PHP实现简单的GET、POST、Cookie、Session等功能
本篇文章主要介绍PHP实现简单的GET、POST、Cookie、Session等功能,感兴趣的朋友参考下,希望对大家有所帮助。
本文实例讲述了PHP封装的HttpClient类,具体分析如下:
这是一段php封装的HttpClient类,可实现GET POST Cookie Session等简单的功能。原来做过,这两天重新修改了一下。
<?php /* * Filename: httpclient.php * Created on 2012-12-21 * Created by RobinTang * To change the template for this generated file go to * Window - Preferences - PHPeclipse - PHP - Code Templates */ class SinCookie { public $name; // Cookie名称 public $value; // Cookie值 // 下面三个属性现在未实现 public $expires; // 过期时间 public $path; // 路径 public $domain; // 域 // 从Cookie字符串创建一个Cookie对象 function __construct($s = false) { if ($s) { $i1 = strpos($s, '='); $i2 = strpos($s, ';'); $this->name = trim(substr($s, 0, $i1)); $this->value = trim(substr($s, $i1 +1, $i2 - $i1 -1)); } } // 获取Cookie键值对 function getKeyValue() { return "$this->name=$this->value"; } } // 会话上下文 class SinHttpContext { public $cookies; // 会话Cookies public $referer; // 前一个页面地址 function __construct() { $this->cookies = array (); $this->refrer = ""; } // 设置Cookie function cookie($key, $val) { $ck = new SinCookie(); $ck->name = $key; $ck->value = $val; $this->addCookie($ck); } // 添加Cookie function addCookie($ck) { $this->cookies[$ck->name] = $ck; } // 获取Cookies字串,请求时用到 function cookiesString() { $res = ''; foreach ($this->cookies as $ck) { $res .= $ck->getKeyValue() . ';'; } return $res; } } // Http请求对象 class SinHttpRequest { public $url; // 请求地址 public $method = 'GET'; // 请求方法 public $host; // 主机 public $path; // 路径 public $scheme; // 协议,http public $port; // 端口 public $header; // 请求头 public $body; // 请求正文 // 设置头 function setHeader($k, $v) { if (!isset ($this->header)) { $this->header = array (); } $this->header[$k] = $v; } // 获取请求字符串 // 包含头和请求正文 // 获取之后直接写socket就行 function reqString() { $matches = parse_url($this->url); !isset ($matches['host']) && $matches['host'] = ''; !isset ($matches['path']) && $matches['path'] = ''; !isset ($matches['query']) && $matches['query'] = ''; !isset ($matches['port']) && $matches['port'] = ''; $host = $matches['host']; $path = $matches['path'] ? $matches['path'] . ($matches['query'] ? '?' . $matches['query'] : '') : '/'; $port = !empty ($matches['port']) ? $matches['port'] : 80; $scheme = $matches['scheme'] ? $matches['scheme'] : 'http'; $this->host = $host; $this->path = $path; $this->scheme = $scheme; $this->port = $port; $method = strtoupper($this->method); $res = "$method $path HTTP/1.1\r\n"; $res .= "Host: $host\r\n"; if ($this->header) { reset($this->header); while (list ($k, $v) = each($this->header)) { if (isset ($v) && strlen($v) > 0) $res .= "$k: $v\r\n"; } } $res .= "\r\n"; if ($this->body) { $res .= $this->body; $res .= "\r\n\r\n"; } return $res; } } // Http响应 class SinHttpResponse { public $scheme; // 协议 public $stasus; // 状态,成功的时候是ok public $code; // 状态码,成功的时候是200 public $header; // 响应头 public $body; // 响应正文 function __construct() { $this->header = array (); $this->body = null; } function setHeader($key, $val) { $this->header[$key] = $val; } } // HttpClient class SinHttpClient { public $keepcontext = true; // 是否维持会话 public $context; // 上下文 public $request; // 请求 public $response; // 响应 public $debug = false; // 是否在Debug模式, //为true的时候会打印出请求内容和相同的头部 function __construct() { $this->request = new SinHttpRequest(); $this->response = new SinHttpResponse(); $this->context = new SinHttpContext(); $this->timeout = 15; // 默认的超时为15s } // 清除上一次的请求内容 function clearRequest() { $this->request->body = ''; $this->request->setHeader('Content-Length', false); $this->request->setHeader('Content-Type', false); } // post方法 // data为请求的数据 // 为键值对的时候模拟表单提交 // 其他时候为数据提交,提交的形式为xml // 如有其他需求,请自行扩展 function post($url, $data = false) { $this->clearRequest(); if ($data) { if (is_array($data)) { $con = http_build_query($data); $this->request->setHeader('Content-Type', 'application/x-www-form-urlencoded'); } else { $con = $data; $this->request->setHeader('Content-Type', 'text/xml; charset=utf-8'); } $this->request->body = $con; $this->request->method = "POST"; $this->request->setHeader('Content-Length', strlen($con)); } $this->startRequest($url); } // get方法 function get($url) { $this->clearRequest(); $this->request->method = "GET"; $this->startRequest($url); } // 该方法为内部调用方法,不用直接调用 function startRequest($url) { $this->request->url = $url; if ($this->keepcontext) { // 如果保存上下文的话设置相关信息 $this->request->setHeader('Referer', $this->context->refrer); $cks = $this->context->cookiesString(); if (strlen($cks) > 0) $this->request->setHeader('Cookie', $cks); } // 获取请求内容 $reqstring = $this->request->reqString(); if ($this->debug) echo "Request:\n$reqstring\n"; try { $fp = fsockopen($this->request->host, $this->request->port, $errno, $errstr, $this->timeout); } catch (Exception $ex) { echo $ex->getMessage(); exit (0); } if ($fp) { stream_set_blocking($fp, true); stream_set_timeout($fp, $this->timeout); // 写数据 fwrite($fp, $reqstring); $status = stream_get_meta_data($fp); if (!$status['timed_out']) { //未超时 // 下面的循环用来读取响应头部 while (!feof($fp)) { $h = fgets($fp); if ($this->debug) echo $h; if ($h && ($h == "\r\n" || $h == "\n")) break; $pos = strpos($h, ':'); if ($pos) { $k = strtolower(trim(substr($h, 0, $pos))); $v = trim(substr($h, $pos +1)); if ($k == 'set-cookie') { // 更新Cookie if ($this->keepcontext) { $this->context->addCookie(new SinCookie($v)); } } else { // 添加到头里面去 $this->response->setHeader($k, $v); } } else { // 第一行数据 // 解析响应状态 $preg = '/^(\S*) (\S*) (.*)$/'; preg_match_all($preg, $h, $arr); isset ($arr[1][0]) & $this->response->scheme = trim($arr[1][0]); isset ($arr[2][0]) & $this->response->stasus = trim($arr[2][0]); isset ($arr[3][0]) & $this->response->code = trim($arr[3][0]); } } // 获取响应正文长度 $len = (int) $this->response->header['content-length']; $res = ''; // 下面的循环读取正文 while (!feof($fp) && $len > 0) { $c = fread($fp, $len); $res .= $c; $len -= strlen($c); } $this->response->body = $res; } // 关闭Socket fclose($fp); // 把返回保存到上下文维持中 $this->context->refrer = $url; } } } // demo // now let begin test it $client = new SinHttpClient(); // create a client $client->get('http://www.baidu.com/'); // get echo $client->response->body; // echo ?>
总结:以上就是本篇文的全部内容,希望能对大家的学习有所帮助。
相关推荐:
Atas ialah kandungan terperinci PHP实现简单的GET、POST、Cookie、Session等功能. Untuk maklumat lanjut, sila ikut artikel berkaitan lain di laman web China PHP!

Alat AI Hot

Undresser.AI Undress
Apl berkuasa AI untuk mencipta foto bogel yang realistik

AI Clothes Remover
Alat AI dalam talian untuk mengeluarkan pakaian daripada foto.

Undress AI Tool
Gambar buka pakaian secara percuma

Clothoff.io
Penyingkiran pakaian AI

AI Hentai Generator
Menjana ai hentai secara percuma.

Artikel Panas

Alat panas

Notepad++7.3.1
Editor kod yang mudah digunakan dan percuma

SublimeText3 versi Cina
Versi Cina, sangat mudah digunakan

Hantar Studio 13.0.1
Persekitaran pembangunan bersepadu PHP yang berkuasa

Dreamweaver CS6
Alat pembangunan web visual

SublimeText3 versi Mac
Perisian penyuntingan kod peringkat Tuhan (SublimeText3)

Topik panas


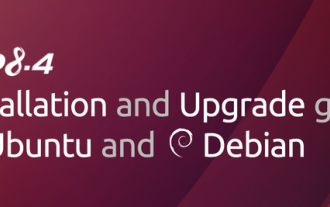
PHP 8.4 membawa beberapa ciri baharu, peningkatan keselamatan dan peningkatan prestasi dengan jumlah penamatan dan penyingkiran ciri yang sihat. Panduan ini menerangkan cara memasang PHP 8.4 atau naik taraf kepada PHP 8.4 pada Ubuntu, Debian, atau terbitan mereka
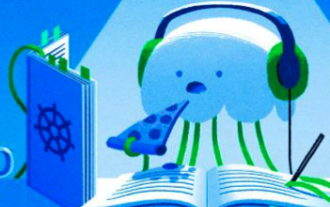
Kod Visual Studio, juga dikenali sebagai Kod VS, ialah editor kod sumber percuma — atau persekitaran pembangunan bersepadu (IDE) — tersedia untuk semua sistem pengendalian utama. Dengan koleksi sambungan yang besar untuk banyak bahasa pengaturcaraan, Kod VS boleh menjadi c
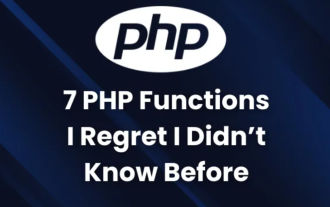
Jika anda seorang pembangun PHP yang berpengalaman, anda mungkin merasakan bahawa anda telah berada di sana dan telah melakukannya. Anda telah membangunkan sejumlah besar aplikasi, menyahpenyahpepijat berjuta-juta baris kod dan mengubah suai sekumpulan skrip untuk mencapai op
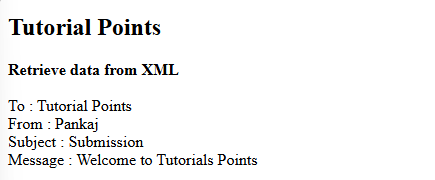
Tutorial ini menunjukkan cara memproses dokumen XML dengan cekap menggunakan PHP. XML (bahasa markup extensible) adalah bahasa markup berasaskan teks yang serba boleh yang direka untuk pembacaan manusia dan parsing mesin. Ia biasanya digunakan untuk penyimpanan data
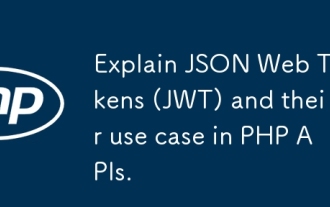
JWT adalah standard terbuka berdasarkan JSON, yang digunakan untuk menghantar maklumat secara selamat antara pihak, terutamanya untuk pengesahan identiti dan pertukaran maklumat. 1. JWT terdiri daripada tiga bahagian: header, muatan dan tandatangan. 2. Prinsip kerja JWT termasuk tiga langkah: menjana JWT, mengesahkan JWT dan muatan parsing. 3. Apabila menggunakan JWT untuk pengesahan di PHP, JWT boleh dijana dan disahkan, dan peranan pengguna dan maklumat kebenaran boleh dimasukkan dalam penggunaan lanjutan. 4. Kesilapan umum termasuk kegagalan pengesahan tandatangan, tamat tempoh, dan muatan besar. Kemahiran penyahpepijatan termasuk menggunakan alat debugging dan pembalakan. 5. Pengoptimuman prestasi dan amalan terbaik termasuk menggunakan algoritma tandatangan yang sesuai, menetapkan tempoh kesahihan dengan munasabah,
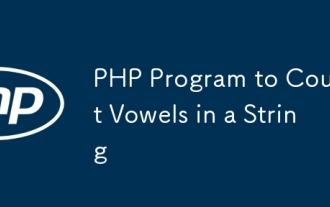
Rentetan adalah urutan aksara, termasuk huruf, nombor, dan simbol. Tutorial ini akan mempelajari cara mengira bilangan vokal dalam rentetan yang diberikan dalam PHP menggunakan kaedah yang berbeza. Vokal dalam bahasa Inggeris adalah a, e, i, o, u, dan mereka boleh menjadi huruf besar atau huruf kecil. Apa itu vokal? Vokal adalah watak abjad yang mewakili sebutan tertentu. Terdapat lima vokal dalam bahasa Inggeris, termasuk huruf besar dan huruf kecil: a, e, i, o, u Contoh 1 Input: String = "TutorialSpoint" Output: 6 menjelaskan Vokal dalam rentetan "TutorialSpoint" adalah u, o, i, a, o, i. Terdapat 6 yuan sebanyak 6
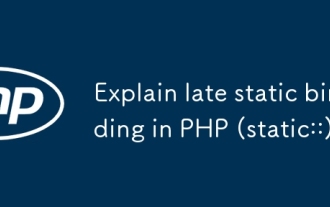
Mengikat statik (statik: :) Melaksanakan pengikatan statik lewat (LSB) dalam PHP, yang membolehkan kelas panggilan dirujuk dalam konteks statik dan bukannya menentukan kelas. 1) Proses parsing dilakukan pada masa runtime, 2) Cari kelas panggilan dalam hubungan warisan, 3) ia boleh membawa overhead prestasi.

Apakah kaedah sihir PHP? Kaedah sihir PHP termasuk: 1. \ _ \ _ Membina, digunakan untuk memulakan objek; 2. \ _ \ _ Destruct, digunakan untuk membersihkan sumber; 3. \ _ \ _ Call, mengendalikan panggilan kaedah yang tidak wujud; 4. \ _ \ _ Mendapatkan, melaksanakan akses atribut dinamik; 5. \ _ \ _ Set, melaksanakan tetapan atribut dinamik. Kaedah ini secara automatik dipanggil dalam situasi tertentu, meningkatkan fleksibiliti dan kecekapan kod.
