


Melaksanakan Sistem Pemprosesan Pesanan: Kesediaan Pengeluaran Bahagian dan Kebolehskalaan
1. Pengenalan dan Matlamat
Selamat datang ke ansuran keenam dan terakhir siri kami untuk melaksanakan sistem pemprosesan pesanan yang canggih! Sepanjang siri ini, kami telah membina sistem berasaskan perkhidmatan mikro yang teguh yang mampu mengendalikan aliran kerja yang kompleks. Kini, tiba masanya untuk meletakkan sentuhan akhir pada sistem kami dan memastikan ia bersedia untuk kegunaan pengeluaran secara berskala.
Rekap Catatan Sebelumnya
- Dalam Bahagian 1, kami menyediakan struktur projek kami dan melaksanakan API CRUD asas.
- Bahagian 2 memfokuskan pada mengembangkan penggunaan Temporal kami untuk aliran kerja yang kompleks.
- Dalam Bahagian 3, kami menyelidiki operasi pangkalan data lanjutan, termasuk pengoptimuman dan sharding.
- Bahagian 4 merangkumi pemantauan dan amaran komprehensif menggunakan Prometheus dan Grafana.
- Dalam Bahagian 5, kami melaksanakan pengesanan teragih dan pembalakan berpusat.
Kepentingan Kesediaan Pengeluaran dan Kebolehskalaan
Semasa kami bersedia untuk menggunakan sistem kami ke pengeluaran, kami perlu memastikan ia boleh mengendalikan beban dunia sebenar, mengekalkan keselamatan dan skala semasa perniagaan kami berkembang. Kesediaan pengeluaran melibatkan menangani kebimbangan seperti pengesahan, pengurusan konfigurasi dan strategi penggunaan. Kebolehskalaan memastikan sistem kami boleh mengendalikan beban yang meningkat tanpa peningkatan sumber yang berkadar.
Gambaran Keseluruhan Topik
Dalam siaran ini, kami akan membincangkan:
- Pengesahan dan Keizinan
- Pengurusan Konfigurasi
- Penghadan Kadar dan Pendikitan
- Mengoptimumkan untuk Keselarasan Tinggi
- Strategi Caching
- Penskalaan Mendatar
- Ujian dan Pengoptimuman Prestasi
- Pemantauan dan Makluman dalam Pengeluaran
- Strategi Penerapan
- Pemulihan Bencana dan Kesinambungan Perniagaan
- Pertimbangan Keselamatan
- Dokumentasi dan Perkongsian Ilmu
Matlamat untuk Bahagian Akhir ini
Menjelang akhir siaran ini, anda akan dapat:
- Laksanakan pengesahan dan kebenaran yang teguh
- Urus konfigurasi dan rahsia dengan selamat
- Lindungi perkhidmatan anda dengan pengehadan kadar dan pendikitan
- Optimumkan sistem anda untuk keselarasan tinggi dan laksanakan caching yang berkesan
- Sediakan sistem anda untuk penskalaan mendatar
- Menjalankan ujian dan pengoptimuman prestasi yang menyeluruh
- Sediakan pemantauan dan makluman gred pengeluaran
- Melaksanakan strategi penggunaan yang selamat dan cekap
- Rancang untuk pemulihan bencana dan pastikan kesinambungan perniagaan
- Atasi pertimbangan keselamatan kritikal
- Buat dokumentasi komprehensif untuk sistem anda
Mari menyelami dan jadikan sistem pemprosesan pesanan kami sedia dan berskala pengeluaran!
2. Melaksanakan Pengesahan dan Kebenaran
Keselamatan adalah terpenting dalam mana-mana sistem pengeluaran. Mari laksanakan pengesahan dan kebenaran yang teguh untuk sistem pemprosesan pesanan kami.
Memilih Strategi Pengesahan
Untuk sistem kami, kami akan menggunakan Token Web JSON (JWT) untuk pengesahan. JWT tidak bernegara, boleh mengandungi tuntutan tentang pengguna dan sesuai untuk seni bina perkhidmatan mikro.
Pertama, mari tambah kebergantungan yang diperlukan:
go get github.com/golang-jwt/jwt/v4 go get golang.org/x/crypto/bcrypt
Melaksanakan Pengesahan Pengguna
Mari buat perkhidmatan pengguna ringkas yang mengendalikan pendaftaran dan log masuk:
package auth import ( "time" "github.com/golang-jwt/jwt/v4" "golang.org/x/crypto/bcrypt" ) type User struct { ID int64 `json:"id"` Username string `json:"username"` Password string `json:"-"` // Never send password in response } type UserService struct { // In a real application, this would be a database users map[string]User } func NewUserService() *UserService { return &UserService{ users: make(map[string]User), } } func (s *UserService) Register(username, password string) error { if _, exists := s.users[username]; exists { return errors.New("user already exists") } hashedPassword, err := bcrypt.GenerateFromPassword([]byte(password), bcrypt.DefaultCost) if err != nil { return err } s.users[username] = User{ ID: int64(len(s.users) + 1), Username: username, Password: string(hashedPassword), } return nil } func (s *UserService) Authenticate(username, password string) (string, error) { user, exists := s.users[username] if !exists { return "", errors.New("user not found") } if err := bcrypt.CompareHashAndPassword([]byte(user.Password), []byte(password)); err != nil { return "", errors.New("invalid password") } token := jwt.NewWithClaims(jwt.SigningMethodHS256, jwt.MapClaims{ "sub": user.ID, "exp": time.Now().Add(time.Hour * 24).Unix(), }) return token.SignedString([]byte("your-secret-key")) }
Kawalan Akses Berasaskan Peranan (RBAC)
Mari kita laksanakan sistem RBAC yang mudah:
type Role string const ( RoleUser Role = "user" RoleAdmin Role = "admin" ) type UserWithRole struct { User Role Role `json:"role"` } func (s *UserService) AssignRole(userID int64, role Role) error { for _, user := range s.users { if user.ID == userID { s.users[user.Username] = UserWithRole{ User: user, Role: role, } return nil } } return errors.New("user not found") }
Menjamin Komunikasi Perkhidmatan-ke-Perkhidmatan
Untuk komunikasi perkhidmatan ke perkhidmatan, kami boleh menggunakan TLS (mTLS) bersama. Berikut ialah contoh mudah tentang cara menyediakan pelayan HTTPS dengan pengesahan sijil klien:
package main import ( "crypto/tls" "crypto/x509" "io/ioutil" "log" "net/http" ) func main() { // Load CA cert caCert, err := ioutil.ReadFile("ca.crt") if err != nil { log.Fatal(err) } caCertPool := x509.NewCertPool() caCertPool.AppendCertsFromPEM(caCert) // Create the TLS Config with the CA pool and enable Client certificate validation tlsConfig := &tls.Config{ ClientCAs: caCertPool, ClientAuth: tls.RequireAndVerifyClientCert, } tlsConfig.BuildNameToCertificate() // Create a Server instance to listen on port 8443 with the TLS config server := &http.Server{ Addr: ":8443", TLSConfig: tlsConfig, } // Listen to HTTPS connections with the server certificate and wait log.Fatal(server.ListenAndServeTLS("server.crt", "server.key")) }
Mengendalikan Kunci API untuk Penyepaduan Luaran
Untuk penyepaduan luaran, kami boleh menggunakan kunci API. Berikut ialah perisian tengah yang mudah untuk menyemak kunci API:
func APIKeyMiddleware(next http.HandlerFunc) http.HandlerFunc { return func(w http.ResponseWriter, r *http.Request) { key := r.Header.Get("X-API-Key") if key == "" { http.Error(w, "Missing API key", http.StatusUnauthorized) return } // In a real application, you would validate the key against a database if key != "valid-api-key" { http.Error(w, "Invalid API key", http.StatusUnauthorized) return } next.ServeHTTP(w, r) } }
Dengan adanya mekanisme pengesahan dan kebenaran ini, kami telah meningkatkan keselamatan sistem pemprosesan pesanan kami dengan ketara. Dalam bahagian seterusnya, kita akan melihat cara mengurus konfigurasi dan rahsia dengan selamat.
3. Pengurusan Konfigurasi
Pengurusan konfigurasi yang betul adalah penting untuk mengekalkan sistem yang fleksibel dan selamat. Mari kita laksanakan sistem pengurusan konfigurasi yang mantap untuk aplikasi pemprosesan pesanan kami.
Melaksanakan Sistem Pengurusan Konfigurasi
Kami akan menggunakan perpustakaan viper yang popular untuk pengurusan konfigurasi. Mula-mula, mari tambahkannya pada projek kami:
go get github.com/spf13/viper
Sekarang, mari buat pengurus konfigurasi:
package config import ( "github.com/spf13/viper" ) type Config struct { Server ServerConfig Database DatabaseConfig Redis RedisConfig } type ServerConfig struct { Port int Host string } type DatabaseConfig struct { Host string Port int User string Password string DBName string } type RedisConfig struct { Host string Port int Password string } func LoadConfig() (*Config, error) { viper.SetConfigName("config") viper.SetConfigType("yaml") viper.AddConfigPath(".") viper.AddConfigPath("$HOME/.orderprocessing") viper.AddConfigPath("/etc/orderprocessing/") viper.AutomaticEnv() if err := viper.ReadInConfig(); err != nil { return nil, err } var config Config if err := viper.Unmarshal(&config); err != nil { return nil, err } return &config, nil }
Using Environment Variables for Configuration
Viper automatically reads environment variables. We can override configuration values by setting environment variables with the prefix ORDERPROCESSING_. For example:
export ORDERPROCESSING_SERVER_PORT=8080 export ORDERPROCESSING_DATABASE_PASSWORD=mysecretpassword
Secrets Management
For managing secrets, we’ll use HashiCorp Vault. First, let’s add the Vault client to our project:
go get github.com/hashicorp/vault/api
Now, let’s create a secrets manager:
package secrets import ( "fmt" vault "github.com/hashicorp/vault/api" ) type SecretsManager struct { client *vault.Client } func NewSecretsManager(address, token string) (*SecretsManager, error) { config := vault.DefaultConfig() config.Address = address client, err := vault.NewClient(config) if err != nil { return nil, fmt.Errorf("unable to initialize Vault client: %w", err) } client.SetToken(token) return &SecretsManager{client: client}, nil } func (sm *SecretsManager) GetSecret(path string) (string, error) { secret, err := sm.client.Logical().Read(path) if err != nil { return "", fmt.Errorf("unable to read secret: %w", err) } if secret == nil { return "", fmt.Errorf("secret not found") } value, ok := secret.Data["value"].(string) if !ok { return "", fmt.Errorf("value is not a string") } return value, nil }
Feature Flags for Controlled Rollouts
For feature flags, we can use a simple in-memory implementation, which can be easily replaced with a distributed solution later:
package featureflags import ( "sync" ) type FeatureFlags struct { flags map[string]bool mu sync.RWMutex } func NewFeatureFlags() *FeatureFlags { return &FeatureFlags{ flags: make(map[string]bool), } } func (ff *FeatureFlags) SetFlag(name string, enabled bool) { ff.mu.Lock() defer ff.mu.Unlock() ff.flags[name] = enabled } func (ff *FeatureFlags) IsEnabled(name string) bool { ff.mu.RLock() defer ff.mu.RUnlock() return ff.flags[name] }
Dynamic Configuration Updates
To support dynamic configuration updates, we can implement a configuration watcher:
package config import ( "log" "time" "github.com/fsnotify/fsnotify" "github.com/spf13/viper" ) func WatchConfig(configPath string, callback func(*Config)) { viper.WatchConfig() viper.OnConfigChange(func(e fsnotify.Event) { log.Println("Config file changed:", e.Name) config, err := LoadConfig() if err != nil { log.Println("Error reloading config:", err) return } callback(config) }) }
With these configuration management tools in place, our system is now more flexible and secure. We can easily manage different configurations for different environments, handle secrets securely, and implement feature flags for controlled rollouts.
In the next section, we’ll implement rate limiting and throttling to protect our services from abuse and ensure fair usage.
4. Rate Limiting and Throttling
Implementing rate limiting and throttling is crucial for protecting your services from abuse, ensuring fair usage, and maintaining system stability under high load.
Implementing Rate Limiting at the API Gateway Level
We’ll implement a simple rate limiter using an in-memory store. In a production environment, you’d want to use a distributed cache like Redis for this.
package ratelimit import ( "net/http" "sync" "time" "golang.org/x/time/rate" ) type IPRateLimiter struct { ips map[string]*rate.Limiter mu *sync.RWMutex r rate.Limit b int } func NewIPRateLimiter(r rate.Limit, b int) *IPRateLimiter { i := &IPRateLimiter{ ips: make(map[string]*rate.Limiter), mu: &sync.RWMutex{}, r: r, b: b, } return i } func (i *IPRateLimiter) AddIP(ip string) *rate.Limiter { i.mu.Lock() defer i.mu.Unlock() limiter := rate.NewLimiter(i.r, i.b) i.ips[ip] = limiter return limiter } func (i *IPRateLimiter) GetLimiter(ip string) *rate.Limiter { i.mu.Lock() limiter, exists := i.ips[ip] if !exists { i.mu.Unlock() return i.AddIP(ip) } i.mu.Unlock() return limiter } func RateLimitMiddleware(next http.HandlerFunc, limiter *IPRateLimiter) http.HandlerFunc { return func(w http.ResponseWriter, r *http.Request) { limiter := limiter.GetLimiter(r.RemoteAddr) if !limiter.Allow() { http.Error(w, http.StatusText(http.StatusTooManyRequests), http.StatusTooManyRequests) return } next.ServeHTTP(w, r) } }
Per-User and Per-IP Rate Limiting
To implement per-user rate limiting, we can modify our rate limiter to use the user ID instead of (or in addition to) the IP address:
func (i *IPRateLimiter) GetLimiterForUser(userID string) *rate.Limiter { i.mu.Lock() limiter, exists := i.ips[userID] if !exists { i.mu.Unlock() return i.AddIP(userID) } i.mu.Unlock() return limiter } func UserRateLimitMiddleware(next http.HandlerFunc, limiter *IPRateLimiter) http.HandlerFunc { return func(w http.ResponseWriter, r *http.Request) { userID := r.Header.Get("X-User-ID") // Assume user ID is passed in header if userID == "" { http.Error(w, "Missing user ID", http.StatusBadRequest) return } limiter := limiter.GetLimiterForUser(userID) if !limiter.Allow() { http.Error(w, http.StatusText(http.StatusTooManyRequests), http.StatusTooManyRequests) return } next.ServeHTTP(w, r) } }
Implementing Backoff Strategies for Retry Logic
When services are rate-limited, it’s important to implement proper backoff strategies for retries. Here’s a simple exponential backoff implementation:
package retry import ( "context" "math" "time" ) func ExponentialBackoff(ctx context.Context, maxRetries int, baseDelay time.Duration, maxDelay time.Duration, operation func() error) error { var err error for i := 0; i < maxRetries; i++ { err = operation() if err == nil { return nil } delay := time.Duration(math.Pow(2, float64(i))) * baseDelay if delay > maxDelay { delay = maxDelay } select { case <-time.After(delay): case <-ctx.Done(): return ctx.Err() } } return err }
Throttling Background Jobs and Batch Processes
For background jobs and batch processes, we can use a worker pool with a limited number of concurrent workers:
package worker import ( "context" "sync" ) type Job func(context.Context) error type WorkerPool struct { workerCount int jobs chan Job results chan error done chan struct{} } func NewWorkerPool(workerCount int) *WorkerPool { return &WorkerPool{ workerCount: workerCount, jobs: make(chan Job), results: make(chan error), done: make(chan struct{}), } } func (wp *WorkerPool) Start(ctx context.Context) { var wg sync.WaitGroup for i := 0; i < wp.workerCount; i++ { wg.Add(1) go func() { defer wg.Done() for { select { case job, ok := <-wp.jobs: if !ok { return } wp.results <- job(ctx) case <-ctx.Done(): return } } }() } go func() { wg.Wait() close(wp.results) close(wp.done) }() } func (wp *WorkerPool) Submit(job Job) { wp.jobs <- job } func (wp *WorkerPool) Results() <-chan error { return wp.results } func (wp *WorkerPool) Done() <-chan struct{} { return wp.done }
Communicating Rate Limit Information to Clients
To help clients manage their request rate, we can include rate limit information in our API responses:
func RateLimitMiddleware(next http.HandlerFunc, limiter *IPRateLimiter) http.HandlerFunc { return func(w http.ResponseWriter, r *http.Request) { limiter := limiter.GetLimiter(r.RemoteAddr) if !limiter.Allow() { w.Header().Set("X-RateLimit-Limit", fmt.Sprintf("%d", limiter.Limit())) w.Header().Set("X-RateLimit-Remaining", "0") w.Header().Set("X-RateLimit-Reset", fmt.Sprintf("%d", time.Now().Add(time.Second).Unix())) http.Error(w, http.StatusText(http.StatusTooManyRequests), http.StatusTooManyRequests) return } w.Header().Set("X-RateLimit-Limit", fmt.Sprintf("%d", limiter.Limit())) w.Header().Set("X-RateLimit-Remaining", fmt.Sprintf("%d", limiter.Tokens())) w.Header().Set("X-RateLimit-Reset", fmt.Sprintf("%d", time.Now().Add(time.Second).Unix())) next.ServeHTTP(w, r) } }
5. Optimizing for High Concurrency
To handle high concurrency efficiently, we need to optimize our system at various levels. Let’s explore some strategies to achieve this.
Implementing Connection Pooling for Databases
Connection pooling helps reduce the overhead of creating new database connections for each request. Here’s how we can implement it using the sql package in Go:
package database import ( "database/sql" "time" _ "github.com/lib/pq" ) func NewDBPool(dataSourceName string) (*sql.DB, error) { db, err := sql.Open("postgres", dataSourceName) if err != nil { return nil, err } // Set maximum number of open connections db.SetMaxOpenConns(25) // Set maximum number of idle connections db.SetMaxIdleConns(25) // Set maximum lifetime of a connection db.SetConnMaxLifetime(5 * time.Minute) return db, nil }
Using Worker Pools for CPU-Bound Tasks
For CPU-bound tasks, we can use a worker pool to limit the number of concurrent operations:
package worker import ( "context" "sync" ) type Task func() error type WorkerPool struct { tasks chan Task results chan error numWorkers int } func NewWorkerPool(numWorkers int) *WorkerPool { return &WorkerPool{ tasks: make(chan Task), results: make(chan error), numWorkers: numWorkers, } } func (wp *WorkerPool) Start(ctx context.Context) { var wg sync.WaitGroup for i := 0; i < wp.numWorkers; i++ { wg.Add(1) go func() { defer wg.Done() for { select { case task, ok := <-wp.tasks: if !ok { return } wp.results <- task() case <-ctx.Done(): return } } }() } go func() { wg.Wait() close(wp.results) }() } func (wp *WorkerPool) Submit(task Task) { wp.tasks <- task } func (wp *WorkerPool) Results() <-chan error { return wp.results }
Leveraging Go’s Concurrency Primitives
Go’s goroutines and channels are powerful tools for handling concurrency. Here’s an example of how we might use them to process orders concurrently:
func ProcessOrders(orders []Order) []error { errChan := make(chan error, len(orders)) var wg sync.WaitGroup for _, order := range orders { wg.Add(1) go func(o Order) { defer wg.Done() if err := processOrder(o); err != nil { errChan <- err } }(order) } go func() { wg.Wait() close(errChan) }() var errs []error for err := range errChan { errs = append(errs, err) } return errs }
Implementing Circuit Breakers for External Service Calls
Circuit breakers can help prevent cascading failures when external services are experiencing issues. Here’s a simple implementation:
package circuitbreaker import ( "errors" "sync" "time" ) type CircuitBreaker struct { mu sync.Mutex failureThreshold uint resetTimeout time.Duration failureCount uint lastFailure time.Time state string } func NewCircuitBreaker(failureThreshold uint, resetTimeout time.Duration) *CircuitBreaker { return &CircuitBreaker{ failureThreshold: failureThreshold, resetTimeout: resetTimeout, state: "closed", } } func (cb *CircuitBreaker) Execute(fn func() error) error { cb.mu.Lock() defer cb.mu.Unlock() if cb.state == "open" { if time.Since(cb.lastFailure) > cb.resetTimeout { cb.state = "half-open" } else { return errors.New("circuit breaker is open") } } err := fn() if err != nil { cb.failureCount++ cb.lastFailure = time.Now() if cb.failureCount >= cb.failureThreshold { cb.state = "open" } return err } if cb.state == "half-open" { cb.state = "closed" } cb.failureCount = 0 return nil }
Optimizing Lock Contention in Concurrent Operations
To reduce lock contention, we can use techniques like sharding or lock-free data structures. Here’s an example of a sharded map:
package shardedmap import ( "hash/fnv" "sync" ) type ShardedMap struct { shards []*Shard } type Shard struct { mu sync.RWMutex data map[string]interface{} } func NewShardedMap(shardCount int) *ShardedMap { sm := &ShardedMap{ shards: make([]*Shard, shardCount), } for i := 0; i < shardCount; i++ { sm.shards[i] = &Shard{ data: make(map[string]interface{}), } } return sm } func (sm *ShardedMap) getShard(key string) *Shard { hash := fnv.New32() hash.Write([]byte(key)) return sm.shards[hash.Sum32()%uint32(len(sm.shards))] } func (sm *ShardedMap) Set(key string, value interface{}) { shard := sm.getShard(key) shard.mu.Lock() defer shard.mu.Unlock() shard.data[key] = value } func (sm *ShardedMap) Get(key string) (interface{}, bool) { shard := sm.getShard(key) shard.mu.RLock() defer shard.mu.RUnlock() val, ok := shard.data[key] return val, ok }
By implementing these optimizations, our order processing system will be better equipped to handle high concurrency scenarios. In the next section, we’ll explore caching strategies to further improve performance and scalability.
6. Caching Strategies
Implementing effective caching strategies can significantly improve the performance and scalability of our order processing system. Let’s explore various caching techniques and their implementations.
Implementing Application-Level Caching
We’ll use Redis for our application-level cache. First, let’s set up a Redis client:
package cache import ( "context" "encoding/json" "time" "github.com/go-redis/redis/v8" ) type RedisCache struct { client *redis.Client } func NewRedisCache(addr string) *RedisCache { client := redis.NewClient(&redis.Options{ Addr: addr, }) return &RedisCache{client: client} } func (c *RedisCache) Set(ctx context.Context, key string, value interface{}, expiration time.Duration) error { json, err := json.Marshal(value) if err != nil { return err } return c.client.Set(ctx, key, json, expiration).Err() } func (c *RedisCache) Get(ctx context.Context, key string, dest interface{}) error { val, err := c.client.Get(ctx, key).Result() if err != nil { return err } return json.Unmarshal([]byte(val), dest) }
Cache Invalidation Strategies
Implementing an effective cache invalidation strategy is crucial. Let’s implement a simple time-based and version-based invalidation:
func (c *RedisCache) SetWithVersion(ctx context.Context, key string, value interface{}, version int, expiration time.Duration) error { data := struct { Value interface{} `json:"value"` Version int `json:"version"` }{ Value: value, Version: version, } return c.Set(ctx, key, data, expiration) } func (c *RedisCache) GetWithVersion(ctx context.Context, key string, dest interface{}, currentVersion int) (bool, error) { var data struct { Value json.RawMessage `json:"value"` Version int `json:"version"` } err := c.Get(ctx, key, &data) if err != nil { return false, err } if data.Version != currentVersion { return false, nil } return true, json.Unmarshal(data.Value, dest) }
Implementing a Distributed Cache for Scalability
For a distributed cache, we can use Redis Cluster. Here’s how we might set it up:
func NewRedisClusterCache(addrs []string) *RedisCache { client := redis.NewClusterClient(&redis.ClusterOptions{ Addrs: addrs, }) return &RedisCache{client: client} }
Using Read-Through and Write-Through Caching Patterns
Let’s implement a read-through caching pattern:
func GetOrder(ctx context.Context, cache *RedisCache, db *sql.DB, orderID string) (Order, error) { var order Order // Try to get from cache err := cache.Get(ctx, "order:"+orderID, &order) if err == nil { return order, nil } // If not in cache, get from database order, err = getOrderFromDB(ctx, db, orderID) if err != nil { return Order{}, err } // Store in cache for future requests cache.Set(ctx, "order:"+orderID, order, 1*time.Hour) return order, nil }
And a write-through caching pattern:
func CreateOrder(ctx context.Context, cache *RedisCache, db *sql.DB, order Order) error { // Store in database err := storeOrderInDB(ctx, db, order) if err != nil { return err } // Store in cache return cache.Set(ctx, "order:"+order.ID, order, 1*time.Hour) }
Caching in Different Layers
We can implement caching at different layers of our application. For example, we might cache database query results:
func GetOrdersByUser(ctx context.Context, cache *RedisCache, db *sql.DB, userID string) ([]Order, error) { var orders []Order // Try to get from cache err := cache.Get(ctx, "user_orders:"+userID, &orders) if err == nil { return orders, nil } // If not in cache, query database orders, err = getOrdersByUserFromDB(ctx, db, userID) if err != nil { return nil, err } // Store in cache for future requests cache.Set(ctx, "user_orders:"+userID, orders, 15*time.Minute) return orders, nil }
We might also implement HTTP caching headers in our API responses:
func OrderHandler(w http.ResponseWriter, r *http.Request) { // ... get order ... w.Header().Set("Cache-Control", "public, max-age=300") w.Header().Set("ETag", calculateETag(order)) json.NewEncoder(w).Encode(order) }
7. Preparing for Horizontal Scaling
As our order processing system grows, we need to ensure it can scale horizontally. Let’s explore strategies to achieve this.
Designing Stateless Services for Easy Scaling
Ensure your services are stateless by moving all state to external stores (databases, caches, etc.):
type OrderService struct { DB *sql.DB Cache *RedisCache } func (s *OrderService) GetOrder(ctx context.Context, orderID string) (Order, error) { // All state is stored in the database or cache return GetOrder(ctx, s.Cache, s.DB, orderID) }
Implementing Service Discovery and Registration
We can use a service like Consul for service discovery. Here’s a simple wrapper:
package discovery import ( "github.com/hashicorp/consul/api" ) type ServiceDiscovery struct { client *api.Client } func NewServiceDiscovery(address string) (*ServiceDiscovery, error) { config := api.DefaultConfig() config.Address = address client, err := api.NewClient(config) if err != nil { return nil, err } return &ServiceDiscovery{client: client}, nil } func (sd *ServiceDiscovery) Register(name, address string, port int) error { return sd.client.Agent().ServiceRegister(&api.AgentServiceRegistration{ Name: name, Address: address, Port: port, }) } func (sd *ServiceDiscovery) Discover(name string) ([]*api.ServiceEntry, error) { return sd.client.Health().Service(name, "", true, nil) }
Load Balancing Strategies
Implement a simple round-robin load balancer:
type LoadBalancer struct { services []*api.ServiceEntry current int } func NewLoadBalancer(services []*api.ServiceEntry) *LoadBalancer { return &LoadBalancer{ services: services, current: 0, } } func (lb *LoadBalancer) Next() *api.ServiceEntry { service := lb.services[lb.current] lb.current = (lb.current + 1) % len(lb.services) return service }
Handling Distributed Transactions in a Scalable Way
For distributed transactions, we can use the Saga pattern. Here’s a simple implementation:
type Saga struct { actions []func() error compensations []func() error } func (s *Saga) AddStep(action, compensation func() error) { s.actions = append(s.actions, action) s.compensations = append(s.compensations, compensation) } func (s *Saga) Execute() error { for i, action := range s.actions { if err := action(); err != nil { // Compensate for the error for j := i - 1; j >= 0; j-- { s.compensations[j]() } return err } } return nil }
Scaling the Database Layer
For database scaling, we can implement read replicas and sharding. Here’s a simple sharding strategy:
type ShardedDB struct { shards []*sql.DB } func (sdb *ShardedDB) Shard(key string) *sql.DB { hash := fnv.New32a() hash.Write([]byte(key)) return sdb.shards[hash.Sum32()%uint32(len(sdb.shards))] } func (sdb *ShardedDB) ExecOnShard(key string, query string, args ...interface{}) (sql.Result, error) { return sdb.Shard(key).Exec(query, args...) }
By implementing these strategies, our order processing system will be well-prepared for horizontal scaling. In the next section, we’ll cover performance testing and optimization to ensure our system can handle increased load efficiently.
8. Performance Testing and Optimization
To ensure our order processing system can handle the expected load and perform efficiently, we need to conduct thorough performance testing and optimization.
Setting up a Performance Testing Environment
First, let’s set up a performance testing environment using a tool like k6:
import http from 'k6/http'; import { sleep } from 'k6'; export let options = { vus: 100, duration: '5m', }; export default function() { let payload = JSON.stringify({ userId: 'user123', items: [ { productId: 'prod456', quantity: 2 }, { productId: 'prod789', quantity: 1 }, ], }); let params = { headers: { 'Content-Type': 'application/json', }, }; http.post('http://api.example.com/orders', payload, params); sleep(1); }
Conducting Load Tests and Stress Tests
Run the load test:
k6 run loadtest.js
For stress testing, gradually increase the number of virtual users until the system starts to show signs of stress.
Profiling and Optimizing Go Code
Use Go’s built-in profiler to identify bottlenecks:
import ( "net/http" _ "net/http/pprof" "runtime" ) func main() { runtime.SetBlockProfileRate(1) go func() { http.ListenAndServe("localhost:6060", nil) }() // Rest of your application code... }
Then use go tool pprof to analyze the profile:
go tool pprof http://localhost:6060/debug/pprof/profile
Database Query Optimization
Use EXPLAIN to analyze and optimize your database queries:
EXPLAIN ANALYZE SELECT * FROM orders WHERE user_id = 'user123';
Based on the results, you might add indexes:
CREATE INDEX idx_orders_user_id ON orders(user_id);
Identifying and Resolving Bottlenecks
Use tools like httptrace to identify network-related bottlenecks:
import ( "net/http/httptrace" "time" ) func traceHTTP(req *http.Request) { trace := &httptrace.ClientTrace{ GotConn: func(info httptrace.GotConnInfo) { fmt.Printf("Connection reused: %v\n", info.Reused) }, GotFirstResponseByte: func() { fmt.Printf("First byte received: %v\n", time.Now()) }, } req = req.WithContext(httptrace.WithClientTrace(req.Context(), trace)) // Make the request... }
9. Monitoring and Alerting in Production
Effective monitoring and alerting are crucial for maintaining a healthy production system.
Setting up Production-Grade Monitoring
Implement a monitoring solution using Prometheus and Grafana. First, instrument your code with Prometheus metrics:
import ( "github.com/prometheus/client_golang/prometheus" "github.com/prometheus/client_golang/prometheus/promauto" ) var ( ordersProcessed = promauto.NewCounter(prometheus.CounterOpts{ Name: "orders_processed_total", Help: "The total number of processed orders", }) ) func processOrder(order Order) { // Process the order... ordersProcessed.Inc() }
Implementing Health Checks and Readiness Probes
Add health check and readiness endpoints:
func healthCheckHandler(w http.ResponseWriter, r *http.Request) { w.WriteHeader(http.StatusOK) w.Write([]byte("OK")) } func readinessHandler(w http.ResponseWriter, r *http.Request) { // Check if the application is ready to serve traffic if isReady() { w.WriteHeader(http.StatusOK) w.Write([]byte("Ready")) } else { w.WriteHeader(http.StatusServiceUnavailable) w.Write([]byte("Not Ready")) } }
Creating SLOs (Service Level Objectives) and SLAs (Service Level Agreements)
Define SLOs for your system, for example:
- 99.9% of orders should be processed within 5 seconds
- The system should have 99.99% uptime
Implement tracking for these SLOs:
var ( orderProcessingDuration = promauto.NewHistogram(prometheus.HistogramOpts{ Name: "order_processing_duration_seconds", Help: "Duration of order processing in seconds", Buckets: []float64{0.1, 0.5, 1, 2, 5}, }) ) func processOrder(order Order) { start := time.Now() // Process the order... duration := time.Since(start).Seconds() orderProcessingDuration.Observe(duration) }
Setting up Alerting for Critical Issues
Configure alerting rules in Prometheus. For example:
groups: - name: example rules: - alert: HighOrderProcessingTime expr: histogram_quantile(0.95, rate(order_processing_duration_seconds_bucket[5m])) > 5 for: 10m labels: severity: critical annotations: summary: High order processing time
Implementing On-Call Rotations and Incident Response Procedures
Set up an on-call rotation using a tool like PagerDuty. Define incident response procedures, for example:
- Acknowledge the alert
- Assess the severity of the issue
- Start a video call with the on-call team if necessary
- Investigate and resolve the issue
- Write a post-mortem report
10. Deployment Strategies
Implementing safe and efficient deployment strategies is crucial for maintaining system reliability while allowing for frequent updates.
Implementing CI/CD Pipelines
Set up a CI/CD pipeline using a tool like GitLab CI. Here’s an example .gitlab-ci.yml:
stages: - test - build - deploy test: stage: test script: - go test ./... build: stage: build script: - docker build -t myapp . only: - master deploy: stage: deploy script: - kubectl apply -f k8s/ only: - master
Blue-Green Deployments
Implement blue-green deployments to minimize downtime:
func blueGreenDeploy(newVersion string) error { // Deploy new version if err := deployVersion(newVersion); err != nil { return err } // Run health checks on new version if err := runHealthChecks(newVersion); err != nil { rollback(newVersion) return err } // Switch traffic to new version if err := switchTraffic(newVersion); err != nil { rollback(newVersion) return err } return nil }
Canary Releases
Implement canary releases to gradually roll out changes:
func canaryRelease(newVersion string, percentage int) error { // Deploy new version if err := deployVersion(newVersion); err != nil { return err } // Gradually increase traffic to new version for p := 1; p <= percentage; p++ { if err := setTrafficPercentage(newVersion, p); err != nil { rollback(newVersion) return err } time.Sleep(5 * time.Minute) if err := runHealthChecks(newVersion); err != nil { rollback(newVersion) return err } } return nil }
Rollback Strategies
Implement a rollback mechanism:
func rollback(version string) error { previousVersion := getPreviousVersion() if err := switchTraffic(previousVersion); err != nil { return err } if err := removeVersion(version); err != nil { return err } return nil }
Managing Database Migrations in Production
Use a database migration tool like golang-migrate:
import "github.com/golang-migrate/migrate/v4" func runMigrations(dbURL string) error { m, err := migrate.New( "file://migrations", dbURL, ) if err != nil { return err } if err := m.Up(); err != nil && err != migrate.ErrNoChange { return err } return nil }
By implementing these deployment strategies, we can ensure that our order processing system remains reliable and up-to-date, while minimizing the risk of downtime or errors during updates.
In the next sections, we’ll cover disaster recovery, business continuity, and security considerations to further enhance the robustness of our system.
11. Disaster Recovery and Business Continuity
Ensuring our system can recover from disasters and maintain business continuity is crucial for a production-ready application.
Implementing Regular Backups
Set up a regular backup schedule for your databases and critical data:
import ( "os/exec" "time" ) func performBackup() error { cmd := exec.Command("pg_dump", "-h", "localhost", "-U", "username", "-d", "database", "-f", "backup.sql") return cmd.Run() } func scheduleBackups() { ticker := time.NewTicker(24 * time.Hour) for { select { case <-ticker.C: if err := performBackup(); err != nil { log.Printf("Backup failed: %v", err) } } } }
Setting up Cross-Region Replication
Implement cross-region replication for your databases to ensure data availability in case of regional outages:
func setupCrossRegionReplication(primaryDB, replicaDB *sql.DB) error { // Set up logical replication on the primary if _, err := primaryDB.Exec("CREATE PUBLICATION my_publication FOR ALL TABLES"); err != nil { return err } // Set up subscription on the replica if _, err := replicaDB.Exec("CREATE SUBSCRIPTION my_subscription CONNECTION 'host=primary dbname=mydb' PUBLICATION my_publication"); err != nil { return err } return nil }
Disaster Recovery Planning and Testing
Create a disaster recovery plan and regularly test it:
func testDisasterRecovery() error { // Simulate primary database failure if err := shutdownPrimaryDB(); err != nil { return err } // Promote replica to primary if err := promoteReplicaToPrimary(); err != nil { return err } // Update application configuration to use new primary if err := updateDBConfig(); err != nil { return err } // Verify system functionality if err := runSystemTests(); err != nil { return err } return nil }
Implementing Chaos Engineering Principles
Introduce controlled chaos to test system resilience:
import "github.com/DataDog/chaos-controller/types" func setupChaosTests() { chaosConfig := types.ChaosConfig{ Attacks: []types.AttackInfo{ { Attack: types.CPUPressure, ConfigMap: map[string]string{ "intensity": "50", }, }, { Attack: types.NetworkCorruption, ConfigMap: map[string]string{ "corruption": "30", }, }, }, } chaosController := chaos.NewController(chaosConfig) chaosController.Start() }
Managing Data Integrity During Recovery Scenarios
Implement data integrity checks during recovery:
func verifyDataIntegrity() error { // Check for any inconsistencies in order data if err := checkOrderConsistency(); err != nil { return err } // Verify inventory levels if err := verifyInventoryLevels(); err != nil { return err } // Ensure all payments are accounted for if err := reconcilePayments(); err != nil { return err } return nil }
12. Security Considerations
Ensuring the security of our order processing system is paramount. Let’s address some key security considerations.
Implementing Regular Security Audits
Schedule regular security audits:
func performSecurityAudit() error { // Run automated vulnerability scans if err := runVulnerabilityScans(); err != nil { return err } // Review access controls if err := auditAccessControls(); err != nil { return err } // Check for any suspicious activity in logs if err := analyzeLogs(); err != nil { return err } return nil }
Managing Dependencies and Addressing Vulnerabilities
Regularly update dependencies and scan for vulnerabilities:
import "github.com/sonatard/go-mod-up" func updateDependencies() error { if err := modUp.Run(modUp.Options{}); err != nil { return err } // Run security scan cmd := exec.Command("gosec", "./...") return cmd.Run() }
Implementing Proper Error Handling to Prevent Information Leakage
Ensure errors don’t leak sensitive information:
func handleError(err error, w http.ResponseWriter) { log.Printf("Internal error: %v", err) http.Error(w, "An internal error occurred", http.StatusInternalServerError) }
Setting up a Bug Bounty Program
Consider setting up a bug bounty program to encourage security researchers to responsibly disclose vulnerabilities:
func setupBugBountyProgram() { // This would typically involve setting up a page on your website or using a service like HackerOne http.HandleFunc("/security/bug-bounty", func(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Our bug bounty program details and rules can be found here...") }) }
Compliance with Relevant Standards
Ensure compliance with relevant standards such as PCI DSS for payment processing:
func ensurePCIDSSCompliance() error { // Implement PCI DSS requirements if err := encryptSensitiveData(); err != nil { return err } if err := implementAccessControls(); err != nil { return err } if err := setupSecureNetworks(); err != nil { return err } // ... other PCI DSS requirements return nil }
13. Documentation and Knowledge Sharing
Comprehensive documentation is crucial for maintaining and scaling a complex system like our order processing application.
Creating Comprehensive System Documentation
Document your system architecture, components, and interactions:
func generateSystemDocumentation() error { doc := &SystemDocumentation{ Architecture: describeArchitecture(), Components: listComponents(), Interactions: describeInteractions(), } return doc.SaveToFile("system_documentation.md") }
Implementing API Documentation
Use a tool like Swagger to document your API:
// @title Order Processing API // @version 1.0 // @description This is the API for our order processing system // @host localhost:8080 // @BasePath /api/v1 func main() { r := gin.Default() v1 := r.Group("/api/v1") { v1.POST("/orders", createOrder) v1.GET("/orders/:id", getOrder) // ... other routes } r.Run() } // @Summary Create a new order // @Description Create a new order with the input payload // @Accept json // @Produce json // @Param order body Order true "Create order" // @Success 200 {object} Order // @Router /orders [post] func createOrder(c *gin.Context) { // Implementation }
Setting up a Knowledge Base for Common Issues and Resolutions
Create a knowledge base to document common issues and their resolutions:
type KnowledgeBaseEntry struct { Issue string Resolution string DateAdded time.Time } func addToKnowledgeBase(issue, resolution string) error { entry := KnowledgeBaseEntry{ Issue: issue, Resolution: resolution, DateAdded: time.Now(), } // In a real scenario, this would be saved to a database return saveEntryToDB(entry) }
Creating Runbooks for Operational Tasks
Develop runbooks for common operational tasks:
type Runbook struct { Name string Description string Steps []string } func createDeploymentRunbook() Runbook { return Runbook{ Name: "Deployment Process", Description: "Steps to deploy a new version of the application", Steps: []string{ "1. Run all tests", "2. Build Docker image", "3. Push image to registry", "4. Update Kubernetes manifests", "5. Apply Kubernetes updates", "6. Monitor deployment progress", "7. Run post-deployment tests", }, } }
Implementing a System for Capturing and Sharing Lessons Learned
Set up a process for capturing and sharing lessons learned:
type LessonLearned struct { Incident string Description string LessonsLearned []string DateAdded time.Time } func addLessonLearned(incident, description string, lessons []string) error { entry := LessonLearned{ Incident: incident, Description: description, LessonsLearned: lessons, DateAdded: time.Now(), } // In a real scenario, this would be saved to a database return saveEntryToDB(entry) }
14. Future Considerations and Potential Improvements
As we look to the future, there are several areas where we could further improve our order processing system.
Potential Migration to Kubernetes for Orchestration
Consider migrating to Kubernetes for improved orchestration and scaling:
func deployToKubernetes() error { cmd := exec.Command("kubectl", "apply", "-f", "k8s-manifests/") return cmd.Run() }
Exploring Serverless Architectures for Certain Components
Consider moving some components to a serverless architecture:
import ( "github.com/aws/aws-lambda-go/lambda" ) func handleOrder(request events.APIGatewayProxyRequest) (events.APIGatewayProxyResponse, error) { // Process order // ... return events.APIGatewayProxyResponse{ StatusCode: 200, Body: "Order processed successfully", }, nil } func main() { lambda.Start(handleOrder) }
Considering Event-Driven Architectures for Further Decoupling
Implement an event-driven architecture for improved decoupling:
type OrderEvent struct { Type string Order Order } func publishOrderEvent(event OrderEvent) error { // Publish event to message broker // ... } func handleOrderCreated(order Order) error { return publishOrderEvent(OrderEvent{Type: "OrderCreated", Order: order}) }
Potential Use of GraphQL for More Flexible APIs
Consider implementing GraphQL for more flexible APIs:
import ( "github.com/graphql-go/graphql" ) var orderType = graphql.NewObject( graphql.ObjectConfig{ Name: "Order", Fields: graphql.Fields{ "id": &graphql.Field{ Type: graphql.String, }, "customerName": &graphql.Field{ Type: graphql.String, }, // ... other fields }, }, ) var queryType = graphql.NewObject( graphql.ObjectConfig{ Name: "Query", Fields: graphql.Fields{ "order": &graphql.Field{ Type: orderType, Args: graphql.FieldConfigArgument{ "id": &graphql.ArgumentConfig{ Type: graphql.String, }, }, Resolve: func(p graphql.ResolveParams) (interface{}, error) { // Fetch order by ID // ... }, }, }, }, )
Exploring Machine Learning for Demand Forecasting and Fraud Detection
Consider implementing machine learning models for demand forecasting and fraud detection:
import ( "github.com/sajari/regression" ) func predictDemand(historicalData []float64) (float64, error) { r := new(regression.Regression) r.SetObserved("demand") r.SetVar(0, "time") for i, demand := range historicalData { r.Train(regression.DataPoint(demand, []float64{float64(i)})) } r.Run() return r.Predict([]float64{float64(len(historicalData))}) }
15. Conclusion and Series Wrap-up
In this final post of our series, we’ve covered the crucial aspects of making our order processing system production-ready and scalable. We’ve implemented robust monitoring and alerting, set up effective deployment strategies, addressed security concerns, and planned for disaster recovery.
We’ve also looked at ways to document our system effectively and share knowledge among team members. Finally, we’ve considered potential future improvements to keep our system at the cutting edge of technology.
Dengan mengikuti amalan dan melaksanakan contoh kod yang telah kami bincangkan sepanjang siri ini, anda kini seharusnya mempunyai asas yang kukuh untuk membina, menggunakan dan mengekalkan sistem pemprosesan pesanan yang sedia pengeluaran dan boleh skala.
Ingat, membina sistem yang mantap adalah proses yang berterusan. Teruskan memantau, menguji dan memperbaik sistem anda apabila perniagaan anda berkembang dan teknologi berkembang. Kekal ingin tahu, teruskan belajar dan selamat mengekod!
Perlukan Bantuan?
Adakah anda menghadapi masalah yang mencabar, atau memerlukan perspektif luaran tentang idea atau projek baharu? Saya boleh tolong! Sama ada anda ingin membina konsep bukti teknologi sebelum membuat pelaburan yang lebih besar, atau anda memerlukan panduan tentang isu yang sukar, saya sedia membantu.
Perkhidmatan yang Ditawarkan:
- Penyelesaian Masalah: Menangani isu yang rumit dengan penyelesaian yang inovatif.
- Perundingan: Memberikan nasihat pakar dan pandangan baharu tentang projek anda.
- Bukti Konsep: Membangunkan model awal untuk menguji dan mengesahkan idea anda.
Jika anda berminat untuk bekerja dengan saya, sila hubungi melalui e-mel di hungaikevin@gmail.com.
Mari jadikan cabaran anda sebagai peluang!
Atas ialah kandungan terperinci Melaksanakan Sistem Pemprosesan Pesanan: Kesediaan Pengeluaran Bahagian dan Kebolehskalaan. Untuk maklumat lanjut, sila ikut artikel berkaitan lain di laman web China PHP!

Alat AI Hot

Undresser.AI Undress
Apl berkuasa AI untuk mencipta foto bogel yang realistik

AI Clothes Remover
Alat AI dalam talian untuk mengeluarkan pakaian daripada foto.

Undress AI Tool
Gambar buka pakaian secara percuma

Clothoff.io
Penyingkiran pakaian AI

Video Face Swap
Tukar muka dalam mana-mana video dengan mudah menggunakan alat tukar muka AI percuma kami!

Artikel Panas

Alat panas

Notepad++7.3.1
Editor kod yang mudah digunakan dan percuma

SublimeText3 versi Cina
Versi Cina, sangat mudah digunakan

Hantar Studio 13.0.1
Persekitaran pembangunan bersepadu PHP yang berkuasa

Dreamweaver CS6
Alat pembangunan web visual

SublimeText3 versi Mac
Perisian penyuntingan kod peringkat Tuhan (SublimeText3)

Topik panas










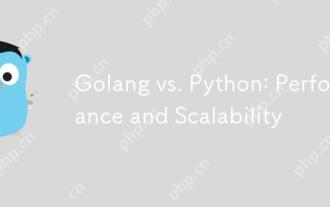
Golang lebih baik daripada Python dari segi prestasi dan skalabiliti. 1) Ciri-ciri jenis kompilasi Golang dan model konkurensi yang cekap menjadikannya berfungsi dengan baik dalam senario konvensional yang tinggi. 2) Python, sebagai bahasa yang ditafsirkan, melaksanakan perlahan -lahan, tetapi dapat mengoptimumkan prestasi melalui alat seperti Cython.
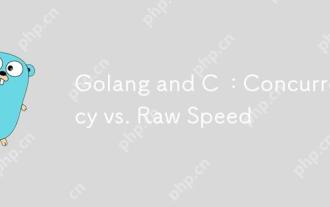
Golang lebih baik daripada C dalam kesesuaian, manakala C lebih baik daripada Golang dalam kelajuan mentah. 1) Golang mencapai kesesuaian yang cekap melalui goroutine dan saluran, yang sesuai untuk mengendalikan sejumlah besar tugas serentak. 2) C Melalui pengoptimuman pengkompil dan perpustakaan standard, ia menyediakan prestasi tinggi yang dekat dengan perkakasan, sesuai untuk aplikasi yang memerlukan pengoptimuman yang melampau.
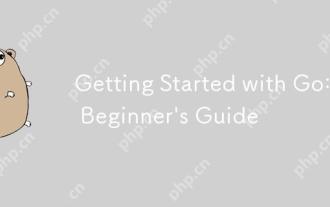
GoisidealforbeginnersandSuekableforcloudandnetworkservicesduetoitssimplicity, kecekapan, danconcurrencyfeatures.1) installgofromtheofficialwebsiteandverifywith'goversion'.2)
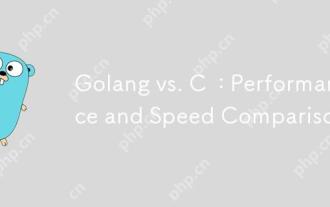
Golang sesuai untuk pembangunan pesat dan senario serentak, dan C sesuai untuk senario di mana prestasi ekstrem dan kawalan peringkat rendah diperlukan. 1) Golang meningkatkan prestasi melalui pengumpulan sampah dan mekanisme konvensional, dan sesuai untuk pembangunan perkhidmatan web yang tinggi. 2) C mencapai prestasi muktamad melalui pengurusan memori manual dan pengoptimuman pengkompil, dan sesuai untuk pembangunan sistem tertanam.
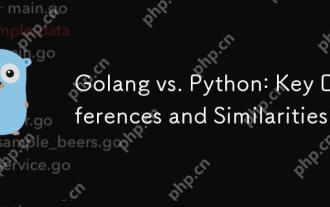
Golang dan Python masing -masing mempunyai kelebihan mereka sendiri: Golang sesuai untuk prestasi tinggi dan pengaturcaraan serentak, sementara Python sesuai untuk sains data dan pembangunan web. Golang terkenal dengan model keserasiannya dan prestasi yang cekap, sementara Python terkenal dengan sintaks ringkas dan ekosistem perpustakaan yang kaya.
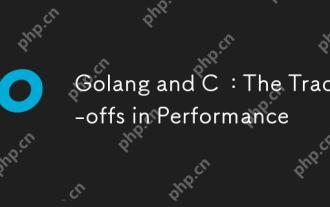
Perbezaan prestasi antara Golang dan C terutamanya ditunjukkan dalam pengurusan ingatan, pengoptimuman kompilasi dan kecekapan runtime. 1) Mekanisme pengumpulan sampah Golang adalah mudah tetapi boleh menjejaskan prestasi, 2) Pengurusan memori manual C dan pengoptimuman pengkompil lebih cekap dalam pengkomputeran rekursif.
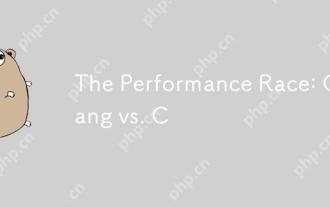
Golang dan C masing-masing mempunyai kelebihan sendiri dalam pertandingan prestasi: 1) Golang sesuai untuk kesesuaian tinggi dan perkembangan pesat, dan 2) C menyediakan prestasi yang lebih tinggi dan kawalan halus. Pemilihan harus berdasarkan keperluan projek dan tumpukan teknologi pasukan.
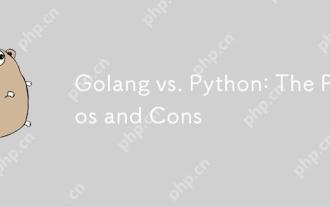
Golangisidealforbuildingscalablesystemsduetoitseficiencyandcurrency, whilepythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.golang'sdesignencouragescouragescouragescouragescourageSlean, readablecodeanditsouragescouragescourscean,
