


Pelajaran Bekerja dengan API dan Pengikisan Web untuk Automasi HR
Python from 0 to Hero 시리즈에 다시 오신 것을 환영합니다! 지금까지 우리는 급여 및 HR 시스템과 관련된 작업을 위해 데이터를 조작하고 강력한 외부 라이브러리를 사용하는 방법을 배웠습니다. 하지만 실시간 데이터를 가져오거나 외부 서비스와 상호작용해야 한다면 어떻게 해야 할까요? 바로 이것이 API와 웹 스크래핑이 중요한 역할을 하는 곳입니다.
이 강의에서 다룰 내용은 다음과 같습니다.
- API란 무엇이며 왜 유용한가요?
- Python의 요청 라이브러리를 사용하여 REST API와 상호작용하는 방법
- 웹 스크래핑 기술을 적용하여 웹사이트에서 데이터를 추출하는 방법
- 급여에 대한 실시간 세율 가져오기 또는 웹사이트에서 직원 복리후생 데이터 스크랩과 같은 실제 사례.
이 강의가 끝나면 외부 데이터 검색을 자동화하여 HR 시스템을 더욱 동적이고 데이터 중심적으로 만들 수 있게 됩니다.
1. API란 무엇입니까?
API(애플리케이션 프로그래밍 인터페이스)는 서로 다른 소프트웨어 애플리케이션이 서로 통신할 수 있도록 하는 일련의 규칙입니다. 간단히 말해서, 코드에서 직접 다른 서비스나 데이터베이스와 상호 작용할 수 있습니다.
예:
- API를 사용하여 급여 계산을 위한 실시간 세율을 가져올 수 있습니다.
- HR 소프트웨어 API와 통합하여 직원 데이터를 시스템으로 직접 가져올 수 있습니다.
- 또는 날씨 API를 사용하여 극단적인 기상 조건에 따라 직원에게 특별 혜택을 제공할 시기를 알 수 있습니다.
대부분의 API는 REST(Representational State Transfer)라는 표준을 사용합니다. 이를 통해 HTTP 요청(예: GET 또는 POST)을 보내 데이터에 액세스하거나 업데이트할 수 있습니다.
2. 요청 라이브러리를 사용하여 API와 상호작용
Python의 요청 라이브러리를 사용하면 API 작업이 쉬워집니다. 다음을 실행하여 설치할 수 있습니다.
pip install requests
기본 API 요청하기
GET 요청을 사용하여 API에서 데이터를 가져오는 방법에 대한 간단한 예부터 시작해 보겠습니다.
import requests # Example API to get public data url = "https://jsonplaceholder.typicode.com/users" response = requests.get(url) # Check if the request was successful (status code 200) if response.status_code == 200: data = response.json() # Parse the response as JSON print(data) else: print(f"Failed to retrieve data. Status code: {response.status_code}")
이 예에서는:
- Requests.get() 함수를 사용하여 API에서 데이터를 가져옵니다.
- 요청이 성공하면 데이터가 JSON으로 구문 분석되어 처리됩니다.
HR 애플리케이션 예: 실시간 세금 데이터 가져오기
급여 목적으로 실시간 세율을 가져오고 싶다고 가정해 보겠습니다. 많은 국가에서 세율에 대한 공개 API를 제공합니다.
이 예에서는 세금 API에서 데이터 가져오기를 시뮬레이션합니다. 실제 API를 사용해도 로직은 비슷할 것입니다.
import requests # Simulated API for tax rates api_url = "https://api.example.com/tax-rates" response = requests.get(api_url) if response.status_code == 200: tax_data = response.json() federal_tax = tax_data['federal_tax'] state_tax = tax_data['state_tax'] print(f"Federal Tax Rate: {federal_tax}%") print(f"State Tax Rate: {state_tax}%") # Use the tax rates to calculate total tax for an employee's salary salary = 5000 total_tax = salary * (federal_tax + state_tax) / 100 print(f"Total tax for a salary of ${salary}: ${total_tax:.2f}") else: print(f"Failed to retrieve tax rates. Status code: {response.status_code}")
이 스크립트는 실제 세율 API와 함께 작동하도록 조정될 수 있으므로 급여 시스템을 최신 세율로 최신 상태로 유지하는 데 도움이 됩니다.
3. 데이터 수집을 위한 웹 스크래핑
API는 데이터를 가져오는 데 선호되는 방법이지만 모든 웹사이트에서 API를 제공하는 것은 아닙니다. 이러한 경우 웹 스크래핑을 사용하여 웹페이지에서 데이터를 추출할 수 있습니다.
Python의 BeautifulSoup 라이브러리는 요청과 함께 웹 스크래핑을 쉽게 만듭니다. 다음을 실행하여 설치할 수 있습니다.
pip install beautifulsoup4
예: 웹사이트에서 직원 복리후생 데이터 스크래핑
회사의 HR 웹사이트에서 직원 복리후생에 대한 데이터를 스크랩한다고 가정해 보세요. 기본적인 예는 다음과 같습니다.
import requests from bs4 import BeautifulSoup # URL of the webpage you want to scrape url = "https://example.com/employee-benefits" response = requests.get(url) # Parse the page content with BeautifulSoup soup = BeautifulSoup(response.content, 'html.parser') # Find and extract the data you need (e.g., benefits list) benefits = soup.find_all("div", class_="benefit-item") # Loop through and print out the benefits for benefit in benefits: title = benefit.find("h3").get_text() description = benefit.find("p").get_text() print(f"Benefit: {title}") print(f"Description: {description}\n")
이 예에서는:
- requests.get()을 사용하여 웹페이지의 콘텐츠를 요청합니다.
- BeautifulSoup 개체는 HTML 콘텐츠를 구문 분석합니다.
- 그런 다음 find_all()을 사용하여 관심 있는 특정 요소(예: 혜택 제목 및 설명)를 추출합니다.
이 기술은 복리후생, 채용 공고, 급여 벤치마크 등 HR 관련 데이터를 웹에서 수집하는 데 유용합니다.
4. HR 애플리케이션에서 API와 웹 스크래핑 결합
모든 것을 하나로 모아 실제 HR 시나리오에 맞게 API 사용과 웹 스크래핑을 결합한 미니 애플리케이션을 만들어 보겠습니다. 직원의 총 비용을 계산합니다.
우리는:
- Use an API to get real-time tax rates.
- Scrape a webpage for additional employee benefit costs.
Example: Total Employee Cost Calculator
import requests from bs4 import BeautifulSoup # Step 1: Get tax rates from API def get_tax_rates(): api_url = "https://api.example.com/tax-rates" response = requests.get(api_url) if response.status_code == 200: tax_data = response.json() federal_tax = tax_data['federal_tax'] state_tax = tax_data['state_tax'] return federal_tax, state_tax else: print("Error fetching tax rates.") return None, None # Step 2: Scrape employee benefit costs from a website def get_benefit_costs(): url = "https://example.com/employee-benefits" response = requests.get(url) if response.status_code == 200: soup = BeautifulSoup(response.content, 'html.parser') # Let's assume the page lists the monthly benefit cost benefit_costs = soup.find("div", class_="benefit-total").get_text() return float(benefit_costs.strip("$")) else: print("Error fetching benefit costs.") return 0.0 # Step 3: Calculate total employee cost def calculate_total_employee_cost(salary): federal_tax, state_tax = get_tax_rates() benefits_cost = get_benefit_costs() if federal_tax is not None and state_tax is not None: # Total tax deduction total_tax = salary * (federal_tax + state_tax) / 100 # Total cost = salary + benefits + tax total_cost = salary + benefits_cost + total_tax return total_cost else: return None # Example usage employee_salary = 5000 total_cost = calculate_total_employee_cost(employee_salary) if total_cost: print(f"Total cost for the employee: ${total_cost:.2f}") else: print("Could not calculate employee cost.")
How It Works:
- The get_tax_rates() function retrieves tax rates from an API.
- The get_benefit_costs() function scrapes a webpage for the employee benefits cost.
- The calculate_total_employee_cost() function calculates the total cost by combining salary, taxes, and benefits.
This is a simplified example but demonstrates how you can combine data from different sources (APIs and web scraping) to create more dynamic and useful HR applications.
Best Practices for Web Scraping
While web scraping is powerful, there are some important best practices to follow:
- Respect the website’s robots.txt: Some websites don’t allow scraping, and you should check their robots.txt file before scraping.
- Use appropriate intervals between requests: Avoid overloading the server by adding delays between requests using the time.sleep() function.
- Avoid scraping sensitive or copyrighted data: Always make sure you’re not violating any legal or ethical rules when scraping data.
Conclusion
In this lesson, we explored how to interact with external services using APIs and how to extract data from websites through web scraping. These techniques open up endless possibilities for integrating external data into your Python applications, especially in an HR context.
Atas ialah kandungan terperinci Pelajaran Bekerja dengan API dan Pengikisan Web untuk Automasi HR. Untuk maklumat lanjut, sila ikut artikel berkaitan lain di laman web China PHP!

Alat AI Hot

Undresser.AI Undress
Apl berkuasa AI untuk mencipta foto bogel yang realistik

AI Clothes Remover
Alat AI dalam talian untuk mengeluarkan pakaian daripada foto.

Undress AI Tool
Gambar buka pakaian secara percuma

Clothoff.io
Penyingkiran pakaian AI

Video Face Swap
Tukar muka dalam mana-mana video dengan mudah menggunakan alat tukar muka AI percuma kami!

Artikel Panas

Alat panas

Notepad++7.3.1
Editor kod yang mudah digunakan dan percuma

SublimeText3 versi Cina
Versi Cina, sangat mudah digunakan

Hantar Studio 13.0.1
Persekitaran pembangunan bersepadu PHP yang berkuasa

Dreamweaver CS6
Alat pembangunan web visual

SublimeText3 versi Mac
Perisian penyuntingan kod peringkat Tuhan (SublimeText3)

Topik panas










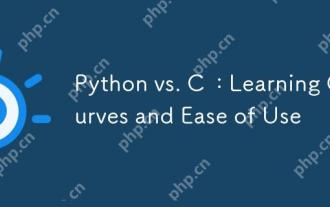
Python lebih mudah dipelajari dan digunakan, manakala C lebih kuat tetapi kompleks. 1. Sintaks Python adalah ringkas dan sesuai untuk pemula. Penaipan dinamik dan pengurusan memori automatik menjadikannya mudah digunakan, tetapi boleh menyebabkan kesilapan runtime. 2.C menyediakan kawalan peringkat rendah dan ciri-ciri canggih, sesuai untuk aplikasi berprestasi tinggi, tetapi mempunyai ambang pembelajaran yang tinggi dan memerlukan memori manual dan pengurusan keselamatan jenis.
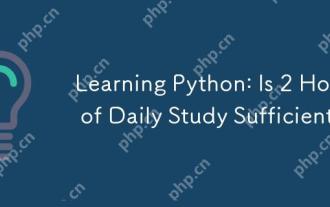
Adakah cukup untuk belajar Python selama dua jam sehari? Ia bergantung pada matlamat dan kaedah pembelajaran anda. 1) Membangunkan pelan pembelajaran yang jelas, 2) Pilih sumber dan kaedah pembelajaran yang sesuai, 3) mengamalkan dan mengkaji semula dan menyatukan amalan tangan dan mengkaji semula dan menyatukan, dan anda secara beransur-ansur boleh menguasai pengetahuan asas dan fungsi lanjutan Python dalam tempoh ini.
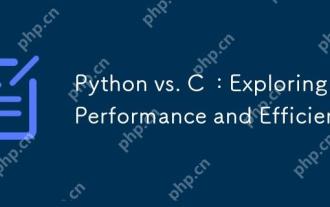
Python lebih baik daripada C dalam kecekapan pembangunan, tetapi C lebih tinggi dalam prestasi pelaksanaan. 1. Sintaks ringkas Python dan perpustakaan yang kaya meningkatkan kecekapan pembangunan. 2. Ciri-ciri jenis kompilasi dan kawalan perkakasan meningkatkan prestasi pelaksanaan. Apabila membuat pilihan, anda perlu menimbang kelajuan pembangunan dan kecekapan pelaksanaan berdasarkan keperluan projek.
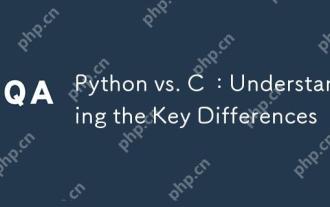
Python dan C masing -masing mempunyai kelebihan sendiri, dan pilihannya harus berdasarkan keperluan projek. 1) Python sesuai untuk pembangunan pesat dan pemprosesan data kerana sintaks ringkas dan menaip dinamik. 2) C sesuai untuk prestasi tinggi dan pengaturcaraan sistem kerana menaip statik dan pengurusan memori manual.
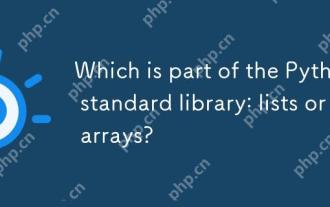
Pythonlistsarepartofthestandardlibrary, sementara
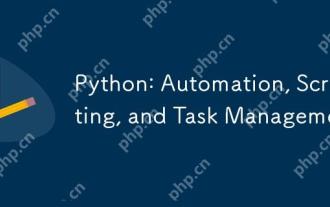
Python cemerlang dalam automasi, skrip, dan pengurusan tugas. 1) Automasi: Sandaran fail direalisasikan melalui perpustakaan standard seperti OS dan Shutil. 2) Penulisan Skrip: Gunakan Perpustakaan Psutil untuk memantau sumber sistem. 3) Pengurusan Tugas: Gunakan perpustakaan jadual untuk menjadualkan tugas. Kemudahan penggunaan Python dan sokongan perpustakaan yang kaya menjadikannya alat pilihan di kawasan ini.
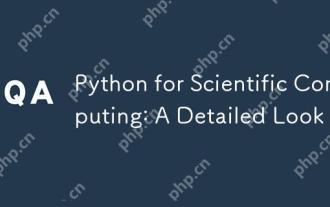
Aplikasi Python dalam pengkomputeran saintifik termasuk analisis data, pembelajaran mesin, simulasi berangka dan visualisasi. 1.Numpy menyediakan susunan pelbagai dimensi yang cekap dan fungsi matematik. 2. Scipy memanjangkan fungsi numpy dan menyediakan pengoptimuman dan alat algebra linear. 3. Pandas digunakan untuk pemprosesan dan analisis data. 4.Matplotlib digunakan untuk menghasilkan pelbagai graf dan hasil visual.
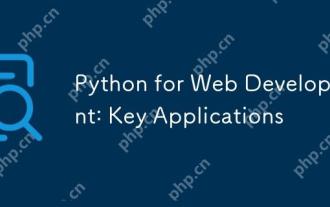
Aplikasi utama Python dalam pembangunan web termasuk penggunaan kerangka Django dan Flask, pembangunan API, analisis data dan visualisasi, pembelajaran mesin dan AI, dan pengoptimuman prestasi. 1. Rangka Kerja Django dan Flask: Django sesuai untuk perkembangan pesat aplikasi kompleks, dan Flask sesuai untuk projek kecil atau sangat disesuaikan. 2. Pembangunan API: Gunakan Flask atau DjangorestFramework untuk membina Restfulapi. 3. Analisis Data dan Visualisasi: Gunakan Python untuk memproses data dan memaparkannya melalui antara muka web. 4. Pembelajaran Mesin dan AI: Python digunakan untuk membina aplikasi web pintar. 5. Pengoptimuman Prestasi: Dioptimumkan melalui pengaturcaraan, caching dan kod tak segerak
