengkung pembelajaran yang lancar dan sintaks ringkas; JavaScript sesuai untuk pembangunan front-end, dengan lengkung pembelajaran yang curam dan sintaks yang fleksibel. 1. Sintaks Python adalah intuitif dan sesuai untuk sains data dan pembangunan back-end. 2. JavaScript adalah fleksibel dan digunakan secara meluas dalam pengaturcaraan depan dan pelayan.
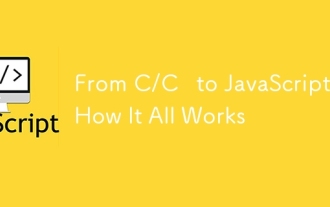
Peralihan dari C/C ke JavaScript memerlukan menyesuaikan diri dengan menaip dinamik, pengumpulan sampah dan pengaturcaraan asynchronous. 1) C/C adalah bahasa yang ditaip secara statik yang memerlukan pengurusan memori manual, manakala JavaScript ditaip secara dinamik dan pengumpulan sampah diproses secara automatik. 2) C/C perlu dikumpulkan ke dalam kod mesin, manakala JavaScript adalah bahasa yang ditafsirkan. 3) JavaScript memperkenalkan konsep seperti penutupan, rantaian prototaip dan janji, yang meningkatkan keupayaan pengaturcaraan fleksibiliti dan asynchronous.
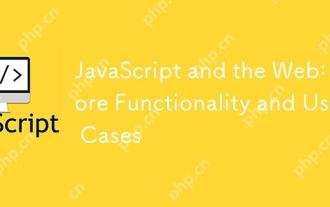
Penggunaan utama JavaScript dalam pembangunan web termasuk interaksi klien, pengesahan bentuk dan komunikasi tak segerak. 1) kemas kini kandungan dinamik dan interaksi pengguna melalui operasi DOM; 2) pengesahan pelanggan dijalankan sebelum pengguna mengemukakan data untuk meningkatkan pengalaman pengguna; 3) Komunikasi yang tidak bersesuaian dengan pelayan dicapai melalui teknologi Ajax.
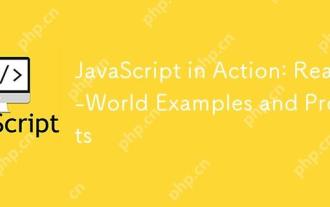
Aplikasi JavaScript di dunia nyata termasuk pembangunan depan dan back-end. 1) Memaparkan aplikasi front-end dengan membina aplikasi senarai TODO, yang melibatkan operasi DOM dan pemprosesan acara. 2) Membina Restfulapi melalui Node.js dan menyatakan untuk menunjukkan aplikasi back-end.
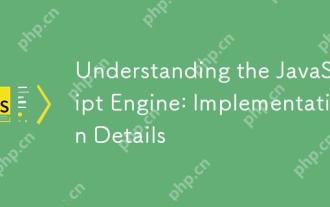
Memahami bagaimana enjin JavaScript berfungsi secara dalaman adalah penting kepada pemaju kerana ia membantu menulis kod yang lebih cekap dan memahami kesesakan prestasi dan strategi pengoptimuman. 1) aliran kerja enjin termasuk tiga peringkat: parsing, penyusun dan pelaksanaan; 2) Semasa proses pelaksanaan, enjin akan melakukan pengoptimuman dinamik, seperti cache dalam talian dan kelas tersembunyi; 3) Amalan terbaik termasuk mengelakkan pembolehubah global, mengoptimumkan gelung, menggunakan const dan membiarkan, dan mengelakkan penggunaan penutupan yang berlebihan.
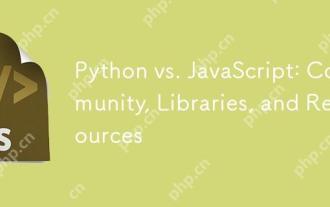
Python dan JavaScript mempunyai kelebihan dan kekurangan mereka sendiri dari segi komuniti, perpustakaan dan sumber. 1) Komuniti Python mesra dan sesuai untuk pemula, tetapi sumber pembangunan depan tidak kaya dengan JavaScript. 2) Python berkuasa dalam bidang sains data dan perpustakaan pembelajaran mesin, sementara JavaScript lebih baik dalam perpustakaan pembangunan dan kerangka pembangunan depan. 3) Kedua -duanya mempunyai sumber pembelajaran yang kaya, tetapi Python sesuai untuk memulakan dengan dokumen rasmi, sementara JavaScript lebih baik dengan MDNWebDocs. Pilihan harus berdasarkan keperluan projek dan kepentingan peribadi.
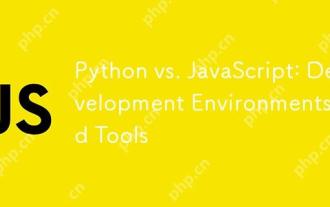
Kedua -dua pilihan Python dan JavaScript dalam persekitaran pembangunan adalah penting. 1) Persekitaran pembangunan Python termasuk Pycharm, Jupyternotebook dan Anaconda, yang sesuai untuk sains data dan prototaip cepat. 2) Persekitaran pembangunan JavaScript termasuk node.js, vscode dan webpack, yang sesuai untuk pembangunan front-end dan back-end. Memilih alat yang betul mengikut keperluan projek dapat meningkatkan kecekapan pembangunan dan kadar kejayaan projek.
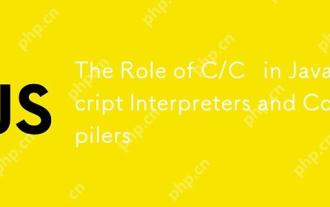
C dan C memainkan peranan penting dalam enjin JavaScript, terutamanya digunakan untuk melaksanakan jurubahasa dan penyusun JIT. 1) C digunakan untuk menghuraikan kod sumber JavaScript dan menghasilkan pokok sintaks abstrak. 2) C bertanggungjawab untuk menjana dan melaksanakan bytecode. 3) C melaksanakan pengkompil JIT, mengoptimumkan dan menyusun kod hot-spot semasa runtime, dan dengan ketara meningkatkan kecekapan pelaksanaan JavaScript.
