如何用html5 canvas实现匀速运动
匀速运动:指的是物体在一条直线上运动,并且物体在任何相等时间间隔内通过的位移都是相等的。其实就是匀速直线运动,它的特点是加速度为0,从定义可知,在任何相等的时间间隔内,速度大小和方向是相同的。
<head> <meta charset='utf-8' /> <style> #canvas { border: 1px dashed #aaa; } </style> <script> window.onload = function () { var oCanvas = document.querySelector("#canvas"), oGc = oCanvas.getContext('2d'), width = oCanvas.width, height = oCanvas.height, x = 0; function drawBall( x, y, cxt ){ cxt.fillStyle = '#09f'; cxt.beginPath(); cxt.arc( x, y, 20, 0, 2 * Math.PI ); cxt.closePath(); cxt.fill(); } ( function linear(){ oGc.clearRect( 0, 0, width, height ); drawBall( x, height / 2, oGc ); x += 2; console.log( x ); requestAnimationFrame( linear ); } )(); } </script> </head> <body> <canvas id="canvas" width="1200" height="600"></canvas> </body>
上述实例让一个半径20px的小球 从x=0, y=canvas高度的一半,以每帧2px的速度向右匀速运动.
我们可以把小球封装成一个对象:
ball.js文件:
function Ball( x, y, r, color ){ this.x = x || 0; this.y = y || 0; this.radius = r || 20; this.color = color || '#09f'; } Ball.prototype = { constructor : Ball, stroke : function( cxt ){ cxt.strokeStyle = this.color; cxt.beginPath(); cxt.arc( this.x, this.y, this.radius, 0, 2 * Math.PI ); cxt.closePath(); cxt.stroke(); }, fill : function( cxt ){ cxt.fillStyle = this.color; cxt.beginPath(); cxt.arc( this.x, this.y, this.radius, 0, 2 * Math.PI ); cxt.closePath(); cxt.fill(); } }
该小球对象,可以定制位置半径和颜色,支持两种渲染方式(描边和填充)
<head> <meta charset='utf-8' /> <style> #canvas { border: 1px dashed #aaa; } </style> <script src="./ball.js"></script> <script> window.onload = function () { var oCanvas = document.querySelector("#canvas"), oGc = oCanvas.getContext('2d'), width = oCanvas.width, height = oCanvas.height, ball = new Ball( 0, height / 2 ); (function linear() { oGc.clearRect(0, 0, width, height); ball.fill( oGc ); ball.x += 2; requestAnimationFrame(linear); })(); } </script> </head> <body> <canvas id="canvas" width="1200" height="600"></canvas> </body>
斜线匀速运动:
<head> <meta charset='utf-8' /> <style> #canvas { border: 1px dashed #aaa; } </style> <script src="./ball.js"></script> <script> window.onload = function () { var oCanvas = document.querySelector("#canvas"), oGc = oCanvas.getContext('2d'), width = oCanvas.width, height = oCanvas.height, ball = new Ball( 0, height ); (function linear() { oGc.clearRect(0, 0, width, height); ball.fill( oGc ); ball.x += 2; ball.y -= 1; requestAnimationFrame(linear); })(); } </script> </head> <body> <canvas id="canvas" width="1200" height="600"></canvas> </body>
任意方向的匀速运动(速度分解)
<head> <meta charset='utf-8' /> <style> #canvas { border: 1px dashed #aaa; } </style> <script src="./ball.js"></script> <script> window.onload = function () { var oCanvas = document.querySelector("#canvas"), oGc = oCanvas.getContext('2d'), width = oCanvas.width, height = oCanvas.height, ball = new Ball( 0, 0 ), speed = 5, vx = speed * Math.cos( 10 * Math.PI / 180 ), vy = speed * Math.sin( 10 * Math.PI / 180 ); (function linear() { oGc.clearRect(0, 0, width, height); ball.fill( oGc ); ball.x += vx; ball.y += vy; requestAnimationFrame(linear); })(); } </script> </head> <body> <canvas id="canvas" width="1200" height="600"></canvas> </body>
Atas ialah kandungan terperinci 如何用html5 canvas实现匀速运动. Untuk maklumat lanjut, sila ikut artikel berkaitan lain di laman web China PHP!

Alat AI Hot

Undresser.AI Undress
Apl berkuasa AI untuk mencipta foto bogel yang realistik

AI Clothes Remover
Alat AI dalam talian untuk mengeluarkan pakaian daripada foto.

Undress AI Tool
Gambar buka pakaian secara percuma

Clothoff.io
Penyingkiran pakaian AI

AI Hentai Generator
Menjana ai hentai secara percuma.

Artikel Panas

Alat panas

Notepad++7.3.1
Editor kod yang mudah digunakan dan percuma

SublimeText3 versi Cina
Versi Cina, sangat mudah digunakan

Hantar Studio 13.0.1
Persekitaran pembangunan bersepadu PHP yang berkuasa

Dreamweaver CS6
Alat pembangunan web visual

SublimeText3 versi Mac
Perisian penyuntingan kod peringkat Tuhan (SublimeText3)

Topik panas


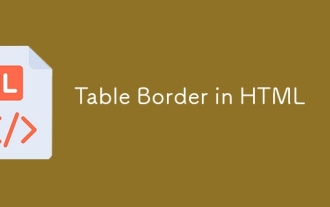
Panduan untuk Sempadan Jadual dalam HTML. Di sini kita membincangkan pelbagai cara untuk menentukan sempadan jadual dengan contoh Sempadan Jadual dalam HTML.
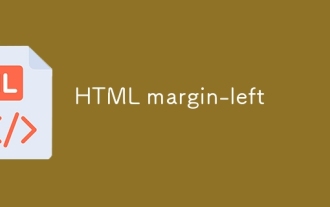
Panduan untuk HTML margin-kiri. Di sini kita membincangkan gambaran keseluruhan ringkas tentang HTML margin-left dan Contoh-contohnya bersama-sama dengan Pelaksanaan Kodnya.
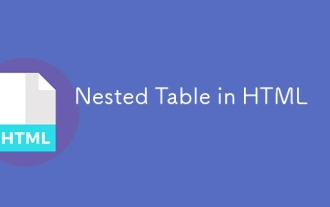
Ini ialah panduan untuk Nested Table dalam HTML. Di sini kita membincangkan cara membuat jadual dalam jadual bersama-sama dengan contoh masing-masing.
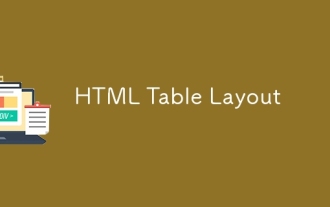
Panduan untuk Susun Atur Jadual HTML. Di sini kita membincangkan Nilai Susun Atur Jadual HTML bersama-sama dengan contoh dan output n perincian.
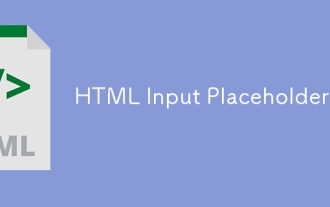
Panduan untuk Pemegang Tempat Input HTML. Di sini kita membincangkan Contoh Pemegang Tempat Input HTML bersama-sama dengan kod dan output.
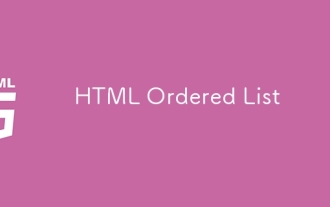
Panduan kepada Senarai Tertib HTML. Di sini kami juga membincangkan pengenalan senarai dan jenis Tertib HTML bersama-sama dengan contoh mereka masing-masing
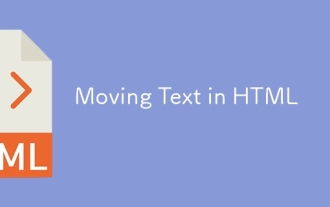
Panduan untuk Memindahkan Teks dalam HTML. Di sini kita membincangkan pengenalan, cara teg marquee berfungsi dengan sintaks dan contoh untuk dilaksanakan.
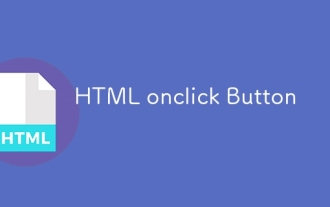
Panduan untuk Butang onclick HTML. Di sini kita membincangkan pengenalan, kerja, contoh dan onclick Event masing-masing dalam pelbagai acara.
