实例分析一个实用的php验证码类
这篇文章主要为大家详细介绍了一个实用的php验证码类,具有一定的参考价值,感兴趣的小伙伴们可以参考一下
万能php验证码类,供大家参考,具体内容如下
code.php是验证码类,类的名称最好和文件名的名称一样,这样有利于我们的查看。
code.php
<?php header('Content-type:text/html;charset=utf8'); class Code{ // 验证码个数$number protected $number; // 验证码类型$codeType protected $codeType; // 验证码图像宽度$width protected $width; // 验证码$height protected $height; // 验证码字符串$code protected $code; // 图像资源$image protected $image; public function __construct($number=4,$codeType=0,$height=50,$width=100){ //初始化自己的成员属性 $this->number=$number; $this->codeType=$codeType; $this->width = $width; $this->height= $height; //生成验证码函数 $this->code = $this ->createCode(); } public function __get($name){ if ($name == 'code'){ return $this->code; } return false; } /*获取验证码*/ public function getCode() { return $this->code; } /*图像资源销毁*/ public function __destruct(){ imagedestroy($this->image); } protected function createCode(){ //通过你的验证码类型生成验证码 switch ($this->codeType){ case 0: //纯数字 $code = $this->getNumberCode(); break; case 1: //纯字母的 $code = $this->getCharCode(); break; case 2: //数字和字母混合 $code = $this->getNumCharCode(); break; default: die('不支持此类验证码类型'); } return $code; } protected function getNumberCode(){ $str = join('', range(0, 9)); return substr(str_shuffle($str),0, $this->number); } protected function getCharCode(){ $str = join('', range('a', 'z')); $str = $str.strtoupper($str); return substr(str_shuffle($str),0,$this->number); } protected function getNumCharCode(){ $numstr = join('',range(0, 9)); $str =join('', range('a', 'z')); $str =$numstr.$str.strtoupper($str); return substr(str_shuffle($str), 0,$this->number); } protected function createImage(){ $this->image = imagecreatetruecolor($this->width, $this->height); } protected function fillBack(){ imagefill($this->image, 0, 0, $this->lightColor()); } /*浅色*/ protected function lightColor(){ return imagecolorallocate($this->image, mt_rand(133,255), mt_rand(133,255), mt_rand(133,255)); } /*深色*/ protected function darkColor(){ return imagecolorallocate($this->image, mt_rand(0,120), mt_rand(0,120), mt_rand(0,120)); } protected function drawChar(){ $width = ceil($this->width / $this->number); for ($i=0; $i< $this->number;$i++){ $x = mt_rand($i*$width+5, ($i+1)*$width-10); $y = mt_rand(0, $this->height -15); imagechar($this->image, 5, $x, $y, $this->code[$i], $this->darkColor()); } } protected function drawLine(){ for ($i=0;$i<5;$i++) { imageline($this->image,mt_rand(0,$this->width),mt_rand(0,$this->height),mt_rand(0,$this->width),mt_rand(0,$this->height),$this->darkColor()); } } protected function drawDisturb(){ for ($i=0;$i<150;$i++){ $x=mt_rand(0, $this->width); $y=mt_rand(0, $this->height); imagesetpixel($this->image, $x, $y, $this->lightColor()); } } protected function show(){ header('Content-Type:image/png'); imagepng($this->image); } public function outImage(){ // 创建画布 $this->createImage(); // 填充背景色 $this->fillBack(); // 将验证码字符串花到画布上 $this->drawChar(); // 添加干扰元素 $this->drawDisturb(); // 添加线条 $this->drawLine(); // 输出并显示 $this->show(); } }
test.php是new一个新的验证码,并把它保存到session中,为我们验证码的验证起到保存和存储的作用。
test.php
<?php //开启session session_start(); require_once 'code.php'; $code= new Code(4,1,50,100); $_SESSION['code']= $code->getCode(); $code->outImage();
login.php就是最后的验证。
login.php
<?php //开启Session session_start(); //判断是否提交 if(isset($_POST['dosubmit'])){ //获取session中的验证码并转为小写 $sessionCode=strtolower($_SESSION['code']); //获取输入的验证码 $code=strtolower($_POST['code']); //判断是否相等 if($sessionCode==$code){ echo "<script type='text/javascript'>alert('验证码正确!');</script>"; }else{ echo "<script type='text/javascript'>alert('验证码错误!');</script>"; } } ?> <!DOCTYPE html> <html> <head> <title></title> <meta http-equiv="Content-Type" content="text/html;charset=UTF-8"/> <style type="text/css"> *{margin:0px;padding:0px;} ul{ width:400px; list-style:none; margin:50px auto; } li{ padding:12px; position:relative; } label{ width:80px; display:inline-block; float:left; line-height:30px; } input[type='text'],input[type='password']{ height:30px; } img{ margin-left:10px; } input[type="submit"]{ margin-left:80px; padding:5px 10px; } </style> </head> <body> <form action="login.php" method="post"> <ul> <li> <label>用户名:</label> <input type="text" name="username"/> </li> <li> <label>密码:</label> <input type="password" name="password"/> </li> <li> <label>验证码:</label> <input type="text" name="code" size="4" style="float:left"/> <img src="test.php" onclick="this.src='test.php?Math.random()'"/> </li> <li> <input type="submit" value="登录" name="dosubmit"/> </li> </ul> </form> </body> </html>
以上就是本文的全部内容,希望对大家的学习有所帮助。
相关推荐:
Atas ialah kandungan terperinci 实例分析一个实用的php验证码类. Untuk maklumat lanjut, sila ikut artikel berkaitan lain di laman web China PHP!

Alat AI Hot

Undresser.AI Undress
Apl berkuasa AI untuk mencipta foto bogel yang realistik

AI Clothes Remover
Alat AI dalam talian untuk mengeluarkan pakaian daripada foto.

Undress AI Tool
Gambar buka pakaian secara percuma

Clothoff.io
Penyingkiran pakaian AI

Video Face Swap
Tukar muka dalam mana-mana video dengan mudah menggunakan alat tukar muka AI percuma kami!

Artikel Panas

Alat panas

Notepad++7.3.1
Editor kod yang mudah digunakan dan percuma

SublimeText3 versi Cina
Versi Cina, sangat mudah digunakan

Hantar Studio 13.0.1
Persekitaran pembangunan bersepadu PHP yang berkuasa

Dreamweaver CS6
Alat pembangunan web visual

SublimeText3 versi Mac
Perisian penyuntingan kod peringkat Tuhan (SublimeText3)

Topik panas
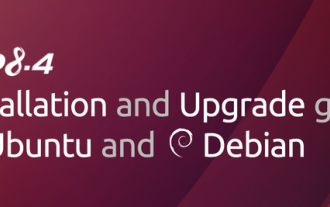
PHP 8.4 membawa beberapa ciri baharu, peningkatan keselamatan dan peningkatan prestasi dengan jumlah penamatan dan penyingkiran ciri yang sihat. Panduan ini menerangkan cara memasang PHP 8.4 atau naik taraf kepada PHP 8.4 pada Ubuntu, Debian, atau terbitan mereka
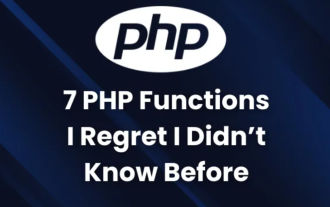
Jika anda seorang pembangun PHP yang berpengalaman, anda mungkin merasakan bahawa anda telah berada di sana dan telah melakukannya. Anda telah membangunkan sejumlah besar aplikasi, menyahpenyahpepijat berjuta-juta baris kod dan mengubah suai sekumpulan skrip untuk mencapai op
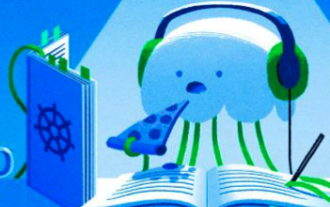
Kod Visual Studio, juga dikenali sebagai Kod VS, ialah editor kod sumber percuma — atau persekitaran pembangunan bersepadu (IDE) — tersedia untuk semua sistem pengendalian utama. Dengan koleksi sambungan yang besar untuk banyak bahasa pengaturcaraan, Kod VS boleh menjadi c
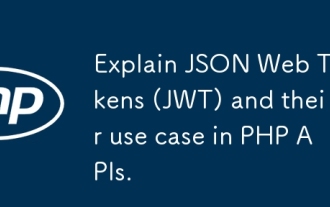
JWT adalah standard terbuka berdasarkan JSON, yang digunakan untuk menghantar maklumat secara selamat antara pihak, terutamanya untuk pengesahan identiti dan pertukaran maklumat. 1. JWT terdiri daripada tiga bahagian: header, muatan dan tandatangan. 2. Prinsip kerja JWT termasuk tiga langkah: menjana JWT, mengesahkan JWT dan muatan parsing. 3. Apabila menggunakan JWT untuk pengesahan di PHP, JWT boleh dijana dan disahkan, dan peranan pengguna dan maklumat kebenaran boleh dimasukkan dalam penggunaan lanjutan. 4. Kesilapan umum termasuk kegagalan pengesahan tandatangan, tamat tempoh, dan muatan besar. Kemahiran penyahpepijatan termasuk menggunakan alat debugging dan pembalakan. 5. Pengoptimuman prestasi dan amalan terbaik termasuk menggunakan algoritma tandatangan yang sesuai, menetapkan tempoh kesahihan dengan munasabah,
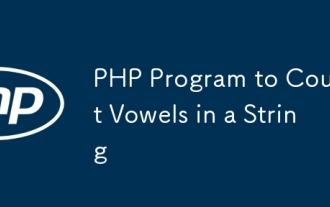
Rentetan adalah urutan aksara, termasuk huruf, nombor, dan simbol. Tutorial ini akan mempelajari cara mengira bilangan vokal dalam rentetan yang diberikan dalam PHP menggunakan kaedah yang berbeza. Vokal dalam bahasa Inggeris adalah a, e, i, o, u, dan mereka boleh menjadi huruf besar atau huruf kecil. Apa itu vokal? Vokal adalah watak abjad yang mewakili sebutan tertentu. Terdapat lima vokal dalam bahasa Inggeris, termasuk huruf besar dan huruf kecil: a, e, i, o, u Contoh 1 Input: String = "TutorialSpoint" Output: 6 menjelaskan Vokal dalam rentetan "TutorialSpoint" adalah u, o, i, a, o, i. Terdapat 6 yuan sebanyak 6
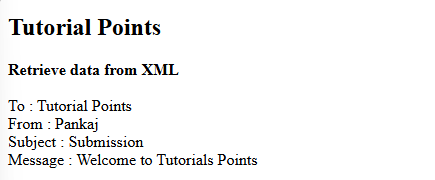
Tutorial ini menunjukkan cara memproses dokumen XML dengan cekap menggunakan PHP. XML (bahasa markup extensible) adalah bahasa markup berasaskan teks yang serba boleh yang direka untuk pembacaan manusia dan parsing mesin. Ia biasanya digunakan untuk penyimpanan data
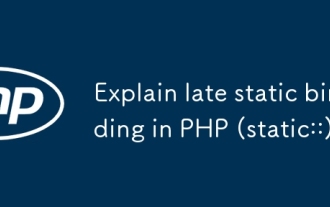
Mengikat statik (statik: :) Melaksanakan pengikatan statik lewat (LSB) dalam PHP, yang membolehkan kelas panggilan dirujuk dalam konteks statik dan bukannya menentukan kelas. 1) Proses parsing dilakukan pada masa runtime, 2) Cari kelas panggilan dalam hubungan warisan, 3) ia boleh membawa overhead prestasi.

Apakah kaedah sihir PHP? Kaedah sihir PHP termasuk: 1. \ _ \ _ Membina, digunakan untuk memulakan objek; 2. \ _ \ _ Destruct, digunakan untuk membersihkan sumber; 3. \ _ \ _ Call, mengendalikan panggilan kaedah yang tidak wujud; 4. \ _ \ _ Mendapatkan, melaksanakan akses atribut dinamik; 5. \ _ \ _ Set, melaksanakan tetapan atribut dinamik. Kaedah ini secara automatik dipanggil dalam situasi tertentu, meningkatkan fleksibiliti dan kecekapan kod.
