PHP finds common multiples of consecutive numbers
Jul 06, 2016 pm 01:51 PM
Why are there 60 minutes in an hour instead of 100 minutes? This is caused by historical habits. But it's not purely accidental: 60 is an excellent number, and it has many factors.
In fact, it is a multiple of every number from 1 to 6. That is, 1,2,3,4,5,6 can all divide 60.We want to find the smallest integer that can divide every number from 1 to n.
Don’t underestimate this number, it may be very large, for example, n=100, then the number is:
69720375229712477164533808935312303556800Please write a program to find the least common multiple of 1~n for n (n<100) input by the user.
For example: User input: 6 Program output: 60
User input: 10 Program output: 2520
Please use PHP to implement this method. It is best if you can explain the idea first
Reply content:
Why are there 60 minutes in an hour instead of 100 minutes? This is caused by historical habits. But it's not purely accidental: 60 is an excellent number, and it has many factors.
In fact, it is a multiple of every number from 1 to 6. That is, 1,2,3,4,5,6 can all divide 60.We want to find the smallest integer that can divide every number from 1 to n.
Don’t underestimate this number, it may be very large, for example, n=100, then the number is:
69720375229712477164533808935312303556800Please write a program to find the least common multiple of 1~n for n (n<100) input by the user.
For example: User input: 6 Program output: 60
User input: 10 Program output: 2520
Please use PHP to implement this method. It is best if you can explain the idea first
The general idea is: first find the least common multiple of two numbers (in which the euclidean division method is used to find the greatest common divisor of the two numbers, and then use the formula to get the least common multiple), then find the least common multiple of the next number, and so on Until the last number. .
The code is as follows: (It should be noted that if the int type of php is too large, it will cause overflow)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
|

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
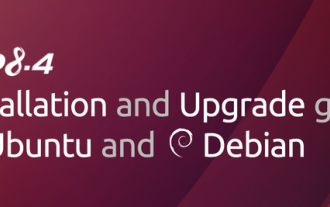
PHP 8.4 Installation and Upgrade guide for Ubuntu and Debian
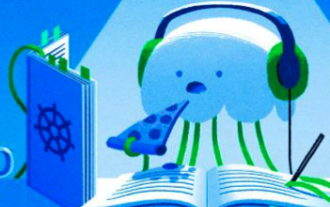
How To Set Up Visual Studio Code (VS Code) for PHP Development
