


Bagaimana untuk menukar data JSON ke jadual html menggunakan JavaScript/jQuery?
JSON (JavaScript Object Notation) ialah format data berkuasa yang digunakan untuk bertukar-tukar data antara pelayan dan pelanggan. Jadual HTML ialah alat yang berkuasa untuk mewakili data dalam format jadual, menjadikannya sangat mudah untuk dibaca, dianalisis dan dibandingkan. Dalam pembangunan web, adalah perkara biasa untuk menukar data JSON ke dalam jadual HTML.
Dalam artikel ini, kita akan belajar cara menukar data JSON ke dalam jadual HTML menggunakan Javascript dan jQuery. Selepas membaca artikel ini, anda akan mempunyai pemahaman yang kukuh tentang penukaran jadual JSON kepada HTML.
Tukar data JSON kepada jadual HTML menggunakan JavaScript
Berikut ialah langkah untuk membuat jadual HTML menggunakan data JSON.
Buat fungsi yang dipanggil "tukar".
Buat sampel data JSON.
Gunakan getElementByID("bekas") untuk mendapatkan bekas yang akan kami tambahkan jadual.
Dapatkan kunci objek pertama data JSON supaya kita boleh mendapatkan tajuk jadual.
Gelung nama lajur, buat sel pengepala dan tetapkan nama lajur pada teks sel pengepala.
Tambahkan sel pengepala pada baris pengepala dan kemudian tambahkan baris pengepala ke pengepala
Tambahkan tajuk pada jadual
Gelung melalui data JSON, buat baris jadual, gunakan Object.values(item) untuk mendapatkan nilai objek semasa dalam data JSON dan buat sel jadual.
Tetapkan nilai pada teks sel jadual, tambahkan sel jadual pada baris jadual, dan kemudian tambahkan baris jadual pada jadual.
Contoh
Dalam contoh ini, kami menggunakan Javascript untuk menukar data JSON kepada jadual HTML.
<html> <head> <style> table, th, td { border: 1px solid black; border-collapse: collapse; } td, th { padding: 10px; } </style> </head> <body> <h2>Convert JSON data into a html table using Javascript</h2> <p>Click the following button to convert JSON results into HTML table</p><br> <button id="btn" onclick="convert( )"> Click Here </button> <br> <h3> Resulting Table: </h3> <div id="container"></div> <script> // Function to convert JSON data to HTML table function convert() { // Sample JSON data let jsonData = [ { name: "Saurabh", age: "20", city: "Prayagraj" }, { name: "Vipin", age: 23, city: "Lucknow", }, { name: "Saksham", age: 21, city: "Noida" } ]; // Get the container element where the table will be inserted let container = document.getElementById("container"); // Create the table element let table = document.createElement("table"); // Get the keys (column names) of the first object in the JSON data let cols = Object.keys(jsonData[0]); // Create the header element let thead = document.createElement("thead"); let tr = document.createElement("tr"); // Loop through the column names and create header cells cols.forEach((item) => { let th = document.createElement("th"); th.innerText = item; // Set the column name as the text of the header cell tr.appendChild(th); // Append the header cell to the header row }); thead.appendChild(tr); // Append the header row to the header table.append(tr) // Append the header to the table // Loop through the JSON data and create table rows jsonData.forEach((item) => { let tr = document.createElement("tr"); // Get the values of the current object in the JSON data let vals = Object.values(item); // Loop through the values and create table cells vals.forEach((elem) => { let td = document.createElement("td"); td.innerText = elem; // Set the value as the text of the table cell tr.appendChild(td); // Append the table cell to the table row }); table.appendChild(tr); // Append the table row to the table }); container.appendChild(table) // Append the table to the container element } </script> </body> </html>
Contoh: Tukar data JSON kepada jadual HTML menggunakan jQuery
Berikut ialah kod untuk menukar data JSON ke dalam jadual HTML menggunakan jQuery.
<html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.3/jquery.min.js"></script> <style> table, th, td { border: 1px solid black; border-collapse: collapse; } td, th {padding: 10px;} </style> </head> <body> <h2>Convert JSON data into a html table using Jquery</h2> <p>Click the following button to convert JSON results into HTML table</p> <br> <button id="btn" onclick="convert( )"> Click Here </button> <br> <h3> Resulting Table: </h3> <div id="container"></div> <script> // Function to convert JSON data to HTML table function convert() { // Sample JSON data let jsonData = [ { name: "Saurabh", age: "20", city: "Prayagraj" }, { name: "Vipin", age: 23, city: "Lucknow", }, { name: "Saksham", age: 21, city: "Noida" } ]; // Get the container element where the table will be inserted let container = $("#container"); // Create the table element let table = $("<table>"); // Get the keys (column names) of the first object in the JSON data let cols = Object.keys(jsonData[0]); // Create the header element let thead = $("<thead>"); let tr = $("<tr>"); // Loop through the column names and create header cells $.each(cols, function(i, item){ let th = $("<th>"); th.text(item); // Set the column name as the text of the header cell tr.append(th); // Append the header cell to the header row }); thead.append(tr); // Append the header row to the header table.append(tr) // Append the header to the table // Loop through the JSON data and create table rows $.each(jsonData, function(i, item){ let tr = $("<tr>"); // Get the values of the current object in the JSON data let vals = Object.values(item); // Loop through the values and create table cells $.each(vals, (i, elem) => { let td = $("<td>"); td.text(elem); // Set the value as the text of the table cell tr.append(td); // Append the table cell to the table row }); table.append(tr); // Append the table row to the table }); container.append(table) // Append the table to the container element } </script> </body> </html>
Atas ialah kandungan terperinci Bagaimana untuk menukar data JSON ke jadual html menggunakan JavaScript/jQuery?. Untuk maklumat lanjut, sila ikut artikel berkaitan lain di laman web China PHP!

Alat AI Hot

Undresser.AI Undress
Apl berkuasa AI untuk mencipta foto bogel yang realistik

AI Clothes Remover
Alat AI dalam talian untuk mengeluarkan pakaian daripada foto.

Undress AI Tool
Gambar buka pakaian secara percuma

Clothoff.io
Penyingkiran pakaian AI

AI Hentai Generator
Menjana ai hentai secara percuma.

Artikel Panas

Alat panas

Notepad++7.3.1
Editor kod yang mudah digunakan dan percuma

SublimeText3 versi Cina
Versi Cina, sangat mudah digunakan

Hantar Studio 13.0.1
Persekitaran pembangunan bersepadu PHP yang berkuasa

Dreamweaver CS6
Alat pembangunan web visual

SublimeText3 versi Mac
Perisian penyuntingan kod peringkat Tuhan (SublimeText3)

Topik panas
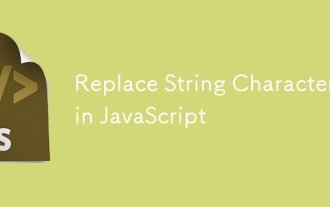
Penjelasan terperinci mengenai kaedah penggantian rentetan javascript dan Soalan Lazim Artikel ini akan meneroka dua cara untuk menggantikan watak rentetan dalam JavaScript: Kod JavaScript dalaman dan HTML dalaman untuk laman web. Ganti rentetan di dalam kod JavaScript Cara yang paling langsung ialah menggunakan kaedah pengganti (): str = str.replace ("cari", "ganti"); Kaedah ini hanya menggantikan perlawanan pertama. Untuk menggantikan semua perlawanan, gunakan ungkapan biasa dan tambahkan bendera global g: str = str.replace (/fi
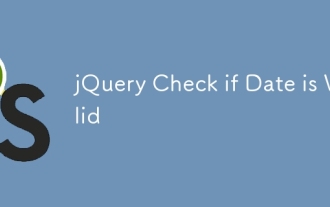
Fungsi JavaScript mudah digunakan untuk memeriksa sama ada tarikh sah. fungsi isvaliddate (s) { var bits = s.split ('/'); var d = tarikh baru (bit [2] '/' bits [1] '/' bits [0]); kembali !! (d && (d.getmonth () 1) == bit [1] && d.getdate () == nombor (bit [0])); } // ujian var
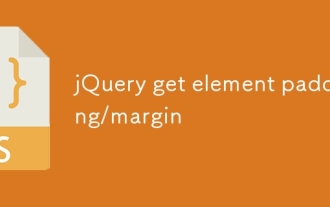
Artikel ini membincangkan cara menggunakan jQuery untuk mendapatkan dan menetapkan margin dalaman dan nilai margin elemen DOM, terutama lokasi tertentu margin luar dan margin dalaman elemen. Walaupun ada kemungkinan untuk menetapkan margin dalaman dan luar elemen menggunakan CSS, nilai yang tepat boleh menjadi rumit. // Sediakan $ ("div.header"). css ("margin", "10px"); $ ("div.header"). css ("padding", "10px"); Anda mungkin menganggap kod ini
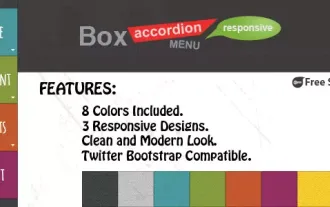
Artikel ini meneroka sepuluh tab jQuery yang luar biasa dan akordion. Perbezaan utama antara tab dan akordion terletak pada bagaimana panel kandungan mereka dipaparkan dan tersembunyi. Mari kita menyelidiki sepuluh contoh ini. Artikel Berkaitan: 10 JQuery Tab Plugin
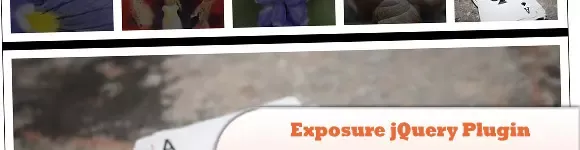
Temui sepuluh plugin jQuery yang luar biasa untuk meningkatkan dinamisme dan daya tarikan visual laman web anda! Koleksi ini menawarkan pelbagai fungsi, dari animasi imej ke galeri interaktif. Mari kita meneroka alat yang berkuasa ini: Posting Berkaitan: 1
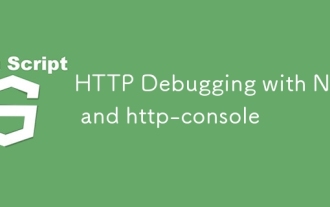
HTTP-CONSOLE adalah modul nod yang memberi anda antara muka baris arahan untuk melaksanakan arahan HTTP. Ia bagus untuk menyahpepijat dan melihat apa yang sedang berlaku dengan permintaan HTTP anda, tanpa mengira sama ada mereka dibuat terhadap pelayan web, Serv Web
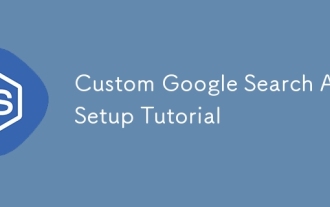
Tutorial ini menunjukkan kepada anda bagaimana untuk mengintegrasikan API carian Google tersuai ke dalam blog atau laman web anda, menawarkan pengalaman carian yang lebih halus daripada fungsi carian tema WordPress standard. Ia menghairankan mudah! Anda akan dapat menyekat carian ke y
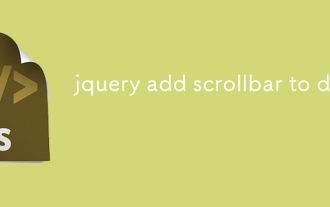
Coretan kod jQuery berikut boleh digunakan untuk menambah bar skrol apabila kandungan div melebihi kawasan elemen kontena. (Tiada demonstrasi, sila salin terus ke Firebug) // d = dokumen // w = tetingkap // $ = jQuery var contentArea = $ (ini), Wintop = contentArea.scrollTop (), docheight = $ (d) .height (), winheight = $ (w) .height (), Divheight = $ ('#c
