


Yii learning summary data access object (DAO), yiidao_PHP tutorial
Yii Learning Summary Data Access Object (DAO), yiidao
Yii provides powerful database programming support. Yii Data Access Object (DAO) is built on the PHP Data Object (PDO) extension, allowing access to different database management systems (DBMS) through a single unified interface. Applications developed using Yii's DAO can easily switch to use different database management systems without modifying the data access code.
Data Access Object (DAO) provides a common API for accessing data stored in different database management systems (DBMS). Therefore, when changing the underlying DBMS to another, there is no need to modify the code that uses DAO to access data.
Yii DAO is built on PHP Data Objects (PDO). It is an extension that provides unified data access for many popular DBMS, including MySQL, PostgreSQL, etc. Therefore, to use Yii DAO, the PDO extension and specific PDO database driver (such as PDO_MYSQL) must be installed.
Yii DAO mainly includes the following four categories:
CDbConnection: Represents a database connection.
CDbCommand: Represents a SQL statement executed through the database.
CDbDataReader: Represents a forward-only stream of rows from a query result set.
CDbTransaction: Represents a database transaction.
Below, we introduce the application of Yii DAO in different scenarios.
1. Establish database connection
To establish a database connection, create a CDbConnection instance and activate it. Connecting to a database requires a data source name (DSN) to specify connection information. A username and password may also be used. When an error occurs while connecting to the database (for example, wrong DSN or invalid username/password), an exception will be thrown.
$connection=new CDbConnection($dsn,$username,$password);
// Establish a connection. You can use try...catch to catch exceptions that may be thrown
$connection->active=true;
......
$connection->active=false; // Close connection
The format of the DSN depends on the PDO database driver used. In general, the DSN contains the name of the PDO driver, followed by a colon, followed by driver-specific connection syntax. Check out the PDO documentation for more information. Below is a list of commonly used DSN formats.
SQLite: sqlite:/path/to/dbfile
MySQL: mysql:host=localhost;dbname=testdb
PostgreSQL: pgsql:host=localhost;port=5432;dbname=testdb
SQL Server: mssql:host=localhost;dbname=testdb
Oracle: oci:dbname=//localhost:1521/testdb
Since CDbConnection inherits from CApplicationComponent, we can also use it as an application component. To do this, configure a db (or other name) application component in the application configuration as follows:
array(
…
'components'=>array(
… …
'db'=>array(
'class'=>'CDbConnection',
'connectionString'=>'mysql:host=localhost;dbname=testdb',
'username'=>'root',
'password'=>'password',
'emulatePrepare'=>true, // needed by some MySQL installations
),
),
)
Then we can access the database connection through Yii::app()->db. It is automatically activated unless we specifically configure CDbConnection::autoConnect to false. This way, this single DB connection can be shared in many places in our code.
2. Execute SQL statement
After the database connection is established, SQL statements can be executed by using CDbCommand. You create a CDbCommand instance by calling CDbConnection::createCommand() with the specified SQL statement as argument.
$connection=Yii::app()->db; // Assume you have established a "db" connection
// If not, you may need to explicitly establish a connection:
// $connection=new CDbConnection($dsn,$username,$password);
$command=$connection->createCommand($sql);
// If necessary, this SQL statement can be modified as follows:
// $command->text=$newSQL;
A SQL statement will be executed through CDbCommand in the following two ways:
execute(): Execute a non-query SQL statement, such as INSERT, UPDATE and DELETE. If successful, it returns the number of rows affected by this execution.
query(): Execute a SQL statement that returns several rows of data, such as SELECT. If successful, it returns a CDbDataReader instance through which the resulting rows of data can be iterated. For simplicity, (Yii) also implements a series of queryXXX() methods to directly return query results.
If an error occurs while executing the SQL statement, an exception will be thrown.
$rowCount=$command->execute(); // Execute no query SQL
$dataReader=$command->query(); // Execute a SQL query
$rows=$command->queryAll(); // Query and return all rows in the result
$row=$command->queryRow(); // Query and return the first row in the result
$column=$command->queryColumn(); // Query and return the first column in the result
$value=$command->queryScalar(); // Query and return the first field of the first row in the result
3. Get query results
After CDbCommand::query() generates a CDbDataReader instance, you can obtain rows in the result by repeatedly calling CDbDataReader::read(). You can also use CDbDataReader in PHP's foreach language construct to retrieve data row by row.
$dataReader=$command->query();
// Call read() repeatedly until it returns false
while(($row=$dataReader->read())!==false) { ... }
// Use foreach to iterate through each row in the data
foreach($dataReader as $row) { ... }
// Extract all rows into an array at once
$rows=$dataReader->readAll();
Note: Unlike query(), all queryXXX() methods will return data directly. For example, queryRow() returns an array representing the first row of the query results.
4. Using transactions
When an application executes several queries, each of which reads information from and/or writes information to the database, it is important to ensure that the database does not leave several queries behind while only executing others. Transactions, represented in Yii as CDbTransaction instances, may be initiated in the following situations:
Start transaction.
Execute queries one by one. Any updates to the database are not visible to the outside world.
Commit the transaction. If the transaction succeeds, the update becomes visible.
If one of the queries fails, the entire transaction is rolled back.
The above workflow can be implemented through the following code:
$transaction=$connection->beginTransaction();
try
{
$connection->createCommand($sql1)->execute();
$connection->createCommand($sql2)->execute();
//.... other SQL executions
$transaction->commit();
}
catch(Exception $e) // If a query fails, an exception will be thrown
{
$transaction->rollBack();
}
5. Binding parameters
To avoid SQL injection attacks and improve the efficiency of repeatedly executing SQL statements, you can "prepare" a SQL statement with optional parameter placeholders. When the parameters are bound, these placeholders will be replaced with actual parameters.
Parameter placeholders can be named (appear as a unique token) or unnamed (appear as a question mark). Call CDbCommand::bindParam() or CDbCommand::bindValue() to replace these placeholders with actual parameters. These parameters do not need to be enclosed in quotes: the underlying database driver takes care of this for you. Parameter binding must be completed before the SQL statement is executed.
// A SQL
with two placeholders ":username" and ":email" $sql="INSERT INTO tbl_user (username, email) VALUES(:username,:email)";
$command=$connection->createCommand($sql);
// Replace the placeholder ":username" with the actual username
$command->bindParam(":username",$username,PDO::PARAM_STR);
// Replace the placeholder ":email" with the actual Email
$command->bindParam(":email",$email,PDO::PARAM_STR);
$command->execute();
// Insert another row with new parameter set
$command->bindParam(":username",$username2,PDO::PARAM_STR);
$command->bindParam(":email",$email2,PDO::PARAM_STR);
$command->execute();
Methods bindParam() and bindValue() are very similar. The only difference is that the former uses a PHP variable to bind the parameter, while the latter uses a value. For those parameters with large data blocks in memory, for performance reasons, the former should be used first.
For more information about binding parameters, please refer to the relevant PHP documentation.
6. Binding columns
You can also use PHP variables to bind columns when getting query results. This will automatically fill in the latest value every time a row in the query results is obtained.
$sql="SELECT username, email FROM tbl_user";
$dataReader=$connection->createCommand($sql)->query();
// Use the $username variable to bind the first column (username)
$dataReader->bindColumn(1,$username);
// Use the $email variable to bind the second column (email)
$dataReader->bindColumn(2,$email);
while($dataReader->read()!==false)
{
// $username and $email contain the username and email in the current line
}
7. Use table prefixes
Starting from version 1.1.0, Yii provides integrated support for using table prefixes. The table prefix refers to a string added in front of the name of the data table in the currently connected database. It is often used in shared server environments, where multiple applications may share the same database and use different table prefixes to distinguish them from each other. For example, one application can use tbl_ as a table prefix while another can use yii_.
To use a table prefix, configure the CDbConnection::tablePrefix property to the desired table prefix. Then, use {{TableName}} to represent the name of the table in the SQL statement, where TableName refers to the table name without the prefix. For example, if the database contains a table named tbl_user, and tbl_ is configured as the table prefix, then we can use the following code to perform user-related queries:
$sql='SELECT * FROM {{user}}';
$users=$connection->createCommand($sql)->queryAll();

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










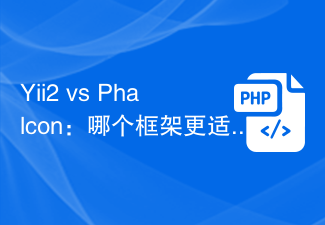
In the current information age, big data, artificial intelligence, cloud computing and other technologies have become the focus of major enterprises. Among these technologies, graphics card rendering technology, as a high-performance graphics processing technology, has received more and more attention. Graphics card rendering technology is widely used in game development, film and television special effects, engineering modeling and other fields. For developers, choosing a framework that suits their projects is a very important decision. Among current languages, PHP is a very dynamic language. Some excellent PHP frameworks such as Yii2, Ph
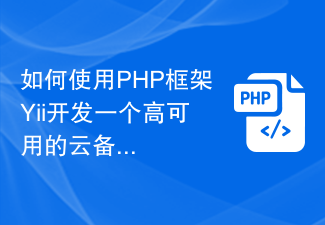
With the continuous development of cloud computing technology, data backup has become something that every enterprise must do. In this context, it is particularly important to develop a highly available cloud backup system. The PHP framework Yii is a powerful framework that can help developers quickly build high-performance web applications. The following will introduce how to use the Yii framework to develop a highly available cloud backup system. Designing the database model In the Yii framework, the database model is a very important part. Because the data backup system requires a lot of tables and relationships
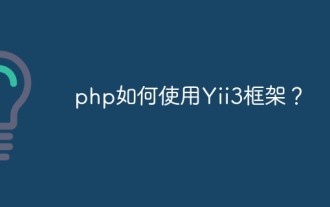
As the Internet continues to develop, the demand for web application development is also getting higher and higher. For developers, developing applications requires a stable, efficient, and powerful framework, which can improve development efficiency. Yii is a leading high-performance PHP framework that provides rich features and good performance. Yii3 is the next generation version of the Yii framework, which further optimizes performance and code quality based on Yii2. In this article, we will introduce how to use Yii3 framework to develop PHP applications.
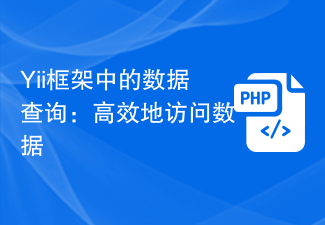
The Yii framework is an open source PHP Web application framework that provides numerous tools and components to simplify the process of Web application development, of which data query is one of the important components. In the Yii framework, we can use SQL-like syntax to access the database to query and manipulate data efficiently. The query builder of the Yii framework mainly includes the following types: ActiveRecord query, QueryBuilder query, command query and original SQL query
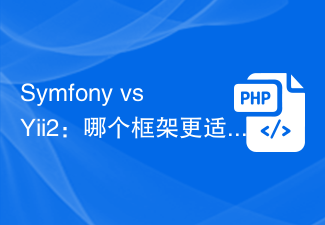
As the demand for web applications continues to grow, developers have more and more choices in choosing development frameworks. Symfony and Yii2 are two popular PHP frameworks. They both have powerful functions and performance, but when faced with the need to develop large-scale web applications, which framework is more suitable? Next we will conduct a comparative analysis of Symphony and Yii2 to help you make a better choice. Basic Overview Symphony is an open source web application framework written in PHP and is built on
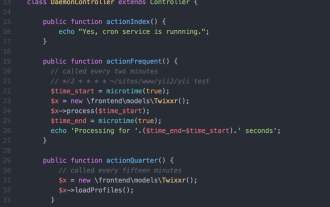
If you're asking "What is Yii?" check out my previous tutorial: Introduction to the Yii Framework, which reviews the benefits of Yii and outlines what's new in Yii 2.0, released in October 2014. Hmm> In this Programming with Yii2 series, I will guide readers in using the Yii2PHP framework. In today's tutorial, I will share with you how to leverage Yii's console functionality to run cron jobs. In the past, I've used wget - a web-accessible URL - in a cron job to run my background tasks. This raises security concerns and has some performance issues. While I discussed some ways to mitigate the risk in our Security for Startup series, I had hoped to transition to console-driven commands
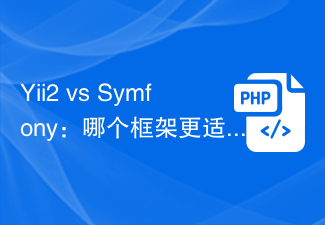
With the rapid development of the Internet, APIs have become an important way to exchange data between various applications. Therefore, it has become increasingly important to develop an API framework that is easy to maintain, efficient, and stable. When choosing an API framework, Yii2 and Symfony are two popular choices among developers. So, which one is more suitable for API development? This article will compare these two frameworks and give some conclusions. 1. Basic introduction Yii2 and Symfony are mature PHP frameworks with corresponding extensions that can be used to develop
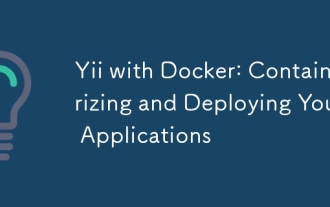
The steps to containerize and deploy Yii applications using Docker include: 1. Create a Dockerfile and define the image building process; 2. Use DockerCompose to launch Yii applications and MySQL database; 3. Optimize image size and performance. This involves not only specific technical operations, but also understanding the working principles and best practices of Dockerfile to ensure efficient and reliable deployment.
