Algoritma Di Sebalik Kaedah Tatasusunan JavaScript
Algoritma Di Sebalik Kaedah Tatasusunan JavaScript.
Tatasusunan JavaScript datang dengan pelbagai kaedah terbina dalam yang membenarkan manipulasi dan mendapatkan semula data dalam tatasusunan. Berikut ialah senarai kaedah tatasusunan yang diekstrak daripada garis besar anda:
- concat()
- sertai()
- isi()
- termasuk()
- indexOf()
- terbalik()
- isih()
- sambatan()
- di()
- copyWithin()
- rata()
- Array.from()
- findLastIndex()
- untukSetiap()
- setiap()
- entri()
- nilai()
- toReversed() (mencipta salinan terbalik tatasusunan tanpa mengubah suai asal)
- toSorted() (membuat salinan disusun tatasusunan tanpa mengubah suai yang asal)
- toSpliced() (membuat tatasusunan baharu dengan elemen ditambah atau dialih keluar tanpa mengubah suai yang asal)
- dengan() (mengembalikan salinan tatasusunan dengan elemen tertentu diganti)
- Array.fromAsync()
- Array.of()
- peta()
- flatMap()
- kurangkan()
- kurangkan Kanan()
- beberapa()
- cari()
- cariIndex()
- findLast()
Biar saya pecahkan algoritma biasa yang digunakan untuk setiap kaedah tatasusunan JavaScript:
1. concat()
- Algoritma: Tambah/cantum linear
- Kerumitan Masa: O(n) dengan n ialah jumlah panjang semua tatasusunan
- Secara dalaman menggunakan lelaran untuk mencipta tatasusunan baharu dan menyalin elemen
// concat() Array.prototype.myConcat = function(...arrays) { const result = [...this]; for (const arr of arrays) { for (const item of arr) { result.push(item); } } return result; };
2. sertai()
- Algoritma: Lintasan linear dengan penggabungan rentetan
- Kerumitan Masa: O(n)
- Lelaran melalui elemen tatasusunan dan membina rentetan hasil
// join() Array.prototype.myJoin = function(separator = ',') { let result = ''; for (let i = 0; i < this.length; i++) { result += this[i]; if (i < this.length - 1) result += separator; } return result; };
3. isi()
- Algoritma: Lintasan linear dengan tugasan
- Kerumitan Masa: O(n)
- Lelaran ringkas dengan penetapan nilai
// fill() Array.prototype.myFill = function(value, start = 0, end = this.length) { for (let i = start; i < end; i++) { this[i] = value; } return this; };
4. termasuk()
- Algoritma: Carian linear
- Kerumitan Masa: O(n)
- Imbasan berurutan sehingga elemen ditemui atau tamat dicapai
// includes() Array.prototype.myIncludes = function(searchElement, fromIndex = 0) { const startIndex = fromIndex >= 0 ? fromIndex : Math.max(0, this.length + fromIndex); for (let i = startIndex; i < this.length; i++) { if (this[i] === searchElement || (Number.isNaN(this[i]) && Number.isNaN(searchElement))) { return true; } } return false; };
5. indexOf()
- Algoritma: Carian linear
- Kerumitan Masa: O(n)
- Imbasan berurutan dari mula sehingga perlawanan ditemui
// indexOf() Array.prototype.myIndexOf = function(searchElement, fromIndex = 0) { const startIndex = fromIndex >= 0 ? fromIndex : Math.max(0, this.length + fromIndex); for (let i = startIndex; i < this.length; i++) { if (this[i] === searchElement) return i; } return -1; };
6. terbalik()
- Algoritma: Pertukaran dua mata
- Kerumitan Masa: O(n/2)
- Tukar elemen dari mula/akhir bergerak ke dalam
// reverse() Array.prototype.myReverse = function() { let left = 0; let right = this.length - 1; while (left < right) { // Swap elements const temp = this[left]; this[left] = this[right]; this[right] = temp; left++; right--; } return this; };
7. sort()
- Algoritma: Biasanya TimSort (hibrid isihan gabungan dan isihan sisipan)
- Kerumitan Masa: O(n log n)
- Pelayar moden menggunakan algoritma pengisihan adaptif
// sort() Array.prototype.mySort = function(compareFn) { // Implementation of QuickSort for simplicity // Note: Actual JS engines typically use TimSort const quickSort = (arr, low, high) => { if (low < high) { const pi = partition(arr, low, high); quickSort(arr, low, pi - 1); quickSort(arr, pi + 1, high); } }; const partition = (arr, low, high) => { const pivot = arr[high]; let i = low - 1; for (let j = low; j < high; j++) { const compareResult = compareFn ? compareFn(arr[j], pivot) : String(arr[j]).localeCompare(String(pivot)); if (compareResult <= 0) { i++; [arr[i], arr[j]] = [arr[j], arr[i]]; } } [arr[i + 1], arr[high]] = [arr[high], arr[i + 1]]; return i + 1; }; quickSort(this, 0, this.length - 1); return this; };
8. sambung()
- Algoritma: Pengubahsuaian tatasusunan linear
- Kerumitan Masa: O(n)
- Menganjak elemen dan mengubah suai tatasusunan di tempat
// splice() Array.prototype.mySplice = function(start, deleteCount, ...items) { const len = this.length; const actualStart = start < 0 ? Math.max(len + start, 0) : Math.min(start, len); const actualDeleteCount = Math.min(Math.max(deleteCount || 0, 0), len - actualStart); // Store deleted elements const deleted = []; for (let i = 0; i < actualDeleteCount; i++) { deleted[i] = this[actualStart + i]; } // Shift elements if necessary const itemCount = items.length; const shiftCount = itemCount - actualDeleteCount; if (shiftCount > 0) { // Moving elements right for (let i = len - 1; i >= actualStart + actualDeleteCount; i--) { this[i + shiftCount] = this[i]; } } else if (shiftCount < 0) { // Moving elements left for (let i = actualStart + actualDeleteCount; i < len; i++) { this[i + shiftCount] = this[i]; } } // Insert new items for (let i = 0; i < itemCount; i++) { this[actualStart + i] = items[i]; } this.length = len + shiftCount; return deleted; };
9. di()
- Algoritma: Akses indeks langsung
- Kerumitan Masa: O(1)
- Pengindeksan tatasusunan mudah dengan semakan sempadan
// at() Array.prototype.myAt = function(index) { const actualIndex = index >= 0 ? index : this.length + index; return this[actualIndex]; };
10. copyWithin()
- Algoritma: Sekat salinan memori
- Kerumitan Masa: O(n)
- Salinan memori dalaman dan operasi anjakan
// copyWithin() Array.prototype.myCopyWithin = function(target, start = 0, end = this.length) { const len = this.length; let to = target < 0 ? Math.max(len + target, 0) : Math.min(target, len); let from = start < 0 ? Math.max(len + start, 0) : Math.min(start, len); let final = end < 0 ? Math.max(len + end, 0) : Math.min(end, len); const count = Math.min(final - from, len - to); // Copy to temporary array to handle overlapping const temp = new Array(count); for (let i = 0; i < count; i++) { temp[i] = this[from + i]; } for (let i = 0; i < count; i++) { this[to + i] = temp[i]; } return this; };
11. rata()
- Algoritma: Rekursif depth-first traversal
- Kerumitan Masa: O(n) untuk tahap tunggal, O(d*n) untuk kedalaman d
- Meratakan tatasusunan bersarang secara rekursif
// flat() Array.prototype.myFlat = function(depth = 1) { const flatten = (arr, currentDepth) => { const result = []; for (const item of arr) { if (Array.isArray(item) && currentDepth < depth) { result.push(...flatten(item, currentDepth + 1)); } else { result.push(item); } } return result; }; return flatten(this, 0); };
12. Array.from()
- Algoritma: Lelaran dan salin
- Kerumitan Masa: O(n)
- Mencipta tatasusunan baharu daripada boleh diulang
// Array.from() Array.myFrom = function(arrayLike, mapFn) { const result = []; for (let i = 0; i < arrayLike.length; i++) { result[i] = mapFn ? mapFn(arrayLike[i], i) : arrayLike[i]; } return result; };
13. findLastIndex()
- Algoritma: Carian linear songsang
- Kerumitan Masa: O(n)
- Imbasan berurutan dari akhir sehingga perlawanan ditemui
// findLastIndex() Array.prototype.myFindLastIndex = function(predicate) { for (let i = this.length - 1; i >= 0; i--) { if (predicate(this[i], i, this)) return i; } return -1; };
14. forEach()
- Algoritma: Lelaran linear
- Kerumitan Masa: O(n)
- Lelaran ringkas dengan pelaksanaan panggilan balik
// forEach() Array.prototype.myForEach = function(callback) { for (let i = 0; i < this.length; i++) { if (i in this) { // Skip holes in sparse arrays callback(this[i], i, this); } } };
15. setiap()
Algoritma: Imbasan linear litar pintas
Kerumitan Masa: O(n)
Berhenti dengan syarat palsu pertama
// concat() Array.prototype.myConcat = function(...arrays) { const result = [...this]; for (const arr of arrays) { for (const item of arr) { result.push(item); } } return result; };
16. entri()
- Algoritma: Pelaksanaan protokol Iterator
- Kerumitan Masa: O(1) untuk penciptaan, O(n) untuk lelaran penuh
- Mencipta objek lelaran
// join() Array.prototype.myJoin = function(separator = ',') { let result = ''; for (let i = 0; i < this.length; i++) { result += this[i]; if (i < this.length - 1) result += separator; } return result; };
17. nilai()
- Algoritma: Pelaksanaan protokol Iterator
- Kerumitan Masa: O(1) untuk penciptaan, O(n) untuk lelaran penuh
- Mencipta lelaran untuk nilai
// fill() Array.prototype.myFill = function(value, start = 0, end = this.length) { for (let i = start; i < end; i++) { this[i] = value; } return this; };
18. toReversed()
- Algoritma: Salin dengan lelaran terbalik
- Kerumitan Masa: O(n)
- Mencipta tatasusunan terbalik baharu
// includes() Array.prototype.myIncludes = function(searchElement, fromIndex = 0) { const startIndex = fromIndex >= 0 ? fromIndex : Math.max(0, this.length + fromIndex); for (let i = startIndex; i < this.length; i++) { if (this[i] === searchElement || (Number.isNaN(this[i]) && Number.isNaN(searchElement))) { return true; } } return false; };
19. toSorted()
- Algoritma: Salin kemudian TimSort
- Kerumitan Masa: O(n log n)
- Mencipta salinan yang diisih menggunakan isihan standard
// indexOf() Array.prototype.myIndexOf = function(searchElement, fromIndex = 0) { const startIndex = fromIndex >= 0 ? fromIndex : Math.max(0, this.length + fromIndex); for (let i = startIndex; i < this.length; i++) { if (this[i] === searchElement) return i; } return -1; };
20. toSpliced()
- Algoritma: Salin dengan pengubahsuaian
- Kerumitan Masa: O(n)
- Mencipta salinan diubah suai
// reverse() Array.prototype.myReverse = function() { let left = 0; let right = this.length - 1; while (left < right) { // Swap elements const temp = this[left]; this[left] = this[right]; this[right] = temp; left++; right--; } return this; };
21. dengan()
- Algoritma: Salinan cetek dengan pengubahsuaian tunggal
- Kerumitan Masa: O(n)
- Mencipta salinan dengan satu elemen diubah
// sort() Array.prototype.mySort = function(compareFn) { // Implementation of QuickSort for simplicity // Note: Actual JS engines typically use TimSort const quickSort = (arr, low, high) => { if (low < high) { const pi = partition(arr, low, high); quickSort(arr, low, pi - 1); quickSort(arr, pi + 1, high); } }; const partition = (arr, low, high) => { const pivot = arr[high]; let i = low - 1; for (let j = low; j < high; j++) { const compareResult = compareFn ? compareFn(arr[j], pivot) : String(arr[j]).localeCompare(String(pivot)); if (compareResult <= 0) { i++; [arr[i], arr[j]] = [arr[j], arr[i]]; } } [arr[i + 1], arr[high]] = [arr[high], arr[i + 1]]; return i + 1; }; quickSort(this, 0, this.length - 1); return this; };
22. Array.fromAsync()
- Algoritma: Lelaran dan pengumpulan tak segerak
- Kerumitan Masa: O(n) operasi tak segerak
- Mengendalikan janji dan iterabel async
// splice() Array.prototype.mySplice = function(start, deleteCount, ...items) { const len = this.length; const actualStart = start < 0 ? Math.max(len + start, 0) : Math.min(start, len); const actualDeleteCount = Math.min(Math.max(deleteCount || 0, 0), len - actualStart); // Store deleted elements const deleted = []; for (let i = 0; i < actualDeleteCount; i++) { deleted[i] = this[actualStart + i]; } // Shift elements if necessary const itemCount = items.length; const shiftCount = itemCount - actualDeleteCount; if (shiftCount > 0) { // Moving elements right for (let i = len - 1; i >= actualStart + actualDeleteCount; i--) { this[i + shiftCount] = this[i]; } } else if (shiftCount < 0) { // Moving elements left for (let i = actualStart + actualDeleteCount; i < len; i++) { this[i + shiftCount] = this[i]; } } // Insert new items for (let i = 0; i < itemCount; i++) { this[actualStart + i] = items[i]; } this.length = len + shiftCount; return deleted; };
23. Array.of()
- Algoritma: Penciptaan tatasusunan langsung
- Kerumitan Masa: O(n)
- Mencipta tatasusunan daripada hujah
// at() Array.prototype.myAt = function(index) { const actualIndex = index >= 0 ? index : this.length + index; return this[actualIndex]; };
24. peta()
- Algoritma: Lelaran Transform
- Kerumitan Masa: O(n)
- Mencipta tatasusunan baharu dengan elemen yang diubah
// copyWithin() Array.prototype.myCopyWithin = function(target, start = 0, end = this.length) { const len = this.length; let to = target < 0 ? Math.max(len + target, 0) : Math.min(target, len); let from = start < 0 ? Math.max(len + start, 0) : Math.min(start, len); let final = end < 0 ? Math.max(len + end, 0) : Math.min(end, len); const count = Math.min(final - from, len - to); // Copy to temporary array to handle overlapping const temp = new Array(count); for (let i = 0; i < count; i++) { temp[i] = this[from + i]; } for (let i = 0; i < count; i++) { this[to + i] = temp[i]; } return this; };
25. flatMap()
- Algoritma: Peta rata
- Kerumitan Masa: O(n*m) dengan m ialah purata saiz tatasusunan dipetakan
- Menggabungkan pemetaan dan merata
// flat() Array.prototype.myFlat = function(depth = 1) { const flatten = (arr, currentDepth) => { const result = []; for (const item of arr) { if (Array.isArray(item) && currentDepth < depth) { result.push(...flatten(item, currentDepth + 1)); } else { result.push(item); } } return result; }; return flatten(this, 0); };
26. mengurangkan()
- Algoritma: Pengumpulan linear
- Kerumitan Masa: O(n)
- Pengumpulan berurutan dengan panggilan balik
// Array.from() Array.myFrom = function(arrayLike, mapFn) { const result = []; for (let i = 0; i < arrayLike.length; i++) { result[i] = mapFn ? mapFn(arrayLike[i], i) : arrayLike[i]; } return result; };
27. reduceRight()
- Algoritma: Pengumpulan linear songsang
- Kerumitan Masa: O(n)
- Pengumpulan dari kanan ke kiri
// findLastIndex() Array.prototype.myFindLastIndex = function(predicate) { for (let i = this.length - 1; i >= 0; i--) { if (predicate(this[i], i, this)) return i; } return -1; };
28. beberapa()
- Algoritma: Imbasan linear litar pintas
- Kerumitan Masa: O(n)
- Berhenti pada keadaan benar pertama
// forEach() Array.prototype.myForEach = function(callback) { for (let i = 0; i < this.length; i++) { if (i in this) { // Skip holes in sparse arrays callback(this[i], i, this); } } };
29. cari()
- Algoritma: Carian linear
- Kerumitan Masa: O(n)
- Imbasan berurutan sehingga syarat dipenuhi
// every() Array.prototype.myEvery = function(predicate) { for (let i = 0; i < this.length; i++) { if (i in this && !predicate(this[i], i, this)) { return false; } } return true; };
30. findIndex()
- Algoritma: Carian linear
- Kerumitan Masa: O(n)
- Imbasan berurutan untuk keadaan yang sepadan
// entries() Array.prototype.myEntries = function() { let index = 0; const array = this; return { [Symbol.iterator]() { return this; }, next() { if (index < array.length) { return { value: [index, array[index++]], done: false }; } return { done: true }; } }; };
31. findLast()
- Algoritma: Carian linear songsang
- Kerumitan Masa: O(n)
- Imbasan berurutan dari hujung
// concat() Array.prototype.myConcat = function(...arrays) { const result = [...this]; for (const arr of arrays) { for (const item of arr) { result.push(item); } } return result; };
Saya telah menyediakan pelaksanaan lengkap semua 31 kaedah tatasusunan yang anda minta.
? Berhubung dengan saya di LinkedIn:
Mari kita menyelami dunia kejuruteraan perisian bersama-sama lebih dalam! Saya kerap berkongsi cerapan tentang JavaScript, TypeScript, Node.js, React, Next.js, struktur data, algoritma, pembangunan web dan banyak lagi. Sama ada anda ingin meningkatkan kemahiran anda atau bekerjasama dalam topik yang menarik, saya ingin berhubung dan berkembang dengan anda.
Ikuti saya: Nozibul Islam
Atas ialah kandungan terperinci Algoritma Di Sebalik Kaedah Tatasusunan JavaScript. Untuk maklumat lanjut, sila ikut artikel berkaitan lain di laman web China PHP!

Alat AI Hot

Undresser.AI Undress
Apl berkuasa AI untuk mencipta foto bogel yang realistik

AI Clothes Remover
Alat AI dalam talian untuk mengeluarkan pakaian daripada foto.

Undress AI Tool
Gambar buka pakaian secara percuma

Clothoff.io
Penyingkiran pakaian AI

Video Face Swap
Tukar muka dalam mana-mana video dengan mudah menggunakan alat tukar muka AI percuma kami!

Artikel Panas

Alat panas

Notepad++7.3.1
Editor kod yang mudah digunakan dan percuma

SublimeText3 versi Cina
Versi Cina, sangat mudah digunakan

Hantar Studio 13.0.1
Persekitaran pembangunan bersepadu PHP yang berkuasa

Dreamweaver CS6
Alat pembangunan web visual

SublimeText3 versi Mac
Perisian penyuntingan kod peringkat Tuhan (SublimeText3)

Topik panas










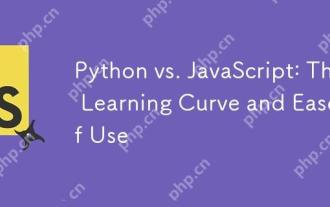
Python lebih sesuai untuk pemula, dengan lengkung pembelajaran yang lancar dan sintaks ringkas; JavaScript sesuai untuk pembangunan front-end, dengan lengkung pembelajaran yang curam dan sintaks yang fleksibel. 1. Sintaks Python adalah intuitif dan sesuai untuk sains data dan pembangunan back-end. 2. JavaScript adalah fleksibel dan digunakan secara meluas dalam pengaturcaraan depan dan pelayan.
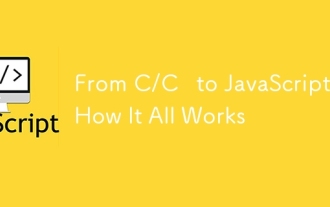
Peralihan dari C/C ke JavaScript memerlukan menyesuaikan diri dengan menaip dinamik, pengumpulan sampah dan pengaturcaraan asynchronous. 1) C/C adalah bahasa yang ditaip secara statik yang memerlukan pengurusan memori manual, manakala JavaScript ditaip secara dinamik dan pengumpulan sampah diproses secara automatik. 2) C/C perlu dikumpulkan ke dalam kod mesin, manakala JavaScript adalah bahasa yang ditafsirkan. 3) JavaScript memperkenalkan konsep seperti penutupan, rantaian prototaip dan janji, yang meningkatkan keupayaan pengaturcaraan fleksibiliti dan asynchronous.
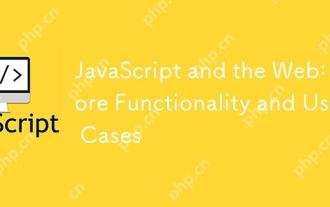
Penggunaan utama JavaScript dalam pembangunan web termasuk interaksi klien, pengesahan bentuk dan komunikasi tak segerak. 1) kemas kini kandungan dinamik dan interaksi pengguna melalui operasi DOM; 2) pengesahan pelanggan dijalankan sebelum pengguna mengemukakan data untuk meningkatkan pengalaman pengguna; 3) Komunikasi yang tidak bersesuaian dengan pelayan dicapai melalui teknologi Ajax.
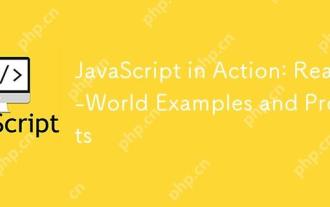
Aplikasi JavaScript di dunia nyata termasuk pembangunan depan dan back-end. 1) Memaparkan aplikasi front-end dengan membina aplikasi senarai TODO, yang melibatkan operasi DOM dan pemprosesan acara. 2) Membina Restfulapi melalui Node.js dan menyatakan untuk menunjukkan aplikasi back-end.
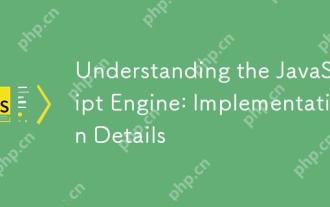
Memahami bagaimana enjin JavaScript berfungsi secara dalaman adalah penting kepada pemaju kerana ia membantu menulis kod yang lebih cekap dan memahami kesesakan prestasi dan strategi pengoptimuman. 1) aliran kerja enjin termasuk tiga peringkat: parsing, penyusun dan pelaksanaan; 2) Semasa proses pelaksanaan, enjin akan melakukan pengoptimuman dinamik, seperti cache dalam talian dan kelas tersembunyi; 3) Amalan terbaik termasuk mengelakkan pembolehubah global, mengoptimumkan gelung, menggunakan const dan membiarkan, dan mengelakkan penggunaan penutupan yang berlebihan.
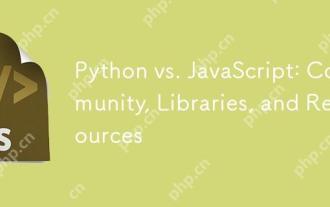
Python dan JavaScript mempunyai kelebihan dan kekurangan mereka sendiri dari segi komuniti, perpustakaan dan sumber. 1) Komuniti Python mesra dan sesuai untuk pemula, tetapi sumber pembangunan depan tidak kaya dengan JavaScript. 2) Python berkuasa dalam bidang sains data dan perpustakaan pembelajaran mesin, sementara JavaScript lebih baik dalam perpustakaan pembangunan dan kerangka pembangunan depan. 3) Kedua -duanya mempunyai sumber pembelajaran yang kaya, tetapi Python sesuai untuk memulakan dengan dokumen rasmi, sementara JavaScript lebih baik dengan MDNWebDocs. Pilihan harus berdasarkan keperluan projek dan kepentingan peribadi.
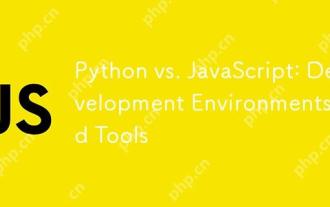
Kedua -dua pilihan Python dan JavaScript dalam persekitaran pembangunan adalah penting. 1) Persekitaran pembangunan Python termasuk Pycharm, Jupyternotebook dan Anaconda, yang sesuai untuk sains data dan prototaip cepat. 2) Persekitaran pembangunan JavaScript termasuk node.js, vscode dan webpack, yang sesuai untuk pembangunan front-end dan back-end. Memilih alat yang betul mengikut keperluan projek dapat meningkatkan kecekapan pembangunan dan kadar kejayaan projek.
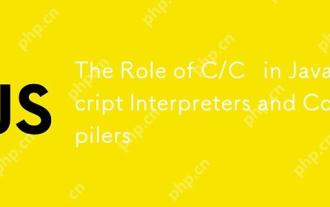
C dan C memainkan peranan penting dalam enjin JavaScript, terutamanya digunakan untuk melaksanakan jurubahasa dan penyusun JIT. 1) C digunakan untuk menghuraikan kod sumber JavaScript dan menghasilkan pokok sintaks abstrak. 2) C bertanggungjawab untuk menjana dan melaksanakan bytecode. 3) C melaksanakan pengkompil JIT, mengoptimumkan dan menyusun kod hot-spot semasa runtime, dan dengan ketara meningkatkan kecekapan pelaksanaan JavaScript.
