
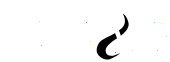
PHP 8.3 ialah kemas kini utama bahasa PHP.
Ia mengandungi banyak ciri baharu, seperti penaipan eksplisit pemalar kelas, pengklonan dalam sifat baca sahaja dan penambahan pada fungsi rawak. Seperti biasa ia juga termasuk peningkatan prestasi, pembetulan pepijat dan pembersihan umum.
Naik taraf kepada PHP 8.3 sekarang!Pemalar kelas ditaip
interface
I
{
// We may naively assume that the PHP constant is always a string.
const
PHP
=
'PHP 8.2'
;
}
class
Foo
implements
I
{
// But implementing classes may define it as an array.
const
PHP
=
[];
}

interface
I
{
const
string PHP
=
'PHP 8.2'
;
}
class
Foo
implements
I
{
const
string PHP
=
[];
}
// Fatal error: Cannot use array as value for class constant
// Foo::PHP of type string
Pengambilan tetap kelas dinamik
class
Foo
{
const
PHP
=
'PHP 8.2'
;
}
$searchableConstant
=
'PHP'
;
var_dump
(
constant
(
Foo
::class .
"::
{
$searchableConstant
}
"
));

class
Foo
{
const
PHP
=
'PHP 8.3'
;
}
$searchableConstant
=
'PHP'
;
var_dump
(
Foo
::
{
$searchableConstant
}
);
New #[Override] attribute
use
PHPUnit\Framework\TestCase
;
final class
MyTest
extends
TestCase
{
protected
$logFile
;
protected function
setUp
():
void
{
$this
->
logFile
=
fopen
(
'/tmp/logfile'
,
'w'
);
}
protected function
taerDown
():
void
{
fclose
(
$this
->
logFile
);
unlink
(
'/tmp/logfile'
);
}
}
// The log file will never be removed, because the
// method name was mistyped (taerDown vs tearDown).

use
PHPUnit\Framework\TestCase
;
final class
MyTest
extends
TestCase
{
protected
$logFile
;
protected function
setUp
():
void
{
$this
->
logFile
=
fopen
(
'/tmp/logfile'
,
'w'
);
}
#[
\Override
]
protected function
taerDown
():
void
{
fclose
(
$this
->
logFile
);
unlink
(
'/tmp/logfile'
);
}
}
// The log file will never be removed, because the
// method name was mistyped (taerDown vs tearDown).
By adding the #[Override] attribute to a method, PHP will ensure that a method with the same name exists in a parent class or in an implemented interface. Adding the attribute makes it clear that overriding a parent method is intentional and simplifies refactoring, because the removal of an overridden parent method will be detected.
Pengklonan mendalam bagi sifat baca sahaja
class
PHP
{
public
string $version
=
'8.2'
;
}
readonly class
Foo
{
public function
__construct
(
public
PHP $php
) {}
public function
__clone
():
void
{
$this
->
php
= clone
$this
->
php
;
}
}
$instance
= new
Foo
(new
PHP
());
$cloned
= clone
$instance
;
// Fatal error: Cannot modify readonly property Foo::$php

class
PHP
{
public
string $version
=
'8.2'
;
}
readonly class
Foo
{
public function
__construct
(
public
PHP $php
) {}
public function
__clone
():
void
{
$this
->
php
= clone
$this
->
php
;
}
}
$instance
= new
Foo
(new
PHP
());
$cloned
= clone
$instance
;
$cloned
->
php
->
version
=
'8.3'
;
sifat baca sahaja kini boleh diubah suai sekali dalam kaedah __klon ajaib untuk membolehkan pengklonan dalam sifat baca sahaja.
Fungsi json_validate() baharu
function
json_validate
(
string $string
):
bool
{
json_decode
(
$string
);
return
json_last_error
() ===
JSON_ERROR_NONE
;
}
var_dump
(
json_validate
(
'{ "test": { "foo": "bar" }
}'
));
// true

var_dump
(
json_validate
(
'{ "test": { "foo": "bar" }
}'
));
// true
json_validate() membenarkan untuk menyemak sama ada rentetan adalah JSON sah dari segi sintaksis, sambil lebih cekap daripada json_decode().
Kaedah Randomizer::getBytesFromString() baharu
// This function needs to be manually implemented.
function
getBytesFromString
(
string $string
,
int
$length
) {
$stringLength
=
strlen
(
$string
);
$result
=
''
;
for (
$i
=
0
;
$i
<
$length
;
$i
++) {
// random_int is not seedable for testing, but secure.
$result
.=
$string
[
random_int
(
0
,
$stringLength
-
1
)];
}
return
$result
;
}
$randomDomain
=
sprintf
(
"%s.example.com"
,
getBytesFromString
(
'abcdefghijklmnopqrstuvwxyz0123456789'
,
16
,
),
);
echo
$randomDomain
;

// A \Random\Engine may be passed for seeding,
// the default is the secure engine.
$randomizer
= new
\Random\Randomizer
();
$randomDomain
=
sprintf
(
"%s.example.com"
,
$randomizer
->
getBytesFromString
(
'abcdefghijklmnopqrstuvwxyz0123456789'
,
16
,
),
);
echo
$randomDomain
;
Sambungan Rawak yang telah ditambahkan dalam PHP 8.2 telah dilanjutkan dengan kaedah baharu untuk menjana rentetan rawak yang terdiri daripada bait tertentu sahaja. Kaedah ini membolehkan pembangun menjana pengecam rawak dengan mudah, seperti nama domain dan rentetan berangka dengan panjang arbitrari.
Kaedah Randomizer::getFloat() dan Randomizer::nextFloat() baharu
// Returns a random float between $min and $max, both including.
function
getFloat
(
float $min
,
float $max
) {
// This algorithm is biased for specific inputs and may
// return values outside the given range. This is impossible
// to work around in userland.
$offset
=
random_int
(
0
,
PHP_INT_MAX
) /
PHP_INT_MAX
;
return
$offset
* (
$max
-
$min
) +
$min
;
}
$temperature
=
getFloat
(-
89.2
,
56.7
);
$chanceForTrue
=
0.1
;
// getFloat(0, 1) might return the upper bound, i.e. 1,
// introducing a small bias.
$myBoolean
=
getFloat
(
0
,
1
) <
$chanceForTrue
;

$randomizer
= new
\Random\Randomizer
();
$temperature
=
$randomizer
->
getFloat
(
-89.2
,
56.7
,
\Random\IntervalBoundary
::
ClosedClosed
,
);
$chanceForTrue
=
0.1
;
// Randomizer::nextFloat() is equivalent to
// Randomizer::getFloat(0, 1, \Random\IntervalBoundary::ClosedOpen).
// The upper bound, i.e. 1, will not be returned.
$myBoolean
=
$randomizer
->
nextFloat
() <
$chanceForTrue
;
Disebabkan ketepatan terhad dan pembundaran tersirat nombor titik terapung, menghasilkan apungan tidak berat sebelah yang terletak dalam selang waktu tertentu adalah bukan remeh dan penyelesaian userland yang biasa digunakan boleh menjana hasil atau nombor yang berat sebelah di luar julat yang diminta.
Randomizer juga diperluaskan dengan dua kaedah untuk menjana terapung rawak dengan cara yang tidak berat sebelah. Kaedah Randomizer::getFloat() menggunakan algoritma bahagian γ yang diterbitkan dalam Melukis Nombor Titik Terapung Rawak dari Selang. Frédéric Goualard, ACM Trans. Model. Pengiraan. Simul., 32:3, 2022.
Linter baris arahan menyokong berbilang fail
php -l foo.php bar.php
No syntax errors detected in foo.php

php -l foo.php bar.php
No syntax errors detected in foo.php
No syntax errors detected in bar.php
Linter baris arahan kini menerima input variadic untuk nama fail untuk lint
Kelas, Antara Muka dan Fungsi Baharu
- DOMElement Baharu::getAttributeNames(), DOMElement::insertAdjacentElement(), DOMElement::insertAdjacentText(), DOMElement::toggleAttribute(), DOMNode::contains(), DOMNode::getRootNode(), DOMNode::isEqualNode(), DOMNameSpaceNode::contains(), dan kaedah DOMParentNode::replaceChildren().
- Kaedah IntlCalendar::setDate(), IntlCalendar::setDateTime(), IntlGregorianCalendar::createFromDate(), dan IntlGregorianCalendar::createFromDateTime().
- Fungsi ldap_connect_wallet(), dan ldap_exop_sync() baharu.
- Fungsi mb_str_pad() baharu.
- Fungsi posix_sysconf(), posix_pathconf(), posix_fpathconf(), dan posix_eaccess().
- Kaedah ReflectionMethod::createFromMethodName() kaedah.
- Fungsi socket_atmark() baharu.
- Fungsi str_increment(), str_decrement(), dan stream_context_set_options().
- Kaedah ZipArchive::getArchiveFlag() baharu.
- Support for generation EC keys with custom EC parameters in OpenSSL extension.
- Tetapan INI baharu zend.max_allowed_stack_size untuk menetapkan saiz tindanan maksimum yang dibenarkan.
- php.ini kini menyokong sintaks nilai sandaran/lalai.
- Kelas tanpa nama kini boleh dibaca sahaja.
Penamatan dan pemecahan keserasian ke belakang
- Pengecualian Tarikh/Masa Lebih Sesuai.
- Menetapkan indeks negatif n kepada tatasusunan kosong kini akan memastikan bahawa indeks seterusnya ialah n 1 dan bukannya 0.
- Perubahan kepada fungsi julat().
- Changes in re-declaration of static properties in traits.
- Pemalar U_MULTIPLE_DECIMAL_SEPERATORS ditamatkan dan memihak kepada U_MULTIPLE_DECIMAL_SEPARATORS.
- Varian MT_RAND_PHP Mt19937 tidak digunakan lagi.
- ReflectionClass::getStaticProperties() tidak lagi boleh dibatalkan.
- Tetapan INI assert.active, assert.bail, assert.callback, assert.exception dan assert.warning telah ditamatkan.
- Memanggil get_class() dan get_parent_class() tanpa hujah tidak digunakan lagi.
- SQLite3: Mod ralat lalai ditetapkan kepada pengecualian.
Panduan migrasi tersedia dalam Manual PHP. Sila rujuknya untuk mendapatkan senarai terperinci ciri baharu dan perubahan yang tidak serasi ke belakang.
Jika anda perlu memuat turun versi sebelumnya, anda boleh melihat Lagi ChangeLog